Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Objects / ObjectStateEntryBaseUpdatableDataRecord.cs / 1305376 / ObjectStateEntryBaseUpdatableDataRecord.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Reflection; namespace System.Data.Objects { ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbUpdatableDataRecord : DbDataRecord, IExtendedDataRecord { internal readonly StateManagerTypeMetadata _metadata; internal readonly ObjectStateEntry _cacheEntry; internal readonly object _userObject; internal DataRecordInfo _recordInfo; internal DbUpdatableDataRecord(ObjectStateEntry cacheEntry, StateManagerTypeMetadata metadata, object userObject) { _cacheEntry = cacheEntry; _userObject = userObject; _metadata = metadata; } internal DbUpdatableDataRecord(ObjectStateEntry cacheEntry) : this(cacheEntry, null, null) { } ////// Returns the number of fields in the record. /// override public int FieldCount { get { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); return _cacheEntry.GetFieldCount(_metadata); } } ////// Retrieves a value with the given field ordinal /// /// The ordinal of the field ///The field value override public object this[int ordinal] { get { return GetValue(ordinal); } } ////// Retrieves a value with the given field name /// /// The name of the field ///The field value override public object this[string name] { get { return GetValue(GetOrdinal(name)); } } ////// Retrieves the field value as a boolean /// /// The ordinal of the field ///The field value as a boolean override public bool GetBoolean(int ordinal) { return (bool)GetValue(ordinal); } ////// Retrieves the field value as a byte /// /// The ordinal of the field ///The field value as a byte override public byte GetByte(int ordinal) { return (byte)GetValue(ordinal); } ////// Retrieves the field value as a byte array /// /// The ordinal of the field /// The index at which to start copying data /// The destination buffer where data is copied to /// The index in the destination buffer where copying will begin /// The number of bytes to copy ///The number of bytes copied override public long GetBytes(int ordinal, long dataIndex, byte[] buffer, int bufferIndex, int length) { byte[] tempBuffer; tempBuffer = (byte[])GetValue(ordinal); if (buffer == null) { return tempBuffer.Length; } int srcIndex = (int)dataIndex; int byteCount = Math.Min(tempBuffer.Length - srcIndex, length); if (srcIndex < 0) { throw EntityUtil.InvalidSourceBufferIndex(tempBuffer.Length, srcIndex, "dataIndex"); } else if ((bufferIndex < 0) || (bufferIndex > 0 && bufferIndex >= buffer.Length)) { throw EntityUtil.InvalidDestinationBufferIndex(buffer.Length, bufferIndex, "bufferIndex"); } if (0 < byteCount) { Array.Copy(tempBuffer, dataIndex, buffer, bufferIndex, byteCount); } else if (length < 0) { throw EntityUtil.InvalidDataLength(length); } else { byteCount = 0; } return byteCount; } ////// Retrieves the field value as a char /// /// The ordinal of the field ///The field value as a char override public char GetChar(int ordinal) { return (char)GetValue(ordinal); } ////// Retrieves the field value as a char array /// /// The ordinal of the field /// The index at which to start copying data /// The destination buffer where data is copied to /// The index in the destination buffer where copying will begin /// The number of chars to copy ///The number of chars copied override public long GetChars(int ordinal, long dataIndex, char[] buffer, int bufferIndex, int length) { char[] tempBuffer; tempBuffer = (char[])GetValue(ordinal); if (buffer == null) { return tempBuffer.Length; } int srcIndex = (int)dataIndex; int charCount = Math.Min(tempBuffer.Length - srcIndex, length); if (srcIndex < 0) { throw EntityUtil.InvalidSourceBufferIndex(tempBuffer.Length, srcIndex, "dataIndex"); } else if ((bufferIndex < 0) || (bufferIndex > 0 && bufferIndex >= buffer.Length)) { throw EntityUtil.InvalidDestinationBufferIndex(buffer.Length, bufferIndex, "bufferIndex"); } if (0 < charCount) { Array.Copy(tempBuffer, dataIndex, buffer, bufferIndex, charCount); } else if (length < 0) { throw EntityUtil.InvalidDataLength(length); } else { charCount = 0; } return charCount; } IDataReader IDataRecord.GetData(int ordinal) { return GetDbDataReader(ordinal); } ////// Retrieves the field value as a DbDataReader /// /// The ordinal of the field ///override protected DbDataReader GetDbDataReader(int ordinal) { throw EntityUtil.NotSupported(); } /// /// Retrieves the name of the field data type /// /// The ordinal of the field ///The name of the field data type override public string GetDataTypeName(int ordinal) { return ((Type)GetFieldType(ordinal)).Name; } ////// Retrieves the field value as a DateTime /// /// The ordinal of the field ///The field value as a DateTime override public DateTime GetDateTime(int ordinal) { return (DateTime)GetValue(ordinal); } ////// Retrieves the field value as a decimal /// /// The ordinal of the field ///The field value as a decimal override public Decimal GetDecimal(int ordinal) { return (Decimal)GetValue(ordinal); } ////// Retrieves the field value as a double /// /// The ordinal of the field ///The field value as a double override public double GetDouble(int ordinal) { return (double)GetValue(ordinal); } ////// Retrieves the type of a field /// /// The ordinal of the field ///The field type public override Type GetFieldType(int ordinal) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); return _cacheEntry.GetFieldType(ordinal, _metadata); } ////// Retrieves the field value as a float /// /// The ordinal of the field ///The field value as a float override public float GetFloat(int ordinal) { return (float)GetValue(ordinal); } ////// Retrieves the field value as a Guid /// /// The ordinal of the field ///The field value as a Guid override public Guid GetGuid(int ordinal) { return (Guid)GetValue(ordinal); } ////// Retrieves the field value as an Int16 /// /// The ordinal of the field ///The field value as an Int16 override public Int16 GetInt16(int ordinal) { return (Int16)GetValue(ordinal); } ////// Retrieves the field value as an Int32 /// /// The ordinal of the field ///The field value as an Int32 override public Int32 GetInt32(int ordinal) { return (Int32)GetValue(ordinal); } ////// Retrieves the field value as an Int64 /// /// The ordinal of the field ///The field value as an Int64 override public Int64 GetInt64(int ordinal) { return (Int64)GetValue(ordinal); } ////// Retrieves the name of a field /// /// The ordinal of the field ///The name of the field override public string GetName(int ordinal) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); return _cacheEntry.GetCLayerName(ordinal, _metadata); } ////// Retrieves the ordinal of a field by name /// /// The name of the field ///The ordinal of the field override public int GetOrdinal(string name) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); int ordinal = _cacheEntry.GetOrdinalforCLayerName(name, _metadata); if (ordinal == -1) { throw EntityUtil.ArgumentOutOfRange("name"); } return ordinal; } ////// Retrieves the field value as a string /// /// The ordinal of the field ///The field value as a string override public string GetString(int ordinal) { return (string)GetValue(ordinal); } ////// Retrieves the value of a field /// /// The ordinal of the field ///The field value override public object GetValue(int ordinal) { return GetRecordValue(ordinal); } ////// In derived classes, retrieves the record value for a field /// /// The ordinal of the field ///The field value protected abstract object GetRecordValue(int ordinal); ////// Retrieves all field values in the record into an object array /// /// An array of objects to store the field values ///The number of field values returned override public int GetValues(object[] values) { if (values == null) { throw EntityUtil.ArgumentNull("values"); } int minValue = Math.Min(values.Length, FieldCount); for (int i = 0; i < minValue; i++) { values[i] = GetValue(i); } return minValue; } ////// Determines if a field has a DBNull value /// /// The ordinal of the field ///True if the field has a DBNull value override public bool IsDBNull(int ordinal) { return (GetValue(ordinal) == DBNull.Value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// public void