Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / ComponentModel / SortDescriptionCollection.cs / 1305600 / SortDescriptionCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: dynamic collection of SortDescriptions // // See spec at http://avalon/connecteddata/Specs/CollectionView.mht // // History: // 03/24/2005 : [....] - created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Windows; using MS.Utility; namespace System.ComponentModel { ////// Implementation of a dynamic data collection of SortDescriptions. /// public class SortDescriptionCollection : Collection, INotifyCollectionChanged { //----------------------------------------------------- // // Public Events // //----------------------------------------------------- #region Public Events /// /// Occurs when the collection changes, either by adding or removing an item. /// ////// see event NotifyCollectionChangedEventHandler INotifyCollectionChanged.CollectionChanged { add { CollectionChanged += value; } remove { CollectionChanged -= value; } } ////// /// Occurs when the collection changes, either by adding or removing an item. /// protected event NotifyCollectionChangedEventHandler CollectionChanged; #endregion Public Events //------------------------------------------------------ // // Protected Methods // //----------------------------------------------------- #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { base.ClearItems(); OnCollectionChanged(NotifyCollectionChangedAction.Reset); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { SortDescription removedItem = this[index]; base.RemoveItem(index); OnCollectionChanged(NotifyCollectionChangedAction.Remove, removedItem, index); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, SortDescription item) { item.Seal(); base.InsertItem(index, item); OnCollectionChanged(NotifyCollectionChangedAction.Add, item, index); } ////// called by base class Collection<T> when an item is set in the list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, SortDescription item) { item.Seal(); SortDescription originalItem = this[index]; base.SetItem(index, item); OnCollectionChanged(NotifyCollectionChangedAction.Remove, originalItem, index); OnCollectionChanged(NotifyCollectionChangedAction.Add, item, index); } ////// raise CollectionChanged event to any listeners /// private void OnCollectionChanged(NotifyCollectionChangedAction action, object item, int index) { if (CollectionChanged != null) { CollectionChanged(this, new NotifyCollectionChangedEventArgs(action, item, index)); } } // raise CollectionChanged event to any listeners void OnCollectionChanged(NotifyCollectionChangedAction action) { if (CollectionChanged != null) { CollectionChanged(this, new NotifyCollectionChangedEventArgs(action)); } } #endregion Protected Methods ////// Immutable, read-only SortDescriptionCollection /// class EmptySortDescriptionCollection : SortDescriptionCollection, IList { //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// called by base class Collection<T> when the list is being cleared; /// raises a CollectionChanged event to any listeners /// protected override void ClearItems() { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is removed from list; /// raises a CollectionChanged event to any listeners /// protected override void RemoveItem(int index) { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is added to list; /// raises a CollectionChanged event to any listeners /// protected override void InsertItem(int index, SortDescription item) { throw new NotSupportedException(); } ////// called by base class Collection<T> when an item is set in list; /// raises a CollectionChanged event to any listeners /// protected override void SetItem(int index, SortDescription item) { throw new NotSupportedException(); } #endregion Protected Methods #region IList Implementations // explicit implementation to override the IsReadOnly and IsFixedSize properties bool IList.IsFixedSize { get { return true; } } bool IList.IsReadOnly { get { return true; } } #endregion IList Implementations } ////// returns an empty and non-modifiable SortDescriptionCollection /// public static readonly SortDescriptionCollection Empty = new EmptySortDescriptionCollection(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
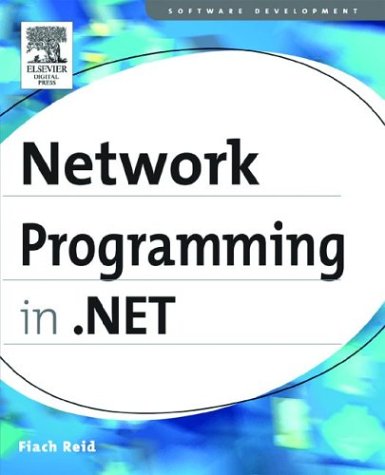
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- log.cs
- ServiceModelActivationSectionGroup.cs
- RegistryPermission.cs
- infer.cs
- FrugalMap.cs
- PageTextBox.cs
- DispatcherExceptionFilterEventArgs.cs
- TextRange.cs
- WindowsGraphicsCacheManager.cs
- DescendantOverDescendantQuery.cs
- PropertyKey.cs
- RestHandler.cs
- MultipartContentParser.cs
- AtlasWeb.Designer.cs
- __Filters.cs
- DrawingVisualDrawingContext.cs
- SessionIDManager.cs
- RegionData.cs
- ZipIOFileItemStream.cs
- SizeAnimation.cs
- ChannelDispatcherCollection.cs
- FastPropertyAccessor.cs
- ServiceReflector.cs
- XmlDataCollection.cs
- InvokeHandlers.cs
- DragEvent.cs
- TagPrefixInfo.cs
- HttpProfileGroupBase.cs
- Panel.cs
- Listbox.cs
- ObjectComplexPropertyMapping.cs
- CodeDOMUtility.cs
- ReferencedType.cs
- ComAdminInterfaces.cs
- WebPartVerbsEventArgs.cs
- SystemColorTracker.cs
- UnknownWrapper.cs
- InvalidContentTypeException.cs
- SqlLiftIndependentRowExpressions.cs
- SchemaImporterExtension.cs
- XmlReaderSettings.cs
- BitmapFrameDecode.cs
- Char.cs
- DataGridViewRowsRemovedEventArgs.cs
- Token.cs
- _CommandStream.cs
- ToolStripComboBox.cs
- TcpSocketManager.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- DeadCharTextComposition.cs
- WinFormsSpinner.cs
- log.cs
- ClientSettingsSection.cs
- ReturnValue.cs
- FileDialog_Vista_Interop.cs
- XpsViewerException.cs
- HttpCookieCollection.cs
- StandardCommands.cs
- HtmlInputFile.cs
- ComponentEditorForm.cs
- IOException.cs
- FontFamily.cs
- PublishLicense.cs
- SqlPersonalizationProvider.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- GridViewDeleteEventArgs.cs
- MaskedTextBox.cs
- NativeMethods.cs
- MexHttpBindingElement.cs
- SymmetricAlgorithm.cs
- CompiledRegexRunnerFactory.cs
- WebPartUserCapability.cs
- SqlResolver.cs
- SafeHandles.cs
- WebPartZone.cs
- DeferredTextReference.cs
- OleDbConnection.cs
- TCPListener.cs
- Filter.cs
- ClientOptions.cs
- TaskHelper.cs
- TrackingServices.cs
- HtmlLink.cs
- FormattedTextSymbols.cs
- Itemizer.cs
- DataServices.cs
- HttpResponseWrapper.cs
- EntityDataSourceSelectingEventArgs.cs
- ReferenceConverter.cs
- ContextMenu.cs
- ExpressionVisitor.cs
- Quaternion.cs
- XamlClipboardData.cs
- XmlText.cs
- PtsHost.cs
- LocalFileSettingsProvider.cs
- ContainerFilterService.cs
- StringSource.cs
- RelatedPropertyManager.cs
- KeySpline.cs