Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / InertiaRotationBehavior.cs / 1305600 / InertiaRotationBehavior.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Input.Manipulations; namespace System.Windows.Input { ////// Provides information about the inertia behavior. /// public class InertiaRotationBehavior { ////// Instantiates a new instance of this class. /// public InertiaRotationBehavior() { } ////// Instantiates a new instance of this class. /// internal InertiaRotationBehavior(double initialVelocity) { _initialVelocity = initialVelocity; } ////// The initial rate of angular change of the element at the start of the inertia phase in degrees/ms. /// public double InitialVelocity { get { return _initialVelocity; } set { _isInitialVelocitySet = true; _initialVelocity = value; } } ////// The desired rate of change of velocity in degrees/ms^2. /// public double DesiredDeceleration { get { return _desiredDeceleration; } set { if (Double.IsInfinity(value) || Double.IsNaN(value)) { throw new ArgumentOutOfRangeException("value"); } _isDesiredDecelerationSet = true; _desiredDeceleration = value; _isDesiredRotationSet = false; _desiredRotation = double.NaN; } } ////// The desired total change in angle in degrees. /// public double DesiredRotation { get { return _desiredRotation; } set { if (Double.IsInfinity(value) || Double.IsNaN(value)) { throw new ArgumentOutOfRangeException("value"); } _isDesiredRotationSet = true; _desiredRotation = value; _isDesiredDecelerationSet = false; _desiredDeceleration = double.NaN; } } internal bool CanUseForInertia() { return _isInitialVelocitySet || _isDesiredDecelerationSet || _isDesiredRotationSet; } internal static void ApplyParameters(InertiaRotationBehavior behavior, InertiaProcessor2D processor, double initialVelocity) { if (behavior != null && behavior.CanUseForInertia()) { InertiaRotationBehavior2D behavior2D = new InertiaRotationBehavior2D(); if (behavior._isInitialVelocitySet) { behavior2D.InitialVelocity = (float)AngleUtil.DegreesToRadians(behavior._initialVelocity); } else { behavior2D.InitialVelocity = (float)AngleUtil.DegreesToRadians(initialVelocity); } if (behavior._isDesiredDecelerationSet) { behavior2D.DesiredDeceleration = (float)AngleUtil.DegreesToRadians(behavior._desiredDeceleration); } if (behavior._isDesiredRotationSet) { behavior2D.DesiredRotation = (float)AngleUtil.DegreesToRadians(behavior._desiredRotation); } processor.RotationBehavior = behavior2D; } } private bool _isInitialVelocitySet; private double _initialVelocity = double.NaN; private bool _isDesiredDecelerationSet; private double _desiredDeceleration = double.NaN; private bool _isDesiredRotationSet; private double _desiredRotation = double.NaN; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
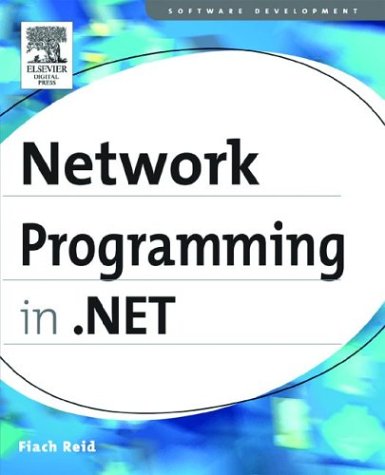
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextChange.cs
- Model3DGroup.cs
- SafeCryptoHandles.cs
- DataContractSerializerSection.cs
- MetadataArtifactLoaderCompositeFile.cs
- DataGridColumnHeaderAutomationPeer.cs
- BindingBase.cs
- DataGridViewImageCell.cs
- CompoundFileIOPermission.cs
- SQLGuidStorage.cs
- ConnectionsZone.cs
- RegexCode.cs
- ListViewItemSelectionChangedEvent.cs
- AbstractExpressions.cs
- ServiceModelConfigurationSectionCollection.cs
- ActivityFunc.cs
- WindowsMenu.cs
- UniqueIdentifierService.cs
- RectangleGeometry.cs
- TabControl.cs
- ExceptionRoutedEventArgs.cs
- CharacterBufferReference.cs
- DiscardableAttribute.cs
- ScriptBehaviorDescriptor.cs
- ProviderSettingsCollection.cs
- DataObjectMethodAttribute.cs
- DropDownList.cs
- UInt16.cs
- XmlSchemaAnyAttribute.cs
- PropertyFilter.cs
- COM2ColorConverter.cs
- CustomErrorsSectionWrapper.cs
- AddingNewEventArgs.cs
- ModelItemCollection.cs
- FilterException.cs
- DataGridViewRowEventArgs.cs
- HttpModuleCollection.cs
- CommunicationObject.cs
- ILGenerator.cs
- Literal.cs
- NestPullup.cs
- AsymmetricSignatureDeformatter.cs
- AssemblyFilter.cs
- ReferentialConstraint.cs
- DataGridViewImageColumn.cs
- InputManager.cs
- PrivateFontCollection.cs
- XmlReturnWriter.cs
- KeyValueInternalCollection.cs
- DayRenderEvent.cs
- SessionStateSection.cs
- RSAProtectedConfigurationProvider.cs
- RegularExpressionValidator.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- TextBox.cs
- ClientFormsIdentity.cs
- IdentityModelDictionary.cs
- SQLSingleStorage.cs
- WebServiceMethodData.cs
- DbParameterHelper.cs
- ClipboardProcessor.cs
- XmlJsonWriter.cs
- RegexRunner.cs
- SliderAutomationPeer.cs
- ProfilePropertySettingsCollection.cs
- TraceEventCache.cs
- QueryGenerator.cs
- Convert.cs
- XmlSchemaAttributeGroupRef.cs
- IdentifierCreationService.cs
- WebRequest.cs
- ItemsChangedEventArgs.cs
- SqlBuffer.cs
- basevalidator.cs
- XmlSchemaAttribute.cs
- XPathNodeInfoAtom.cs
- BevelBitmapEffect.cs
- WebFormsRootDesigner.cs
- GridViewCommandEventArgs.cs
- StyleBamlTreeBuilder.cs
- SecurityTokenValidationException.cs
- InternalControlCollection.cs
- FileLevelControlBuilderAttribute.cs
- ServiceBehaviorElement.cs
- DirectoryLocalQuery.cs
- SecurityBindingElement.cs
- ADRoleFactory.cs
- CodePropertyReferenceExpression.cs
- File.cs
- Graphics.cs
- ConfigurationConverterBase.cs
- DrawingImage.cs
- TabItemWrapperAutomationPeer.cs
- HttpModuleCollection.cs
- ContentType.cs
- SqlMethodTransformer.cs
- SystemDiagnosticsSection.cs
- VerificationException.cs
- SqlColumnizer.cs
- brushes.cs