Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / XmlUtil.cs / 1305376 / XmlUtil.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static xml utility functions // //--------------------------------------------------------------------- #if ASTORIA_CLIENT namespace System.Data.Services.Client #else namespace System.Data.Services #endif { using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Xml; ////// static uri utility functions /// internal static partial class UriUtil { #if !ASTORIA_CLIENT ///Creates a URI suitable for host-agnostic comparison purposes. /// URI to compare. ///URI suitable for comparison. private static Uri CreateBaseComparableUri(Uri uri) { Debug.Assert(uri != null, "uri != null"); uri = new Uri(uri.OriginalString.ToUpper(CultureInfo.InvariantCulture), UriKind.RelativeOrAbsolute); UriBuilder builder = new UriBuilder(uri); builder.Host = "h"; builder.Port = 80; builder.Scheme = "http"; return builder.Uri; } #endif ///Parse the atom link relation attribute value /// atom link relation attribute value ///the assocation name or null internal static string GetNameFromAtomLinkRelationAttribute(string value) { string name = null; if (!String.IsNullOrEmpty(value)) { Uri uri = null; try { uri = new Uri(value, UriKind.RelativeOrAbsolute); } catch (UriFormatException) { // ignore the exception, obviously we don't have our expected relation value } if ((null != uri) && uri.IsAbsoluteUri) { string unescaped = uri.GetComponents(UriComponents.AbsoluteUri, UriFormat.SafeUnescaped); if (unescaped.StartsWith(XmlConstants.DataWebRelatedNamespace, StringComparison.Ordinal)) { name = unescaped.Substring(XmlConstants.DataWebRelatedNamespace.Length); } } } return name; } #if !ASTORIA_CLIENT ///is the serviceRoot the base of the request uri /// baseUriWithSlash /// requestUri ///true if the serviceRoot is the base of the request uri internal static bool IsBaseOf(Uri baseUriWithSlash, Uri requestUri) { return baseUriWithSlash.IsBaseOf(requestUri); } ////// Determines whether the /// Candidate base URI. /// The specified Uri instance to test. ///Uri instance is a /// base of the specified Uri instance. /// true if the current Uri instance is a base of uri; otherwise, false. internal static bool UriInvariantInsensitiveIsBaseOf(Uri current, Uri uri) { Debug.Assert(current != null, "current != null"); Debug.Assert(uri != null, "uri != null"); Uri upperCurrent = CreateBaseComparableUri(current); Uri upperUri = CreateBaseComparableUri(uri); return UriUtil.IsBaseOf(upperCurrent, upperUri); } #endif } ////// static xml utility function /// internal static partial class XmlUtil { ////// An initial nametable so that string comparisons during /// deserialization become reference comparisions /// ///nametable with element names used in application/atom+xml payload private static NameTable CreateAtomNameTable() { NameTable table = new NameTable(); table.Add(XmlConstants.AtomNamespace); table.Add(XmlConstants.DataWebNamespace); table.Add(XmlConstants.DataWebMetadataNamespace); table.Add(XmlConstants.AtomContentElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomContentSrcAttributeName); #endif table.Add(XmlConstants.AtomEntryElementName); table.Add(XmlConstants.AtomETagAttributeName); table.Add(XmlConstants.AtomFeedElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomIdElementName); #endif table.Add(XmlConstants.AtomInlineElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomLinkElementName); table.Add(XmlConstants.AtomLinkRelationAttributeName); #endif table.Add(XmlConstants.AtomNullAttributeName); table.Add(XmlConstants.AtomPropertiesElementName); table.Add(XmlConstants.AtomTitleElementName); table.Add(XmlConstants.AtomTypeAttributeName); table.Add(XmlConstants.XmlErrorCodeElementName); table.Add(XmlConstants.XmlErrorElementName); table.Add(XmlConstants.XmlErrorInnerElementName); table.Add(XmlConstants.XmlErrorMessageElementName); table.Add(XmlConstants.XmlErrorTypeElementName); return table; } ////// Creates a new XmlReader instance using the specified stream reader /// /// The stream reader from which you want to read /// The encoding of the stream ///XmlReader with the appropriate xml settings [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2000:Dispose objects before losing scope", Justification = "Caller is responsible for disposing the XmlReader")] internal static XmlReader CreateXmlReader(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null stream"); XmlReaderSettings settings = new XmlReaderSettings(); settings.CheckCharacters = false; #if ASTORIA_CLIENT // we