Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / SimplePropertyEntry.cs / 1305376 / SimplePropertyEntry.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.CodeDom; using System.Web.Compilation; using Debug=System.Web.Util.Debug; ////// PropertyEntry for simple attributes /// public class SimplePropertyEntry : PropertyEntry { private string _persistedValue; private bool _useSetAttribute; private object _value; internal SimplePropertyEntry() { } ////// // public string PersistedValue { get { return _persistedValue; } set { _persistedValue = value; } } ////// public bool UseSetAttribute { get { return _useSetAttribute; } set { _useSetAttribute = value; } } ////// public object Value { get { return _value; } set { _value = value; } } // Build the statement that assigns this property internal CodeStatement GetCodeStatement(BaseTemplateCodeDomTreeGenerator generator, CodeExpression ctrlRefExpr) { // If we don't have a type, use IAttributeAccessor.SetAttribute if (UseSetAttribute) { // e.g. ((IAttributeAccessor)__ctrl).SetAttribute("{{_name}}", "{{_value}}"); CodeMethodInvokeExpression methCallExpression = new CodeMethodInvokeExpression( new CodeCastExpression(typeof(IAttributeAccessor), ctrlRefExpr), "SetAttribute"); methCallExpression.Parameters.Add(new CodePrimitiveExpression(Name)); methCallExpression.Parameters.Add(new CodePrimitiveExpression(Value)); return new CodeExpressionStatement(methCallExpression); } CodeExpression leftExpr, rightExpr = null; if (PropertyInfo != null) { leftExpr = CodeDomUtility.BuildPropertyReferenceExpression(ctrlRefExpr, Name); } else { // In case of a field, there should only be one (unlike properties) Debug.Assert(Name.IndexOf('.') < 0, "_name.IndexOf('.') < 0"); leftExpr = new CodeFieldReferenceExpression(ctrlRefExpr, Name); } if (Type == typeof(string)) { rightExpr = generator.BuildStringPropertyExpression(this); } else { rightExpr = CodeDomUtility.GenerateExpressionForValue(PropertyInfo, Value, Type); } // Now that we have both side, add the assignment return new CodeAssignStatement(leftExpr, rightExpr); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System.CodeDom; using System.Web.Compilation; using Debug=System.Web.Util.Debug; ////// PropertyEntry for simple attributes /// public class SimplePropertyEntry : PropertyEntry { private string _persistedValue; private bool _useSetAttribute; private object _value; internal SimplePropertyEntry() { } ////// // public string PersistedValue { get { return _persistedValue; } set { _persistedValue = value; } } ////// public bool UseSetAttribute { get { return _useSetAttribute; } set { _useSetAttribute = value; } } ////// public object Value { get { return _value; } set { _value = value; } } // Build the statement that assigns this property internal CodeStatement GetCodeStatement(BaseTemplateCodeDomTreeGenerator generator, CodeExpression ctrlRefExpr) { // If we don't have a type, use IAttributeAccessor.SetAttribute if (UseSetAttribute) { // e.g. ((IAttributeAccessor)__ctrl).SetAttribute("{{_name}}", "{{_value}}"); CodeMethodInvokeExpression methCallExpression = new CodeMethodInvokeExpression( new CodeCastExpression(typeof(IAttributeAccessor), ctrlRefExpr), "SetAttribute"); methCallExpression.Parameters.Add(new CodePrimitiveExpression(Name)); methCallExpression.Parameters.Add(new