Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / NativeMethods.cs / 1 / NativeMethods.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using Microsoft.Win32.SafeHandles; using System; using System.ComponentModel; using System.Diagnostics; using System.Runtime.ConstrainedExecution; using System.Runtime.InteropServices; using System.Security.AccessControl; using System.Text; internal static class NativeMethods { // Service Control Manager constants internal const int ACCESS_SYSTEM_SECURITY = 0x01000000; internal const int DACL_SECURITY_INFORMATION = 0x00000004; internal const int DELETE = 0x00010000; // Http API constants internal const int HTTP_INITIALIZE_CONFIG = 0x00000002; internal const int HTTP_INITIALIZE_SERVER = 0x00000001; internal static HTTPAPI_VERSION HTTPAPI_VERSION = new HTTPAPI_VERSION(1, 0); internal const int SACL_SECURITY_INFORMATION = 0x00000008; internal const int SC_ACTION_NONE = 0; internal const int SC_ACTION_RESTART = 1; internal const int SC_MANAGER_ALL_ACCESS = 0x000F003F; internal const int SE_PRIVILEGE_DISABLED = 0; internal const int SE_PRIVILEGE_ENABLED = 0x00000002; internal const string SE_SECURITY_NAME = "SeSecurityPrivilege"; internal const int SERVICE_CHANGE_CONFIG = 0x0002; internal const int SERVICE_CONFIG_DESCRIPTION = 1; internal const int SERVICE_CONFIG_FAILURE_ACTIONS = 2; internal const int SERVICE_DISABLED = 0x00000004; internal const int SERVICE_ERROR_NORMAL = 0x00000001; internal const int SERVICE_NO_CHANGE = -1; internal const int SERVICE_QUERY_CONFIG = 0x00000001; internal const int SERVICE_START = 0x0010; internal const int SERVICE_WIN32_SHARE_PROCESS = 0x00000020; internal const int TOKEN_ADJUST_PRIVILEGES = 0x0020; internal const int WRITE_DAC = 0x00040000; [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool AdjustTokenPrivileges( SafeTokenHandle TokenHandle, bool DisableAllPrivileges, ref TOKEN_PRIVILEGES NewState, uint BufferLength, IntPtr PreviousState, IntPtr ReturnLength); [DllImport("advapi32.dll", EntryPoint = "ChangeServiceConfig2", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool ChangeFailureActions( ServiceHandle hService, int dwInfoLevel, ref SERVICE_FAILURE_ACTIONS lpInfo); [DllImport("advapi32.dll", EntryPoint = "ChangeServiceConfig", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool ChangeServiceConfig( ServiceHandle hService, int dwServiceType, int dwStartType, int dwErrorControl, string lpBinaryPathName, string lpLoadOrderGroup, IntPtr lpdwTagId, string lpDependencies, string lpServiceStartName, string lpPassword, string lpDisplayName); [DllImport("advapi32.dll", EntryPoint = "ChangeServiceConfig2", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool ChangeServiceDescription( ServiceHandle hService, int dwInfoLevel, ref SERVICE_DESCRIPTION lpInfo); [DllImport("kernel32.dll", SetLastError = true)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static extern bool CloseHandle(IntPtr hObject); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool CloseServiceHandle(IntPtr hSCHandle); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern ServiceHandle CreateService( ServiceManagerHandle hSCManager, string lpServiceName, string lpDisplayName, int dwDesiredAccess, int dwServiceType, int dwStartType, int dwErrorControl, string lpBinaryPathName, string lpLoadOrderGroup, IntPtr lpdwTagId, string lpDependencies, string lpServiceStartName, string lpPassword); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool DeleteService(ServiceHandle hService); [DllImport("httpapi.dll", SetLastError = true)] internal static extern int HttpDeleteServiceConfiguration( IntPtr serviceIntPtr, HTTP_SERVICE_CONFIG_ID configId, ref HTTP_SERVICE_CONFIG_URLACL_SET configInformation, int configInformationLength, IntPtr pOverlapped); [DllImport("httpapi.dll", SetLastError = true)] internal static extern int HttpInitialize( HTTPAPI_VERSION version, int flags, IntPtr pReserved); [DllImport("httpapi.dll", SetLastError = true)] internal static extern int HttpQueryServiceConfiguration( IntPtr ServiceHandle, HTTP_SERVICE_CONFIG_ID ConfigId, ref HTTP_SERVICE_CONFIG_URLACL_QUERY pInputConfigInfo, int InputConfigInfoLength, IntPtr pOutputConfigInfo, int OutputConfigInfoLength, out int pReturnLength, IntPtr pOverlapped); [DllImport("httpapi.dll", SetLastError = true)] internal static extern int HttpSetServiceConfiguration( IntPtr serviceIntPtr, HTTP_SERVICE_CONFIG_ID configId, ref HTTP_SERVICE_CONFIG_URLACL_SET