should close the underlying stream only for the client. // In server, we should never close the underlying stream - neither after reading nor after writing, // since the underlying host owns the stream. settings.CloseInput = true; #endif settings.IgnoreWhitespace = true; settings.NameTable = XmlUtil.CreateAtomNameTable(); if (null == encoding) { // auto-detect the encoding return XmlReader.Create(stream, settings); } return XmlReader.Create(new StreamReader(stream, encoding), settings); } ////// Creates a new XmlWriterSettings instance using the encoding. /// /// Encoding that you want to specify in the reader settings as well as the processing instruction internal static XmlWriterSettings CreateXmlWriterSettings(Encoding encoding) { Debug.Assert(null != encoding, "null != encoding"); // No need to close the underlying stream here for client, // since it always MemoryStream for writing i.e. it caches the response before processing. XmlWriterSettings settings = new XmlWriterSettings(); settings.CheckCharacters = false; settings.ConformanceLevel = ConformanceLevel.Fragment; settings.Encoding = encoding; settings.Indent = true; settings.NewLineHandling = NewLineHandling.Entitize; Debug.Assert(!settings.CloseOutput, "!settings.CloseOutput -- otherwise default changed?"); return settings; } ////// Creates a new XmlWriter instance using the specified stream and writers the processing instruction /// with the given encoding value /// /// The stream to which you want to write /// Encoding that you want to specify in the reader settings as well as the processing instruction ///XmlWriter with the appropriate xml settings and processing instruction internal static XmlWriter CreateXmlWriterAndWriteProcessingInstruction(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null != stream"); Debug.Assert(null != encoding, "null != encoding"); XmlWriterSettings settings = CreateXmlWriterSettings(encoding); XmlWriter writer = XmlWriter.Create(stream, settings); writer.WriteProcessingInstruction("xml", "version=\"1.0\" encoding=\"" + encoding.WebName + "\" standalone=\"yes\""); return writer; } #if ASTORIA_CLIENT ////// get attribute value from specified namespace or empty namespace /// /// reader /// attributeName /// namespaceUri ///attribute value internal static string GetAttributeEx(this XmlReader reader, string attributeName, string namespaceUri) { return reader.GetAttribute(attributeName, namespaceUri) ?? reader.GetAttribute(attributeName); } ///Recursively removes duplicate namespace attributes from the specified /// Element to remove duplicate attributes from. internal static void RemoveDuplicateNamespaceAttributes(System.Xml.Linq.XElement element) { Debug.Assert(element != null, "element != null"); // Nesting XElements through our client wrappers may produce duplicate namespaces. // Might need an XML fix, but won't happen for 3.5 for back compat. HashSet. names = new HashSet (EqualityComparer .Default); foreach (System.Xml.Linq.XElement e in element.DescendantsAndSelf()) { bool attributesFound = false; foreach (var attribute in e.Attributes()) { if (!attributesFound) { attributesFound = true; names.Clear(); } if (attribute.IsNamespaceDeclaration) { string localName = attribute.Name.LocalName; bool alreadyPresent = names.Add(localName) == false; if (alreadyPresent) { attribute.Remove(); } } } } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// static xml utility functions // //--------------------------------------------------------------------- #if ASTORIA_CLIENT namespace System.Data.Services.Client #else namespace System.Data.Services #endif { using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Xml; ////// static uri utility functions /// internal static partial class UriUtil { #if !ASTORIA_CLIENT ///Creates a URI suitable for host-agnostic comparison purposes. /// URI to compare. ///URI suitable for comparison. private static Uri CreateBaseComparableUri(Uri uri) { Debug.Assert(uri != null, "uri != null"); uri = new Uri(uri.OriginalString.ToUpper(CultureInfo.InvariantCulture), UriKind.RelativeOrAbsolute); UriBuilder builder = new UriBuilder(uri); builder.Host = "h"; builder.Port = 80; builder.Scheme = "http"; return builder.Uri; } #endif ///Parse the atom link relation attribute value /// atom link relation attribute value ///the assocation name or null internal static string GetNameFromAtomLinkRelationAttribute(string value) { string name = null; if (!String.IsNullOrEmpty(value)) { Uri uri = null; try { uri = new Uri(value, UriKind.RelativeOrAbsolute); } catch (UriFormatException) { // ignore