Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / AttributeSetAction.cs / 1 / AttributeSetAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Collections; internal class AttributeSetAction : ContainerAction { internal XmlQualifiedName name; internal XmlQualifiedName Name { get { return this.name; } } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.name, Keywords.s_Name); CompileContent(compiler); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { Debug.Assert(this.name == null); this.name = compiler.CreateXPathQName(value); } else if (Keywords.Equals(name, compiler.Atoms.UseAttributeSets)) { // create a UseAttributeSetsAction // sets come before xsl:attributes AddAction(compiler.CreateUseAttributeSetsAction()); } else { return false; } return true; } private void CompileContent(Compiler compiler) { NavigatorInput input = compiler.Input; if (compiler.Recurse()) { do { switch(input.NodeType) { case XPathNodeType.Element: compiler.PushNamespaceScope(); string nspace = input.NamespaceURI; string name = input.LocalName; if (Keywords.Equals(nspace, input.Atoms.XsltNamespace) && Keywords.Equals(name, input.Atoms.Attribute)) { // found attribute so add it AddAction(compiler.CreateAttributeAction()); } else { throw compiler.UnexpectedKeyword(); } compiler.PopScope(); break; case XPathNodeType.Comment: case XPathNodeType.ProcessingInstruction: case XPathNodeType.Whitespace: case XPathNodeType.SignificantWhitespace: break; default: throw XsltException.Create(Res.Xslt_InvalidContents, Keywords.s_AttributeSet); } } while(compiler.Advance()); compiler.ToParent(); } } internal void Merge(AttributeSetAction attributeAction) { // add the contents of "attributeAction" to this action // place them at the end Action action; int i = 0; while((action = attributeAction.GetAction(i)) != null) { AddAction(action); i++; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; using System.Collections; internal class AttributeSetAction : ContainerAction { internal XmlQualifiedName name; internal XmlQualifiedName Name { get { return this.name; } } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.name, Keywords.s_Name); CompileContent(compiler); } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { Debug.Assert(this.name == null); this.name = compiler.CreateXPathQName(value); } else if (Keywords.Equals(name, compiler.Atoms.UseAttributeSets)) { // create a UseAttributeSetsAction // sets come before xsl:attributes AddAction(compiler.CreateUseAttributeSetsAction()); } else { return false; } return true; } private void CompileContent(Compiler compiler) { NavigatorInput input = compiler.Input; if (compiler.Recurse()) { do { switch(input.NodeType) { case XPathNodeType.Element: compiler.PushNamespaceScope(); string nspace = input.NamespaceURI; string name = input.LocalName; if (Keywords.Equals(nspace, input.Atoms.XsltNamespace) && Keywords.Equals(name, input.Atoms.Attribute)) { // found attribute so add it AddAction(compiler.CreateAttributeAction()); } else { throw compiler.UnexpectedKeyword(); } compiler.PopScope(); break; case XPathNodeType.Comment: case XPathNodeType.ProcessingInstruction: case XPathNodeType.Whitespace: case XPathNodeType.SignificantWhitespace: break; default: throw XsltException.Create(Res.Xslt_InvalidContents, Keywords.s_AttributeSet); } } while(compiler.Advance()); compiler.ToParent(); } } internal void Merge(AttributeSetAction attributeAction) { // add the contents of "attributeAction" to this action // place them at the end Action action; int i = 0; while((action = attributeAction.GetAction(i)) != null) { AddAction(action); i++; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
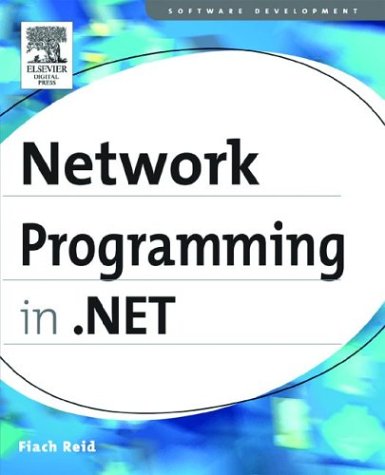
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TraceListeners.cs
- SqlXml.cs
- ObjectDataSourceSelectingEventArgs.cs
- ProcessMonitor.cs
- TransformGroup.cs
- TypeNameConverter.cs
- ComponentTray.cs
- WorkflowPageSetupDialog.cs
- ZoneButton.cs
- Button.cs
- PaperSize.cs
- DocComment.cs
- DataGridViewControlCollection.cs
- MarkupObject.cs
- __Error.cs
- ActivityTypeResolver.xaml.cs
- LinkedResource.cs
- SizeConverter.cs
- TextOnlyOutput.cs
- Preprocessor.cs
- SubMenuStyleCollection.cs
- SettingsPropertyValueCollection.cs
- CheckBox.cs
- LOSFormatter.cs
- TableLayoutRowStyleCollection.cs
- HttpException.cs
- SponsorHelper.cs
- SQLBytes.cs
- HeaderCollection.cs
- SafeProcessHandle.cs
- SortedDictionary.cs
- GridViewSortEventArgs.cs
- ZipFileInfoCollection.cs
- KeyValuePairs.cs
- RecordManager.cs
- ZeroOpNode.cs
- RootBrowserWindowProxy.cs
- LicenseException.cs
- ZeroOpNode.cs
- ValidationResult.cs
- ExpressionList.cs
- XmlTextReader.cs
- DeclaredTypeElementCollection.cs
- ObjectParameter.cs
- DataGridViewToolTip.cs
- CreateCardRequest.cs
- linebase.cs
- OutOfProcStateClientManager.cs
- RootAction.cs
- CheckBoxList.cs
- CoreSwitches.cs
- GraphicsPath.cs
- BehaviorDragDropEventArgs.cs
- Semaphore.cs
- TriState.cs
- safemediahandle.cs
- METAHEADER.cs
- AutomationFocusChangedEventArgs.cs
- Encoder.cs
- ReferenceEqualityComparer.cs
- ListViewDeleteEventArgs.cs
- PartitionResolver.cs
- XmlSchemaType.cs
- PlatformCulture.cs
- FixedMaxHeap.cs
- FileEnumerator.cs
- WebPartZoneCollection.cs
- QilStrConcat.cs
- ListMarkerSourceInfo.cs
- ManipulationCompletedEventArgs.cs
- DataGridState.cs
- ConnectionPool.cs
- SiteMapProvider.cs
- FormsAuthenticationConfiguration.cs
- JsonByteArrayDataContract.cs
- dbenumerator.cs
- TextSelection.cs
- xmlglyphRunInfo.cs
- DataServiceContext.cs
- DataGridColumnCollection.cs
- DecimalMinMaxAggregationOperator.cs
- WebPartConnectionCollection.cs
- TextRunTypographyProperties.cs
- _Rfc2616CacheValidators.cs
- SineEase.cs
- EdgeModeValidation.cs
- StandardToolWindows.cs
- UrlPath.cs
- ObjectListDesigner.cs
- SetStateEventArgs.cs
- Exceptions.cs
- CopyAction.cs
- ResourcesChangeInfo.cs
- COM2IDispatchConverter.cs
- Transform3DGroup.cs
- CustomAttribute.cs
- ScriptReferenceBase.cs
- BitmapImage.cs
- ControllableStoryboardAction.cs
- MultiTrigger.cs