Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / RayMeshGeometry3DHitTestResult.cs / 1305600 / RayMeshGeometry3DHitTestResult.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // History: // 06/22/2005 : [....] - Integrated from RayHitTestResult. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; namespace System.Windows.Media.Media3D { ////// The HitTestResult of a Visual3D.HitTest(...) where the parameter /// was a RayHitTestParameter and the ray intersected a MeshGeometry3D. /// /// NOTE: This might have originated as a PointHitTest on a 2D Visual /// which was extended into 3D. /// public sealed class RayMeshGeometry3DHitTestResult : RayHitTestResult { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal RayMeshGeometry3DHitTestResult( Visual3D visualHit, Model3D modelHit, MeshGeometry3D meshHit, Point3D pointHit, double distanceToRayOrigin, int vertexIndex1, int vertexIndex2, int vertexIndex3, Point barycentricCoordinate) : base (visualHit, modelHit) { _meshHit = meshHit; _pointHit = pointHit; _distanceToRayOrigin = distanceToRayOrigin; _vertexIndex1 = vertexIndex1; _vertexIndex2 = vertexIndex2; _vertexIndex3 = vertexIndex3; _barycentricCoordinate = barycentricCoordinate; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// This is a point in 3-space at which the ray intersected /// the geometry of the hit Model3D. This point is in the /// coordinate system of the Visual3D. /// public override Point3D PointHit { get { return _pointHit; } } ////// This is the distance between the ray's origin and the /// point the PointHit. /// public override double DistanceToRayOrigin { get { return _distanceToRayOrigin; } } ////// Index of the 1st vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex1 { get { return _vertexIndex1; } } ////// Index of the 2nd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex2 { get { return _vertexIndex2; } } ////// Index of the 3rd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex3 { get { return _vertexIndex3; } } ///public double VertexWeight1 { get { return 1 - VertexWeight2 - VertexWeight3; } } /// public double VertexWeight2 { get { return _barycentricCoordinate.X; } } /// public double VertexWeight3 { get { return _barycentricCoordinate.Y; } } /// /// The MeshGeometry3D which was intersected by the ray. /// public MeshGeometry3D MeshHit { get { return _meshHit; } } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- internal override void SetDistanceToRayOrigin(double distance) { _distanceToRayOrigin = distance; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private double _distanceToRayOrigin; // Not readonly because it can be adjusted after construction. private readonly int _vertexIndex1; private readonly int _vertexIndex2; private readonly int _vertexIndex3; private readonly Point _barycentricCoordinate; private readonly MeshGeometry3D _meshHit; private readonly Point3D _pointHit; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // History: // 06/22/2005 : [....] - Integrated from RayHitTestResult. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; namespace System.Windows.Media.Media3D { ////// The HitTestResult of a Visual3D.HitTest(...) where the parameter /// was a RayHitTestParameter and the ray intersected a MeshGeometry3D. /// /// NOTE: This might have originated as a PointHitTest on a 2D Visual /// which was extended into 3D. /// public sealed class RayMeshGeometry3DHitTestResult : RayHitTestResult { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal RayMeshGeometry3DHitTestResult( Visual3D visualHit, Model3D modelHit, MeshGeometry3D meshHit, Point3D pointHit, double distanceToRayOrigin, int vertexIndex1, int vertexIndex2, int vertexIndex3, Point barycentricCoordinate) : base (visualHit, modelHit) { _meshHit = meshHit; _pointHit = pointHit; _distanceToRayOrigin = distanceToRayOrigin; _vertexIndex1 = vertexIndex1; _vertexIndex2 = vertexIndex2; _vertexIndex3 = vertexIndex3; _barycentricCoordinate = barycentricCoordinate; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// This is a point in 3-space at which the ray intersected /// the geometry of the hit Model3D. This point is in the /// coordinate system of the Visual3D. /// public