Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Mapping / StorageEntityTypeMapping.cs / 1305376 / StorageEntityTypeMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Mapping metadata for Entity type. /// If an EntitySet represents entities of more than one type, than we will have /// more than one EntityTypeMapping for an EntitySet( For ex : if /// PersonSet Entity extent represents entities of types Person and Customer, /// than we will have two EntityType Mappings under mapping for PersonSet). /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all entity Type map elements in the /// above example. Users can access the table mapping fragments under the /// entity type mapping through this class. /// internal class StorageEntityTypeMapping : StorageTypeMapping { #region Constructors ////// Construct the new EntityTypeMapping object. /// /// Set Mapping that contains this Type mapping internal StorageEntityTypeMapping(StorageSetMapping setMapping) : base(setMapping) { } #endregion #region Fields private Dictionarym_entityTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for. private Dictionary m_isOfEntityTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for // not only the type specified but the sub-types of that type as well. #endregion #region Properties /// /// a list of TypeMetadata that this mapping holds true for. /// internal override ReadOnlyCollectionTypes { get { return new List (m_entityTypes.Values).AsReadOnly(); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal override ReadOnlyCollectionIsOfTypes { get { return new List (m_isOfEntityTypes.Values).AsReadOnly(); } } #endregion #region Methods /// /// Add a Type to the list of types that this mapping is valid for /// internal void AddType(EdmType type) { this.m_entityTypes.Add(type.FullName, type); } ////// Add a Type to the list of Is-Of types that this mapping is valid for /// internal void AddIsOfType(EdmType type) { this.m_isOfEntityTypes.Add(type.FullName, type); } internal EntityType GetContainerType(string memberName) { foreach (EntityType type in m_entityTypes.Values) { if (type.Properties.Contains(memberName)) { return type; } } foreach (EntityType type in m_isOfEntityTypes.Values) { if (type.Properties.Contains(memberName)) { return type; } } return null; } ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal override void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("EntityTypeMapping"); sb.Append(" "); foreach (EdmType type in m_entityTypes.Values) { sb.Append("Types:"); sb.Append(type.FullName); sb.Append(" "); } foreach (EdmType type in m_isOfEntityTypes.Values) { sb.Append("Is-Of Types:"); sb.Append(type.FullName); sb.Append(" "); } Console.WriteLine(sb.ToString()); foreach (StorageMappingFragment fragment in MappingFragments) { fragment.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; namespace System.Data.Mapping { ////// Mapping metadata for Entity type. /// If an EntitySet represents entities of more than one type, than we will have /// more than one EntityTypeMapping for an EntitySet( For ex : if /// PersonSet Entity extent represents entities of types Person and Customer, /// than we will have two EntityType Mappings under mapping for PersonSet). /// ////// For Example if conceptually you could represent the CS MSL file as following /// --Mapping /// --EntityContainerMapping ( CNorthwind-->SNorthwind ) /// --EntitySetMapping /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityTypeMapping /// --TableMappingFragment /// --EntityKey /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// --AssociationSetMapping /// --AssociationTypeMapping /// --TableMappingFragment /// --EndPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --EndPropertyMap /// --ScalarPropertyMap /// --EntityContainerMapping ( CMyDatabase-->SMyDatabase ) /// --CompositionSetMapping /// --CompositionTypeMapping /// --TableMappingFragment /// --ParentEntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --EntityKey /// --ScalarPropertyMap /// --ScalarPropertyMap /// --ComplexPropertyMap /// --ScalarPropertyMap /// --ScalarProperyMap /// --ScalarPropertyMap /// This class represents the metadata for all entity Type map elements in the /// above example. Users can access the table mapping fragments under the /// entity type mapping through this class. /// internal class StorageEntityTypeMapping : StorageTypeMapping { #region Constructors ////// Construct the new EntityTypeMapping object. /// /// Set