Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / GetLedgerRequest.cs / 1 / GetLedgerRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // This class handles a UI request to retrieve all the ledger enteries for a card // class GetLedgerRequest :UIAgentRequest { Uri m_cardId; LedgerEntryCollection m_ledger; // // Summary // Creates a GetLedgerRequest object. // public GetLedgerRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { IDT.Assert ( IntPtr.Zero != rpcHandle, "Null rpc handle" ); IDT.Assert ( null != inArgs, "Null inArgs" ); IDT.Assert ( null != outArgs, "Null out args" ); IDT.TraceDebug ( "Processing a edger retrival request" ); } protected override void OnInitializeAsSystem() { base.OnInitializeAsSystem(); } // // Summary // Read the card ID from in input stream. // // Remarks // Expected sequence of data // string - Id of the infocard // protected override void OnMarshalInArgs() { IDT.Assert ( null != InArgs, "null request argument" ); BinaryReader reader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); m_cardId = Utility.DeserializeUri( reader ); } // // Collect the ledger entries from the store. // protected override void OnProcess() { IDT.Assert( null != m_cardId, "No CardId passed to GetLedgerRequest" ); StoreConnection connection = StoreConnection.GetConnection(); try { // // Retrieve ledger entries from the database // m_ledger = new LedgerEntryCollection( m_cardId ); m_ledger.Get( connection ); } finally { connection.Close(); } } // // Summary // Write the ledger entries to the out stream. // // Remarks // Sequence of serialization // ledgercollection // protected override void OnMarshalOutArgs() { IDT.Assert ( null != m_ledger, "No ledger collection exists to be serialized" ); m_ledger.Serialize( OutArgs ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
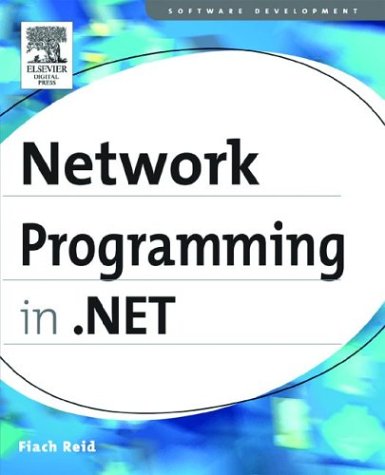
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogPolicy.cs
- RuntimeArgumentHandle.cs
- WorkflowQueuingService.cs
- HtmlWindow.cs
- AvTraceFormat.cs
- ChannelBinding.cs
- FixedPageProcessor.cs
- StateChangeEvent.cs
- _NegoState.cs
- parserscommon.cs
- HttpWebRequest.cs
- ConfigPathUtility.cs
- StrokeCollection2.cs
- VolatileResourceManager.cs
- ProviderException.cs
- OracleColumn.cs
- DbRetry.cs
- RequestCachePolicyConverter.cs
- validationstate.cs
- ControlCachePolicy.cs
- newinstructionaction.cs
- XmlResolver.cs
- WebDescriptionAttribute.cs
- GeometryModel3D.cs
- FixedSOMTextRun.cs
- ConfigurationErrorsException.cs
- DesignParameter.cs
- DirtyTextRange.cs
- FontStretchConverter.cs
- ListItemConverter.cs
- CopyNamespacesAction.cs
- WmlListAdapter.cs
- RunClient.cs
- XmlSchemaInfo.cs
- PerformanceCounterManager.cs
- ImageMap.cs
- SkipQueryOptionExpression.cs
- ClientScriptManagerWrapper.cs
- BitFlagsGenerator.cs
- ProgressBarRenderer.cs
- OfTypeExpression.cs
- FixUpCollection.cs
- BufferBuilder.cs
- RealizedColumnsBlock.cs
- XmlSchemaObject.cs
- EmptyStringExpandableObjectConverter.cs
- DbMetaDataFactory.cs
- RegexStringValidator.cs
- MissingFieldException.cs
- ActiveXHost.cs
- ClientBuildManagerTypeDescriptionProviderBridge.cs
- COM2ComponentEditor.cs
- DesignerAutoFormat.cs
- BaseInfoTable.cs
- HostingEnvironment.cs
- RC2.cs
- DataSourceXmlTextReader.cs
- SerializationAttributes.cs
- ProviderCommandInfoUtils.cs
- ObjectDisposedException.cs
- ObjectStateFormatter.cs
- CapabilitiesPattern.cs
- MimeObjectFactory.cs
- CodeCastExpression.cs
- EditorZone.cs
- Query.cs
- TypeBuilder.cs
- WorkflowCommandExtensionItem.cs
- SchemaDeclBase.cs
- HandleInitializationContext.cs
- CapabilitiesPattern.cs
- XmlValidatingReaderImpl.cs
- Renderer.cs
- Asn1Utilities.cs
- MetadataArtifactLoaderFile.cs
- ToolStripSystemRenderer.cs
- ConfigurationStrings.cs
- COM2ComponentEditor.cs
- log.cs
- OleDbError.cs
- CheckBoxAutomationPeer.cs
- DateTimeValueSerializer.cs
- DescendantOverDescendantQuery.cs
- DataGridItemCollection.cs
- StringValidator.cs
- LayoutEngine.cs
- StreamingContext.cs
- ColorDialog.cs
- IImplicitResourceProvider.cs
- indexingfiltermarshaler.cs
- DataSetMappper.cs
- OdbcDataAdapter.cs
- SoapSchemaImporter.cs
- TextAction.cs
- ServiceOperationParameter.cs
- DataRowComparer.cs
- PieceDirectory.cs
- OrderingQueryOperator.cs
- ProtocolsInstallComponent.cs
- WmlSelectionListAdapter.cs