configInformation, int configInformationLength, IntPtr pOverlapped); [DllImport("httpapi.dll", SetLastError = true)] internal static extern int HttpTerminate( int Flags, IntPtr pReserved); [DllImport("kernel32.dll", SetLastError = true)] internal static extern bool IsWow64Process( IntPtr processHandle, out bool isWow64Process); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool LookupPrivilegeValue(string lpSystemName, string lpName, out LUID lpLuid); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool OpenProcessToken( IntPtr ProcessHandle, int DesiredAccess, out SafeTokenHandle TokenHandle); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern ServiceManagerHandle OpenSCManager( string lpMachineName, string lpDatabaseName, int dwDesiredAccess); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern ServiceHandle OpenService( ServiceManagerHandle hSCManager, string lpServiceName, int dwDesiredAccess); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool QueryServiceConfig( ServiceHandle hService, QueryServiceConfigHandle serviceConfigPtr, int cbBufSize, out int pcbBytesNeeded); [DllImport("advapi32.dll")] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static extern int RegCloseKey(IntPtr handle); [DllImport("advapi32.dll", CharSet = CharSet.Unicode)] internal static extern int RegOpenKeyEx(RegistryHandle hKey, string lpSubKey, uint ulOptions, uint samDesired, out RegistryHandle hkResult); [DllImport("advapi32.dll", CharSet = CharSet.Unicode)] internal static extern int RegQueryValueEx(RegistryHandle hKey, string lpValueName, uint[] lpReserved, out int lpType, StringBuilder lpData, ref int lpcbData); [DllImport("advapi32.dll", CharSet = CharSet.Unicode, SetLastError = true)] internal static extern bool SetServiceObjectSecurity( ServiceHandle hService, int dwSecurityInformation, byte[] lpSecurityDescriptor); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
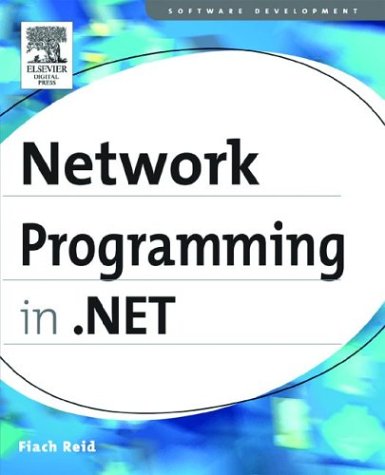
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewUpdatedEventArgs.cs
- WebPartVerb.cs
- TemplateBaseAction.cs
- Int32CollectionValueSerializer.cs
- HttpCacheVaryByContentEncodings.cs
- UrlMappingsSection.cs
- RadioButtonList.cs
- RawUIStateInputReport.cs
- OdbcEnvironment.cs
- CompoundFileStreamReference.cs
- ErrorStyle.cs
- SqlCachedBuffer.cs
- TextSimpleMarkerProperties.cs
- InstalledFontCollection.cs
- UriScheme.cs
- RowToFieldTransformer.cs
- SubMenuStyleCollection.cs
- GetLedgerRequest.cs
- ZoneLinkButton.cs
- RSAPKCS1KeyExchangeFormatter.cs
- XmlMapping.cs
- ListControlConvertEventArgs.cs
- BaseProcessor.cs
- TreeViewDesigner.cs
- GroupByQueryOperator.cs
- RenderDataDrawingContext.cs
- MulticastIPAddressInformationCollection.cs
- CharEntityEncoderFallback.cs
- TableLayoutStyleCollection.cs
- SkewTransform.cs
- SafeEventLogWriteHandle.cs
- CopyAttributesAction.cs
- MemberHolder.cs
- AsyncOperationManager.cs
- BaseTransportHeaders.cs
- RefreshPropertiesAttribute.cs
- StandardOleMarshalObject.cs
- EncodingNLS.cs
- TargetPerspective.cs
- DataKeyArray.cs
- XmlDeclaration.cs
- CredentialCache.cs
- ResourcesChangeInfo.cs
- RegexCharClass.cs
- Translator.cs
- PropertyToken.cs
- ColorPalette.cs
- DesignerSerializerAttribute.cs
- BatchParser.cs
- AlignmentYValidation.cs
- XmlDataSourceView.cs
- NumericUpDown.cs
- Tablet.cs
- Part.cs
- DataSourceView.cs
- PinnedBufferMemoryStream.cs
- ColumnHeaderConverter.cs
- DetailsViewDeletedEventArgs.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- ResXBuildProvider.cs
- AttachedPropertyInfo.cs
- SerializerProvider.cs
- DateTime.cs
- TraceUtility.cs
- ConnectionPointCookie.cs
- PaintValueEventArgs.cs
- Assembly.cs
- RowBinding.cs
- GraphicsPath.cs
- PerformanceCounterManager.cs
- TreeBuilder.cs
- SerialPinChanges.cs
- FileDialog.cs
- ValidationSummary.cs
- AnnotationAdorner.cs
- DbDataSourceEnumerator.cs
- EntityDataSourceSelectedEventArgs.cs
- DefaultParameterValueAttribute.cs
- BitmapSource.cs
- ArrayWithOffset.cs
- EntityCommandDefinition.cs
- PresentationTraceSources.cs
- JsonServiceDocumentSerializer.cs
- OpenTypeMethods.cs
- WindowsProgressbar.cs
- StreamMarshaler.cs
- BindingContext.cs
- BinaryFormatterWriter.cs
- ArrayEditor.cs
- ObjectReferenceStack.cs
- XmlSchemaAppInfo.cs
- ToolTip.cs
- RectangleGeometry.cs
- TemplateKey.cs
- EmptyControlCollection.cs
- ApplicationProxyInternal.cs
- PathFigureCollection.cs
- TypeDefinition.cs
- XamlInterfaces.cs
- FixedDocumentPaginator.cs