Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / PixelFormats.cs / 1305600 / PixelFormats.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: PixelFormats.cs // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; namespace System.Windows.Media { #region PixelFormats ////// PixelFormats - The collection of supported Pixel Formats /// public static class PixelFormats { ////// Default: for situations when the pixel format may not be important /// public static PixelFormat Default { get { return new PixelFormat(PixelFormatEnum.Default); } } ////// Indexed1: Paletted image with 2 colors. /// public static PixelFormat Indexed1 { get { return new PixelFormat(PixelFormatEnum.Indexed1); } } ////// Indexed2: Paletted image with 4 colors. /// public static PixelFormat Indexed2 { get { return new PixelFormat(PixelFormatEnum.Indexed2); } } ////// Indexed4: Paletted image with 16 colors. /// public static PixelFormat Indexed4 { get { return new PixelFormat(PixelFormatEnum.Indexed4); } } ////// Indexed8: Paletted image with 256 colors. /// public static PixelFormat Indexed8 { get { return new PixelFormat(PixelFormatEnum.Indexed8); } } ////// BlackWhite: Monochrome, 2-color image, black and white only. /// public static PixelFormat BlackWhite { get { return new PixelFormat(PixelFormatEnum.BlackWhite); } } ////// Gray2: Image with 4 shades of gray /// public static PixelFormat Gray2 { get { return new PixelFormat(PixelFormatEnum.Gray2); } } ////// Gray4: Image with 16 shades of gray /// public static PixelFormat Gray4 { get { return new PixelFormat(PixelFormatEnum.Gray4); } } ////// Gray8: Image with 256 shades of gray /// public static PixelFormat Gray8 { get { return new PixelFormat(PixelFormatEnum.Gray8); } } ////// Bgr555: 16 bpp SRGB format /// public static PixelFormat Bgr555 { get { return new PixelFormat(PixelFormatEnum.Bgr555); } } ////// Bgr565: 16 bpp SRGB format /// public static PixelFormat Bgr565 { get { return new PixelFormat(PixelFormatEnum.Bgr565); } } ////// Rgb128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb128Float { get { return new PixelFormat(PixelFormatEnum.Rgb128Float); } } ////// Bgr24: 24 bpp SRGB format /// public static PixelFormat Bgr24 { get { return new PixelFormat(PixelFormatEnum.Bgr24); } } ////// Rgb24: 24 bpp SRGB format /// public static PixelFormat Rgb24 { get { return new PixelFormat(PixelFormatEnum.Rgb24); } } ////// Bgr101010: 32 bpp SRGB format /// public static PixelFormat Bgr101010 { get { return new PixelFormat(PixelFormatEnum.Bgr101010); } } ////// Bgr32: 32 bpp SRGB format /// public static PixelFormat Bgr32 { get { return new PixelFormat(PixelFormatEnum.Bgr32); } } ////// Bgra32: 32 bpp SRGB format /// public static PixelFormat Bgra32 { get { return new PixelFormat(PixelFormatEnum.Bgra32); } } ////// Pbgra32: 32 bpp SRGB format /// public static PixelFormat Pbgra32 { get { return new PixelFormat(PixelFormatEnum.Pbgra32); } } ////// Rgb48: 48 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgb48 { get { return new PixelFormat(PixelFormatEnum.Rgb48); } } ////// Rgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba64 { get { return new PixelFormat(PixelFormatEnum.Rgba64); } } ////// Prgba64: 64 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba64 { get { return new PixelFormat(PixelFormatEnum.Prgba64); } } ////// Gray16: 