Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / DynamicDataManager.cs / 1305376 / DynamicDataManager.cs
namespace System.Web.DynamicData { using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.DataAnnotations; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; using System.Security.Permissions; using System.Web.Resources; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.DynamicData.Util; using System.Data.Objects; #if !ORYX_VNEXT using IDataBoundControlInterface = System.Web.UI.WebControls.IDataBoundControl; #else using IDataBoundControlInterface = System.Web.DynamicData.Util.IDataBoundControl; using LinqDataSource = Microsoft.Web.Data.UI.WebControls.LinqDataSource; using LinqDataSourceContextEventArgs = Microsoft.Web.Data.UI.WebControls.LinqDataSourceContextEventArgs; #endif ////// Adds behavior to certain control to make them work with Dynamic Data /// [NonVisualControl()] [ParseChildren(true)] [PersistChildren(false)] [ToolboxBitmap(typeof(DynamicDataManager), "DynamicDataManager.bmp")] #if !ORYX_VNEXT [Designer("System.Web.DynamicData.Design.DynamicDataManagerDesigner, " + AssemblyRef.SystemWebDynamicDataDesign)] #endif public class DynamicDataManager : Control { private DataControlReferenceCollection _dataControls; // Key is used as the set of registered data source controls. Value is ignored. private Dictionary_dataSources = new Dictionary (); /// /// Causes foreign entities to be loaded as well setting the proper DataLoadOptions. /// Only works with Linq To Sql. /// [ Category("Behavior"), DefaultValue(false), ResourceDescription("DynamicDataManager_AutoLoadForeignKeys") ] public bool AutoLoadForeignKeys { get; set; } #if !ORYX_VNEXT [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override string ClientID { get { return base.ClientID; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override ClientIDMode ClientIDMode { get { return base.ClientIDMode; } set { throw new NotImplementedException(); } } #endif [ Category("Behavior"), DefaultValue(null), PersistenceMode(PersistenceMode.InnerProperty), MergableProperty(false), ] public DataControlReferenceCollection DataControls { get { if (_dataControls == null) { _dataControls = new DataControlReferenceCollection(this); } return _dataControls; } } ////// See base class documentation /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override bool Visible { get { return base.Visible; } set { throw new NotImplementedException(); } } ////// See base class documentation /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected override void OnInit(EventArgs e) { base.OnInit(e); // Initialize the collection DataControls.Initialize(); // Subscribe to the Page's Init to register the controls set in the DataControls collection Page.Init += OnPageInit; } private void OnPageInit(object sender, EventArgs e) { foreach (DataControlReference controlReference in DataControls) { Control targetControl = Misc.FindControl(this, controlReference.ControlID); if (targetControl == null) { throw new InvalidOperationException( String.Format(CultureInfo.CurrentCulture, DynamicDataResources.DynamicDataManager_ControlNotFound, controlReference.ControlID)); } RegisterControl(targetControl); } } ////// See base class documentation /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected override void OnLoad(EventArgs e) { base.OnLoad(e); // Go through all the registered data sources foreach (IDynamicDataSource dataSource in _dataSources.Keys) { // Expand any dynamic where parameters that they may use dataSource.ExpandDynamicWhereParameters(); } } ////// Register a data control to give it Dynamic Data behavior /// /// public void RegisterControl(Control control) { RegisterControl(control, false); } ////// Register a data