Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / DataSourceView.cs / 1305376 / DataSourceView.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.Collections; using System.ComponentModel; using System.Security.Permissions; public abstract class DataSourceView { private static readonly object EventDataSourceViewChanged = new object(); private EventHandlerList _events; private string _name; protected DataSourceView(IDataSource owner, string viewName) { if (owner == null) { throw new ArgumentNullException("owner"); } if (viewName == null) { throw new ArgumentNullException("viewName"); } _name = viewName; DataSourceControl dataSourceControl = owner as DataSourceControl; if (dataSourceControl != null) { dataSourceControl.DataSourceChangedInternal += new EventHandler(OnDataSourceChangedInternal); } else { owner.DataSourceChanged += new EventHandler(OnDataSourceChangedInternal); } } // CanX properties indicate whether the data source allows each // operation, and if so, whether it's appropriate to do so. // For instance, a control may allow Deletion, but if a required Delete // command isn't set, CanDelete should be false, because a Delete // operation would fail. public virtual bool CanDelete { get { return false; } } public virtual bool CanInsert { get { return false; } } public virtual bool CanPage { get { return false; } } public virtual bool CanRetrieveTotalRowCount { get { return false; } } public virtual bool CanSort { get { return false; } } public virtual bool CanUpdate { get { return false; } } ////// Indicates the list of event handler delegates for the view. This property is read-only. /// protected EventHandlerList Events { get { if (_events == null) { _events = new EventHandlerList(); } return _events; } } public string Name { get { return _name; } } public event EventHandler DataSourceViewChanged { add { Events.AddHandler(EventDataSourceViewChanged, value); } remove { Events.RemoveHandler(EventDataSourceViewChanged, value); } } public virtual bool CanExecute(string commandName) { return false; } public virtual void Delete(IDictionary keys, IDictionary oldValues, DataSourceViewOperationCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } int affectedRecords = 0; bool performedCallback = false; try { affectedRecords = ExecuteDelete(keys, oldValues); } catch (Exception ex) { performedCallback = true; if (!callback(affectedRecords, ex)) { throw; } } finally { if (!performedCallback) { callback(affectedRecords, null); } } } public virtual void ExecuteCommand(string commandName, IDictionary keys, IDictionary values, DataSourceViewOperationCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } int affectedRecords = 0; bool performedCallback = false; try { affectedRecords = ExecuteCommand(commandName, keys, values); } catch (Exception ex) { performedCallback = true; if (!callback(affectedRecords, ex)) { throw; } } finally { if (!performedCallback) { callback(affectedRecords, null); } } } protected virtual int ExecuteCommand(string commandName, IDictionary keys, IDictionary values) { throw new NotSupportedException(); } ////// Performs a delete operation on the specified list. This is only /// supported by a DataSourceControl when CanDelete returns true. /// /// /// The set of name/value pairs used to filter /// the items in the list that should be deleted. /// /// /// The complete set of name/value pairs used to filter /// the items in the list that should be deleted. /// ////// The number of items that were affected by the operation. /// protected virtual int ExecuteDelete(IDictionary keys, IDictionary oldValues) { throw new NotSupportedException(); } ////// Performs an insert operation on the specified list. This is only /// supported by a DataControl when CanInsert is true. /// /// /// The set of name/value pairs to be used to initialize /// a new item in the list. /// ////// The number of items that were affected by the operation. /// protected virtual int ExecuteInsert(IDictionary values) { throw new NotSupportedException(); } ////// protected internal abstract IEnumerable ExecuteSelect(DataSourceSelectArguments arguments); ////// Performs an update operation on the specified list. This is only /// supported by a DataControl when CanUpdate is true. /// /// /// The set of name/value pairs used to filter /// the items in the list that should be updated. /// /// /// The set of name/value pairs to be used to update the /// items in the list. /// /// /// The set of name/value pairs to be used to identify the /// item to be updated. /// ////// The number of items that were affected by the