Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Serializer / ActivitySurrogateSelector.cs / 1305376 / ActivitySurrogateSelector.cs
namespace System.Workflow.ComponentModel.Serialization { using System; using System.IO; using System.Reflection; using System.Collections; using System.Collections.Generic; using System.Xml; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using System.Security.Permissions; public sealed class ActivitySurrogateSelector : SurrogateSelector { private ActivitySurrogate activitySurrogate = new ActivitySurrogate(); private ActivityExecutorSurrogate activityExecutorSurrogate = new ActivityExecutorSurrogate(); private ObjectSurrogate objectSurrogate = new ObjectSurrogate(); private DependencyStoreSurrogate dependencyStoreSurrogate = new DependencyStoreSurrogate(); private XmlDocumentSurrogate domDocSurrogate = new XmlDocumentSurrogate(); private DictionarySurrogate dictionarySurrogate = new DictionarySurrogate(); private GenericQueueSurrogate genericqueueSurrogate = new GenericQueueSurrogate(); private QueueSurrogate queueSurrogate = new QueueSurrogate(); private ListSurrogate listSurrogate = new ListSurrogate(); private SimpleTypesSurrogate simpleTypesSurrogate = new SimpleTypesSurrogate(); private static ActivitySurrogateSelector defaultActivitySurrogateSelector = new ActivitySurrogateSelector(); private static DictionarysurrogateCache = new Dictionary (); public static ActivitySurrogateSelector Default { get { return defaultActivitySurrogateSelector; } } public override ISerializationSurrogate GetSurrogate(Type type, StreamingContext context, out ISurrogateSelector selector) { if (type == null) throw new ArgumentNullException("type"); selector = this; ISerializationSurrogate result = null; bool found; lock (surrogateCache) { found = surrogateCache.TryGetValue(type, out result); } if (found) { return result == null ? base.GetSurrogate(type, context, out selector) : result; } // if type is assignable to activity, then return the surrogate if (typeof(Activity).IsAssignableFrom(type)) { result = this.activitySurrogate; } else if (typeof(ActivityExecutor).IsAssignableFrom(type)) { result = this.activityExecutorSurrogate; } else if (typeof(IDictionary ).IsAssignableFrom(type)) { result = this.dependencyStoreSurrogate; } else if (typeof(XmlDocument).IsAssignableFrom(type)) { result = this.domDocSurrogate; } /*else if (type.IsGenericType) { Type genericType = type.GetGenericTypeDefinition(); if (genericType == typeof(Dictionary<,>)) { result = this.dictionarySurrogate; } else if (genericType == typeof(Queue<>)) { result = this.genericqueueSurrogate; } else if (genericType == typeof(List<>)) { result = this.listSurrogate; } }*/ else if (typeof(Queue) == type) { result = this.queueSurrogate; } else if (typeof(Guid) == type) { result = this.simpleTypesSurrogate; } // else if (typeof(ActivityBind).IsAssignableFrom(type)) { result = this.objectSurrogate; } // else if (typeof(DependencyObject).IsAssignableFrom(type)) { result = this.objectSurrogate; } lock (surrogateCache) { surrogateCache[type] = result; } return result == null ? base.GetSurrogate(type, context, out selector) : result; } private sealed class ObjectSurrogate : ISerializationSurrogate { public void GetObjectData(object obj, SerializationInfo info, StreamingContext context) { info.AddValue("type", obj.GetType()); string[] names = null; MemberInfo[] members = FormatterServicesNoSerializableCheck.GetSerializableMembers(obj.GetType(), out names); object[] memberDatas = FormatterServices.GetObjectData(obj, members); info.AddValue("memberDatas", memberDatas); info.SetType(typeof(ObjectSerializedRef)); } public object SetObjectData(object obj, SerializationInfo info, StreamingContext context, ISurrogateSelector selector) { return null; } [Serializable] private sealed class ObjectSerializedRef : IObjectReference, IDeserializationCallback { private Type type = null; private object[] memberDatas = null; [NonSerialized] private object returnedObject = null; Object IObjectReference.GetRealObject(StreamingContext context) { if (this.returnedObject == null) this.returnedObject = FormatterServices.GetUninitializedObject(this.type); return this.returnedObject; } void IDeserializationCallback.OnDeserialization(object sender) { if (this.returnedObject != null) { string[] names = null; MemberInfo[] members = FormatterServicesNoSerializableCheck.GetSerializableMembers(this.type, out names); FormatterServices.PopulateObjectMembers(this.returnedObject, members, this.memberDatas); this.returnedObject = null; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Serialization { using System; using System.IO; using System.Reflection; using System.Collections; using System.Collections.Generic; using System.Xml; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using System.Security.Permissions; public sealed class ActivitySurrogateSelector : SurrogateSelector { private ActivitySurrogate activitySurrogate = new ActivitySurrogate(); private ActivityExecutorSurrogate activityExecutorSurrogate = new ActivityExecutorSurrogate(); private ObjectSurrogate objectSurrogate = new ObjectSurrogate(); private DependencyStoreSurrogate dependencyStoreSurrogate = new DependencyStoreSurrogate(); private XmlDocumentSurrogate domDocSurrogate = new XmlDocumentSurrogate(); private DictionarySurrogate dictionarySurrogate = new DictionarySurrogate(); private GenericQueueSurrogate genericqueueSurrogate = new GenericQueueSurrogate(); private QueueSurrogate queueSurrogate = new QueueSurrogate(); private ListSurrogate listSurrogate = new ListSurrogate(); private SimpleTypesSurrogate simpleTypesSurrogate = new SimpleTypesSurrogate(); private static ActivitySurrogateSelector defaultActivitySurrogateSelector = new ActivitySurrogateSelector(); private static Dictionary surrogateCache = new Dictionary (); public static ActivitySurrogateSelector Default { get { return defaultActivitySurrogateSelector; } } public override ISerializationSurrogate GetSurrogate(Type type, StreamingContext context, out ISurrogateSelector selector) { if (type == null) throw new ArgumentNullException("type"); selector = this; ISerializationSurrogate result = null; bool found; lock (surrogateCache) { found = surrogateCache.TryGetValue(type, out result); } if (found) { return result == null ? base.GetSurrogate(type, context, out selector) : result; } // if type is assignable to activity, then return the surrogate if (typeof(Activity).IsAssignableFrom(type)) { result = this.activitySurrogate; } else if (typeof(ActivityExecutor).IsAssignableFrom(type)) { result = this.activityExecutorSurrogate; } else if (typeof(IDictionary ).IsAssignableFrom(type)) { result = this.dependencyStoreSurrogate; } else if (typeof(XmlDocument).IsAssignableFrom(type)) { result = this.domDocSurrogate; } /*else if (type.IsGenericType) { Type genericType = type.GetGenericTypeDefinition(); if (genericType == typeof(Dictionary<,>)) { result = this.dictionarySurrogate; } else if (genericType == typeof(Queue<>)) { result = this.genericqueueSurrogate; } else if (genericType == typeof(List<>)) { result = this.listSurrogate; } }*/ else if (typeof(Queue) == type) { result = this.queueSurrogate; } else if (typeof(Guid) == type) { result = this.simpleTypesSurrogate; } // else if (typeof(ActivityBind).IsAssignableFrom(type)) { result = this.objectSurrogate; } // else if (typeof(DependencyObject).IsAssignableFrom(type)) { result = this.objectSurrogate; } lock (surrogateCache) { surrogateCache[type] = result; } return result == null ? base.GetSurrogate(type, context, out selector) : result; } private sealed class ObjectSurrogate : ISerializationSurrogate { public void GetObjectData(object