control to give it Dynamic Data behavior /// /// When true, if a primary key is found in the route values /// (typically on the query string), it will get be set as the selected item. This only applies /// to list controls. public void RegisterControl(Control control, bool setSelectionFromUrl) { // if (DesignMode) { return; } IDataBoundControlInterface dataBoundControl = DataControlHelper.GetDataBoundControl(control, true /*failIfNotFound*/); // If we can't get an associated IDynamicDataSource, don't do anything IDynamicDataSource dataSource = dataBoundControl.DataSourceObject as IDynamicDataSource; if (dataSource == null) { return; } // If we can't get a MetaTable from the data source, don't do anything MetaTable table = MetaTableHelper.GetTableWithFullFallback(dataSource, Context.ToWrapper()); // Save the datasource so we can process its parameters in OnLoad. The value we set is irrelevant _dataSources[dataSource] = null; ((INamingContainer)control).SetMetaTable(table); BaseDataBoundControl baseDataBoundControl = control as BaseDataBoundControl; if (baseDataBoundControl != null) { EnablePersistedSelection(baseDataBoundControl, table); } RegisterControlInternal(dataBoundControl, dataSource, table, setSelectionFromUrl, Page.IsPostBack); } internal static void EnablePersistedSelection(BaseDataBoundControl baseDataBoundControl, IMetaTable table) { Debug.Assert(baseDataBoundControl != null, "NULL!"); // Make the persisted selection [....] up with the selected index if possible if (!table.IsReadOnly) { DynamicDataExtensions.EnablePersistedSelectionInternal(baseDataBoundControl); } } internal void RegisterControlInternal(IDataBoundControlInterface dataBoundControl, IDynamicDataSource dataSource, IMetaTable table, bool setSelectionFromUrl, bool isPostBack) { // Set the auto field generator (for controls that support it - GridView and DetailsView) #if ORYX_VNEXT dataBoundControl.FieldsGenerator = new DefaultAutoFieldGenerator(table); #else IFieldControl fieldControl = dataBoundControl as IFieldControl; if (fieldControl != null) { fieldControl.FieldsGenerator = new DefaultAutoFieldGenerator(table); } #endif var linqDataSource = dataSource as LinqDataSource; var entityDataSource = dataSource as EntityDataSource; // If the context type is not set, we need to set it if (dataSource.ContextType == null) { dataSource.ContextType = table.DataContextType; // If it's a LinqDataSurce, register for ContextCreating so the context gets created using the correct ctor // Ideally, this would work with other datasource, but we just don't have the right abstraction if (linqDataSource != null) { linqDataSource.ContextCreating += delegate(object sender, LinqDataSourceContextEventArgs e) { e.ObjectInstance = table.CreateContext(); }; } if (entityDataSource != null) { entityDataSource.ContextCreating += delegate(object sender, EntityDataSourceContextCreatingEventArgs e) { e.Context = (ObjectContext)table.CreateContext(); }; } } // If the datasource doesn't have an EntitySetName (aka TableName), set it from the meta table if (String.IsNullOrEmpty(dataSource.EntitySetName)) { dataSource.EntitySetName = table.DataContextPropertyName; } // If there is no Where clause, turn on auto generate if (String.IsNullOrEmpty(dataSource.Where)) { dataSource.AutoGenerateWhereClause = true; } // If it's a LinqDataSource and the flag is set, pre load the foreign keys if (AutoLoadForeignKeys && linqDataSource != null) { linqDataSource.LoadWithForeignKeys(table.EntityType); } if (!isPostBack) { if (table.HasPrimaryKey) { dataBoundControl.DataKeyNames = table.PrimaryKeyNames; // Set the virtual selection from the URL if needed var dataKeySelector = dataBoundControl as IPersistedSelector; if (dataKeySelector != null && setSelectionFromUrl) { DataKey dataKey = table.GetDataKeyFromRoute(); if (dataKey != null) { dataKeySelector.DataKey = dataKey; } } #if ORYX_VNEXT dataBoundControl.EnableModelValidation = true; #endif } else { #if ORYX_VNEXT dataBoundControl.EnableModelValidation = false; #endif } } } internal static IControlParameterTarget GetControlParameterTarget(Control control) { #if ORYX_VNEXT if (control is IControlParameterTarget) return (IControlParameterTarget)control; return (IControlParameterTarget)DataControlHelper.GetControlAdapter(control); #else return (control as IControlParameterTarget) ?? new DataBoundControlParameterTarget(control); #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Web.DynamicData { using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.DataAnnotations; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Globalization; using System.Security.Permissions; using System.Web.Resources; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.DynamicData.Util; using System.Data.Objects; #if !ORYX_VNEXT using IDataBoundControlInterface = System.Web.UI.WebControls.IDataBoundControl; #else using IDataBoundControlInterface = System.Web.DynamicData.Util.IDataBoundControl; using LinqDataSource = Microsoft.Web.Data.UI.WebControls.LinqDataSource; using LinqDataSourceContextEventArgs = Microsoft.Web.Data.UI.WebControls.LinqDataSourceContextEventArgs; #endif ////// Adds behavior to certain control to make them work with Dynamic Data /// [NonVisualControl()] [ParseChildren(true)] [PersistChildren(false)] [ToolboxBitmap(typeof(DynamicDataManager), "DynamicDataManager.bmp")] #if !ORYX_VNEXT [Designer("System.Web.DynamicData.Design.DynamicDataManagerDesigner, " + AssemblyRef.SystemWebDynamicDataDesign)] #endif public class DynamicDataManager : Control { private DataControlReferenceCollection _dataControls; // Key is used as the set of registered data source controls. Value is ignored. private Dictionary_dataSources = new Dictionary (); /// /// Causes foreign entities to be loaded as well setting the proper DataLoadOptions. /// Only works with Linq To Sql. /// [ Category("Behavior"), DefaultValue(false), ResourceDescription("DynamicDataManager_AutoLoadForeignKeys") ] public bool AutoLoadForeignKeys { get; set; } #if !ORYX_VNEXT [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override string ClientID { get { return base.ClientID; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override ClientIDMode ClientIDMode { get { return base.ClientIDMode; } set { throw new NotImplementedException(); } } #endif [ Category("Behavior"), DefaultValue(null), PersistenceMode(PersistenceMode.InnerProperty), MergableProperty(false), ] public DataControlReferenceCollection DataControls { get { if (_dataControls == null) { _dataControls = new DataControlReferenceCollection(this); } return _dataControls; } } ////// See base class documentation /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), EditorBrowsable(EditorBrowsableState.Never) ] public override bool Visible { get { return base.Visible; } set { throw new NotImplementedException(); } } ////// See base class documentation /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected override void OnInit(EventArgs e) { base.OnInit(e); // Initialize the collection DataControls.Initialize(); // Subscribe to the Page's Init to register the controls set in the DataControls collection Page.Init += OnPageInit; } private void OnPageInit(object sender, EventArgs e) { foreach (DataControlReference controlReference in DataControls) { Control targetControl = Misc.FindControl(this, controlReference.ControlID); if (targetControl == null) { throw new