Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Collections / Generic / DebugView.cs / 1 / DebugView.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Collections.ObjectModel; using System.Security.Permissions; using System.Diagnostics; // // VS IDE can't differentiate between types with the same name from different // assembly. So we need to use different names for collection debug view for // collections in mscorlib.dll and system.dll. // internal sealed class Mscorlib_CollectionDebugView{ private ICollection collection; public Mscorlib_CollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryKeyCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryValueCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryDebugView { private IDictionary dict; public Mscorlib_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.dictionary); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_KeyedCollectionDebugView { private KeyedCollection kc; public Mscorlib_KeyedCollectionDebugView(KeyedCollection keyedCollection) { if (keyedCollection == null) { throw new ArgumentNullException("keyedCollection"); } kc = keyedCollection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[kc.Count]; kc.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Collections.ObjectModel; using System.Security.Permissions; using System.Diagnostics; // // VS IDE can't differentiate between types with the same name from different // assembly. So we need to use different names for collection debug view for // collections in mscorlib.dll and system.dll. // internal sealed class Mscorlib_CollectionDebugView { private ICollection collection; public Mscorlib_CollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryKeyCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryValueCollectionDebugView { private ICollection collection; public Mscorlib_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_DictionaryDebugView { private IDictionary dict; public Mscorlib_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.dictionary); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class Mscorlib_KeyedCollectionDebugView { private KeyedCollection kc; public Mscorlib_KeyedCollectionDebugView(KeyedCollection keyedCollection) { if (keyedCollection == null) { throw new ArgumentNullException("keyedCollection"); } kc = keyedCollection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[kc.Count]; kc.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
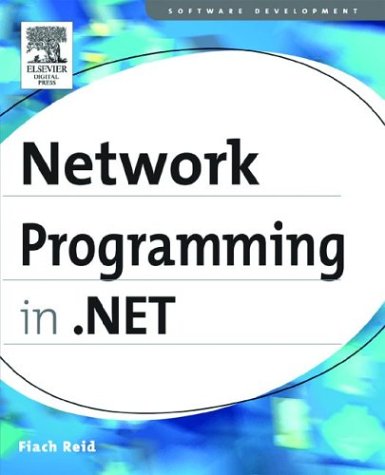
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResXResourceWriter.cs
- x509utils.cs
- _SafeNetHandles.cs
- NestedContainer.cs
- CodeNamespace.cs
- DescriptionAttribute.cs
- TrackingServices.cs
- MeshGeometry3D.cs
- QueryTaskGroupState.cs
- DataErrorValidationRule.cs
- RightsManagementInformation.cs
- ScriptRegistrationManager.cs
- Variant.cs
- EmptyElement.cs
- DecoderReplacementFallback.cs
- HttpHeaderCollection.cs
- peersecuritysettings.cs
- TemplateContent.cs
- CryptoApi.cs
- SQLBinaryStorage.cs
- PassportAuthentication.cs
- SourceFileBuildProvider.cs
- SingleSelectRootGridEntry.cs
- MetaChildrenColumn.cs
- EntityViewContainer.cs
- GenericTextProperties.cs
- MasterPageCodeDomTreeGenerator.cs
- NumberFormatInfo.cs
- VolatileEnlistmentState.cs
- TransactionChannel.cs
- SimpleApplicationHost.cs
- IISMapPath.cs
- OleDbConnection.cs
- PngBitmapDecoder.cs
- RuleEngine.cs
- TraceEventCache.cs
- WebPartRestoreVerb.cs
- objectresult_tresulttype.cs
- TemplateXamlParser.cs
- xmlglyphRunInfo.cs
- ChunkedMemoryStream.cs
- FilterableAttribute.cs
- TreeViewImageGenerator.cs
- WeakEventTable.cs
- CollectionChange.cs
- OracleRowUpdatingEventArgs.cs
- _SslSessionsCache.cs
- EventWaitHandle.cs
- FixedDocument.cs
- InkPresenter.cs
- XsltArgumentList.cs
- SelectionWordBreaker.cs
- DocumentXPathNavigator.cs
- StringFreezingAttribute.cs
- PointCollectionValueSerializer.cs
- AdapterDictionary.cs
- FlowchartDesigner.Helpers.cs
- TraceInternal.cs
- CodeChecksumPragma.cs
- PixelShader.cs
- _ProxyChain.cs
- WmpBitmapDecoder.cs
- AvtEvent.cs
- ChunkedMemoryStream.cs
- WindowsRebar.cs
- TextContainerHelper.cs
- ListControlConvertEventArgs.cs
- PnrpPermission.cs
- Ops.cs
- SecurityPolicySection.cs
- VectorConverter.cs
- HwndSubclass.cs
- WebMessageBodyStyleHelper.cs
- Optimizer.cs
- DataRelationPropertyDescriptor.cs
- LayoutTable.cs
- PeerReferralPolicy.cs
- WindowsAuthenticationEventArgs.cs
- FixedSOMContainer.cs
- Menu.cs
- OrderedDictionary.cs
- PackWebRequest.cs
- CompositeTypefaceMetrics.cs
- WindowsFormsHostAutomationPeer.cs
- EnumType.cs
- HasCopySemanticsAttribute.cs
- ArraySet.cs
- SqlProvider.cs
- EmbeddedObject.cs
- NamedPermissionSet.cs
- OutKeywords.cs
- AsymmetricSignatureFormatter.cs
- EncryptedKey.cs
- EncodingTable.cs
- TraceSource.cs
- PeerNameRecordCollection.cs
- FlowLayoutPanel.cs
- Matrix3DConverter.cs
- HttpListenerException.cs
- Pair.cs