operation. /// protected virtual int ExecuteUpdate(IDictionary keys, IDictionary values, IDictionary oldValues) { throw new NotSupportedException(); } private void OnDataSourceChangedInternal(object sender, EventArgs e) { OnDataSourceViewChanged(e); } protected virtual void OnDataSourceViewChanged(EventArgs e) { EventHandler handler = Events[EventDataSourceViewChanged] as EventHandler; if (handler != null) { handler(this, e); } } public virtual void Insert(IDictionary values, DataSourceViewOperationCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } int affectedRecords = 0; bool performedCallback = false; try { affectedRecords = ExecuteInsert(values); } catch (Exception ex) { performedCallback = true; if (!callback(affectedRecords, ex)) { throw; } } finally { if (!performedCallback) { callback(affectedRecords, null); } } } protected internal virtual void RaiseUnsupportedCapabilityError(DataSourceCapabilities capability) { if (!CanPage && ((capability & DataSourceCapabilities.Page) != 0)) { throw new NotSupportedException(SR.GetString(SR.DataSourceView_NoPaging)); } if (!CanSort && ((capability & DataSourceCapabilities.Sort) != 0)) { throw new NotSupportedException(SR.GetString(SR.DataSourceView_NoSorting)); } if (!CanRetrieveTotalRowCount && ((capability & DataSourceCapabilities.RetrieveTotalRowCount) != 0)) { throw new NotSupportedException(SR.GetString(SR.DataSourceView_NoRowCount)); } } public virtual void Select(DataSourceSelectArguments arguments, DataSourceViewSelectCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } callback(ExecuteSelect(arguments)); } public virtual void Update(IDictionary keys, IDictionary values, IDictionary oldValues, DataSourceViewOperationCallback callback) { if (callback == null) { throw new ArgumentNullException("callback"); } int affectedRecords = 0; bool performedCallback = false; try { affectedRecords = ExecuteUpdate(keys, values, oldValues); } catch (Exception ex) { performedCallback = true; if (!callback(affectedRecords, ex)) { throw; } } finally { if (!performedCallback) { callback(affectedRecords, null); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
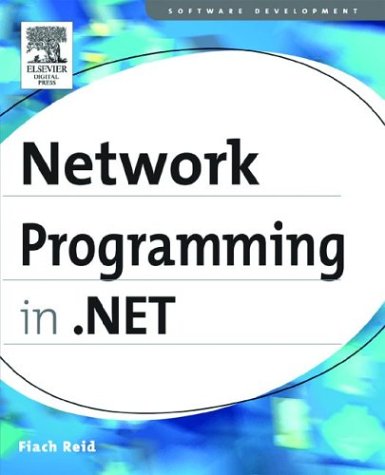
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlNamespaceMappingCollection.cs
- FileVersionInfo.cs
- MetafileHeader.cs
- Double.cs
- TextCompositionEventArgs.cs
- MonitoringDescriptionAttribute.cs
- BinaryReader.cs
- Hashtable.cs
- X509WindowsSecurityToken.cs
- SpecialNameAttribute.cs
- ErasingStroke.cs
- SBCSCodePageEncoding.cs
- DataObjectMethodAttribute.cs
- ProcessModuleCollection.cs
- LayoutUtils.cs
- WebBrowser.cs
- QueryOutputWriter.cs
- ProfileManager.cs
- SafeRightsManagementSessionHandle.cs
- Qualifier.cs
- SqlGenericUtil.cs
- ListView.cs
- XsltSettings.cs
- TagMapInfo.cs
- CompilerState.cs
- DataContractJsonSerializer.cs
- MultipleViewPattern.cs
- OdbcFactory.cs
- CompletionProxy.cs
- TypeConverterAttribute.cs
- Columns.cs
- ListViewSelectEventArgs.cs
- LineVisual.cs
- TreeViewDataItemAutomationPeer.cs
- MbpInfo.cs
- TextTrailingWordEllipsis.cs
- XmlObjectSerializerReadContextComplex.cs
- SystemResourceHost.cs
- ExtensionElementCollection.cs
- WebPartEditVerb.cs
- DrawListViewItemEventArgs.cs
- BrowserCapabilitiesCompiler.cs
- Socket.cs
- TagMapCollection.cs
- TextEditorSpelling.cs
- WindowsToolbarAsMenu.cs
- SemanticKeyElement.cs
- ListView.cs
- Base64WriteStateInfo.cs
- PropertySegmentSerializer.cs
- DataGridItem.cs
- SafeCryptContextHandle.cs
- ViewManager.cs
- SecurityProtocolFactory.cs
- BufferedGraphics.cs
- DriveNotFoundException.cs
- PropertyToken.cs
- KeyValueConfigurationCollection.cs
- _SslStream.cs
- DataGridViewSelectedColumnCollection.cs
- __Error.cs
- WriteTimeStream.cs
- GestureRecognitionResult.cs
- ConfigurationManagerInternalFactory.cs
- EntryIndex.cs
- ExtenderControl.cs
- InvokePattern.cs
- TimeSpanSecondsConverter.cs
- PathFigureCollectionConverter.cs
- DesignerTransaction.cs
- SecurityPolicySection.cs
- AttributeUsageAttribute.cs
- HtmlWindow.cs
- ObjectDataSourceView.cs
- WebZone.cs
- SqlBooleanMismatchVisitor.cs
- FillRuleValidation.cs
- BaseValidator.cs
- VersionPair.cs
- GroupByQueryOperator.cs
- ListManagerBindingsCollection.cs
- PropertyItemInternal.cs
- PenCursorManager.cs
- ImageList.cs
- DbTransaction.cs
- SamlAction.cs
- namescope.cs
- WebPartTracker.cs
- Base64Stream.cs
- WebPartDisplayModeCollection.cs
- HttpValueCollection.cs
- ModifiableIteratorCollection.cs
- XmlILAnnotation.cs
- XmlDataDocument.cs
- Oci.cs
- entityreference_tresulttype.cs
- UIElementIsland.cs
- Visual.cs
- DecimalFormatter.cs
- ToolStripItemRenderEventArgs.cs