Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / ActionMessageFilter.cs / 1 / ActionMessageFilter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel.Channels; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Runtime.Serialization; [DataContract] public class ActionMessageFilter : MessageFilter { Dictionaryactions; ReadOnlyCollection actionSet; [DataMember(IsRequired = true)] internal string[] DCActions { get { string[] act = new string[this.actions.Count]; actions.Keys.CopyTo(act, 0); return act; } set { Init(value); } } public ActionMessageFilter(params string[] actions) { if(actions == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("actions"); } Init(actions); } void Init(string[] actions) { if(actions.Length == 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.ActionFilterEmptyList), "actions")); } this.actions = new Dictionary (); for(int i = 0; i < actions.Length; ++i) { // Duplicates are removed if(!this.actions.ContainsKey(actions[i])) { this.actions.Add(actions[i],0); } } } public ReadOnlyCollection Actions { get { if(this.actionSet == null) { this.actionSet = new ReadOnlyCollection (new List (this.actions.Keys)); } return this.actionSet; } } protected internal override IMessageFilterTable CreateFilterTable () { return new ActionMessageFilterTable (); } bool InnerMatch(Message message) { string act = message.Headers.Action; if(act == null) { act = string.Empty; } return this.actions.ContainsKey(act); } public override bool Match(Message message) { if(message == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("message"); } return InnerMatch(message); } public override bool Match(MessageBuffer messageBuffer) { if(messageBuffer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("messageBuffer"); } Message msg = messageBuffer.CreateMessage(); try { return InnerMatch(msg); } finally { msg.Close(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
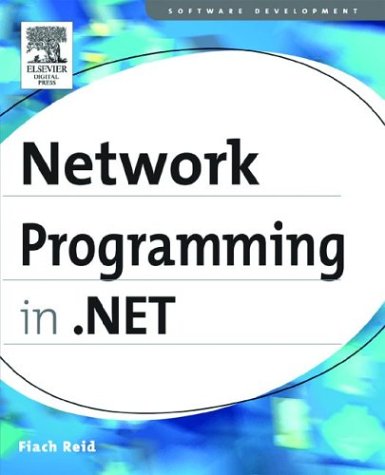
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Metadata.cs
- DecoderNLS.cs
- ExpandSegmentCollection.cs
- DockEditor.cs
- XmlValidatingReaderImpl.cs
- DefaultTextStoreTextComposition.cs
- Converter.cs
- SigningProgress.cs
- SourceItem.cs
- ImportCatalogPart.cs
- TargetConverter.cs
- QueuePropertyVariants.cs
- BinaryWriter.cs
- HwndTarget.cs
- CustomLineCap.cs
- WindowsTokenRoleProvider.cs
- CodePropertyReferenceExpression.cs
- HttpModuleCollection.cs
- CodeNamespaceImportCollection.cs
- InvalidAsynchronousStateException.cs
- Pkcs9Attribute.cs
- MultipleViewProviderWrapper.cs
- SpinWait.cs
- MSHTMLHostUtil.cs
- GridViewColumnCollection.cs
- XmlDictionary.cs
- GroupItemAutomationPeer.cs
- Schema.cs
- AuthStoreRoleProvider.cs
- SizeValueSerializer.cs
- StatusBar.cs
- Automation.cs
- DBPropSet.cs
- SecurityRuntime.cs
- EnterpriseServicesHelper.cs
- DateTimeAutomationPeer.cs
- TextPointer.cs
- PrivateFontCollection.cs
- _FixedSizeReader.cs
- FixedSOMFixedBlock.cs
- UpdateRecord.cs
- DayRenderEvent.cs
- CodeObject.cs
- ChangeTracker.cs
- VisualStyleInformation.cs
- Calendar.cs
- XamlWriter.cs
- dsa.cs
- COM2EnumConverter.cs
- TdsParserSessionPool.cs
- IsolatedStorageFileStream.cs
- ApplyTemplatesAction.cs
- PropVariant.cs
- OdbcPermission.cs
- SerialPort.cs
- ContainerControl.cs
- SQLMembershipProvider.cs
- ProfileGroupSettings.cs
- NativeMethods.cs
- DbUpdateCommandTree.cs
- DataServiceHost.cs
- LinqDataSourceDeleteEventArgs.cs
- AssociationTypeEmitter.cs
- ProtectedProviderSettings.cs
- prefixendpointaddressmessagefiltertable.cs
- AccessDataSource.cs
- WebPartAddingEventArgs.cs
- WebPartTracker.cs
- LinkButton.cs
- WorkflowRequestContext.cs
- DrawingGroupDrawingContext.cs
- ToolStrip.cs
- NativeMethods.cs
- Hash.cs
- SqlClientWrapperSmiStreamChars.cs
- Parallel.cs
- StorageComplexPropertyMapping.cs
- MoveSizeWinEventHandler.cs
- Currency.cs
- PageTheme.cs
- TrustVersion.cs
- ConfigurationManagerHelper.cs
- RealizationContext.cs
- OdbcStatementHandle.cs
- StringStorage.cs
- ControlIdConverter.cs
- ListItemCollection.cs
- ManifestResourceInfo.cs
- FontNamesConverter.cs
- KoreanCalendar.cs
- NamespaceListProperty.cs
- StyleBamlTreeBuilder.cs
- EntityDataSource.cs
- PresentationSource.cs
- CodeCastExpression.cs
- MsmqBindingBase.cs
- Journaling.cs
- PathFigureCollectionConverter.cs
- SpellerHighlightLayer.cs
- mediaclock.cs