InvalidOperationException( String.Format(CultureInfo.CurrentCulture, DynamicDataResources.DynamicDataManager_ControlNotFound, controlReference.ControlID)); } RegisterControl(targetControl); } } ////// See base class documentation /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] protected override void OnLoad(EventArgs e) { base.OnLoad(e); // Go through all the registered data sources foreach (IDynamicDataSource dataSource in _dataSources.Keys) { // Expand any dynamic where parameters that they may use dataSource.ExpandDynamicWhereParameters(); } } ////// Register a data control to give it Dynamic Data behavior /// /// public void RegisterControl(Control control) { RegisterControl(control, false); } ////// Register a data control to give it Dynamic Data behavior /// /// When true, if a primary key is found in the route values /// (typically on the query string), it will get be set as the selected item. This only applies /// to list controls. public void RegisterControl(Control control, bool setSelectionFromUrl) { // if (DesignMode) { return; } IDataBoundControlInterface dataBoundControl = DataControlHelper.GetDataBoundControl(control, true /*failIfNotFound*/); // If we can't get an associated IDynamicDataSource, don't do anything IDynamicDataSource dataSource = dataBoundControl.DataSourceObject as IDynamicDataSource; if (dataSource == null) { return; } // If we can't get a MetaTable from the data source, don't do anything MetaTable table = MetaTableHelper.GetTableWithFullFallback(dataSource, Context.ToWrapper()); // Save the datasource so we can process its parameters in OnLoad. The value we set is irrelevant _dataSources[dataSource] = null; ((INamingContainer)control).SetMetaTable(table); BaseDataBoundControl baseDataBoundControl = control as BaseDataBoundControl; if (baseDataBoundControl != null) { EnablePersistedSelection(baseDataBoundControl, table); } RegisterControlInternal(dataBoundControl, dataSource, table, setSelectionFromUrl, Page.IsPostBack); } internal static void EnablePersistedSelection(BaseDataBoundControl baseDataBoundControl, IMetaTable table) { Debug.Assert(baseDataBoundControl != null, "NULL!"); // Make the persisted selection [....] up with the selected index if possible if (!table.IsReadOnly) { DynamicDataExtensions.EnablePersistedSelectionInternal(baseDataBoundControl); } } internal void RegisterControlInternal(IDataBoundControlInterface dataBoundControl, IDynamicDataSource dataSource, IMetaTable table, bool setSelectionFromUrl, bool isPostBack) { // Set the auto field generator (for controls that support it - GridView and DetailsView) #if ORYX_VNEXT dataBoundControl.FieldsGenerator = new DefaultAutoFieldGenerator(table); #else IFieldControl fieldControl = dataBoundControl as IFieldControl; if (fieldControl != null) { fieldControl.FieldsGenerator = new DefaultAutoFieldGenerator(table); } #endif var linqDataSource = dataSource as LinqDataSource; var entityDataSource = dataSource as EntityDataSource; // If the context type is not set, we need to set it if (dataSource.ContextType == null) { dataSource.ContextType = table.DataContextType; // If it's a LinqDataSurce, register for ContextCreating so the context gets created using the correct ctor // Ideally, this would work with other datasource, but we just don't have the right abstraction if (linqDataSource != null) { linqDataSource.ContextCreating += delegate(object sender, LinqDataSourceContextEventArgs e) { e.ObjectInstance = table.CreateContext(); }; } if (entityDataSource != null) { entityDataSource.ContextCreating += delegate(object sender, EntityDataSourceContextCreatingEventArgs e) { e.Context = (ObjectContext)table.CreateContext(); }; } } // If the datasource doesn't have an EntitySetName (aka TableName), set it from the meta table if (String.IsNullOrEmpty(dataSource.EntitySetName)) { dataSource.EntitySetName = table.DataContextPropertyName; } // If there is no Where clause, turn on auto generate if (String.IsNullOrEmpty(dataSource.Where)) { dataSource.AutoGenerateWhereClause = true; } // If it's a LinqDataSource and the flag is set, pre load the foreign keys if (AutoLoadForeignKeys && linqDataSource != null) { linqDataSource.LoadWithForeignKeys(table.EntityType); } if (!isPostBack) { if (table.HasPrimaryKey) { dataBoundControl.DataKeyNames = table.PrimaryKeyNames; // Set the virtual selection from the URL if needed var dataKeySelector = dataBoundControl as IPersistedSelector; if (dataKeySelector != null && setSelectionFromUrl) { DataKey dataKey = table.GetDataKeyFromRoute(); if (dataKey != null) { dataKeySelector.DataKey = dataKey; } } #if ORYX_VNEXT dataBoundControl.EnableModelValidation = true; #endif } else { #if ORYX_VNEXT dataBoundControl.EnableModelValidation = false; #endif } } } internal static IControlParameterTarget GetControlParameterTarget(Control control) { #if ORYX_VNEXT if (control is IControlParameterTarget) return (IControlParameterTarget)control; return (IControlParameterTarget)DataControlHelper.GetControlAdapter(control); #else return (control as IControlParameterTarget) ?? new DataBoundControlParameterTarget(control); #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
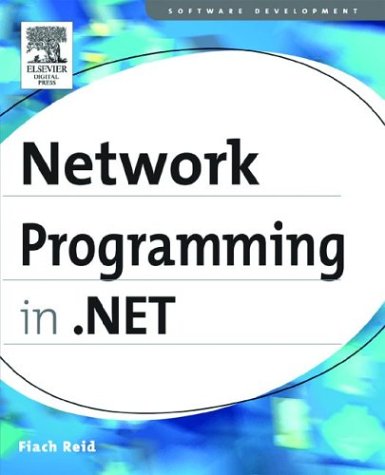
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransactionBridgeSection.cs
- SqlStatistics.cs
- PolyLineSegment.cs
- ForceCopyBuildProvider.cs
- FaultConverter.cs
- PopupEventArgs.cs
- FixedSOMTableRow.cs
- PersonalizationStateInfo.cs
- ExpressionPrefixAttribute.cs
- EmbeddedMailObject.cs
- CodeDomSerializer.cs
- SchemaAttDef.cs
- WindowsSlider.cs
- QueryResults.cs
- OleDbEnumerator.cs
- LocalizabilityAttribute.cs
- WebBrowserDocumentCompletedEventHandler.cs
- ToolZone.cs
- InstanceKeyView.cs
- ProtectedProviderSettings.cs
- InvalidateEvent.cs
- DataGridViewIntLinkedList.cs
- UrlMapping.cs
- HashCodeCombiner.cs
- smtpconnection.cs
- ZipPackagePart.cs
- XmlLangPropertyAttribute.cs
- DBPropSet.cs
- QualifierSet.cs
- HeaderElement.cs
- SmuggledIUnknown.cs
- WebPartCloseVerb.cs
- StringToken.cs
- DetectEofStream.cs
- ParserContext.cs
- BrowserCapabilitiesFactoryBase.cs
- TextBounds.cs
- XMLUtil.cs
- ExcludeFromCodeCoverageAttribute.cs
- OleDbStruct.cs
- ValueOfAction.cs
- SerializationException.cs
- ThreadExceptionDialog.cs
- DeploymentSection.cs
- EntityDataSourceContainerNameConverter.cs
- UnsafeNativeMethodsMilCoreApi.cs
- MediaTimeline.cs
- ExceptionUtil.cs
- BaseServiceProvider.cs
- WindowAutomationPeer.cs
- BitmapEffectGeneralTransform.cs
- ListViewEditEventArgs.cs
- TextSegment.cs
- ColorPalette.cs
- XmlILAnnotation.cs
- SiteIdentityPermission.cs
- DataSourceCacheDurationConverter.cs
- MultiplexingFormatMapping.cs
- URLAttribute.cs
- FixedTextBuilder.cs
- BlurBitmapEffect.cs
- SchemaElement.cs
- BuildManagerHost.cs
- CodePageUtils.cs
- TypeTypeConverter.cs
- SQLCharsStorage.cs
- PageCatalogPart.cs
- FormsAuthenticationTicket.cs
- LoginView.cs
- LabelEditEvent.cs
- XXXInfos.cs
- List.cs
- Schema.cs
- MergeEnumerator.cs
- StorageMappingItemLoader.cs
- SafeRegistryHandle.cs
- GlyphingCache.cs
- DataStorage.cs
- UInt16.cs
- HtmlFormParameterReader.cs
- MessageBox.cs
- XsdDateTime.cs
- SafeFileMapViewHandle.cs
- WebPartEditorApplyVerb.cs
- NonBatchDirectoryCompiler.cs
- DecimalMinMaxAggregationOperator.cs
- TypeElement.cs
- WebPartManager.cs
- SmiGettersStream.cs
- ISAPIApplicationHost.cs
- DynamicDocumentPaginator.cs
- WindowsListViewGroupSubsetLink.cs
- CacheVirtualItemsEvent.cs
- ISCIIEncoding.cs
- DiscoveryDocument.cs
- Interfaces.cs
- ConfigurationStrings.cs
- DelegatedStream.cs
- FlowSwitchDesigner.xaml.cs
- WindowsEditBoxRange.cs