CodePrimitiveExpression(Value)); return new CodeExpressionStatement(methCallExpression); } CodeExpression leftExpr, rightExpr = null; if (PropertyInfo != null) { leftExpr = CodeDomUtility.BuildPropertyReferenceExpression(ctrlRefExpr, Name); } else { // In case of a field, there should only be one (unlike properties) Debug.Assert(Name.IndexOf('.') < 0, "_name.IndexOf('.') < 0"); leftExpr = new CodeFieldReferenceExpression(ctrlRefExpr, Name); } if (Type == typeof(string)) { rightExpr = generator.BuildStringPropertyExpression(this); } else { rightExpr = CodeDomUtility.GenerateExpressionForValue(PropertyInfo, Value, Type); } // Now that we have both side, add the assignment return new CodeAssignStatement(leftExpr, rightExpr); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
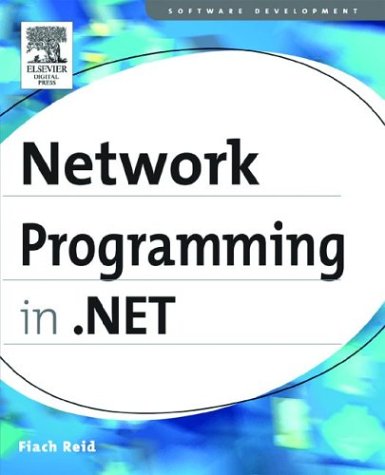
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Label.cs
- XNameConverter.cs
- EntityDataSourceConfigureObjectContext.cs
- UriTemplateVariableQueryValue.cs
- AudienceUriMode.cs
- SocketException.cs
- SurrogateSelector.cs
- DetailsViewUpdatedEventArgs.cs
- FontFamilyValueSerializer.cs
- SynchronizedInputHelper.cs
- PartialArray.cs
- WindowsIdentity.cs
- DrawingImage.cs
- DataGridPagerStyle.cs
- loginstatus.cs
- PasswordTextNavigator.cs
- TracedNativeMethods.cs
- JoinGraph.cs
- ObjectParameter.cs
- StreamGeometryContext.cs
- CryptoKeySecurity.cs
- mansign.cs
- TextRangeAdaptor.cs
- DefaultWorkflowTransactionService.cs
- ToolStripCustomTypeDescriptor.cs
- TreeWalker.cs
- AppDomainAttributes.cs
- BindableAttribute.cs
- IdentityHolder.cs
- KnownTypesHelper.cs
- Route.cs
- Debug.cs
- DataGridViewRowEventArgs.cs
- xmlsaver.cs
- BaseTemplateCodeDomTreeGenerator.cs
- GradientStop.cs
- CombinedGeometry.cs
- ContainerFilterService.cs
- ComboBox.cs
- DBConnection.cs
- WindowProviderWrapper.cs
- elementinformation.cs
- PeerTransportBindingElement.cs
- FontStyle.cs
- Attributes.cs
- _TLSstream.cs
- HierarchicalDataTemplate.cs
- Query.cs
- ResourceReferenceKeyNotFoundException.cs
- NativeMethods.cs
- AvtEvent.cs
- CompiledQuery.cs
- MimeMapping.cs
- HtmlTextArea.cs
- AnnotationService.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- AccessorTable.cs
- BasePropertyDescriptor.cs
- CharStorage.cs
- MobileSysDescriptionAttribute.cs
- brushes.cs
- LambdaCompiler.Address.cs
- ConfigDefinitionUpdates.cs
- ServerValidateEventArgs.cs
- DataControlImageButton.cs
- Int16Converter.cs
- LinqDataSourceDisposeEventArgs.cs
- RegisteredHiddenField.cs
- XmlResolver.cs
- followingquery.cs
- PnrpPeerResolver.cs
- AddressHeaderCollection.cs
- DaylightTime.cs
- SecurityElement.cs
- FileFormatException.cs
- ContextBase.cs
- GlobalizationSection.cs
- VisualBasicReference.cs
- XmlSerializableReader.cs
- AudioFileOut.cs
- ApplyImportsAction.cs
- StackSpiller.Generated.cs
- PrintDocument.cs
- FilePrompt.cs
- SqlDeflator.cs
- PassportAuthenticationModule.cs
- MergeFailedEvent.cs
- PatternMatcher.cs
- BooleanAnimationUsingKeyFrames.cs
- ToolStripItemImageRenderEventArgs.cs
- AnonymousIdentificationSection.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- FormViewUpdatedEventArgs.cs
- InputQueueChannelAcceptor.cs
- UnsafeNativeMethodsMilCoreApi.cs
- AccessViolationException.cs
- SchemaType.cs
- DocumentOrderQuery.cs
- ExtentKey.cs
- Classification.cs