override Point3D PointHit { get { return _pointHit; } } ////// This is the distance between the ray's origin and the /// point the PointHit. /// public override double DistanceToRayOrigin { get { return _distanceToRayOrigin; } } ////// Index of the 1st vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex1 { get { return _vertexIndex1; } } ////// Index of the 2nd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex2 { get { return _vertexIndex2; } } ////// Index of the 3rd vertex of the triangle which was intersected. /// Use this to retrieve the position, texturecoordinate, etc. from /// the MeshHit. /// public int VertexIndex3 { get { return _vertexIndex3; } } ///public double VertexWeight1 { get { return 1 - VertexWeight2 - VertexWeight3; } } /// public double VertexWeight2 { get { return _barycentricCoordinate.X; } } /// public double VertexWeight3 { get { return _barycentricCoordinate.Y; } } /// /// The MeshGeometry3D which was intersected by the ray. /// public MeshGeometry3D MeshHit { get { return _meshHit; } } //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- internal override void SetDistanceToRayOrigin(double distance) { _distanceToRayOrigin = distance; } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private double _distanceToRayOrigin; // Not readonly because it can be adjusted after construction. private readonly int _vertexIndex1; private readonly int _vertexIndex2; private readonly int _vertexIndex3; private readonly Point _barycentricCoordinate; private readonly MeshGeometry3D _meshHit; private readonly Point3D _pointHit; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
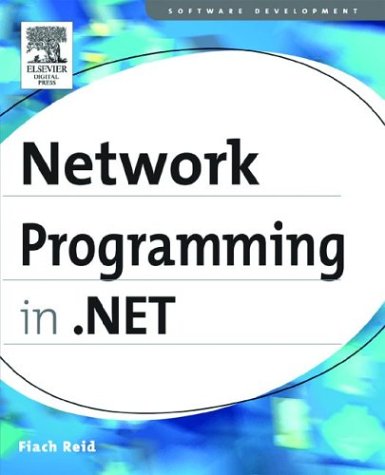
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BehaviorEditorPart.cs
- MappingMetadataHelper.cs
- ToolStripOverflowButton.cs
- WebProxyScriptElement.cs
- Transform3DCollection.cs
- GeometryGroup.cs
- BaseDataListComponentEditor.cs
- SpecularMaterial.cs
- dtdvalidator.cs
- TaskDesigner.cs
- CollectionViewGroup.cs
- DbConnectionPoolGroup.cs
- ContentPathSegment.cs
- NativeCompoundFileAPIs.cs
- PathParser.cs
- StylusEditingBehavior.cs
- ListSurrogate.cs
- ListItemCollection.cs
- MenuAdapter.cs
- Privilege.cs
- GeneralTransform.cs
- Update.cs
- UpDownBase.cs
- GenericTypeParameterBuilder.cs
- EncoderBestFitFallback.cs
- Message.cs
- ConfigurationSectionGroup.cs
- XmlSerializationReader.cs
- SelectedPathEditor.cs
- ClusterRegistryConfigurationProvider.cs
- BaseParser.cs
- EventListener.cs
- FilterEventArgs.cs
- ImageIndexEditor.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- TreeNodeCollection.cs
- AvTraceDetails.cs
- HttpRequest.cs
- XmlAttributeAttribute.cs
- BitConverter.cs
- SchemaReference.cs
- AffineTransform3D.cs
- HandleCollector.cs
- Variable.cs
- NamespaceMapping.cs
- WebServicesInteroperability.cs
- EnumerableWrapperWeakToStrong.cs
- GraphicsPath.cs
- SqlReferenceCollection.cs
- InProcStateClientManager.cs
- ColumnHeader.cs
- Socket.cs
- BitmapScalingModeValidation.cs
- DoubleLinkList.cs
- ACE.cs
- RecognizedWordUnit.cs
- WebPartTransformerAttribute.cs
- TCEAdapterGenerator.cs
- HandleRef.cs
- EnterpriseServicesHelper.cs
- Int16Storage.cs
- ProjectionAnalyzer.cs
- ErrorWrapper.cs
- DrawingDrawingContext.cs
- HashMembershipCondition.cs
- EdmItemCollection.cs
- ToolCreatedEventArgs.cs
- Model3D.cs
- InvalidDocumentContentsException.cs
- LocatorPartList.cs
- IInstanceContextProvider.cs
- SHA1.cs
- SqlTriggerContext.cs
- QueryComponents.cs
- RegisteredExpandoAttribute.cs
- SmiTypedGetterSetter.cs
- WorkflowWebHostingModule.cs
- JapaneseLunisolarCalendar.cs
- IntranetCredentialPolicy.cs
- Normalizer.cs
- XmlTextAttribute.cs
- ContextProperty.cs
- ParallelDesigner.cs
- TextTreeDeleteContentUndoUnit.cs
- Propagator.JoinPropagator.cs
- DefaultAuthorizationContext.cs
- AssemblySettingAttributes.cs
- RuntimeArgumentHandle.cs
- Pkcs7Signer.cs
- WebSysDisplayNameAttribute.cs
- EncoderReplacementFallback.cs
- ParallelEnumerableWrapper.cs
- ipaddressinformationcollection.cs
- InkCanvasFeedbackAdorner.cs
- OneToOneMappingSerializer.cs
- IssuedTokenClientBehaviorsElement.cs
- HashHelper.cs
- HttpFileCollection.cs
- ConditionalBranch.cs
- DiscriminatorMap.cs