Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / SecondaryIndexDefinition.cs / 1 / SecondaryIndexDefinition.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections.Generic; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Encapsulates the Rules and actions of an index. // internal sealed class SecondaryIndexDefinition { // // Summary: // All the pre-defined index names. // public const string GlobalIdIndex = "ix_globalid"; public const string ObjectTypeIndex = "ix_objecttype"; public const string NameIndex = "ix_name"; public const string RecipientIdIndex = "ix_name"; public const string ProductionServiceIndex = "ix_production_svc_uri"; public const string ParentIdIndex = "ix_parentid"; public const string MasterKeyIndex = "ix_masterkey"; public const string SupportedClaimIndex = "ix_supportclaim"; public const string SupportedAuthIndex = "ix_supportauth"; // // Summary: // define the master indexes for use in the inforcard system // static readonly SecondaryIndexDefinition[] s_masterIndexes = new SecondaryIndexDefinition[] { new SecondaryIndexDefinition( GlobalIdIndex,10,20,SecondaryIndexSettings.Unique,Canonicalizers.Binary), new SecondaryIndexDefinition( ObjectTypeIndex,10,20,SecondaryIndexSettings.None,Canonicalizers.Binary ), new SecondaryIndexDefinition( NameIndex,10,20,SecondaryIndexSettings.Nullable,Canonicalizers.CaseInsensitiveWithHashing ), new SecondaryIndexDefinition( ProductionServiceIndex,10,20,SecondaryIndexSettings.Nullable,Canonicalizers.CaseInsensitiveWithHashing ), new SecondaryIndexDefinition( ParentIdIndex,10,20,SecondaryIndexSettings.Nullable,Canonicalizers.Binary ), new SecondaryIndexDefinition( MasterKeyIndex,10,20,SecondaryIndexSettings.Nullable | SecondaryIndexSettings.Unique,Canonicalizers.BinaryWithHashing ), new SecondaryIndexDefinition( SupportedClaimIndex,50,20,SecondaryIndexSettings.Nullable,Canonicalizers.CaseSensitiveWithHashing ), new SecondaryIndexDefinition( SupportedAuthIndex,10,20,SecondaryIndexSettings.Nullable,Canonicalizers.Binary ) }; int m_initialSize; int m_growthFactor; SecondaryIndexSettings m_settings; string m_name; ICanonicalizer m_canonicalizer; private SecondaryIndexDefinition( string name, int initialSize, int growthFactor, SecondaryIndexSettings settings, ICanonicalizer canonicalizer ) { if( String.IsNullOrEmpty( name ) ) { throw IDT.ThrowHelperArgumentNull( "name" ); } if( initialSize <= 0 ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "initialSize", initialSize, SR.GetString( SR.StoreIndexInitialSizeInvalid ) ) ); } if( null == canonicalizer ) { throw IDT.ThrowHelperArgumentNull( "canonicalizer" ); } if( growthFactor <= 0 ) { throw IDT.ThrowHelperError( new ArgumentOutOfRangeException( "growthFactor", growthFactor, SR.GetString( SR.StoreIndexGrowthFactorInvalid ) ) ); } m_initialSize = initialSize; m_growthFactor = growthFactor; m_settings = settings; m_name = name; m_canonicalizer = canonicalizer; } // // Summary: // Gets the global list of indexes for use in the infocard system // public static SecondaryIndexDefinition[] MasterIndexes { get { return s_masterIndexes; } } // // Summary: // Gets the canonicalizer to use with