Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripDropDownButton.cs / 1305376 / ToolStripDropDownButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Collections; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Drawing.Imaging; using System.Threading; using System.Diagnostics; using System.Windows.Forms.ButtonInternal; using System.Windows.Forms.Layout; using System.Security.Permissions; using System.Security; using System.Windows.Forms.Design; ////// /// A ToolStripButton that can display a popup. /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.ToolStrip | ToolStripItemDesignerAvailability.StatusStrip)] public class ToolStripDropDownButton : ToolStripDropDownItem { private bool showDropDownArrow = true; private byte openMouseId = 0; ////// /// Constructs a ToolStripButton that can display a popup. /// public ToolStripDropDownButton() { Initialize(); } public ToolStripDropDownButton(string text):base(text,null,(EventHandler)null) { Initialize(); } public ToolStripDropDownButton(Image image):base(null,image,(EventHandler)null) { Initialize(); } public ToolStripDropDownButton(string text, Image image):base(text,image,(EventHandler)null) { Initialize(); } public ToolStripDropDownButton(string text, Image image, EventHandler onClick):base(text,image,onClick) { Initialize(); } public ToolStripDropDownButton(string text, Image image, EventHandler onClick, string name) :base(text,image,onClick,name){ Initialize(); } public ToolStripDropDownButton(string text, Image image, params ToolStripItem[] dropDownItems):base(text,image,dropDownItems) { Initialize(); } [DefaultValue(true)] public new bool AutoToolTip { get { return base.AutoToolTip; } set { base.AutoToolTip = value; } } protected override bool DefaultAutoToolTip { get { return true; } } ///[ DefaultValue(true), SRDescription(SR.ToolStripDropDownButtonShowDropDownArrowDescr), SRCategory(SR.CatAppearance) ] public bool ShowDropDownArrow { get { return showDropDownArrow; } set { if (showDropDownArrow != value) { showDropDownArrow = value; this.InvalidateItemLayout(PropertyNames.ShowDropDownArrow); } } } /// /// Creates an instance of the object that defines how image and text /// gets laid out in the ToolStripItem /// internal override ToolStripItemInternalLayout CreateInternalLayout() { return new ToolStripDropDownButtonInternalLayout(this); } protected override ToolStripDropDown CreateDefaultDropDown() { // AutoGenerate a Winbar DropDown - set the property so we hook events return new ToolStripDropDownMenu(this, /*isAutoGenerated=*/true); } ////// Called by all constructors of ToolStripButton. /// private void Initialize() { SupportsSpaceKey = true; } ////// /// Overriden to invoke displaying the popup. /// protected override void OnMouseDown(MouseEventArgs e) { if ((Control.ModifierKeys != Keys.Alt) && (e.Button == MouseButtons.Left)) { if (DropDown.Visible) { ToolStripManager.ModalMenuFilter.CloseActiveDropDown(DropDown, ToolStripDropDownCloseReason.AppClicked); } else { // opening should happen on mouse down. Debug.Assert(ParentInternal != null, "Parent is null here, not going to get accurate ID"); openMouseId = (ParentInternal == null) ? (byte)0: ParentInternal.GetMouseId(); this.ShowDropDown(/*mousePush =*/true); } } base.OnMouseDown(e); } protected override void OnMouseUp(MouseEventArgs e) { if ((Control.ModifierKeys != Keys.Alt) && (e.Button == MouseButtons.Left)) { Debug.Assert(ParentInternal != null, "Parent is null