SetBoolean(int ordinal, bool value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// public void SetByte(int ordinal, byte value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetChar(int ordinal, char value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDataRecord(int ordinal, IDataRecord value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDateTime(int ordinal, DateTime value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDecimal(int ordinal, Decimal value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDouble(int ordinal, Double value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetFloat(int ordinal, float value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetGuid(int ordinal, Guid value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetInt16(int ordinal, Int16 value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetInt32(int ordinal, Int32 value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetInt64(int ordinal, Int64 value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetString(int ordinal, string value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetValue(int ordinal, object value) { SetRecordValue(ordinal, value); } ////// Sets field values in a record /// /// ///The number of fields that were set public int SetValues(params Object[] values) { int minValue = Math.Min(values.Length, FieldCount); for (int i = 0; i < minValue; i++) { SetRecordValue(i, values[i]); } return minValue; } ////// Sets a field to the DBNull value /// /// The ordinal of the field public void SetDBNull(int ordinal) { SetRecordValue(ordinal, DBNull.Value); } ////// Retrieve data record information /// public virtual DataRecordInfo DataRecordInfo { get { if (null == _recordInfo) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); _recordInfo = _cacheEntry.GetDataRecordInfo(_metadata, _userObject); } return _recordInfo; } } ////// Retrieves a field value as a DbDataRecord /// /// The ordinal of the field ///The field value as a DbDataRecord public DbDataRecord GetDataRecord(int ordinal) { return (DbDataRecord)GetValue(ordinal); } ////// Used to return a nested result /// /// ///public DbDataReader GetDataReader(int i) { return this.GetDbDataReader(i); } /// /// Sets the field value for a given ordinal /// /// in the cspace mapping /// in CSpace protected abstract void SetRecordValue(int ordinal, object value); } public abstract class CurrentValueRecord : DbUpdatableDataRecord { internal CurrentValueRecord(ObjectStateEntry cacheEntry, StateManagerTypeMetadata metadata, object userObject) : base(cacheEntry, metadata, userObject) { } internal CurrentValueRecord(ObjectStateEntry cacheEntry) : base(cacheEntry) { } } public abstract class OriginalValueRecord : DbUpdatableDataRecord { internal OriginalValueRecord(ObjectStateEntry cacheEntry, StateManagerTypeMetadata metadata, object userObject) : base(cacheEntry, metadata, userObject) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Reflection; namespace System.Data.Objects { ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbUpdatableDataRecord : DbDataRecord, IExtendedDataRecord { internal readonly StateManagerTypeMetadata _metadata; internal readonly ObjectStateEntry _cacheEntry; internal readonly object _userObject; internal DataRecordInfo _recordInfo; internal DbUpdatableDataRecord(ObjectStateEntry cacheEntry, StateManagerTypeMetadata metadata, object userObject) { _cacheEntry = cacheEntry; _userObject = userObject; _metadata = metadata; } internal DbUpdatableDataRecord(ObjectStateEntry cacheEntry) : this(cacheEntry, null, null) { } ////// Returns the number of fields in the record. /// override public int FieldCount { get { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); return _cacheEntry.GetFieldCount(_metadata); } } ////// Retrieves a value with the given field ordinal /// /// The ordinal of the field ///The field value override public object this[int ordinal] { get { return GetValue(ordinal); } } ////// Retrieves a value with the given field name /// /// The name of the field ///The field value override public object this[string name] { get { return GetValue(GetOrdinal(name)); } } ////// Retrieves the field value as a boolean /// /// The ordinal of the field ///The field value as a boolean override public bool GetBoolean(int ordinal) { return (bool)GetValue(ordinal); } ////// Retrieves the field value as a byte /// /// The ordinal of the field ///The field value as a byte override public byte GetByte(int ordinal) { return (byte)GetValue(ordinal); } ////// Retrieves the field value as a byte array /// /// The ordinal of the field /// The index at which to start copying data /// The destination buffer where data is copied to /// The index in the destination buffer where copying will begin /// The number of bytes to copy ///The number of bytes copied override public long GetBytes(int ordinal, long dataIndex, byte[] buffer, int bufferIndex, int length) { byte[] tempBuffer; tempBuffer = (byte[])GetValue(ordinal); if (buffer == null) { return tempBuffer.Length; } int srcIndex = (int)dataIndex; int byteCount = Math.Min(tempBuffer.Length - srcIndex, length); if (srcIndex < 0) { throw EntityUtil.InvalidSourceBufferIndex(tempBuffer.Length, srcIndex, "dataIndex"); } else if ((bufferIndex < 0) || (bufferIndex > 0 && bufferIndex >= buffer.Length)) { throw EntityUtil.InvalidDestinationBufferIndex(buffer.Length, bufferIndex, "bufferIndex"); } if (0 < byteCount) { Array.Copy(tempBuffer, dataIndex, buffer, bufferIndex, byteCount); } else if (length < 0) { throw EntityUtil.InvalidDataLength(length); } else { byteCount = 0; } return byteCount; } ////// Retrieves the field value as a char /// /// The ordinal of the field ///The field value as a char override public char GetChar(int ordinal) { return (char)GetValue(ordinal); } ////// Retrieves the field value as a char array /// /// The ordinal of the field /// The index at which to start copying data /// The destination buffer where data is copied to /// The index in the destination buffer where copying will begin /// The number of chars to copy ///The number of chars copied override public long GetChars(int ordinal, long dataIndex, char[] buffer, int bufferIndex, int length) { char[] tempBuffer; tempBuffer = (char[])GetValue(ordinal); if (buffer == null) { return tempBuffer.Length; } int srcIndex = (int)dataIndex; int charCount = Math.Min(tempBuffer.Length - srcIndex, length); if (srcIndex < 0) { throw EntityUtil.InvalidSourceBufferIndex(tempBuffer.Length, srcIndex, "dataIndex"); } else if ((bufferIndex < 0) || (bufferIndex > 0 && bufferIndex >= buffer.Length)) { throw EntityUtil.InvalidDestinationBufferIndex(buffer.Length, bufferIndex, "bufferIndex"); } if (0 < charCount) { Array.Copy(tempBuffer, dataIndex, buffer, bufferIndex, charCount); } else if (length < 0) { throw EntityUtil.InvalidDataLength(length); } else { charCount = 0; } return charCount; } IDataReader IDataRecord.GetData(int ordinal) { return GetDbDataReader(ordinal); } ////// Retrieves the field value as a DbDataReader /// /// The ordinal of the field ///override protected DbDataReader GetDbDataReader(int ordinal) { throw EntityUtil.NotSupported(); } /// /// Retrieves the name of the field data type /// /// The ordinal of the field ///The name of the field data type override public string GetDataTypeName(int ordinal) { return ((Type)GetFieldType(ordinal)).Name; } ////// Retrieves the field value as a DateTime /// /// The ordinal of the field ///The field value as a DateTime override public DateTime GetDateTime(int ordinal) { return (DateTime)GetValue(ordinal); } ////// Retrieves the field value as a decimal /// /// The ordinal of the field ///The field value as a decimal override public Decimal GetDecimal(int ordinal) { return (Decimal)GetValue(ordinal); } ////// Retrieves the field value as a double /// /// The ordinal of the field ///The field value as a double override public double GetDouble(int ordinal) { return (double)GetValue(ordinal); } ////// Retrieves the type of a field /// /// The ordinal of the field ///The field type public override Type GetFieldType(int ordinal) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); return _cacheEntry.GetFieldType(ordinal, _metadata); } ////// Retrieves