indexes. See Canonicalizers class // public ICanonicalizer Canonicalizer { get{ return m_canonicalizer; } } // // Summary: // Gets the name assiciated with this index. // public string Name { get { return m_name; } } // // Summary: // Gets the initial size of the index. // public int InitialSize { get { return m_initialSize; } } // // Summary: // Gets the growth factor for the index. // public int GrowthFactor { get { return m_growthFactor; } } // // Summary: // Gets the Settings mask for the index. // public SecondaryIndexSettings Settings { get { return m_settings; } } // // Summary: // Return a index definition by name // public static SecondaryIndexDefinition GetByName( string name ) { for( int i=0;i
Link Menu
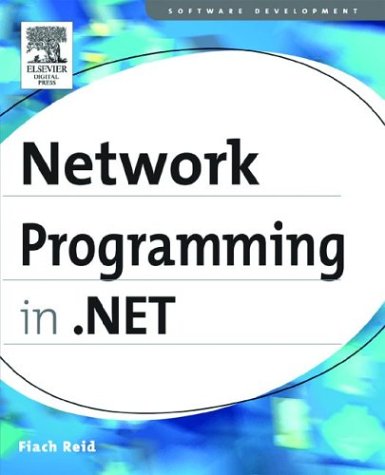
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- webeventbuffer.cs
- FileSystemInfo.cs
- BufferedGraphicsManager.cs
- ClientRoleProvider.cs
- GeneralTransform3DGroup.cs
- ScrollEventArgs.cs
- IResourceProvider.cs
- ToolConsole.cs
- ActionFrame.cs
- XXXInfos.cs
- EmbeddedMailObjectsCollection.cs
- AdRotator.cs
- MultiTrigger.cs
- ThreadStaticAttribute.cs
- PolicyManager.cs
- SettingsBase.cs
- CqlLexerHelpers.cs
- EntityConnectionStringBuilderItem.cs
- XmlSchemas.cs
- ByteAnimationUsingKeyFrames.cs
- SimpleHandlerBuildProvider.cs
- TextLine.cs
- Delay.cs
- ParameterRefs.cs
- SafeEventLogReadHandle.cs
- ObjectNotFoundException.cs
- ColorBlend.cs
- FutureFactory.cs
- ButtonField.cs
- CookielessHelper.cs
- DataControlLinkButton.cs
- ServiceDebugBehavior.cs
- DataGridViewCellEventArgs.cs
- InkCanvasFeedbackAdorner.cs
- Operator.cs
- ExtendedPropertiesHandler.cs
- IPAddressCollection.cs
- XamlTreeBuilderBamlRecordWriter.cs
- UshortList2.cs
- UserControlBuildProvider.cs
- PassportAuthentication.cs
- RelatedView.cs
- StackBuilderSink.cs
- EntityParameter.cs
- GenericIdentity.cs
- TileBrush.cs
- ZoneButton.cs
- WebServiceHandlerFactory.cs
- TemplatePropertyEntry.cs
- ActionMessageFilter.cs
- DrawingVisualDrawingContext.cs
- KeyToListMap.cs
- XmlSubtreeReader.cs
- WindowsToolbarItemAsMenuItem.cs
- FieldNameLookup.cs
- DataKey.cs
- KeyPullup.cs
- XmlDataDocument.cs
- XmlSubtreeReader.cs
- Vector3D.cs
- SmtpNtlmAuthenticationModule.cs
- XmlnsDictionary.cs
- StartFileNameEditor.cs
- TemplateControlParser.cs
- TextUtf8RawTextWriter.cs
- OpenFileDialog.cs
- UnknownBitmapDecoder.cs
- InternalCache.cs
- ExecutionContext.cs
- SafeLibraryHandle.cs
- ArgumentReference.cs
- EnumConverter.cs
- Opcode.cs
- CatalogZone.cs
- DelegateSerializationHolder.cs
- TransactionContext.cs
- OverflowException.cs
- TraceEventCache.cs
- wgx_render.cs
- CanonicalizationDriver.cs
- DelimitedListTraceListener.cs
- ColumnMapTranslator.cs
- ThemeDictionaryExtension.cs
- ComponentEditorForm.cs
- RuntimeTrackingProfile.cs
- BinaryCommonClasses.cs
- DataGridViewColumnCollectionEditor.cs
- CalendarAutoFormat.cs
- Imaging.cs
- ErrorStyle.cs
- WsdlBuildProvider.cs
- RootAction.cs
- ValidationError.cs
- Empty.cs
- ServiceModelEnhancedConfigurationElementCollection.cs
- CodeNamespaceImportCollection.cs
- SubMenuStyle.cs
- XPathNavigatorKeyComparer.cs
- XmlIterators.cs
- DesignTimeValidationFeature.cs