Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Generated / DoubleCollectionConverter.cs / 1305600 / DoubleCollectionConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media { ////// DoubleCollectionConverter - Converter class for converting instances of other types to and from DoubleCollection instances /// public sealed class DoubleCollectionConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a DoubleCollection from the given object. /// ////// The DoubleCollection which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a DoubleCollection. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of DoubleCollection. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return DoubleCollection.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of DoubleCollection to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of DoubleCollection, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the DoubleCollection instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is DoubleCollection) { DoubleCollection instance = (DoubleCollection)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media { ////// DoubleCollectionConverter - Converter class for converting instances of other types to and from DoubleCollection instances /// public sealed class DoubleCollectionConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a DoubleCollection from the given object. /// ////// The DoubleCollection which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a DoubleCollection. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of DoubleCollection. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return DoubleCollection.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of DoubleCollection to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of DoubleCollection, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the DoubleCollection instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is DoubleCollection) { DoubleCollection instance = (DoubleCollection)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
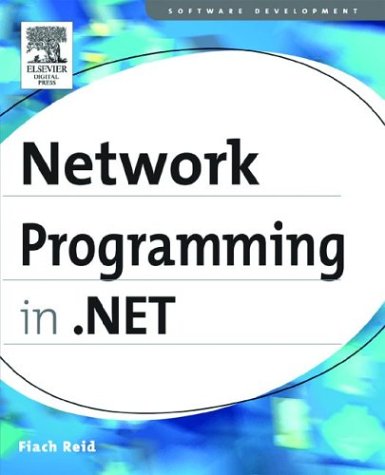
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TraceInternal.cs
- DefaultProxySection.cs
- ReliabilityContractAttribute.cs
- QueuePathEditor.cs
- OdbcConnectionOpen.cs
- TraceXPathNavigator.cs
- RegexWorker.cs
- TextTreeDeleteContentUndoUnit.cs
- SmiXetterAccessMap.cs
- LineBreakRecord.cs
- PriorityItem.cs
- XmlTextAttribute.cs
- CacheHelper.cs
- EventEntry.cs
- ManagedIStream.cs
- EntityTransaction.cs
- LockedAssemblyCache.cs
- BoundingRectTracker.cs
- XPathCompiler.cs
- SharedPerformanceCounter.cs
- ModelPerspective.cs
- InvariantComparer.cs
- SynthesizerStateChangedEventArgs.cs
- ConfigurationManagerInternalFactory.cs
- ReflectTypeDescriptionProvider.cs
- ValidationSummary.cs
- PageCatalogPart.cs
- XmlValueConverter.cs
- BamlRecordWriter.cs
- ConnectionOrientedTransportChannelListener.cs
- SyndicationElementExtensionCollection.cs
- RoutedEventHandlerInfo.cs
- NavigateEvent.cs
- GPPOINTF.cs
- DispatcherFrame.cs
- PointConverter.cs
- ObjectContextServiceProvider.cs
- Gdiplus.cs
- KeyPullup.cs
- Bezier.cs
- HttpVersion.cs
- WebFaultException.cs
- RefType.cs
- DefaultHttpHandler.cs
- LinqDataSourceDisposeEventArgs.cs
- Itemizer.cs
- CategoryNameCollection.cs
- RevocationPoint.cs
- DataRecordInfo.cs
- RoamingStoreFileUtility.cs
- NamespaceList.cs
- Emitter.cs
- SliderAutomationPeer.cs
- HostedNamedPipeTransportManager.cs
- NegotiationTokenAuthenticatorStateCache.cs
- EntityDataSourceEntityTypeFilterItem.cs
- RenderDataDrawingContext.cs
- LeafCellTreeNode.cs
- InfoCardRSACryptoProvider.cs
- ColorInterpolationModeValidation.cs
- ReachDocumentReferenceSerializer.cs
- StringInfo.cs
- SqlUnionizer.cs
- RemotingSurrogateSelector.cs
- IFlowDocumentViewer.cs
- SamlSubjectStatement.cs
- EmbeddedMailObjectsCollection.cs
- DocumentSignatureManager.cs
- TemplateXamlTreeBuilder.cs
- EqualityComparer.cs
- ConstructorNeedsTagAttribute.cs
- If.cs
- EventHandlersDesigner.cs
- HwndSubclass.cs
- GetImportFileNameRequest.cs
- DataGridViewRowStateChangedEventArgs.cs
- MinMaxParagraphWidth.cs
- FontSizeConverter.cs
- ClientSettingsProvider.cs
- QuadraticBezierSegment.cs
- ConstructorBuilder.cs
- ExpandedProjectionNode.cs
- DecimalAnimation.cs
- SelectorAutomationPeer.cs
- XmlUtil.cs
- ObservableDictionary.cs
- CacheAxisQuery.cs
- DetailsViewPageEventArgs.cs
- FlowDocumentReaderAutomationPeer.cs
- ResourcesBuildProvider.cs
- ClientTargetSection.cs
- UnicastIPAddressInformationCollection.cs
- Psha1DerivedKeyGeneratorHelper.cs
- SQLInt64Storage.cs
- SecurityMessageProperty.cs
- HitTestFilterBehavior.cs
- HebrewCalendar.cs
- GridViewColumnCollectionChangedEventArgs.cs
- SafeRightsManagementHandle.cs
- RequestQueryParser.cs