16 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray16 { get { return new PixelFormat(PixelFormatEnum.Gray16); } } ////// Gray32Float: 32 bpp Gray-scale format; Gamma is 1.0 /// public static PixelFormat Gray32Float { get { return new PixelFormat(PixelFormatEnum.Gray32Float); } } ////// Rgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Rgba128Float { get { return new PixelFormat(PixelFormatEnum.Rgba128Float); } } ////// Prgba128Float: 128 bpp extended format; Gamma is 1.0 /// public static PixelFormat Prgba128Float { get { return new PixelFormat(PixelFormatEnum.Prgba128Float); } } ////// Cmyk32: 32 bpp format /// public static PixelFormat Cmyk32 { get { return new PixelFormat(PixelFormatEnum.Cmyk32); } } } #endregion // PixelFormats } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
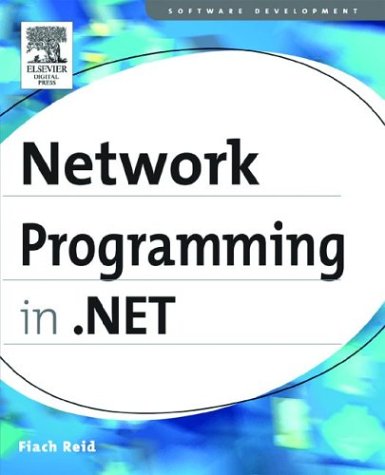
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeListConverter.cs
- MetadataCollection.cs
- WindowsEditBoxRange.cs
- HtmlWindow.cs
- RsaElement.cs
- Base64Encoder.cs
- ToolCreatedEventArgs.cs
- SelectionPattern.cs
- PrinterUnitConvert.cs
- XmlSchemaExternal.cs
- ReachSerializationUtils.cs
- IdentityReference.cs
- SqlBooleanMismatchVisitor.cs
- EndpointAddress10.cs
- DataRow.cs
- LineBreakRecord.cs
- MeasurementDCInfo.cs
- AnnotationComponentChooser.cs
- X509ChainElement.cs
- CustomPopupPlacement.cs
- SessionPageStateSection.cs
- StrokeCollection2.cs
- DataListItemEventArgs.cs
- EnumValidator.cs
- FontNamesConverter.cs
- Line.cs
- _DigestClient.cs
- RouteParser.cs
- IImplicitResourceProvider.cs
- ReadOnlyPermissionSet.cs
- SqlTypeSystemProvider.cs
- ConfigErrorGlyph.cs
- CharacterBuffer.cs
- CalendarAutoFormat.cs
- x509utils.cs
- ProfileSettingsCollection.cs
- GatewayIPAddressInformationCollection.cs
- BitmapEffectState.cs
- SystemIPGlobalProperties.cs
- MonitoringDescriptionAttribute.cs
- PrinterResolution.cs
- D3DImage.cs
- TypeNameConverter.cs
- TextParagraph.cs
- Switch.cs
- ActivityMetadata.cs
- EmptyWithCancelationCheckWorkItem.cs
- AssertHelper.cs
- LinkTarget.cs
- RefreshEventArgs.cs
- NavigationPropertyEmitter.cs
- Transform3DCollection.cs
- SecurityHeaderTokenResolver.cs
- StrongNameKeyPair.cs
- DoubleAnimationClockResource.cs
- CounterCreationData.cs
- DeflateStream.cs
- _ConnectStream.cs
- DataObject.cs
- MatrixTransform.cs
- LongSumAggregationOperator.cs
- MiniConstructorInfo.cs
- ObjectManager.cs
- WebPartConnectionsCloseVerb.cs
- ConstructorNeedsTagAttribute.cs
- WindowsAuthenticationEventArgs.cs
- PasswordBox.cs
- SqlNode.cs
- NotificationContext.cs
- PrintPageEvent.cs
- IChannel.cs
- WeakReferenceEnumerator.cs
- WebBrowserHelper.cs
- DoubleLinkListEnumerator.cs
- WebConfigurationHost.cs
- SessionStateModule.cs
- CodeDirectiveCollection.cs
- OdbcConnectionStringbuilder.cs
- WebPartEditorApplyVerb.cs
- DesignerVerb.cs
- FormViewDeletedEventArgs.cs
- validation.cs
- embossbitmapeffect.cs
- UserControlAutomationPeer.cs
- XmlChoiceIdentifierAttribute.cs
- Config.cs
- PerformanceCounterScope.cs
- MultipartContentParser.cs
- CompilerInfo.cs
- DecoderNLS.cs
- CodeSnippetExpression.cs
- WebPartHelpVerb.cs
- InvalidOperationException.cs
- FixUpCollection.cs
- MultipleViewProviderWrapper.cs
- RoleManagerModule.cs
- _DisconnectOverlappedAsyncResult.cs
- TreeNode.cs
- IBuiltInEvidence.cs
- ManagementOptions.cs