Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputButton.cs / 1305376 / HtmlInputButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputButton.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Globalization; using System.Security.Permissions; ////// [ DefaultEvent("ServerClick"), SupportsEventValidation, ] public class HtmlInputButton : HtmlInputControl, IPostBackEventHandler { private static readonly object EventServerClick = new object(); /* * Creates an intrinsic Html INPUT type=button control. */ ////// The ///class defines the methods, /// properties, and events for the HTML Input Button control. This class allows /// programmatic access to the HTML <input type= /// button>, <input type= /// submit>,and <input /// type= /// reset> elements on /// the server. /// /// public HtmlInputButton() : base("button") { } /* * Creates an intrinsic Html INPUT type=button,submit,reset control. */ ///Initializes a new instance of a ///class using /// default values. /// public HtmlInputButton(string type) : base(type) { } ///Initializes a new instance of a ///class using the /// specified string. /// [ WebCategory("Behavior"), DefaultValue(true), ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? String.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets or sets whether pressing the button causes page validation to fire. This defaults to True so that when /// using validation controls, the validation state of all controls are updated when the button is clicked, both /// on the client and the server. Setting this to False is useful when defining a cancel or reset button on a page /// that has validators. ////// [ WebCategory("Action"), WebSysDescription(SR.HtmlControl_OnServerClick) ] public event EventHandler ServerClick { add { Events.AddHandler(EventServerClick, value); } remove { Events.RemoveHandler(EventServerClick, value); } } ////// Occurs when an HTML Input Button control is clicked on the browser. /// ///protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && Events[EventServerClick] != null) { Page.RegisterPostBackScript(); } } /* * Override to generate postback code for onclick. */ /// /// /// protected override void RenderAttributes(HtmlTextWriter writer) { RenderAttributesInternal(writer); base.RenderAttributes(writer); // this must come last because of the self-closing / } // VSWhidbey 80882: It needs to be overridden by HtmlInputSubmit internal virtual void RenderAttributesInternal(HtmlTextWriter writer) { bool submitsProgramatically = Events[EventServerClick] != null; if (Page != null) { if (submitsProgramatically) { Util.WriteOnClickAttribute( writer, this, false /* submitsAutomatically */, submitsProgramatically, (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0), ValidationGroup); } else { Page.ClientScript.RegisterForEventValidation(UniqueID); } } } ////// protected virtual void OnServerClick(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerClick]; if (handler != null) handler(this, e); } /* * Method of IPostBackEventHandler interface to raise events on post back. * Button fires an OnServerClick event. */ ///Raises the ///event. /// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ////// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnServerClick(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
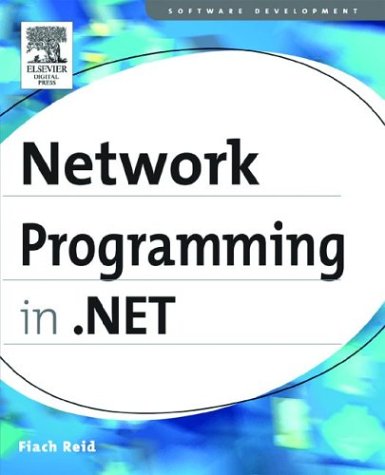
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContainerActivationHelper.cs
- StringReader.cs
- _FtpDataStream.cs
- DataGridParentRows.cs
- DataServiceConfiguration.cs
- HtmlTableRow.cs
- SdlChannelSink.cs
- BStrWrapper.cs
- WebEventTraceProvider.cs
- DependencyPropertyKind.cs
- LocatorPartList.cs
- StartUpEventArgs.cs
- BinaryWriter.cs
- GeneralTransformGroup.cs
- ObjectDataSourceDisposingEventArgs.cs
- DataServiceConfiguration.cs
- EntityDataReader.cs
- ViewStateException.cs
- MeasurementDCInfo.cs
- SqlRowUpdatedEvent.cs
- PropertyGridCommands.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- PackagePartCollection.cs
- TargetInvocationException.cs
- XmlBindingWorker.cs
- LocalFileSettingsProvider.cs
- Compilation.cs
- CompositionTarget.cs
- FixedSOMFixedBlock.cs
- ExpressionBuilderCollection.cs
- StorageEntityTypeMapping.cs
- VectorConverter.cs
- TextWriterTraceListener.cs
- AccessViolationException.cs
- Separator.cs
- WebHostScriptMappingsInstallComponent.cs
- FileLoadException.cs
- XmlSchemaGroup.cs
- ISCIIEncoding.cs
- BatchParser.cs
- HWStack.cs
- WebPartConnectionsCancelVerb.cs
- SortedList.cs
- Random.cs
- RecognizedPhrase.cs
- OdbcException.cs
- TCEAdapterGenerator.cs
- XmlSchemaCollection.cs
- ManagementBaseObject.cs
- ToolStripPanelRow.cs
- HtmlHistory.cs
- EmptyQuery.cs
- XmlAttribute.cs
- ActivityBindForm.cs
- ProjectionAnalyzer.cs
- SecurityContext.cs
- ClientScriptItem.cs
- CodeDefaultValueExpression.cs
- GeometryCombineModeValidation.cs
- StringUtil.cs
- ScrollChrome.cs
- Timer.cs
- NetworkStream.cs
- EntityDataSourceSelectedEventArgs.cs
- SudsParser.cs
- Converter.cs
- BaseServiceProvider.cs
- ObjectViewQueryResultData.cs
- EntityDataSourceSelectingEventArgs.cs
- NetworkInterface.cs
- DataGridViewRowEventArgs.cs
- HtmlContainerControl.cs
- InstanceData.cs
- ExtendedProtectionPolicy.cs
- XmlSchemaSubstitutionGroup.cs
- Logging.cs
- DebugView.cs
- EmptyEnumerator.cs
- DateTimeValueSerializerContext.cs
- ProcessingInstructionAction.cs
- RepeatButtonAutomationPeer.cs
- DesignerSerializerAttribute.cs
- SafeRightsManagementHandle.cs
- TreeNodeCollection.cs
- SerialErrors.cs
- EditorPartDesigner.cs
- PolicyException.cs
- DefaultTextStoreTextComposition.cs
- StringUtil.cs
- ZipIOExtraFieldPaddingElement.cs
- AdRotator.cs
- CacheMemory.cs
- UnmanagedMemoryStream.cs
- SystemGatewayIPAddressInformation.cs
- IdnElement.cs
- QilXmlWriter.cs
- DataGridViewComboBoxEditingControl.cs
- MethodMessage.cs
- DataPagerFieldCollection.cs
- Model3D.cs