Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Protocol / RegistryConfigurationProvider.cs / 1 / RegistryConfigurationProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Implement the ConfigurationProvider base class for the system registry using System; using System.ServiceModel.Channels; using System.IO; using System.Security; using System.Security.AccessControl; using Microsoft.Transactions.Bridge; using Microsoft.Win32; namespace Microsoft.Transactions.Wsat.Protocol { class RegistryConfigurationProvider : ConfigurationProvider { RegistryKey regKey; public RegistryConfigurationProvider(RegistryKey rootKey, string subKey) { try { if (rootKey != null) { // This can return null if the key does not exist this.regKey = rootKey.OpenSubKey(subKey, RegistryKeyPermissionCheck.ReadSubTree, RegistryRights.ReadKey); } } catch (SecurityException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationProviderException(SR.GetString(SR.RegistryKeyOpenSubKeyFailed, subKey, rootKey.Name, e.Message), e)); } catch (IOException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationProviderException(SR.GetString(SR.RegistryKeyOpenSubKeyFailed, subKey, rootKey.Name, e.Message), e)); } } public override void Dispose() { if (this.regKey != null) { this.regKey.Close(); this.regKey = null; } } object ReadValue(string value, object defaultValue) { if (this.regKey == null) return defaultValue; try { return this.regKey.GetValue(value, defaultValue); } catch (SecurityException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationProviderException(SR.GetString(SR.RegistryKeyGetValueFailed, value, this.regKey.Name, e.Message), e)); } catch (IOException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ConfigurationProviderException(SR.GetString(SR.RegistryKeyGetValueFailed, value, this.regKey.Name, e.Message), e)); } } public override int ReadInteger(string value, int defaultValue) { object regValue = ReadValue(value, defaultValue); if (regValue == null || !(regValue is Int32)) return defaultValue; return (int)regValue; } public override string ReadString(string value, string defaultValue) { object regValue = ReadValue(value, defaultValue); if (regValue == null) return defaultValue; string regString = regValue as string; if (regString == null) return defaultValue; return regString; } public override string[] ReadMultiString(string value, string[] defaultValue) { object regValue = ReadValue(value, defaultValue); if (regValue == null) return defaultValue; string[] regMulti = regValue as string[]; if (regMulti == null) return defaultValue; return regMulti; } public override ConfigurationProvider OpenKey(string key) { return new RegistryConfigurationProvider(this.regKey, key); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
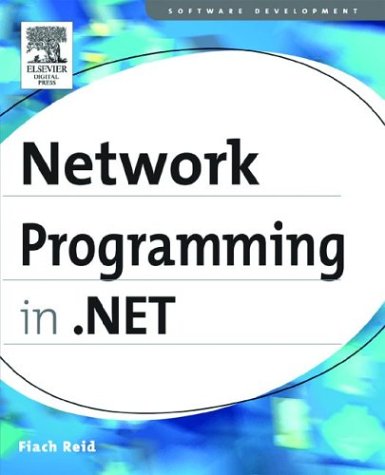
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PackageRelationshipCollection.cs
- ThemeDictionaryExtension.cs
- XmlAutoDetectWriter.cs
- Section.cs
- Manipulation.cs
- StsCommunicationException.cs
- GridViewDeletedEventArgs.cs
- WsatServiceAddress.cs
- ButtonFieldBase.cs
- LexicalChunk.cs
- SoapInteropTypes.cs
- DesignerSerializationOptionsAttribute.cs
- TaiwanCalendar.cs
- FindCriteriaCD1.cs
- UnlockInstanceCommand.cs
- FacetDescription.cs
- UnsafeNativeMethods.cs
- ClientSettingsSection.cs
- RecordsAffectedEventArgs.cs
- BoundField.cs
- FacetEnabledSchemaElement.cs
- TypeConverterAttribute.cs
- TrackingMemoryStreamFactory.cs
- DiffuseMaterial.cs
- ColorDialog.cs
- RemoteWebConfigurationHostStream.cs
- MailMessageEventArgs.cs
- SqlExpressionNullability.cs
- EventLog.cs
- SourceElementsCollection.cs
- UpDownEvent.cs
- HttpPostedFile.cs
- WizardSideBarListControlItemEventArgs.cs
- HitTestParameters.cs
- BitmapEffectDrawing.cs
- CommonServiceBehaviorElement.cs
- DictionaryContent.cs
- ZipIOCentralDirectoryBlock.cs
- MediaPlayerState.cs
- XmlSchema.cs
- ToolStripMenuItem.cs
- BaseDataBoundControl.cs
- TextDecorations.cs
- SchemaTableOptionalColumn.cs
- RowBinding.cs
- ActivityBindForm.cs
- InputBinder.cs
- XmlSerializerFactory.cs
- DecoderNLS.cs
- ImportCatalogPart.cs
- GorillaCodec.cs
- XdrBuilder.cs
- _TransmitFileOverlappedAsyncResult.cs
- InputScopeConverter.cs
- HitTestWithGeometryDrawingContextWalker.cs
- CryptoConfig.cs
- EncoderParameters.cs
- SqlBuffer.cs
- HandleRef.cs
- MeasureItemEvent.cs
- DoubleAnimation.cs
- CompensationHandlingFilter.cs
- FileDialogCustomPlace.cs
- BaseDataListDesigner.cs
- DataGridViewBindingCompleteEventArgs.cs
- EditorAttribute.cs
- NonBatchDirectoryCompiler.cs
- CompiledELinqQueryState.cs
- CachingHintValidation.cs
- RangeContentEnumerator.cs
- DataRecordInternal.cs
- EventItfInfo.cs
- ILGenerator.cs
- TimelineCollection.cs
- RegexReplacement.cs
- MatrixCamera.cs
- FileLogRecordStream.cs
- DispatcherFrame.cs
- OperationExecutionFault.cs
- ByteConverter.cs
- dtdvalidator.cs
- ClientProxyGenerator.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- XmlText.cs
- TypeDescriptorFilterService.cs
- ItemContainerPattern.cs
- MetabaseServerConfig.cs
- TraceInternal.cs
- DockAndAnchorLayout.cs
- FileVersionInfo.cs
- TimerElapsedEvenArgs.cs
- ExpressionPrefixAttribute.cs
- DbProviderFactoriesConfigurationHandler.cs
- TimeEnumHelper.cs
- SystemColors.cs
- TextElement.cs
- InkSerializer.cs
- CodeDelegateInvokeExpression.cs
- FloaterParaClient.cs
- MarshalDirectiveException.cs