Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / IisHelper.cs / 2 / IisHelper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using Microsoft.Win32; using System; using System.Collections; using System.ComponentModel; using System.Configuration; using System.DirectoryServices; using System.Runtime.InteropServices; using System.ServiceModel.Install.Configuration; using System.ServiceProcess; using System.IO; using Microsoft.Tools.ServiceModel; static class IisHelper { static bool isApplicationHostInstalled = false; static bool? isAspNetInstalled = null; static bool isWasInstalled = false; static bool isIisInstalled = false; static bool isIisAdminEnabled = false; static bool hasAttemptedRecovery = false; static IisHelper() { try { IisHelper.isApplicationHostInstalled = IIS7ConfigurationLoader.CheckApplicationHostInstalled(); } catch (System.IO.FileNotFoundException) { // FileNotFoundException means that Microsoft.Web.Administration.dll is not installed and we cannot // install ApplicationHost.config entries IisHelper.isApplicationHostInstalled = false; } IisHelper.CheckWasInstalled(); IisHelper.CheckIisInstalled(); } static internal bool GetIsXP() { const int XPMajorVersion = 5; const int XPMinorVersion = 1; return Environment.OSVersion.Version.Major == XPMajorVersion && Environment.OSVersion.Version.Minor == XPMinorVersion; } static internal bool IsRecoverable(COMException e) { return e.ErrorCode == COMErrors.HRESULT_FROM_ERROR_PATH_BUSY; } static internal bool AttemptRecovery() { // the return value is a bool indicating whether // recovery was actually attempted if (hasAttemptedRecovery) { // our recovery code recovers after running once, // if it doesn't, no need to call it any further return false; } // this block of code is here to workaround possible metabase // locking issues that are known to cause our setup to fail at times // call "iisreset /restart timeout:120" and ignore failures to ensure that the metabase // (IISADMIN) is freshly started before we try calling any of its APIs string iisresetPath = Path.Combine(Environment.SystemDirectory, @"iisreset.exe"); const string iisresetParams = "/restart /timeout:120"; EventLogger.LogInformation(SR2.GetString(SR2.IISResetNeeded, iisresetPath + " " + iisresetParams), false); // if iisreset.exe was somehow missing from the box we won't be able to recover // and the following call to TryExecuteWait will throw a FileNotFoundException InstallHelper.TryExecuteWait(OutputLevel.Normal, iisresetPath, iisresetParams); hasAttemptedRecovery = true; return true; } static internal void ValidateDirectoryEntry(DirectoryEntry entry) { try { // Call RefreshCache to force the ADS object to bind entry.RefreshCache(); } catch (COMException e) { if (GetIsXP() && IsRecoverable(e) && AttemptRecovery()) { entry.RefreshCache(); } else { throw; } } } static void CheckAspNetInstalled() { using (DirectoryEntry iisRootEntry = new DirectoryEntry(ServiceModelInstallStrings.IISAdsRoot)) { try { ValidateDirectoryEntry(iisRootEntry); PropertyValueCollection scriptMapCollection = iisRootEntry.Properties[ServiceModelInstallStrings.ScriptMaps]; // Should never be null, but being overly protective here due to timing of this checkin... if (null != scriptMapCollection) { for (int i = 0; i < scriptMapCollection.Count; i++) { string scriptMapEntry = (string)scriptMapCollection[i]; if (!String.IsNullOrEmpty(scriptMapEntry)) { // Sample script map entry: .svc,C:\WINNT\Microsoft.NET\Framework\v2.0.50727\aspnet_isapi.dll,1,GET,HEAD,POST,DEBUG