here, not going to get accurate ID"); byte closeMouseId = (ParentInternal == null) ? (byte)0: ParentInternal.GetMouseId(); if (closeMouseId != openMouseId) { openMouseId = 0; // reset the mouse id, we should never get this value from toolstrip. ToolStripManager.ModalMenuFilter.CloseActiveDropDown(DropDown, ToolStripDropDownCloseReason.AppClicked); Select(); } } base.OnMouseUp(e); } protected override void OnMouseLeave(EventArgs e) { openMouseId = 0; // reset the mouse id, we should never get this value from toolstrip. base.OnMouseLeave(e); } ////// /// Inheriting classes should override this method to handle this event. /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null) { ToolStripRenderer renderer = this.Renderer; Graphics g = e.Graphics; renderer.DrawDropDownButtonBackground(new ToolStripItemRenderEventArgs(e.Graphics, this)); if ((DisplayStyle & ToolStripItemDisplayStyle.Image) == ToolStripItemDisplayStyle.Image) { renderer.DrawItemImage(new ToolStripItemImageRenderEventArgs(g, this, InternalLayout.ImageRectangle)); } if ((DisplayStyle & ToolStripItemDisplayStyle.Text) == ToolStripItemDisplayStyle.Text) { renderer.DrawItemText(new ToolStripItemTextRenderEventArgs(g, this, this.Text, InternalLayout.TextRectangle, this.ForeColor, this.Font, InternalLayout.TextFormat)); } if (ShowDropDownArrow) { ToolStripDropDownButton.ToolStripDropDownButtonInternalLayout layout = InternalLayout as ToolStripDropDownButtonInternalLayout; Rectangle dropDownArrowRect = (layout != null) ? layout.DropDownArrowRect :Rectangle.Empty; Color arrowColor = Enabled ? SystemColors.ControlText : SystemColors.ControlDark; renderer.DrawArrow(new ToolStripArrowRenderEventArgs(g, this,dropDownArrowRect, arrowColor, ArrowDirection.Down)); } } } ///[UIPermission(SecurityAction.LinkDemand, Window=UIPermissionWindow.AllWindows)] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1720:AvoidTypeNamesInParameters")] // 'charCode' matches control.cs protected internal override bool ProcessMnemonic(char charCode) { // checking IsMnemonic is not necesssary - toolstrip does this for us. if (HasDropDownItems) { Select(); ShowDropDown(); return true; } return false; } internal class ToolStripDropDownButtonInternalLayout : ToolStripItemInternalLayout { private ToolStripDropDownButton ownerItem; private static Size dropDownArrowSize = new Size(5,3); private static Padding dropDownArrowPadding = new Padding(2); private Rectangle dropDownArrowRect = Rectangle.Empty; public ToolStripDropDownButtonInternalLayout(ToolStripDropDownButton ownerItem) : base(ownerItem) { this.ownerItem = ownerItem; } public override Size GetPreferredSize(Size constrainingSize) { Size preferredSize = base.GetPreferredSize(constrainingSize); if (ownerItem.ShowDropDownArrow) { if (ownerItem.TextDirection == ToolStripTextDirection.Horizontal) { preferredSize.Width += DropDownArrowRect.Width + dropDownArrowPadding.Horizontal; } else { preferredSize.Height += DropDownArrowRect.Height + dropDownArrowPadding.Vertical; } } return preferredSize; } protected override ToolStripItemLayoutOptions CommonLayoutOptions() { ToolStripItemLayoutOptions options = base.CommonLayoutOptions(); if (ownerItem.ShowDropDownArrow) { if (ownerItem.TextDirection == ToolStripTextDirection.Horizontal) { // We're rendering horizontal.... make sure to take care of RTL issues. int widthOfDropDown = dropDownArrowSize.Width + dropDownArrowPadding.Horizontal; options.client.Width -= widthOfDropDown; if (ownerItem.RightToLeft == RightToLeft.Yes) { // if RightToLeft.Yes: [ v | rest of drop down button ] options.client.Offset(widthOfDropDown, 0); dropDownArrowRect = new Rectangle(dropDownArrowPadding.Left,0, dropDownArrowSize.Width, ownerItem.Bounds.Height); } else { // if RightToLeft.No [ rest of drop down button | v ] dropDownArrowRect = new Rectangle(options.client.Right,0, dropDownArrowSize.Width, ownerItem.Bounds.Height); } } else { // else we're rendering vertically. int heightOfDropDown = dropDownArrowSize.Height + dropDownArrowPadding.Vertical; options.client.Height -= heightOfDropDown; // [ rest of button / v] dropDownArrowRect = new Rectangle(0,options.client.Bottom + dropDownArrowPadding.Top, ownerItem.Bounds.Width-1, dropDownArrowSize.Height); } } return options; } public Rectangle DropDownArrowRect { get { return dropDownArrowRect; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
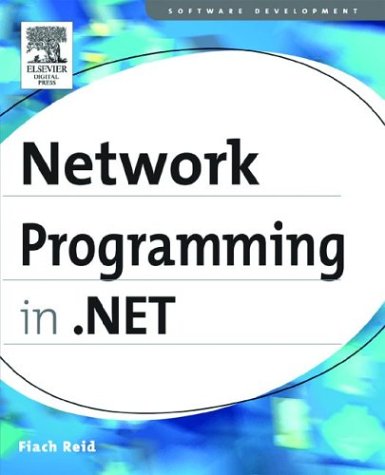
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextElementCollectionHelper.cs
- TypeForwardedToAttribute.cs
- RSAOAEPKeyExchangeDeformatter.cs
- GeneralTransform3DGroup.cs
- EdmItemCollection.cs
- XPathDescendantIterator.cs
- RangeValueProviderWrapper.cs
- XpsFilter.cs
- RepeatButton.cs
- ContainerUtilities.cs
- DataGridRow.cs
- AuthenticationModuleElement.cs
- InvalidCommandTreeException.cs
- WorkflowInstanceProvider.cs
- SafeCryptoKeyHandle.cs
- cookiecollection.cs
- BindMarkupExtensionSerializer.cs
- SqlHelper.cs
- SqlCharStream.cs
- XmlAnyElementAttribute.cs
- ToolStripLocationCancelEventArgs.cs
- Certificate.cs
- MD5.cs
- XmlNamespaceMappingCollection.cs
- ConnectionModeReader.cs
- QueryOperationResponseOfT.cs
- LogRestartAreaEnumerator.cs
- UnsafeNativeMethodsMilCoreApi.cs
- arclist.cs
- CompilerScope.Storage.cs
- XmlAttributeAttribute.cs
- SelectionWordBreaker.cs
- SqlCacheDependencySection.cs
- LayoutInformation.cs
- TransformGroup.cs
- XmlSchemaCollection.cs
- DesignerOptionService.cs
- IItemContainerGenerator.cs
- XappLauncher.cs
- AdjustableArrowCap.cs
- PatternMatcher.cs
- odbcmetadatacollectionnames.cs
- WeakEventTable.cs
- KeySpline.cs
- XmlSerializableServices.cs
- TimeSpanMinutesConverter.cs
- MatrixStack.cs
- SortExpressionBuilder.cs
- DataServiceBehavior.cs
- recordstatefactory.cs
- ActionItem.cs
- ErrorInfoXmlDocument.cs
- DecimalAnimationBase.cs
- HandlerFactoryCache.cs
- InvokeProviderWrapper.cs
- FrameworkName.cs
- TextRunCache.cs
- PathSegmentCollection.cs
- GlyphShapingProperties.cs
- FilteredDataSetHelper.cs
- Transform3D.cs
- AppDomainFactory.cs
- DataControlFieldsEditor.cs
- StringUtil.cs
- PropertyChangingEventArgs.cs
- TakeQueryOptionExpression.cs
- SqlMethodTransformer.cs
- PointAnimationClockResource.cs
- SspiNegotiationTokenProvider.cs
- MD5CryptoServiceProvider.cs
- DbCommandTree.cs
- HtmlTableCell.cs
- DashStyles.cs
- CqlErrorHelper.cs
- WinEventWrap.cs
- CompositeDuplexElement.cs
- LayoutInformation.cs
- D3DImage.cs
- AppSecurityManager.cs
- FeatureSupport.cs
- XmlSchemaAll.cs
- SqlDataReaderSmi.cs
- XmlSerializationReader.cs
- RepeaterItemCollection.cs
- MasterPageBuildProvider.cs
- Compiler.cs
- ReferenceEqualityComparer.cs
- HiddenFieldPageStatePersister.cs
- DataDocumentXPathNavigator.cs
- ExpressionBindings.cs
- ReversePositionQuery.cs
- XmlDataContract.cs
- MetadataImporter.cs
- DefinitionBase.cs
- XmlUtf8RawTextWriter.cs
- SafeFindHandle.cs
- AuthenticationException.cs
- PageFunction.cs
- DBCommand.cs
- TextAdaptor.cs