Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / listitem.cs / 1305376 / listitem.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using AttributeCollection = System.Web.UI.AttributeCollection; using System.Web.Util; ////// public class ListItemControlBuilder : ControlBuilder { public override bool AllowWhitespaceLiterals() { return false; } public override bool HtmlDecodeLiterals() { // ListItem text gets rendered as an encoded attribute value. // At parse time text specified as an attribute gets decoded, and so text specified as a // literal needs to go through the same process. return true; } } ///Interacts with the parser to build a ///control. /// [ ControlBuilderAttribute(typeof(ListItemControlBuilder)), TypeConverterAttribute(typeof(ExpandableObjectConverter)), ParseChildren(true, "Text"), ] public sealed class ListItem : IStateManager, IParserAccessor, IAttributeAccessor { private bool selected; private bool marked; private bool textisdirty; private bool valueisdirty; private bool enabled; private bool enabledisdirty; private string text; private string value; private AttributeCollection _attributes; ///Constructs a list item control and defines /// its properties. This class cannot be inherited. ////// public ListItem() : this(null, null) { } ///Initializes a new instance of the ///class. /// public ListItem(string text) : this(text, null) { } ///Initializes a new instance of the ///class with the specified text data. /// public ListItem(string text, string value) : this(text, value, true) { } ///Initializes a new instance of the ///class with the specified text data. /// public ListItem(string text, string value, bool enabled) { this.text = text; this.value = value; this.enabled = enabled; } ///Initializes a new instance of the ///class with the /// specified text and value data. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public AttributeCollection Attributes { get { if (_attributes == null) _attributes = new AttributeCollection(new StateBag(true)); return _attributes; } } ///Gets the collection of attribute name/value pairs expressed on the list item /// control but not supported by the control's strongly typed properties. ////// Internal property used to manage dirty state of ListItem. /// internal bool Dirty { get { return (textisdirty || valueisdirty || enabledisdirty); } set { textisdirty = value; valueisdirty = value; enabledisdirty = value; } } [ DefaultValue(true) ] public bool Enabled { get { return enabled; } set { enabled = value; if (((IStateManager)this).IsTrackingViewState) enabledisdirty = true; } } internal bool HasAttributes { get { return _attributes != null && _attributes.Count > 0; } } ////// [ DefaultValue(false), TypeConverter(typeof(MinimizableAttributeTypeConverter)) ] public bool Selected { get { return selected; } set { selected = value; } } ///Specifies a value indicating whether the /// item is selected. ////// [ Localizable(true), DefaultValue(""), PersistenceMode(PersistenceMode.EncodedInnerDefaultProperty) ] public string Text { get { if (text != null) return text; if (value != null) return value; return String.Empty; } set { text = value; if (((IStateManager)this).IsTrackingViewState) textisdirty = true; } } ///Gets or sets the display text of the list /// item control. ////// [ Localizable(true), DefaultValue("") ] public string Value { get { if (value != null) return value; if (text != null) return text; return String.Empty; } set { this.value = value; if (((IStateManager)this).IsTrackingViewState) valueisdirty =true; } } ///Gets or sets the value content of the list item control. ///public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(Value.GetHashCode(), Text.GetHashCode()); } /// /// /// public override bool Equals(object o) { ListItem other = o as ListItem; if (other != null) { return Value.Equals(other.Value) && Text.Equals(other.Text); } return false; } ////// public static ListItem FromString(string s) { return new ListItem(s); } ///Creates a ///from the specified string. /// /// public override string ToString() { return this.Text; } ////// /// Return true if tracking state changes. /// Method of private interface, IStateManager. /// bool IStateManager.IsTrackingViewState { get { return marked; } } ///void IStateManager.LoadViewState(object state) { LoadViewState(state); } internal void LoadViewState(object state) { if (state != null) { if (state is Triplet) { Triplet t = (Triplet) state; if (t.First != null) { Text = (string) t.First; } if (t.Second != null) { Value = (string) t.Second; } if (t.Third != null) { try { Enabled = (bool) t.Third; } catch { } } } else if (state is Pair) { Pair p = (Pair) state; if (p.First != null) Text = (string) p.First; Value = (string) p.Second; } else Text = (string) state; } } /// void IStateManager.TrackViewState() { TrackViewState(); } internal void TrackViewState() { marked = true; } internal void RenderAttributes(HtmlTextWriter writer) { if (_attributes != null) { _attributes.AddAttributes(writer); } } /// object IStateManager.SaveViewState() { return SaveViewState(); } internal object SaveViewState() { string text = null; string value = null; if(textisdirty) { text = Text; } if(valueisdirty) { value = Value; } if(enabledisdirty) { return new Triplet(text, value, Enabled); } else if(valueisdirty) { return new Pair(text, value); } else if(textisdirty) { return text; } return null; } /// /// /// Returns the attribute value of the list item control /// having the specified attribute name. /// string IAttributeAccessor.GetAttribute(string name) { return Attributes[name]; } ////// /// void IAttributeAccessor.SetAttribute(string name, string value) { Attributes[name] = value; } ///Sets an attribute of the list /// item control with the specified name and value. ////// /// void IParserAccessor.AddParsedSubObject(object obj) { if (obj is LiteralControl) { Text = ((LiteralControl)obj).Text; } else { if (obj is DataBoundLiteralControl) { throw new HttpException(SR.GetString(SR.Control_Cannot_Databind, "ListItem")); } else { throw new HttpException(SR.GetString(SR.Cannot_Have_Children_Of_Type, "ListItem", obj.GetType().Name.ToString(CultureInfo.InvariantCulture))); } } } ///Allows the ////// property to be persisted as inner content. /// Do not change the signature or remove this method. It is called via reflection /// to support correct serialization behavior for Text and Value which are /// implemented as dependent properties. /// private void ResetText() { Text = null; } ////// Do not change the signature or remove this method. It is called via reflection /// to support correct serialization behavior for Text and Value which are /// implemented as dependent properties. /// private void ResetValue() { Value = null; } ////// Do not change the signature or remove this method. It is called via reflection /// to support correct serialization behavior for Text and Value which are /// implemented as dependent properties. /// private bool ShouldSerializeText() { return (text != null) && (text.Length != 0); } ////// Do not change the signature or remove this method. It is called via reflection /// to support correct serialization behavior for Text and Value which are /// implemented as dependent properties. /// private bool ShouldSerializeValue() { return (value != null) && (value.Length != 0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
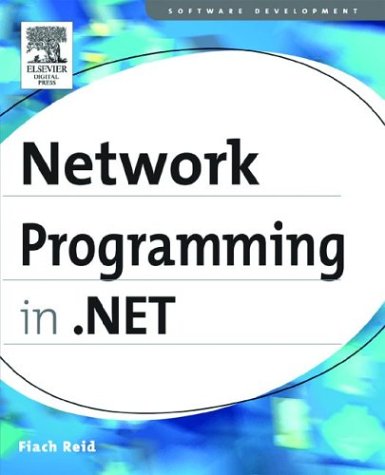
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OrderedDictionaryStateHelper.cs
- ProfileSection.cs
- SchemaLookupTable.cs
- IIS7WorkerRequest.cs
- SqlFunctions.cs
- FlowDocument.cs
- SettingsAttributes.cs
- FieldNameLookup.cs
- LocalsItemDescription.cs
- EditorPartChrome.cs
- UnionQueryOperator.cs
- ScriptingSectionGroup.cs
- RequestedSignatureDialog.cs
- OuterGlowBitmapEffect.cs
- CompilerGeneratedAttribute.cs
- XmlRootAttribute.cs
- OledbConnectionStringbuilder.cs
- DbQueryCommandTree.cs
- DateTimeStorage.cs
- AutoCompleteStringCollection.cs
- ExtensionFile.cs
- DocumentEventArgs.cs
- FileCodeGroup.cs
- DoubleLinkListEnumerator.cs
- PropertyValueChangedEvent.cs
- ConfigurationErrorsException.cs
- HttpRequest.cs
- OrderedDictionaryStateHelper.cs
- ArgIterator.cs
- AssemblyInfo.cs
- TripleDES.cs
- WebBrowserProgressChangedEventHandler.cs
- PointConverter.cs
- PropertyGridCommands.cs
- EllipseGeometry.cs
- ComponentCommands.cs
- SimpleRecyclingCache.cs
- Rotation3DAnimationBase.cs
- X509CertificateEndpointIdentity.cs
- IntegerValidator.cs
- Point3DAnimationUsingKeyFrames.cs
- CultureSpecificCharacterBufferRange.cs
- UiaCoreTypesApi.cs
- PropertyEmitter.cs
- HttpApplicationFactory.cs
- PropertiesTab.cs
- PlatformNotSupportedException.cs
- _SslState.cs
- MenuAdapter.cs
- GPStream.cs
- Color.cs
- TransportationConfigurationTypeInstallComponent.cs
- Int32.cs
- ReachDocumentReferenceCollectionSerializer.cs
- GridEntryCollection.cs
- FixedElement.cs
- BroadcastEventHelper.cs
- MetadataCollection.cs
- WebServiceHostFactory.cs
- WsdlEndpointConversionContext.cs
- ButtonFieldBase.cs
- StorageSetMapping.cs
- CodePageEncoding.cs
- DefaultClaimSet.cs
- Token.cs
- GeneratedCodeAttribute.cs
- FixedPage.cs
- GraphicsPath.cs
- SoapClientProtocol.cs
- CodeNamespace.cs
- RecipientInfo.cs
- TrackPointCollection.cs
- __FastResourceComparer.cs
- SpeakCompletedEventArgs.cs
- AnnotationStore.cs
- ActiveDesignSurfaceEvent.cs
- Variant.cs
- SafeReversePInvokeHandle.cs
- MetabaseSettings.cs
- JsonDataContract.cs
- PageStatePersister.cs
- BamlTreeNode.cs
- PropertyRef.cs
- _ProxyChain.cs
- HandlerMappingMemo.cs
- WsatConfiguration.cs
- LoadedOrUnloadedOperation.cs
- XmlToDatasetMap.cs
- QueryRewriter.cs
- WindowsEditBox.cs
- ToolStripDesignerAvailabilityAttribute.cs
- EntityDesignerUtils.cs
- ValidationSummary.cs
- TailPinnedEventArgs.cs
- Or.cs
- InvalidDataException.cs
- Inflater.cs
- Int32RectValueSerializer.cs
- LinqDataSourceSelectEventArgs.cs
- IsolatedStorageFile.cs