string[] scriptMapParts = scriptMapEntry.Split(new char[] { ',' }, StringSplitOptions.RemoveEmptyEntries); if (scriptMapParts.Length > 1 && scriptMapParts[0].Equals(ServiceModelInstallStrings.AspNetScriptMapPath, StringComparison.OrdinalIgnoreCase) && !String.IsNullOrEmpty((string)scriptMapParts[1])) { // Verify that the ASP.NET ISAPI filter registered is the same as what we expect to register // Normalize current ISAPI filter and expected ISAPI filter in case strings contain // environment variables or are in short path form. string currentIsapiFilter = Environment.ExpandEnvironmentVariables(scriptMapParts[1]); string expectedIsapiFilter = Environment.ExpandEnvironmentVariables(InstallHelper.IsapiFilter); try { currentIsapiFilter = Path.GetFullPath(currentIsapiFilter); expectedIsapiFilter = Path.GetFullPath(expectedIsapiFilter); if (scriptMapParts[1].Equals(InstallHelper.IsapiFilter, StringComparison.OrdinalIgnoreCase)) { IisHelper.isAspNetInstalled = true; break; } } // The system could not retrieve the absolute path catch (ArgumentException) { } // The caller does not have the required permissions catch (System.Security.SecurityException) { } // Path contains a colon (":") catch (NotSupportedException) { } // The specified path, file name, or both exceed the system-defined maximum length catch (PathTooLongException) { } } } } } } catch (COMException e) { throw WrapIisError(e); } } } static internal Exception WrapIisError(COMException e) { if (e.ErrorCode == COMErrors.HRESULT_FROM_ERROR_PATH_BUSY) { return new ApplicationException(SR2.GetString(SR2.LockedIISMetabaseError), e); } return new ApplicationException(SR.GetString(SR.CorruptIISMetabaseError), e); } static void CheckWasInstalled() { try { using (ServiceController controller = new ServiceController(ServiceModelInstallStrings.WasName)) { IisHelper.isWasInstalled = controller.ServiceName.Equals(ServiceModelInstallStrings.WasName, StringComparison.OrdinalIgnoreCase); } } catch (InvalidOperationException exception) { Win32Exception innerException = exception.InnerException as Win32Exception; if (innerException == null || innerException.NativeErrorCode != ErrorCodes.ERROR_SERVICE_DOES_NOT_EXIST) { throw; } } } static void CheckIisInstalled() { try { using (ServiceController w3svcController = new ServiceController(ServiceModelInstallStrings.W3svc)) { if (w3svcController.ServiceName.Equals(ServiceModelInstallStrings.W3svc, StringComparison.OrdinalIgnoreCase)) { IisHelper.isIisInstalled = true; } else { IisHelper.isIisInstalled = false; } } // Only check the enable state for IIS6 or lower. if (IisHelper.isIisInstalled && !IisHelper.isWasInstalled) { if (IisHelper.IsServiceEnabled(ServiceModelInstallStrings.IISAdmin)) { IisHelper.isIisAdminEnabled = true; } else { IisHelper.isIisAdminEnabled = false; } } } catch (InvalidOperationException exception) { Win32Exception innerException = exception.InnerException as Win32Exception; if (innerException == null || innerException.NativeErrorCode != ErrorCodes.ERROR_SERVICE_DOES_NOT_EXIST) { throw; } } } static bool IsServiceEnabled(string serviceName) { bool isServiceEnabled = false; ServiceManagerHandle scManagerHandle = null; ServiceHandle serviceHandle = null; using (scManagerHandle = ServiceManagerHandle.OpenServiceManager()) { using (serviceHandle = scManagerHandle.OpenService(serviceName, NativeMethods.SERVICE_QUERY_CONFIG)) { QUERY_SERVICE_CONFIG serviceConfig = serviceHandle.QueryServiceConfig(); isServiceEnabled = (NativeMethods.SERVICE_DISABLED != serviceConfig.dwStartType); } } return isServiceEnabled; } internal static bool ShouldEnableIISAdmin { get { return IisHelper.isIisAdminEnabled; } } internal static bool ShouldInstallIis { get { return IisHelper.isIisInstalled; } } internal static bool ShouldInstallScriptMaps { get { if (IisHelper.isAspNetInstalled == null) { IisHelper.isAspNetInstalled = false; // What we do here is to check ASP.NET ScriptMaps only if W3SVC is installed, IISAdmin is enabled, // and WAS is not installed. This infers that this is for downlevel only because it cannot happen // that W3SVC & IISAdmin are installed and WAS is not installed on Vista or above. if (IisHelper.isIisInstalled && IisHelper.isIisAdminEnabled && !IisHelper.isWasInstalled) { IisHelper.CheckAspNetInstalled(); } } return IisHelper.isAspNetInstalled.Value; } } internal static bool ShouldInstallWas { get { return IisHelper.isWasInstalled; } } internal static bool ShouldInstallApplicationHost { get { return IisHelper.isApplicationHostInstalled; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
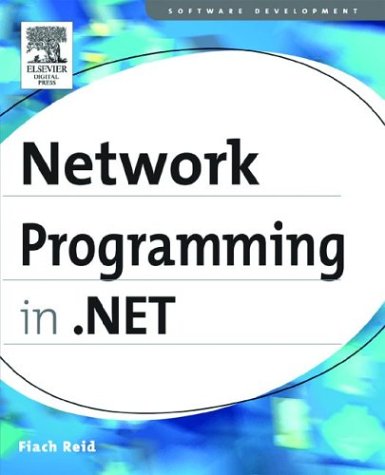
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NetMsmqSecurityElement.cs
- EarlyBoundInfo.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- SAPICategories.cs
- Triplet.cs
- ResourceCodeDomSerializer.cs
- QueryOutputWriter.cs
- ModuleBuilderData.cs
- XmlDocumentFieldSchema.cs
- SelectionListComponentEditor.cs
- SecurityTimestamp.cs
- ExpressionVisitor.cs
- LogSwitch.cs
- LassoSelectionBehavior.cs
- ProfileManager.cs
- NameTable.cs
- COM2FontConverter.cs
- TemplatePartAttribute.cs
- FormViewPageEventArgs.cs
- PropertyDescriptorComparer.cs
- OdbcConnectionStringbuilder.cs
- Rect3D.cs
- LongMinMaxAggregationOperator.cs
- VisualStyleRenderer.cs
- PeerObject.cs
- DriveNotFoundException.cs
- DataServiceRequest.cs
- ServiceBusyException.cs
- ChannelSinkStacks.cs
- WindowsRichEditRange.cs
- RectangleGeometry.cs
- Wildcard.cs
- OrderToken.cs
- ToolStripRenderEventArgs.cs
- VirtualizedItemPattern.cs
- XhtmlConformanceSection.cs
- StickyNoteHelper.cs
- ADRoleFactory.cs
- Statements.cs
- AdornedElementPlaceholder.cs
- ThicknessAnimationBase.cs
- DataGridViewImageColumn.cs
- FontWeightConverter.cs
- XPathEmptyIterator.cs
- BamlReader.cs
- AddingNewEventArgs.cs
- HandlerWithFactory.cs
- TypeUnloadedException.cs
- ControlsConfig.cs
- SettingsSection.cs
- SqlDelegatedTransaction.cs
- AuthenticationSection.cs
- GridSplitterAutomationPeer.cs
- StylusPoint.cs
- Point4D.cs
- RectAnimationClockResource.cs
- StylusTip.cs
- WebColorConverter.cs
- AttributeSetAction.cs
- PropertyGridView.cs
- FreezableOperations.cs
- CompilerCollection.cs
- DetailsViewRow.cs
- PropertyIdentifier.cs
- _NtlmClient.cs
- AdPostCacheSubstitution.cs
- RegistryConfigurationProvider.cs
- StructureChangedEventArgs.cs
- XmlQueryCardinality.cs
- Simplifier.cs
- BooleanSwitch.cs
- FilterEventArgs.cs
- OneOfTypeConst.cs
- DES.cs
- InstanceDataCollectionCollection.cs
- ObjectParameter.cs
- FaultConverter.cs
- Brush.cs
- CalendarDay.cs
- ExceptionAggregator.cs
- MetadataSource.cs
- AttributeData.cs
- arclist.cs
- Cursor.cs
- RadioButton.cs
- RuntimeResourceSet.cs
- SystemUdpStatistics.cs
- MissingSatelliteAssemblyException.cs
- UpdateManifestForBrowserApplication.cs
- PictureBox.cs
- TraceContext.cs
- WebBrowserBase.cs
- Tokenizer.cs
- ProfileSettingsCollection.cs
- HttpContext.cs
- ViewStateModeByIdAttribute.cs
- TypeUnloadedException.cs
- XsdDuration.cs
- ServiceSecurityAuditBehavior.cs
- BaseResourcesBuildProvider.cs