Mapping that contains this Type mapping internal StorageEntityTypeMapping(StorageSetMapping setMapping) : base(setMapping) { } #endregion #region Fields private Dictionarym_entityTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for. private Dictionary m_isOfEntityTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for // not only the type specified but the sub-types of that type as well. #endregion #region Properties /// /// a list of TypeMetadata that this mapping holds true for. /// internal override ReadOnlyCollectionTypes { get { return new List (m_entityTypes.Values).AsReadOnly(); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal override ReadOnlyCollectionIsOfTypes { get { return new List (m_isOfEntityTypes.Values).AsReadOnly(); } } #endregion #region Methods /// /// Add a Type to the list of types that this mapping is valid for /// internal void AddType(EdmType type) { this.m_entityTypes.Add(type.FullName, type); } ////// Add a Type to the list of Is-Of types that this mapping is valid for /// internal void AddIsOfType(EdmType type) { this.m_isOfEntityTypes.Add(type.FullName, type); } internal EntityType GetContainerType(string memberName) { foreach (EntityType type in m_entityTypes.Values) { if (type.Properties.Contains(memberName)) { return type; } } foreach (EntityType type in m_isOfEntityTypes.Values) { if (type.Properties.Contains(memberName)) { return type; } } return null; } ////// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal override void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("EntityTypeMapping"); sb.Append(" "); foreach (EdmType type in m_entityTypes.Values) { sb.Append("Types:"); sb.Append(type.FullName); sb.Append(" "); } foreach (EdmType type in m_isOfEntityTypes.Values) { sb.Append("Is-Of Types:"); sb.Append(type.FullName); sb.Append(" "); } Console.WriteLine(sb.ToString()); foreach (StorageMappingFragment fragment in MappingFragments) { fragment.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
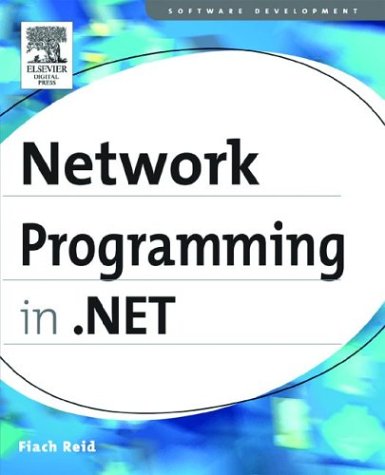
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActivityBindForm.cs
- GridViewEditEventArgs.cs
- FixedSOMTable.cs
- ZoomPercentageConverter.cs
- MessageUtil.cs
- BrowserTree.cs
- DataGridViewCellStyleEditor.cs
- DataGridViewColumnCollection.cs
- XmlElementAttributes.cs
- WebPartManagerInternals.cs
- _IPv4Address.cs
- SchemaReference.cs
- OverflowException.cs
- _ListenerResponseStream.cs
- ZipIOCentralDirectoryFileHeader.cs
- OdbcConnectionFactory.cs
- RequestStatusBarUpdateEventArgs.cs
- CodeTypeConstructor.cs
- UIElementIsland.cs
- WindowsSysHeader.cs
- WindowInteropHelper.cs
- SimpleRecyclingCache.cs
- MimeTextImporter.cs
- XsdCachingReader.cs
- StorageScalarPropertyMapping.cs
- DataGridViewImageColumn.cs
- FrameworkElement.cs
- DataServiceRequestArgs.cs
- ObjectStateFormatter.cs
- CommentEmitter.cs
- PackagingUtilities.cs
- SQLGuidStorage.cs
- CalendarBlackoutDatesCollection.cs
- FamilyTypeface.cs
- SqlRetyper.cs
- versioninfo.cs
- AuthenticationService.cs
- ToolStripOverflow.cs
- WebPartCatalogCloseVerb.cs
- ConfigurationManagerHelperFactory.cs
- CompiledQuery.cs
- ResolveNameEventArgs.cs
- DllNotFoundException.cs
- SqlTriggerContext.cs
- EventRouteFactory.cs
- input.cs
- LayoutUtils.cs
- WebInvokeAttribute.cs
- KernelTypeValidation.cs
- TrimSurroundingWhitespaceAttribute.cs
- AssemblyCache.cs
- Material.cs
- RtfToXamlReader.cs
- thaishape.cs
- DocumentXPathNavigator.cs
- EmissiveMaterial.cs
- DiagnosticTraceSource.cs
- RegistryKey.cs
- XmlDictionary.cs
- SqlInternalConnectionTds.cs
- DelegateHelpers.Generated.cs
- Propagator.JoinPropagator.cs
- ConstraintManager.cs
- FacetDescription.cs
- HashMembershipCondition.cs
- SafeFileMapViewHandle.cs
- ScrollItemPattern.cs
- XmlNamespaceMapping.cs
- MethodCallTranslator.cs
- odbcmetadatafactory.cs
- NonParentingControl.cs
- AccessViolationException.cs
- BaseCAMarshaler.cs
- ColorContext.cs
- BaseCollection.cs
- UnsafeNativeMethodsTablet.cs
- WebBrowser.cs
- LogEntrySerialization.cs
- TranslateTransform.cs
- ListViewGroupItemCollection.cs
- ProxyWebPartManager.cs
- PackWebResponse.cs
- sqlcontext.cs
- XPathNodeIterator.cs
- XmlNodeChangedEventManager.cs
- StrokeCollection2.cs
- DataPagerFieldCommandEventArgs.cs
- BitmapSizeOptions.cs
- LineBreak.cs
- WaitingCursor.cs
- BevelBitmapEffect.cs
- DateTimeConverter2.cs
- DataColumn.cs
- DbCommandTree.cs
- TreeView.cs
- LinkClickEvent.cs
- RandomNumberGenerator.cs
- NetStream.cs
- TextBox.cs
- AndCondition.cs