the field value as a float /// /// The ordinal of the field ///The field value as a float override public float GetFloat(int ordinal) { return (float)GetValue(ordinal); } ////// Retrieves the field value as a Guid /// /// The ordinal of the field ///The field value as a Guid override public Guid GetGuid(int ordinal) { return (Guid)GetValue(ordinal); } ////// Retrieves the field value as an Int16 /// /// The ordinal of the field ///The field value as an Int16 override public Int16 GetInt16(int ordinal) { return (Int16)GetValue(ordinal); } ////// Retrieves the field value as an Int32 /// /// The ordinal of the field ///The field value as an Int32 override public Int32 GetInt32(int ordinal) { return (Int32)GetValue(ordinal); } ////// Retrieves the field value as an Int64 /// /// The ordinal of the field ///The field value as an Int64 override public Int64 GetInt64(int ordinal) { return (Int64)GetValue(ordinal); } ////// Retrieves the name of a field /// /// The ordinal of the field ///The name of the field override public string GetName(int ordinal) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); return _cacheEntry.GetCLayerName(ordinal, _metadata); } ////// Retrieves the ordinal of a field by name /// /// The name of the field ///The ordinal of the field override public int GetOrdinal(string name) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); int ordinal = _cacheEntry.GetOrdinalforCLayerName(name, _metadata); if (ordinal == -1) { throw EntityUtil.ArgumentOutOfRange("name"); } return ordinal; } ////// Retrieves the field value as a string /// /// The ordinal of the field ///The field value as a string override public string GetString(int ordinal) { return (string)GetValue(ordinal); } ////// Retrieves the value of a field /// /// The ordinal of the field ///The field value override public object GetValue(int ordinal) { return GetRecordValue(ordinal); } ////// In derived classes, retrieves the record value for a field /// /// The ordinal of the field ///The field value protected abstract object GetRecordValue(int ordinal); ////// Retrieves all field values in the record into an object array /// /// An array of objects to store the field values ///The number of field values returned override public int GetValues(object[] values) { if (values == null) { throw EntityUtil.ArgumentNull("values"); } int minValue = Math.Min(values.Length, FieldCount); for (int i = 0; i < minValue; i++) { values[i] = GetValue(i); } return minValue; } ////// Determines if a field has a DBNull value /// /// The ordinal of the field ///True if the field has a DBNull value override public bool IsDBNull(int ordinal) { return (GetValue(ordinal) == DBNull.Value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// public void SetBoolean(int ordinal, bool value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// public void SetByte(int ordinal, byte value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetChar(int ordinal, char value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDataRecord(int ordinal, IDataRecord value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDateTime(int ordinal, DateTime value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDecimal(int ordinal, Decimal value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetDouble(int ordinal, Double value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetFloat(int ordinal, float value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetGuid(int ordinal, Guid value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetInt16(int ordinal, Int16 value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetInt32(int ordinal, Int32 value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetInt64(int ordinal, Int64 value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetString(int ordinal, string value) { SetValue(ordinal, value); } ////// Sets the value of a field in a record /// /// The ordinal of the field /// The new field value public void SetValue(int ordinal, object value) { SetRecordValue(ordinal, value); } ////// Sets field values in a record /// /// ///The number of