Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / StreamMarshaler.cs / 1 / StreamMarshaler.cs
//---------------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Stream Helper. // Allocates a global memory buffer to do marshaling between a // binary and a structured data. The global memory size increases and // never shrinks. // ASAP. // // History: // 5/1/2004 jeanfp //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Text; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal { ////// Summary description for StreamMarshaler. /// internal sealed class StreamMarshaler : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal StreamMarshaler () { } internal StreamMarshaler (Stream stream) { _stream = stream; } public void Dispose () { _safeHMem.Dispose (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods internal void ReadArray(T [] ao, int c) { Type type = typeof (T); int sizeOfOne = Marshal.SizeOf (type); int sizeObject = sizeOfOne * c; byte[] ab = Helpers.ReadStreamToByteArray(_stream, sizeObject); IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ab, 0, buffer, sizeObject); for (int i = 0; i < c; i++) { ao [i] = (T) Marshal.PtrToStructure ((IntPtr) ((long) buffer + i * sizeOfOne), type); } } internal void WriteArray (T [] ao, int c) { Type type = typeof (T); int sizeOfOne = Marshal.SizeOf (type); int sizeObject = sizeOfOne * c; byte [] ab = new byte [sizeObject]; IntPtr buffer = _safeHMem.Buffer (sizeObject); for (int i = 0; i < c; i++) { Marshal.StructureToPtr (ao [i], (IntPtr) ((long) buffer + i * sizeOfOne), false); } Marshal.Copy (buffer, ab, 0, sizeObject); _stream.Write (ab, 0, sizeObject); } internal void ReadArrayChar (char [] ach, int c) { int sizeObject = c * Helpers._sizeOfChar; if (sizeObject > 0) { byte[] ab = Helpers.ReadStreamToByteArray(_stream, sizeObject); IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ab, 0, buffer, sizeObject); Marshal.Copy (buffer, ach, 0, c); } } #pragma warning disable 56518 // BinaryReader can't be disposed because underlying stream still in use. // Helper method to read a Unicode string from a stream. internal string ReadNullTerminatedString () { BinaryReader br = new BinaryReader(_stream, Encoding.Unicode); StringBuilder stringBuilder = new StringBuilder(); while (true) { char c = br.ReadChar(); if (c == '\0') { break; } else { stringBuilder.Append(c); } } return stringBuilder.ToString(); } #pragma warning restore 56518 internal void WriteArrayChar(char[] ach, int c) { int sizeObject = c * Helpers._sizeOfChar; if (sizeObject > 0) { byte [] ab = new byte [sizeObject]; IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ach, 0, buffer, c); Marshal.Copy (buffer, ab, 0, sizeObject); _stream.Write (ab, 0, sizeObject); } } internal void ReadStream (object o) { int sizeObject = Marshal.SizeOf (o.GetType ()); byte[] ab = Helpers.ReadStreamToByteArray(_stream, sizeObject); IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ab, 0, buffer, sizeObject); Marshal.PtrToStructure (buffer, o); } internal void WriteStream (object o) { int sizeObject = Marshal.SizeOf (o.GetType ()); byte [] ab = new byte [sizeObject]; IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.StructureToPtr (o, buffer, false); Marshal.Copy (buffer, ab, 0, sizeObject); // Read the Header _stream.Write (ab, 0, sizeObject); } #endregion //******************************************************************** // // Internal Fields // //******************************************************************** #region internal Fields internal Stream Stream { get { return _stream; } #if VSCOMPILE set { _stream = value; } #endif } internal uint Position { // get // { // return (uint) _stream.Position; // } set { _stream.Position = value; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private HGlobalSafeHandle _safeHMem = new HGlobalSafeHandle (); private Stream _stream; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Stream Helper. // Allocates a global memory buffer to do marshaling between a // binary and a structured data. The global memory size increases and // never shrinks. // ASAP. // // History: // 5/1/2004 jeanfp //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Text; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal { ////// Summary description for StreamMarshaler. /// internal sealed class StreamMarshaler : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal StreamMarshaler () { } internal StreamMarshaler (Stream stream) { _stream = stream; } public void Dispose () { _safeHMem.Dispose (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods internal void ReadArray(T [] ao, int c) { Type type = typeof (T); int sizeOfOne = Marshal.SizeOf (type); int sizeObject = sizeOfOne * c; byte[] ab = Helpers.ReadStreamToByteArray(_stream, sizeObject); IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ab, 0, buffer, sizeObject); for (int i = 0; i < c; i++) { ao [i] = (T) Marshal.PtrToStructure ((IntPtr) ((long) buffer + i * sizeOfOne), type); } } internal void WriteArray (T [] ao, int c) { Type type = typeof (T); int sizeOfOne = Marshal.SizeOf (type); int sizeObject = sizeOfOne * c; byte [] ab = new byte [sizeObject]; IntPtr buffer = _safeHMem.Buffer (sizeObject); for (int i = 0; i < c; i++) { Marshal.StructureToPtr (ao [i], (IntPtr) ((long) buffer + i * sizeOfOne), false); } Marshal.Copy (buffer, ab, 0, sizeObject); _stream.Write (ab, 0, sizeObject); } internal void ReadArrayChar (char [] ach, int c) { int sizeObject = c * Helpers._sizeOfChar; if (sizeObject > 0) { byte[] ab = Helpers.ReadStreamToByteArray(_stream, sizeObject); IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ab, 0, buffer, sizeObject); Marshal.Copy (buffer, ach, 0, c); } } #pragma warning disable 56518 // BinaryReader can't be disposed because underlying stream still in use. // Helper method to read a Unicode string from a stream. internal string ReadNullTerminatedString () { BinaryReader br = new BinaryReader(_stream, Encoding.Unicode); StringBuilder stringBuilder = new StringBuilder(); while (true) { char c = br.ReadChar(); if (c == '\0') { break; } else { stringBuilder.Append(c); } } return stringBuilder.ToString(); } #pragma warning restore 56518 internal void WriteArrayChar(char[] ach, int c) { int sizeObject = c * Helpers._sizeOfChar; if (sizeObject > 0) { byte [] ab = new byte [sizeObject]; IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ach, 0, buffer, c); Marshal.Copy (buffer, ab, 0, sizeObject); _stream.Write (ab, 0, sizeObject); } } internal void ReadStream (object o) { int sizeObject = Marshal.SizeOf (o.GetType ()); byte[] ab = Helpers.ReadStreamToByteArray(_stream, sizeObject); IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.Copy (ab, 0, buffer, sizeObject); Marshal.PtrToStructure (buffer, o); } internal void WriteStream (object o) { int sizeObject = Marshal.SizeOf (o.GetType ()); byte [] ab = new byte [sizeObject]; IntPtr buffer = _safeHMem.Buffer (sizeObject); Marshal.StructureToPtr (o, buffer, false); Marshal.Copy (buffer, ab, 0, sizeObject); // Read the Header _stream.Write (ab, 0, sizeObject); } #endregion //******************************************************************** // // Internal Fields // //******************************************************************** #region internal Fields internal Stream Stream { get { return _stream; } #if VSCOMPILE set { _stream = value; } #endif } internal uint Position { // get // { // return (uint) _stream.Position; // } set { _stream.Position = value; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private HGlobalSafeHandle _safeHMem = new HGlobalSafeHandle (); private Stream _stream; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
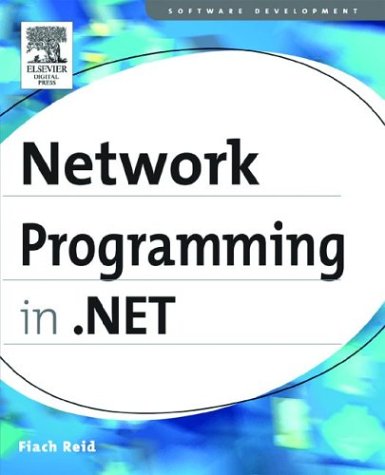
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProxyFragment.cs
- Vector3DCollectionValueSerializer.cs
- DocumentXmlWriter.cs
- SplitContainer.cs
- XPathAncestorQuery.cs
- OleDbConnectionInternal.cs
- ConnectionPool.cs
- ScrollBarAutomationPeer.cs
- ErrorFormatterPage.cs
- DispatcherEventArgs.cs
- XmlQueryOutput.cs
- MobileControlsSectionHandler.cs
- ContentIterators.cs
- VerificationAttribute.cs
- ComplexTypeEmitter.cs
- CodeDOMUtility.cs
- BuilderElements.cs
- KeyValueConfigurationCollection.cs
- KeyPullup.cs
- DodSequenceMerge.cs
- MaterialCollection.cs
- SyndicationSerializer.cs
- StaticTextPointer.cs
- ForwardPositionQuery.cs
- MailMessageEventArgs.cs
- EmptyEnumerable.cs
- ContractMapping.cs
- ObjectFullSpanRewriter.cs
- ConfigurationSchemaErrors.cs
- HandlerFactoryCache.cs
- ConfigurationManagerHelperFactory.cs
- CollectionType.cs
- ButtonFieldBase.cs
- SettingsAttributeDictionary.cs
- EmptyEnumerator.cs
- ToolBar.cs
- MsmqIntegrationOutputChannel.cs
- _UriTypeConverter.cs
- WizardPanelChangingEventArgs.cs
- GridToolTip.cs
- SessionStateItemCollection.cs
- BufferModesCollection.cs
- LayeredChannelFactory.cs
- TypedDataSetSchemaImporterExtensionFx35.cs
- LinearGradientBrush.cs
- ObjectStateFormatter.cs
- CreationContext.cs
- WebPartManagerInternals.cs
- PropertyStore.cs
- LicFileLicenseProvider.cs
- DataGridViewHitTestInfo.cs
- MobileTemplatedControlDesigner.cs
- XmlElementAttribute.cs
- SerializationSectionGroup.cs
- BitConverter.cs
- HttpConfigurationContext.cs
- EdgeModeValidation.cs
- InternalConfigHost.cs
- XmlSchemaNotation.cs
- StrokeCollectionConverter.cs
- SchemaImporter.cs
- HtmlUtf8RawTextWriter.cs
- DotAtomReader.cs
- ThumbButtonInfoCollection.cs
- XsltContext.cs
- SmiMetaData.cs
- CaseCqlBlock.cs
- ISAPIRuntime.cs
- StrongNameKeyPair.cs
- SQLInt64Storage.cs
- XmlKeywords.cs
- BrowserCapabilitiesCodeGenerator.cs
- ObjectSecurity.cs
- XamlWriter.cs
- CustomErrorsSection.cs
- PcmConverter.cs
- ParserOptions.cs
- SourceLineInfo.cs
- BookmarkUndoUnit.cs
- MatrixStack.cs
- DataGridToolTip.cs
- _LocalDataStoreMgr.cs
- RecognitionResult.cs
- MessageBox.cs
- ProcessHostServerConfig.cs
- PanelDesigner.cs
- X509Chain.cs
- Container.cs
- XmlCharType.cs
- UnaryNode.cs
- RefExpr.cs
- TrustSection.cs
- TokenBasedSet.cs
- PageThemeParser.cs
- SqlBooleanMismatchVisitor.cs
- Main.cs
- SiteMapHierarchicalDataSourceView.cs
- safelink.cs
- ExpressionVisitorHelpers.cs
- EventEntry.cs