Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / ComboBoxAutomationPeer.cs / 1305600 / ComboBoxAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Threading; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class ComboBoxAutomationPeer: SelectorAutomationPeer, IValueProvider, IExpandCollapseProvider { /// public ComboBoxAutomationPeer(ComboBox owner): base(owner) {} /// override protected ItemAutomationPeer CreateItemAutomationPeer(object item) { // Use the same peer as ListBox return new ListBoxItemAutomationPeer(item, this); } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.ComboBox; } /// override protected string GetClassNameCore() { return "ComboBox"; } /// override public object GetPattern(PatternInterface pattern) { object iface = null; if (pattern == PatternInterface.Value) { ComboBox owner = (ComboBox)Owner; if (owner.IsEditable) iface = this; } else if(pattern == PatternInterface.ExpandCollapse) { iface = this; } else { iface = base.GetPattern(pattern); } return iface; } /// protected override ListGetChildrenCore() { List children = base.GetChildrenCore(); // include text box part into children collection ComboBox owner = (ComboBox)Owner; TextBox textBox = owner.EditableTextBoxSite; if (textBox != null) { AutomationPeer peer = UIElementAutomationPeer.CreatePeerForElement(textBox); if (peer != null) { if (children == null) children = new List (); children.Insert(0, peer); } } return children; } /// protected override void SetFocusCore() { ComboBox owner = (ComboBox)Owner; if (owner.Focusable) { if (!owner.Focus()) { //The fox might have went to the TextBox inside Combobox if it is editable. if (owner.IsEditable) { TextBox tb = owner.EditableTextBoxSite; if (tb == null || !tb.IsKeyboardFocused) throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } else throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } } else { throw new InvalidOperationException(SR.Get(SRID.SetFocusFailed)); } } /// internal void ScrollItemIntoView(object item) { // Item can be scrolled into view only when the Combo is expanded. // if(((IExpandCollapseProvider)this).ExpandCollapseState == ExpandCollapseState.Expanded) { ComboBox owner = (ComboBox)Owner; if (owner.ItemContainerGenerator.Status == GeneratorStatus.ContainersGenerated) { owner.OnBringItemIntoView(item); } else { // The items aren't generated, try at a later time Dispatcher.BeginInvoke(DispatcherPriority.Loaded, new DispatcherOperationCallback(owner.OnBringItemIntoView), item); } } } #region Value /// /// Request to set the value that this UI element is representing /// /// Value to set the UI to, as an object ///true if the UI element was successfully set to the specified value //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 void IValueProvider.SetValue(string val) { if (val == null) throw new ArgumentNullException("val"); ComboBox owner = (ComboBox)Owner; if (!owner.IsEnabled) throw new ElementNotEnabledException(); owner.SetCurrentValueInternal(ComboBox.TextProperty, val); } ///Value of a value control, as a a string. string IValueProvider.Value { get { return ((ComboBox)(((ComboBoxAutomationPeer)this).Owner)).Text; } } ///Indicates that the value can only be read, not modified. ///returns True if the control is read-only bool IValueProvider.IsReadOnly { get { ComboBox owner = (ComboBox)Owner; return !owner.IsEnabled || owner.IsReadOnly; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseValuePropertyChangedEvent(string oldValue, string newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.ValueProperty, oldValue, newValue); } } #endregion Value #region ExpandCollapse ////// Blocking method that returns after the element has been expanded. /// ///true if the node was successfully expanded void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.SetCurrentValueInternal(ComboBox.IsDropDownOpenProperty, MS.Internal.KnownBoxes.BooleanBoxes.TrueBox); } ////// Blocking method that returns after the element has been collapsed. /// ///true if the node was successfully collapsed void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; owner.SetCurrentValueInternal(ComboBox.IsDropDownOpenProperty, MS.Internal.KnownBoxes.BooleanBoxes.FalseBox); } ///indicates an element's current Collapsed or Expanded state ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { ComboBox owner = (ComboBox)((ComboBoxAutomationPeer)this).Owner; return owner.IsDropDownOpen ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #endregion ExpandCollapse } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
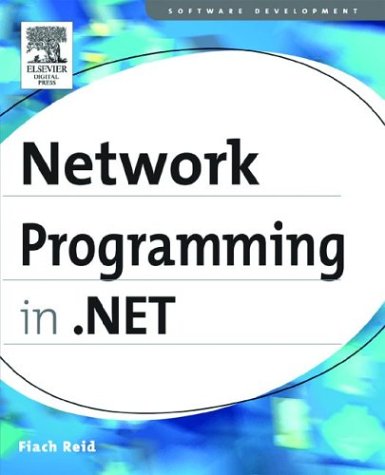
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TopClause.cs
- QueryOpcode.cs
- XmlNamespaceDeclarationsAttribute.cs
- DataServiceHostFactory.cs
- ContentElementAutomationPeer.cs
- TempFiles.cs
- PolyBezierSegment.cs
- ConfigXmlWhitespace.cs
- XmlLoader.cs
- securitycriticaldata.cs
- DataGridViewRowHeaderCell.cs
- GenericWebPart.cs
- XmlEnumAttribute.cs
- ItemsChangedEventArgs.cs
- UrlAuthFailedErrorFormatter.cs
- StyleSelector.cs
- ScrollData.cs
- Size3D.cs
- EntityAdapter.cs
- FontDriver.cs
- indexingfiltermarshaler.cs
- ConfigXmlSignificantWhitespace.cs
- WCFBuildProvider.cs
- RootAction.cs
- FileNotFoundException.cs
- ValidationSummary.cs
- OperationCanceledException.cs
- SolidColorBrush.cs
- MetadataSource.cs
- TextModifierScope.cs
- Int16Converter.cs
- XmlLanguage.cs
- ChannelProtectionRequirements.cs
- XmlSequenceWriter.cs
- DataControlLinkButton.cs
- ColumnTypeConverter.cs
- EncoderParameters.cs
- IPGlobalProperties.cs
- RIPEMD160.cs
- SqlUserDefinedTypeAttribute.cs
- DefaultPropertiesToSend.cs
- InputReferenceExpression.cs
- MethodCallTranslator.cs
- SafeNativeMethods.cs
- XmlReflectionMember.cs
- MultilineStringConverter.cs
- CellLabel.cs
- EventManager.cs
- RoutedEvent.cs
- DefaultPrintController.cs
- SQLBytesStorage.cs
- SourceItem.cs
- TraceHandlerErrorFormatter.cs
- CalendarDay.cs
- RegexBoyerMoore.cs
- MethodBuilder.cs
- AssociationTypeEmitter.cs
- __ComObject.cs
- PlatformCulture.cs
- JournalEntryStack.cs
- UseLicense.cs
- HttpPostServerProtocol.cs
- ConfigXmlWhitespace.cs
- ValueConversionAttribute.cs
- CollectionViewProxy.cs
- InstanceDescriptor.cs
- FormClosingEvent.cs
- Repeater.cs
- SigningDialog.cs
- ResourceAttributes.cs
- SelectionUIService.cs
- ZipIOExtraFieldElement.cs
- PropertyBuilder.cs
- ProjectionCamera.cs
- InputProcessorProfilesLoader.cs
- PiiTraceSource.cs
- PkcsMisc.cs
- GraphicsState.cs
- TemplateKey.cs
- HtmlInputControl.cs
- CircleHotSpot.cs
- DocumentSequence.cs
- GuidelineCollection.cs
- RSAPKCS1KeyExchangeFormatter.cs
- NullableLongMinMaxAggregationOperator.cs
- OleDbConnectionInternal.cs
- XmlBindingWorker.cs
- LinkedList.cs
- XmlSchemaParticle.cs
- ApplicationFileCodeDomTreeGenerator.cs
- Encoder.cs
- LocatorGroup.cs
- ThreadStaticAttribute.cs
- InputLanguageCollection.cs
- TextTreeInsertElementUndoUnit.cs
- TextHidden.cs
- DecoratedNameAttribute.cs
- Compiler.cs
- CompilerScope.cs
- DoubleStorage.cs