Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Serialization / System / Xml / XmlDictionary.cs / 1305376 / XmlDictionary.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Xml { using System; using System.IO; using System.Xml; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Runtime.Serialization; public class XmlDictionary : IXmlDictionary { static IXmlDictionary empty; Dictionarylookup; XmlDictionaryString[] strings; int nextId; static public IXmlDictionary Empty { get { if (empty == null) empty = new EmptyDictionary(); return empty; } } public XmlDictionary() { this.lookup = new Dictionary (); this.strings = null; this.nextId = 0; } public XmlDictionary(int capacity) { this.lookup = new Dictionary (capacity); this.strings = new XmlDictionaryString[capacity]; this.nextId = 0; } public virtual XmlDictionaryString Add(string value) { XmlDictionaryString str; if (!this.lookup.TryGetValue(value, out str)) { if (this.strings == null) { this.strings = new XmlDictionaryString[4]; } else if (this.nextId == this.strings.Length) { int newSize = this.nextId * 2; if (newSize == 0) newSize = 4; Array.Resize(ref this.strings, newSize); } str = new XmlDictionaryString(this, value, this.nextId); this.strings[this.nextId] = str; this.lookup.Add(value, str); this.nextId++; } return str; } public virtual bool TryLookup(string value, out XmlDictionaryString result) { return this.lookup.TryGetValue(value, out result); } public virtual bool TryLookup(int key, out XmlDictionaryString result) { if (key < 0 || key >= this.nextId) { result = null; return false; } result = this.strings[key]; return true; } public virtual bool TryLookup(XmlDictionaryString value, out XmlDictionaryString result) { if (value == null) throw System.Runtime.Serialization.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); if (value.Dictionary != this) { result = null; return false; } result = value; return true; } class EmptyDictionary : IXmlDictionary { public bool TryLookup(string value, out XmlDictionaryString result) { result = null; return false; } public bool TryLookup(int key, out XmlDictionaryString result) { result = null; return false; } public bool TryLookup(XmlDictionaryString value, out XmlDictionaryString result) { result = null; return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
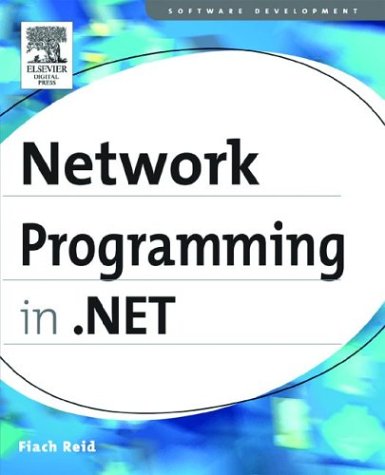
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ModifierKeysConverter.cs
- LayoutTableCell.cs
- HttpListenerPrefixCollection.cs
- AccessControlList.cs
- ColorConverter.cs
- SqlCacheDependencyDatabaseCollection.cs
- HttpRawResponse.cs
- ContextMenu.cs
- ColumnMap.cs
- ContextMenu.cs
- DebuggerService.cs
- HtmlButton.cs
- UrlParameterReader.cs
- CommandValueSerializer.cs
- WsdlBuildProvider.cs
- CompositeKey.cs
- SQLDoubleStorage.cs
- TranslateTransform.cs
- UnmanagedMarshal.cs
- XappLauncher.cs
- CodeSnippetTypeMember.cs
- ShapeTypeface.cs
- followingquery.cs
- SigningDialog.cs
- Properties.cs
- sqlser.cs
- AssociationSet.cs
- Event.cs
- Avt.cs
- RuleSettingsCollection.cs
- InternalEnumValidatorAttribute.cs
- RemotingService.cs
- DataGridColumnHeader.cs
- CodeExporter.cs
- HostProtectionException.cs
- HandoffBehavior.cs
- EdmScalarPropertyAttribute.cs
- RuntimeCompatibilityAttribute.cs
- XmlSchemaSimpleType.cs
- ServicePointManager.cs
- DbConnectionStringCommon.cs
- TargetFrameworkUtil.cs
- AnnotationDocumentPaginator.cs
- StrongBox.cs
- ReferentialConstraintRoleElement.cs
- NotImplementedException.cs
- WindowsFormsDesignerOptionService.cs
- DesignerSerializerAttribute.cs
- InfoCardUIAgent.cs
- MinMaxParagraphWidth.cs
- CacheDependency.cs
- XmlDocument.cs
- nulltextnavigator.cs
- MailAddressCollection.cs
- EventHandlersStore.cs
- AVElementHelper.cs
- Transform3D.cs
- SetIterators.cs
- DelegatingTypeDescriptionProvider.cs
- AxisAngleRotation3D.cs
- FontUnitConverter.cs
- NotifyIcon.cs
- SelectedGridItemChangedEvent.cs
- AssemblyFilter.cs
- EncoderParameter.cs
- ComponentTray.cs
- PropertyChangedEventManager.cs
- InternalConfigConfigurationFactory.cs
- FreeFormDesigner.cs
- InvalidOleVariantTypeException.cs
- SizeAnimation.cs
- MutexSecurity.cs
- EmissiveMaterial.cs
- PocoEntityKeyStrategy.cs
- ToolStripDropDownItem.cs
- Section.cs
- WebPartZone.cs
- RoutedEventArgs.cs
- DesignerActionList.cs
- PackWebRequest.cs
- Rect.cs
- MSAAEventDispatcher.cs
- BaseProcessor.cs
- SqlCachedBuffer.cs
- ControlsConfig.cs
- CodeSnippetStatement.cs
- WebAdminConfigurationHelper.cs
- SimpleNameService.cs
- ParameterDataSourceExpression.cs
- CacheDependency.cs
- PropertyNames.cs
- FloaterBaseParaClient.cs
- WebBrowserProgressChangedEventHandler.cs
- MediaElement.cs
- ColorTransform.cs
- SchemaImporterExtensionElement.cs
- QilTargetType.cs
- SubMenuStyle.cs
- XAMLParseException.cs
- DataGridViewCellConverter.cs