Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / InputBindingCollection.cs / 1305600 / InputBindingCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: InputBindingCollection serves the purpose of Storing/Retrieving InputBindings // // See spec at : http://avalon/coreui/Specs/Commanding(new).mht // // // History: // 04/02/2004 : chandras - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Collections.Specialized; using System.Windows; using System.Windows.Input; using MS.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// InputBindingCollection - Collection of InputBindings. /// Stores the InputBindings Sequentially in an System.Collections.Generic.List"InputBinding"a. /// Will be changed to generic List implementation once the /// parser supports generic collections. /// public sealed class InputBindingCollection : IList { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constructor /// public InputBindingCollection() { } ////// InputBindingCollection /// /// InputBinding array public InputBindingCollection(IList inputBindings) { if (inputBindings != null && inputBindings.Count > 0) { this.AddRange(inputBindings as ICollection); } } ////// Internal Constructor /// internal InputBindingCollection(DependencyObject owner) { _owner = owner; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods #region Implementation of IList #region Implementation of ICollection ////// CopyTo - to copy the entire collection into an array /// /// generic object array /// void ICollection.CopyTo(System.Array array, int index) { if (_innerBindingList != null) { ((ICollection)_innerBindingList).CopyTo(array, index); } } #endregion Implementation of ICollection ////// IList.Contains /// /// key ///true - if found, false - otherwise bool IList.Contains(object key) { return this.Contains(key as InputBinding); } ////// IndexOf - returns the index of the item in the list /// /// item whose index is sought ///index of the item or -1 int IList.IndexOf(object value) { InputBinding inputBinding = value as InputBinding; return ((inputBinding != null) ? this.IndexOf(inputBinding) : -1); } ////// Insert /// /// /// void IList.Insert(int index, object value) { this.Insert(index, value as InputBinding); } ////// Add - appends the given inputbinding to the current list. /// /// InputBinding object to add int IList.Add(object inputBinding) { this.Add(inputBinding as InputBinding); return 0; // ICollection.Add no longer returns the indice } ////// Remove - removes the given inputbinding from the current list. /// /// InputBinding object to remove void IList.Remove(object inputBinding) { this.Remove(inputBinding as InputBinding); } ////// Indexing operator /// object IList.this[int index] { get { return this[index]; } set { InputBinding inputBinding = value as InputBinding; if (inputBinding == null) throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsInputBindings)); this[index] = inputBinding; } } #endregion Implementation of IList ////// Indexing operator /// public InputBinding this[int index] { get { // disable PreSharp warning about throwing exceptions in getter; // this is allowed in an indexed property. (First disable C# // warning about unknown warning numbers.) #pragma warning disable 1634, 1691 #pragma warning disable 6503 if (_innerBindingList != null) { return _innerBindingList[index]; } else { throw new ArgumentOutOfRangeException("index"); } #pragma warning restore 6503 #pragma warning restore 1634, 1691 } set { if (_innerBindingList != null) { InputBinding oldInputBinding = null; if (index >= 0 && index < _innerBindingList.Count) { oldInputBinding = _innerBindingList[index]; } _innerBindingList[index] = value; if (oldInputBinding != null) { InheritanceContextHelper.RemoveContextFromObject(_owner, oldInputBinding); } InheritanceContextHelper.ProvideContextForObject(_owner, value); } else { throw new ArgumentOutOfRangeException("index"); } } } ////// Add /// /// public int Add(InputBinding inputBinding) { if (inputBinding != null) { if (_innerBindingList == null) _innerBindingList = new System.Collections.Generic.List(1); _innerBindingList.Add(inputBinding); InheritanceContextHelper.ProvideContextForObject(_owner, inputBinding); return 0; // ICollection.Add no longer returns the indice } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsInputBindings)); } } /// /// ICollection.IsSynchronized /// public bool IsSynchronized { get { if (_innerBindingList != null) return ((IList)_innerBindingList).IsSynchronized; return false; } } ////// IndexOf /// /// ///public int IndexOf(InputBinding value) { return (_innerBindingList != null) ? _innerBindingList.IndexOf(value) : -1; } /// /// Adds the elements of the given collection to the end of this list. If /// required, the capacity of the list is increased to twice the previous /// capacity or the new size, whichever is larger. /// /// collection to append public void AddRange(ICollection collection) { if (collection == null) { throw new ArgumentNullException("collection"); } if ( collection.Count > 0) { if (_innerBindingList == null) _innerBindingList = new System.Collections.Generic.List(collection.Count); IEnumerator collectionEnum = collection.GetEnumerator(); while(collectionEnum.MoveNext()) { InputBinding inputBinding = collectionEnum.Current as InputBinding; if (inputBinding != null) { _innerBindingList.Add(inputBinding); InheritanceContextHelper.ProvideContextForObject(_owner, inputBinding); } else { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsInputBindings)); } } } } /// /// Insert at given index /// /// index at which to insert the given item /// inputBinding to insert public void Insert(int index, InputBinding inputBinding) { if (inputBinding == null) { throw new NotSupportedException(SR.Get(SRID.CollectionOnlyAcceptsInputBindings)); } if (_innerBindingList != null) { _innerBindingList.Insert(index, inputBinding); InheritanceContextHelper.ProvideContextForObject(_owner, inputBinding); } } ////// Remove /// /// public void Remove(InputBinding inputBinding) { if (_innerBindingList != null && inputBinding != null) { if (_innerBindingList.Remove(inputBinding as InputBinding)) { InheritanceContextHelper.RemoveContextFromObject(_owner, inputBinding); } } } ////// RemoveAt /// /// index at which the item needs to be removed public void RemoveAt(int index) { if (_innerBindingList != null) { InputBinding oldInputBinding = null; if (index >= 0 && index < _innerBindingList.Count) { oldInputBinding = _innerBindingList[index]; } _innerBindingList.RemoveAt(index); if (oldInputBinding != null) { InheritanceContextHelper.RemoveContextFromObject(_owner, oldInputBinding); } } } ////// IsFixedSize - if readonly - fixed, else false. /// public bool IsFixedSize { get { return IsReadOnly; } } ////// Count /// public int Count { get { return (_innerBindingList != null ? _innerBindingList.Count : 0); } } ////// ICollection.SyncRoot /// public object SyncRoot { get { return this; } } ////// Clears the Entire InputBindingCollection /// public void Clear() { if (_innerBindingList != null) { ListoldInputBindings = new List (_innerBindingList); _innerBindingList.Clear(); _innerBindingList = null; foreach (InputBinding inputBinding in oldInputBindings) { InheritanceContextHelper.RemoveContextFromObject(_owner, inputBinding); } } } #region Implementation of Enumerable /// /// IEnumerable.GetEnumerator - For Enumeration purposes /// ///public IEnumerator GetEnumerator() { if (_innerBindingList != null) return _innerBindingList.GetEnumerator(); System.Collections.Generic.List list = new System.Collections.Generic.List (0); return list.GetEnumerator(); } #endregion Implementation of IEnumberable /// /// IList.IsReadOnly - Tells whether this is readonly Collection. /// public bool IsReadOnly { get { return _isReadOnly; } } ////// Contains /// /// key ///true - if found, false - otherwise public bool Contains(InputBinding key) { if (_innerBindingList != null && key != null) { return _innerBindingList.Contains(key); } return false; } ////// CopyTo - to copy the entire collection into an array /// /// type-safe InputBinding array /// start index in the current list to copy public void CopyTo(InputBinding[] inputBindings, int index) { if (_innerBindingList != null) { _innerBindingList.CopyTo(inputBindings, index); } } #endregion Public #region internal internal InputBinding FindMatch(object targetElement, InputEventArgs inputEventArgs) { for (int i = Count - 1; i >= 0; i--) { InputBinding inputBinding = this[i]; if ((inputBinding.Command != null) && (inputBinding.Gesture != null) && inputBinding.Gesture.Matches(targetElement, inputEventArgs)) { return inputBinding; } } return null; } #endregion internal //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private List_innerBindingList; private bool _isReadOnly = false; private DependencyObject _owner = null; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
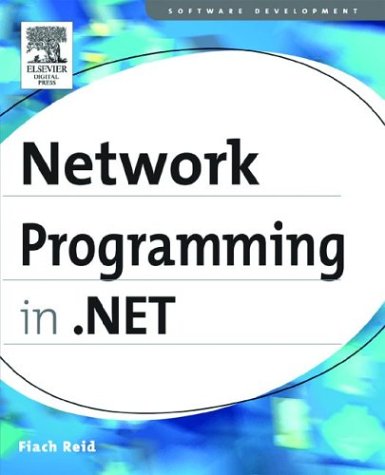
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SliderAutomationPeer.cs
- PickBranch.cs
- LineSegment.cs
- ListCardsInFileRequest.cs
- Timeline.cs
- PackageRelationshipSelector.cs
- DecoderFallbackWithFailureFlag.cs
- CompilationLock.cs
- OleDbCommand.cs
- Variable.cs
- ServicePointManager.cs
- HttpModulesSection.cs
- ModelPerspective.cs
- ValidationSettings.cs
- pingexception.cs
- Brush.cs
- GradientStop.cs
- UpnEndpointIdentityExtension.cs
- FigureParaClient.cs
- StoragePropertyMapping.cs
- Journaling.cs
- TableRow.cs
- diagnosticsswitches.cs
- AccessDataSourceWizardForm.cs
- XPathSingletonIterator.cs
- TraceEventCache.cs
- DataSysAttribute.cs
- UInt32.cs
- InfiniteIntConverter.cs
- PartialCachingAttribute.cs
- WSTrustFeb2005.cs
- MissingFieldException.cs
- TraceFilter.cs
- Missing.cs
- LinkUtilities.cs
- ThicknessConverter.cs
- MatrixTransform.cs
- RegexStringValidatorAttribute.cs
- MaskedTextBoxTextEditor.cs
- DragCompletedEventArgs.cs
- CollaborationHelperFunctions.cs
- ToolStripItemCollection.cs
- IncrementalCompileAnalyzer.cs
- SchemaLookupTable.cs
- InstanceKeyNotReadyException.cs
- ObjectComplexPropertyMapping.cs
- XmlUTF8TextReader.cs
- SchemaDeclBase.cs
- AtomEntry.cs
- DataGridAddNewRow.cs
- DebugView.cs
- JobDuplex.cs
- ToolStripItemCollection.cs
- ZoneButton.cs
- _CookieModule.cs
- PropertyInformationCollection.cs
- CodeTypeReferenceSerializer.cs
- TimeoutException.cs
- MsmqIntegrationInputChannel.cs
- OdbcCommand.cs
- HwndProxyElementProvider.cs
- PageBuildProvider.cs
- StructuralCache.cs
- XmlHierarchyData.cs
- HtmlMobileTextWriter.cs
- RuleSettings.cs
- ModelEditingScope.cs
- CollectionAdapters.cs
- OperationAbortedException.cs
- XpsPackagingException.cs
- WebAdminConfigurationHelper.cs
- ClientSettings.cs
- SmtpException.cs
- StdValidatorsAndConverters.cs
- DataSourceCacheDurationConverter.cs
- CommandSet.cs
- DefaultHttpHandler.cs
- DecimalAnimationUsingKeyFrames.cs
- DependencyPropertyConverter.cs
- ProjectedWrapper.cs
- FileIOPermission.cs
- DesignerActionListCollection.cs
- FieldAccessException.cs
- CommonGetThemePartSize.cs
- HuffmanTree.cs
- DrawingCollection.cs
- PropertyStore.cs
- ChunkedMemoryStream.cs
- WorkflowQueueInfo.cs
- X509SecurityTokenProvider.cs
- DecoderExceptionFallback.cs
- AdRotatorDesigner.cs
- wgx_exports.cs
- UpdatePanel.cs
- NotificationContext.cs
- controlskin.cs
- DropTarget.cs
- WinEventTracker.cs
- PaperSource.cs
- BitmapFrame.cs