Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / KeySplineConverter.cs / 1305600 / KeySplineConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001-2003 // // File: KeySplineConverter.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Windows.Media.Animation; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// PointConverter - Converter class for converting instances of other types to Point instances /// ///public class KeySplineConverter : TypeConverter { /// /// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptor, Type destinationType) { if (destinationType == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// public override object ConvertFrom( ITypeDescriptorContext context, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (value == null) { throw new NotSupportedException(SR.Get(SRID.Converter_ConvertFromNotSupported)); } TokenizerHelper th = new TokenizerHelper(stringValue, cultureInfo); return new KeySpline( Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo)); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs), null is a valid value /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for KeySpline, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { KeySpline keySpline = value as KeySpline; if (keySpline != null && destinationType != null) { if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(KeySpline).GetConstructor(new Type[] { typeof(double), typeof(double), typeof(double), typeof(double) }); return new InstanceDescriptor(ci, new object[] { keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y }); } else if (destinationType == typeof(string)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'cultureInfo.TextInfo' to this public method must be validated: A null-dereference can occur here. return String.Format( cultureInfo, "{0}{4}{1}{4}{2}{4}{3}", keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y, cultureInfo != null ? cultureInfo.TextInfo.ListSeparator : CultureInfo.InvariantCulture.TextInfo.ListSeparator); #pragma warning restore 56506 } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001-2003 // // File: KeySplineConverter.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using MS.Internal; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Windows.Media.Animation; using System.Security; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// PointConverter - Converter class for converting instances of other types to Point instances /// ///public class KeySplineConverter : TypeConverter { /// /// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptor, Type destinationType) { if (destinationType == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// public override object ConvertFrom( ITypeDescriptorContext context, CultureInfo cultureInfo, object value) { string stringValue = value as string; if (value == null) { throw new NotSupportedException(SR.Get(SRID.Converter_ConvertFromNotSupported)); } TokenizerHelper th = new TokenizerHelper(stringValue, cultureInfo); return new KeySpline( Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo), Convert.ToDouble(th.NextTokenRequired(), cultureInfo)); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs), null is a valid value /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for KeySpline, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { KeySpline keySpline = value as KeySpline; if (keySpline != null && destinationType != null) { if (destinationType == typeof(InstanceDescriptor)) { ConstructorInfo ci = typeof(KeySpline).GetConstructor(new Type[] { typeof(double), typeof(double), typeof(double), typeof(double) }); return new InstanceDescriptor(ci, new object[] { keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y }); } else if (destinationType == typeof(string)) { #pragma warning disable 56506 // Suppress presharp warning: Parameter 'cultureInfo.TextInfo' to this public method must be validated: A null-dereference can occur here. return String.Format( cultureInfo, "{0}{4}{1}{4}{2}{4}{3}", keySpline.ControlPoint1.X, keySpline.ControlPoint1.Y, keySpline.ControlPoint2.X, keySpline.ControlPoint2.Y, cultureInfo != null ? cultureInfo.TextInfo.ListSeparator : CultureInfo.InvariantCulture.TextInfo.ListSeparator); #pragma warning restore 56506 } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
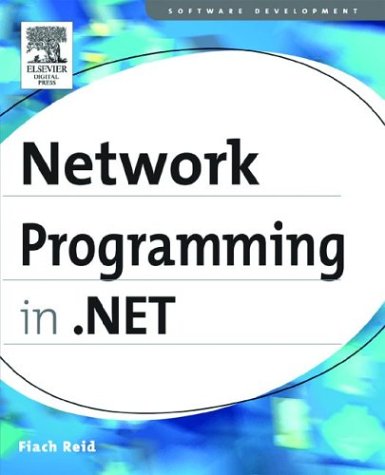
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileChangesMonitor.cs
- DataContract.cs
- Geometry.cs
- BaseTemplateParser.cs
- SmtpReplyReaderFactory.cs
- RegexTypeEditor.cs
- WindowsListViewItemCheckBox.cs
- RegexCharClass.cs
- HiddenFieldDesigner.cs
- ClientScriptItemCollection.cs
- DBCommand.cs
- MultiSelectRootGridEntry.cs
- RepeaterCommandEventArgs.cs
- Attachment.cs
- WebPartDescriptionCollection.cs
- HttpWebRequest.cs
- RemoteWebConfigurationHostServer.cs
- EntityProxyFactory.cs
- IisTraceWebEventProvider.cs
- CheckBoxFlatAdapter.cs
- TableLayoutStyleCollection.cs
- XmlDownloadManager.cs
- TabItemAutomationPeer.cs
- DrawingCollection.cs
- BridgeDataReader.cs
- ViewBase.cs
- DocumentApplicationState.cs
- DataBoundControlHelper.cs
- ProgressBarAutomationPeer.cs
- DataBinder.cs
- ProgressBarRenderer.cs
- EntityDataSourceWrapperCollection.cs
- DataRecord.cs
- XhtmlConformanceSection.cs
- OleDbInfoMessageEvent.cs
- SystemNetHelpers.cs
- Image.cs
- TextDecoration.cs
- SQLGuid.cs
- PriorityItem.cs
- MsmqChannelFactory.cs
- HttpWebResponse.cs
- PropertyToken.cs
- ProvidersHelper.cs
- TextEditorLists.cs
- OutputCacheProfile.cs
- FilteredAttributeCollection.cs
- ControlBindingsCollection.cs
- EdmError.cs
- WebRequest.cs
- IgnoreSectionHandler.cs
- FocusChangedEventArgs.cs
- WsatServiceCertificate.cs
- WebDisplayNameAttribute.cs
- Converter.cs
- TimelineClockCollection.cs
- ItemMap.cs
- IMembershipProvider.cs
- TextEndOfSegment.cs
- LinkedDataMemberFieldEditor.cs
- Error.cs
- PersonalizableTypeEntry.cs
- DataGridViewCell.cs
- DBCommandBuilder.cs
- RawStylusInputCustomDataList.cs
- RowSpanVector.cs
- XmlDataSourceView.cs
- PseudoWebRequest.cs
- SessionPageStatePersister.cs
- QuaternionRotation3D.cs
- ISAPIApplicationHost.cs
- ReceiveMessageRecord.cs
- Visitors.cs
- DiscardableAttribute.cs
- TableFieldsEditor.cs
- SynchronousSendBindingElement.cs
- PanningMessageFilter.cs
- WebPartZone.cs
- entitydatasourceentitysetnameconverter.cs
- EncodedStreamFactory.cs
- X509CertificateClaimSet.cs
- ThemeInfoAttribute.cs
- CustomAttributeSerializer.cs
- SynchronousChannelMergeEnumerator.cs
- DockingAttribute.cs
- ListManagerBindingsCollection.cs
- EnumBuilder.cs
- GPPOINT.cs
- XmlAttributeProperties.cs
- DataFieldCollectionEditor.cs
- Button.cs
- ResourceKey.cs
- BatchParser.cs
- Renderer.cs
- CachedPathData.cs
- ControlType.cs
- MatchingStyle.cs
- ListBindingConverter.cs
- ExpressionServices.cs
- ConfigurationValue.cs