obj, SerializationInfo info, StreamingContext context) { info.AddValue("type", obj.GetType()); string[] names = null; MemberInfo[] members = FormatterServicesNoSerializableCheck.GetSerializableMembers(obj.GetType(), out names); object[] memberDatas = FormatterServices.GetObjectData(obj, members); info.AddValue("memberDatas", memberDatas); info.SetType(typeof(ObjectSerializedRef)); } public object SetObjectData(object obj, SerializationInfo info, StreamingContext context, ISurrogateSelector selector) { return null; } [Serializable] private sealed class ObjectSerializedRef : IObjectReference, IDeserializationCallback { private Type type = null; private object[] memberDatas = null; [NonSerialized] private object returnedObject = null; Object IObjectReference.GetRealObject(StreamingContext context) { if (this.returnedObject == null) this.returnedObject = FormatterServices.GetUninitializedObject(this.type); return this.returnedObject; } void IDeserializationCallback.OnDeserialization(object sender) { if (this.returnedObject != null) { string[] names = null; MemberInfo[] members = FormatterServicesNoSerializableCheck.GetSerializableMembers(this.type, out names); FormatterServices.PopulateObjectMembers(this.returnedObject, members, this.memberDatas); this.returnedObject = null; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
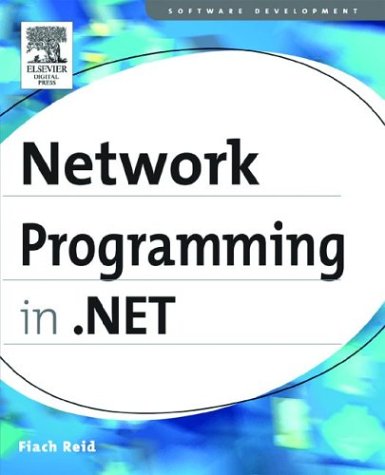
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BooleanToVisibilityConverter.cs
- FontClient.cs
- PageCodeDomTreeGenerator.cs
- BitFlagsGenerator.cs
- DebugView.cs
- HttpAsyncResult.cs
- BlobPersonalizationState.cs
- TypeToTreeConverter.cs
- VisualStyleElement.cs
- Application.cs
- Color.cs
- AutoGeneratedField.cs
- InternalPolicyElement.cs
- WinCategoryAttribute.cs
- AppModelKnownContentFactory.cs
- StreamDocument.cs
- ItemMap.cs
- WindowsScrollBar.cs
- BindUriHelper.cs
- DateTimeFormatInfoScanner.cs
- XmlWrappingReader.cs
- RsaKeyIdentifierClause.cs
- AuthStoreRoleProvider.cs
- HtmlInputPassword.cs
- DbMetaDataCollectionNames.cs
- ElementProxy.cs
- AsnEncodedData.cs
- HebrewCalendar.cs
- SingleSelectRootGridEntry.cs
- NavigationProgressEventArgs.cs
- StylusCaptureWithinProperty.cs
- FileSystemInfo.cs
- IntersectQueryOperator.cs
- NavigationExpr.cs
- WebControlsSection.cs
- ServerTooBusyException.cs
- Image.cs
- FileInfo.cs
- ContentTextAutomationPeer.cs
- DnsEndpointIdentity.cs
- WebServiceParameterData.cs
- ForEachAction.cs
- ValidatingPropertiesEventArgs.cs
- HtmlInputSubmit.cs
- BaseInfoTable.cs
- Literal.cs
- ActivityDesigner.cs
- RadioButtonRenderer.cs
- RegularExpressionValidator.cs
- BlurEffect.cs
- Common.cs
- BamlLocalizer.cs
- UnaryExpressionHelper.cs
- GridViewDeletedEventArgs.cs
- AssociationSetEnd.cs
- KnownBoxes.cs
- ListViewHitTestInfo.cs
- DefaultTraceListener.cs
- _NestedSingleAsyncResult.cs
- InvalidDataException.cs
- OneOfElement.cs
- Html32TextWriter.cs
- TextEffectCollection.cs
- LogicalExpressionTypeConverter.cs
- StructuralComparisons.cs
- MyContact.cs
- ChangeToolStripParentVerb.cs
- TextSelectionHighlightLayer.cs
- CollectionContainer.cs
- SqlProviderManifest.cs
- CodeChecksumPragma.cs
- ContentPlaceHolder.cs
- SqlBuilder.cs
- CompressEmulationStream.cs
- ColorConvertedBitmap.cs
- Rotation3D.cs
- UriExt.cs
- RelationshipConverter.cs
- ColorConverter.cs
- TableMethodGenerator.cs
- ToolStripArrowRenderEventArgs.cs
- XmlTextReader.cs
- DispatchChannelSink.cs
- ComponentSerializationService.cs
- PasswordRecovery.cs
- ParallelDesigner.cs
- Crypto.cs
- HTMLTagNameToTypeMapper.cs
- ResXResourceSet.cs
- ContextMenuStrip.cs
- DataGridViewCheckBoxColumn.cs
- SignatureHelper.cs
- PackageDigitalSignatureManager.cs
- TextContainerChangeEventArgs.cs
- ImageFormat.cs
- ProgramNode.cs
- ConfigurationManagerInternal.cs
- SingleObjectCollection.cs
- ExplicitDiscriminatorMap.cs
- Attachment.cs