the exception, obviously we don't have our expected relation value } if ((null != uri) && uri.IsAbsoluteUri) { string unescaped = uri.GetComponents(UriComponents.AbsoluteUri, UriFormat.SafeUnescaped); if (unescaped.StartsWith(XmlConstants.DataWebRelatedNamespace, StringComparison.Ordinal)) { name = unescaped.Substring(XmlConstants.DataWebRelatedNamespace.Length); } } } return name; } #if !ASTORIA_CLIENT ///is the serviceRoot the base of the request uri /// baseUriWithSlash /// requestUri ///true if the serviceRoot is the base of the request uri internal static bool IsBaseOf(Uri baseUriWithSlash, Uri requestUri) { return baseUriWithSlash.IsBaseOf(requestUri); } ////// Determines whether the /// Candidate base URI. /// The specified Uri instance to test. ///Uri instance is a /// base of the specified Uri instance. /// true if the current Uri instance is a base of uri; otherwise, false. internal static bool UriInvariantInsensitiveIsBaseOf(Uri current, Uri uri) { Debug.Assert(current != null, "current != null"); Debug.Assert(uri != null, "uri != null"); Uri upperCurrent = CreateBaseComparableUri(current); Uri upperUri = CreateBaseComparableUri(uri); return UriUtil.IsBaseOf(upperCurrent, upperUri); } #endif } ////// static xml utility function /// internal static partial class XmlUtil { ////// An initial nametable so that string comparisons during /// deserialization become reference comparisions /// ///nametable with element names used in application/atom+xml payload private static NameTable CreateAtomNameTable() { NameTable table = new NameTable(); table.Add(XmlConstants.AtomNamespace); table.Add(XmlConstants.DataWebNamespace); table.Add(XmlConstants.DataWebMetadataNamespace); table.Add(XmlConstants.AtomContentElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomContentSrcAttributeName); #endif table.Add(XmlConstants.AtomEntryElementName); table.Add(XmlConstants.AtomETagAttributeName); table.Add(XmlConstants.AtomFeedElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomIdElementName); #endif table.Add(XmlConstants.AtomInlineElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomLinkElementName); table.Add(XmlConstants.AtomLinkRelationAttributeName); #endif table.Add(XmlConstants.AtomNullAttributeName); table.Add(XmlConstants.AtomPropertiesElementName); table.Add(XmlConstants.AtomTitleElementName); table.Add(XmlConstants.AtomTypeAttributeName); table.Add(XmlConstants.XmlErrorCodeElementName); table.Add(XmlConstants.XmlErrorElementName); table.Add(XmlConstants.XmlErrorInnerElementName); table.Add(XmlConstants.XmlErrorMessageElementName); table.Add(XmlConstants.XmlErrorTypeElementName); return table; } ////// Creates a new XmlReader instance using the specified stream reader /// /// The stream reader from which you want to read /// The encoding of the stream ///XmlReader with the appropriate xml settings [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2000:Dispose objects before losing scope", Justification = "Caller is responsible for disposing the XmlReader")] internal static XmlReader CreateXmlReader(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null stream"); XmlReaderSettings settings = new XmlReaderSettings(); settings.CheckCharacters = false; #if ASTORIA_CLIENT // we should close the underlying stream only for the client. // In server, we should never close the underlying stream - neither after reading nor after writing, // since the underlying host owns the stream. settings.CloseInput = true; #endif settings.IgnoreWhitespace = true; settings.NameTable = XmlUtil.CreateAtomNameTable(); if (null == encoding) { // auto-detect the encoding return XmlReader.Create(stream, settings); } return XmlReader.Create(new StreamReader(stream, encoding), settings); } ////// Creates a new XmlWriterSettings instance using the encoding. /// /// Encoding that you want to specify in the reader settings as well as the processing instruction internal static XmlWriterSettings CreateXmlWriterSettings(Encoding encoding) { Debug.Assert(null != encoding, "null != encoding"); // No need to close the underlying stream here for client, // since it always MemoryStream for writing i.e. it caches the response before processing. XmlWriterSettings settings = new XmlWriterSettings(); settings.CheckCharacters = false; settings.ConformanceLevel = ConformanceLevel.Fragment; settings.Encoding = encoding; settings.Indent = true; settings.NewLineHandling = NewLineHandling.Entitize; Debug.Assert(!settings.CloseOutput, "!settings.CloseOutput -- otherwise default changed?"); return