fields that were set public int SetValues(params Object[] values) { int minValue = Math.Min(values.Length, FieldCount); for (int i = 0; i < minValue; i++) { SetRecordValue(i, values[i]); } return minValue; } ////// Sets a field to the DBNull value /// /// The ordinal of the field public void SetDBNull(int ordinal) { SetRecordValue(ordinal, DBNull.Value); } ////// Retrieve data record information /// public virtual DataRecordInfo DataRecordInfo { get { if (null == _recordInfo) { Debug.Assert(_cacheEntry != null, "CacheEntry is required."); _recordInfo = _cacheEntry.GetDataRecordInfo(_metadata, _userObject); } return _recordInfo; } } ////// Retrieves a field value as a DbDataRecord /// /// The ordinal of the field ///The field value as a DbDataRecord public DbDataRecord GetDataRecord(int ordinal) { return (DbDataRecord)GetValue(ordinal); } ////// Used to return a nested result /// /// ///public DbDataReader GetDataReader(int i) { return this.GetDbDataReader(i); } /// /// Sets the field value for a given ordinal /// /// in the cspace mapping /// in CSpace protected abstract void SetRecordValue(int ordinal, object value); } public abstract class CurrentValueRecord : DbUpdatableDataRecord { internal CurrentValueRecord(ObjectStateEntry cacheEntry, StateManagerTypeMetadata metadata, object userObject) : base(cacheEntry, metadata, userObject) { } internal CurrentValueRecord(ObjectStateEntry cacheEntry) : base(cacheEntry) { } } public abstract class OriginalValueRecord : DbUpdatableDataRecord { internal OriginalValueRecord(ObjectStateEntry cacheEntry, StateManagerTypeMetadata metadata, object userObject) : base(cacheEntry, metadata, userObject) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
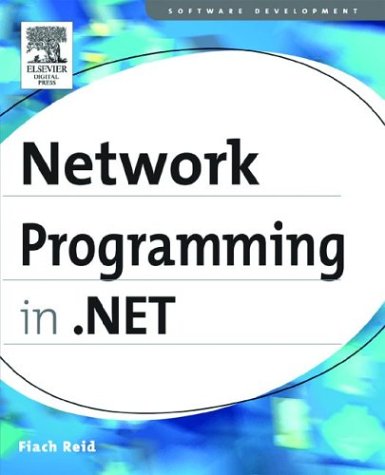
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImageField.cs
- MarkupCompilePass1.cs
- DataContractSerializerSection.cs
- RegexMatch.cs
- XpsS0ValidatingLoader.cs
- coordinator.cs
- EntitySetBaseCollection.cs
- SessionEndedEventArgs.cs
- SimpleFileLog.cs
- OdbcConnectionHandle.cs
- DataServiceConfiguration.cs
- MultiSelector.cs
- InputReferenceExpression.cs
- EntityContainerEmitter.cs
- HtmlPageAdapter.cs
- CLRBindingWorker.cs
- Timer.cs
- UserControlBuildProvider.cs
- DependencyPropertyKey.cs
- documentsequencetextview.cs
- ProgressBarHighlightConverter.cs
- SqlDataSourceSelectingEventArgs.cs
- MenuStrip.cs
- IIS7ConfigurationLoader.cs
- DesignObjectWrapper.cs
- TextBox.cs
- SAPICategories.cs
- UrlAuthFailedErrorFormatter.cs
- BasePattern.cs
- MessageFilterException.cs
- InputMethodStateTypeInfo.cs
- GridSplitter.cs
- QuaternionRotation3D.cs
- IpcChannelHelper.cs
- nulltextcontainer.cs
- Error.cs
- EFColumnProvider.cs
- MD5.cs
- NativeMethods.cs
- BufferModeSettings.cs
- CustomValidator.cs
- BidOverLoads.cs
- ReferenceConverter.cs
- Normalization.cs
- CellCreator.cs
- XmlBuffer.cs
- RelationshipEndCollection.cs
- ContextMarshalException.cs
- DbMetaDataFactory.cs
- FileIOPermission.cs
- SpeakInfo.cs
- ReferentialConstraint.cs
- ServiceNameElementCollection.cs
- DoubleAnimation.cs
- HMACMD5.cs
- WebPartManagerInternals.cs
- StyleCollection.cs
- XmlUTF8TextWriter.cs
- TextTreeInsertElementUndoUnit.cs
- Int32CollectionConverter.cs
- EntityKeyElement.cs
- AffineTransform3D.cs
- XmlUtil.cs
- UserControlCodeDomTreeGenerator.cs
- Walker.cs
- UIElement.cs
- ProvidersHelper.cs
- Registration.cs
- BigIntegerStorage.cs
- EventData.cs
- SQLDecimalStorage.cs
- CustomDictionarySources.cs
- DynamicResourceExtensionConverter.cs
- XamlToRtfWriter.cs
- HopperCache.cs
- EllipseGeometry.cs
- EntityUtil.cs
- RC2CryptoServiceProvider.cs
- MutexSecurity.cs
- DataGridCellAutomationPeer.cs
- PublisherIdentityPermission.cs
- VerificationAttribute.cs
- ScrollBar.cs
- HttpRawResponse.cs
- SecurityContext.cs
- MulticastNotSupportedException.cs
- CustomSignedXml.cs
- ExpressionNode.cs
- FrugalMap.cs
- TextTreeExtractElementUndoUnit.cs
- ImageDrawing.cs
- TreeViewEvent.cs
- CurrencyWrapper.cs
- QuestionEventArgs.cs
- XmlQueryStaticData.cs
- ObjectDataSourceMethodEventArgs.cs
- TextInfo.cs
- RegexGroup.cs
- AuthStoreRoleProvider.cs
- ScaleTransform3D.cs