settings; } ////// Creates a new XmlWriter instance using the specified stream and writers the processing instruction /// with the given encoding value /// /// The stream to which you want to write /// Encoding that you want to specify in the reader settings as well as the processing instruction ///XmlWriter with the appropriate xml settings and processing instruction internal static XmlWriter CreateXmlWriterAndWriteProcessingInstruction(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null != stream"); Debug.Assert(null != encoding, "null != encoding"); XmlWriterSettings settings = CreateXmlWriterSettings(encoding); XmlWriter writer = XmlWriter.Create(stream, settings); writer.WriteProcessingInstruction("xml", "version=\"1.0\" encoding=\"" + encoding.WebName + "\" standalone=\"yes\""); return writer; } #if ASTORIA_CLIENT ////// get attribute value from specified namespace or empty namespace /// /// reader /// attributeName /// namespaceUri ///attribute value internal static string GetAttributeEx(this XmlReader reader, string attributeName, string namespaceUri) { return reader.GetAttribute(attributeName, namespaceUri) ?? reader.GetAttribute(attributeName); } ///Recursively removes duplicate namespace attributes from the specified /// Element to remove duplicate attributes from. internal static void RemoveDuplicateNamespaceAttributes(System.Xml.Linq.XElement element) { Debug.Assert(element != null, "element != null"); // Nesting XElements through our client wrappers may produce duplicate namespaces. // Might need an XML fix, but won't happen for 3.5 for back compat. HashSet. names = new HashSet (EqualityComparer .Default); foreach (System.Xml.Linq.XElement e in element.DescendantsAndSelf()) { bool attributesFound = false; foreach (var attribute in e.Attributes()) { if (!attributesFound) { attributesFound = true; names.Clear(); } if (attribute.IsNamespaceDeclaration) { string localName = attribute.Name.LocalName; bool alreadyPresent = names.Add(localName) == false; if (alreadyPresent) { attribute.Remove(); } } } } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
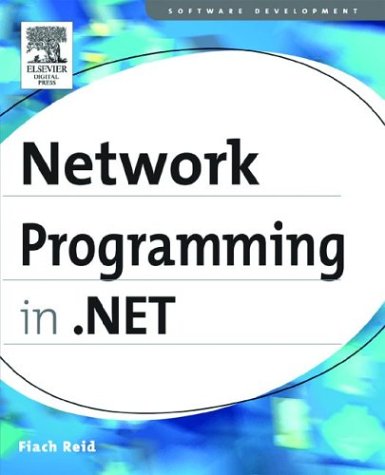
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpContextServiceHost.cs
- WorkItem.cs
- remotingproxy.cs
- WebPartConnectionsEventArgs.cs
- EventDescriptor.cs
- XmlKeywords.cs
- UserPreferenceChangedEventArgs.cs
- arclist.cs
- OperationInfoBase.cs
- Restrictions.cs
- XmlElement.cs
- RequestNavigateEventArgs.cs
- DataServiceClientException.cs
- WebConfigurationHostFileChange.cs
- ReadOnlyMetadataCollection.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- QilLoop.cs
- DataGridViewComboBoxEditingControl.cs
- RecognitionResult.cs
- ButtonColumn.cs
- PathNode.cs
- SectionInformation.cs
- BrowserDefinition.cs
- DecoderFallbackWithFailureFlag.cs
- OdbcStatementHandle.cs
- MultipleViewPattern.cs
- EventLogConfiguration.cs
- LayoutEvent.cs
- UrlPath.cs
- TextBoxBase.cs
- DelegateBodyWriter.cs
- ContractValidationHelper.cs
- SimpleHandlerBuildProvider.cs
- PerformanceCounter.cs
- WebPartCollection.cs
- TCPClient.cs
- SystemColors.cs
- DelegatingTypeDescriptionProvider.cs
- CompoundFileStorageReference.cs
- PingReply.cs
- ComponentResourceKey.cs
- XPathItem.cs
- LongCountAggregationOperator.cs
- RegionInfo.cs
- ConfigurationSettings.cs
- WindowsListViewItemCheckBox.cs
- PrintDialog.cs
- RoleExceptions.cs
- ItemCollectionEditor.cs
- SharedPersonalizationStateInfo.cs
- StylusPointProperties.cs
- HierarchicalDataSourceConverter.cs
- SafeNativeMethods.cs
- Property.cs
- ProxyWebPart.cs
- FieldAccessException.cs
- Variable.cs
- KeyedCollection.cs
- WebPartChrome.cs
- UIntPtr.cs
- CreateUserWizard.cs
- Utils.cs
- WebException.cs
- CompilerResults.cs
- WizardForm.cs
- RegisteredScript.cs
- VerificationException.cs
- SecurityRuntime.cs
- LifetimeServices.cs
- AppDomainUnloadedException.cs
- ParagraphResult.cs
- DurableInstanceManager.cs
- TypeDescriptionProvider.cs
- CustomValidator.cs
- QilName.cs
- ChameleonKey.cs
- MediaContextNotificationWindow.cs
- LoadWorkflowByKeyAsyncResult.cs
- ScrollEvent.cs
- DataGridViewComboBoxColumn.cs
- ListenerUnsafeNativeMethods.cs
- RandomDelaySendsAsyncResult.cs
- EndpointBehaviorElement.cs
- HealthMonitoringSection.cs
- HtmlInputFile.cs
- EntityContainerEmitter.cs
- ToolboxComponentsCreatingEventArgs.cs
- ParserExtension.cs
- UIElementPropertyUndoUnit.cs
- HttpModuleAction.cs
- UniqueSet.cs
- DataKey.cs
- StrokeNodeOperations2.cs
- WsdlInspector.cs
- XPathBinder.cs
- UICuesEvent.cs
- ProxyBuilder.cs
- ThicknessAnimation.cs
- FilterableAttribute.cs
- PathSegment.cs