Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Configuration / AddressHeaderCollectionElement.cs / 1 / AddressHeaderCollectionElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Configuration { using System; using System.Configuration; using System.ServiceModel.Channels; using System.Xml; using System.Security; public sealed partial class AddressHeaderCollectionElement : ConfigurationElement { public AddressHeaderCollectionElement() { } [ConfigurationProperty(ConfigurationStrings.Headers, DefaultValue = null)] public AddressHeaderCollection Headers { get { AddressHeaderCollection retVal = (AddressHeaderCollection)base[ConfigurationStrings.Headers]; if (null == retVal) { retVal = AddressHeaderCollection.EmptyHeaderCollection; } return retVal; } set { if (value == null) { value = AddressHeaderCollection.EmptyHeaderCollection; } base[ConfigurationStrings.Headers] = value; } } ////// Critical - uses the critical helper SetIsPresent /// Safe - controls how/when SetIsPresent is used, not arbitrarily callable from PT (method is protected and class is sealed) /// [SecurityCritical, SecurityTreatAsSafe] protected override void DeserializeElement(XmlReader reader, bool serializeCollectionKey) { SetIsPresent(); DeserializeElementCore(reader); } private void DeserializeElementCore(XmlReader reader) { this.Headers = AddressHeaderCollection.ReadServiceParameters(XmlDictionaryReader.CreateDictionaryReader(reader)); } ////// Critical - calls ConfigurationHelpers.SetIsPresent which elevates in order to set a property /// Safe - only passes 'this', does not let caller influence parameter /// [SecurityCritical] void SetIsPresent() { ConfigurationHelpers.SetIsPresent(this); } protected override bool SerializeToXmlElement(XmlWriter writer, String elementName) { bool dataToWrite = this.Headers.Count != 0; if (dataToWrite && writer != null) { writer.WriteStartElement(elementName); this.Headers.WriteContentsTo(XmlDictionaryWriter.CreateDictionaryWriter(writer)); writer.WriteEndElement(); } return dataToWrite; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
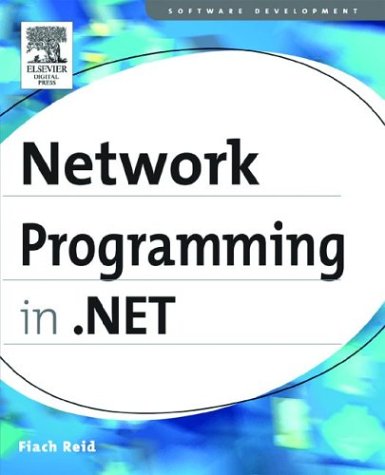
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchema.cs
- IERequestCache.cs
- StateItem.cs
- ColorConverter.cs
- CallbackValidator.cs
- ItemCheckedEvent.cs
- EncryptedData.cs
- ApplicationBuildProvider.cs
- PasswordPropertyTextAttribute.cs
- TableLayoutSettingsTypeConverter.cs
- PageHandlerFactory.cs
- SByteConverter.cs
- WeakReference.cs
- DropTarget.cs
- Model3D.cs
- XPathDocumentBuilder.cs
- IssuedTokenParametersEndpointAddressElement.cs
- StreamWithDictionary.cs
- CodeGenerator.cs
- DocumentSignatureManager.cs
- GlyphsSerializer.cs
- TextDpi.cs
- DataGridState.cs
- CodePageUtils.cs
- BamlCollectionHolder.cs
- QueryOperationResponseOfT.cs
- Frame.cs
- RegistryExceptionHelper.cs
- SamlAttributeStatement.cs
- HttpSessionStateWrapper.cs
- RequestSecurityTokenResponseCollection.cs
- LinkConverter.cs
- MemoryPressure.cs
- bidPrivateBase.cs
- AccessibleObject.cs
- HtmlHead.cs
- PrivilegedConfigurationManager.cs
- FileCodeGroup.cs
- GenericQueueSurrogate.cs
- LoadGrammarCompletedEventArgs.cs
- CompositeControl.cs
- PointAnimationUsingKeyFrames.cs
- ObservableDictionary.cs
- TextBoxView.cs
- ConfigurationLocationCollection.cs
- AsyncStreamReader.cs
- LayoutTable.cs
- CapabilitiesRule.cs
- Substitution.cs
- CurrentChangedEventManager.cs
- GenericIdentity.cs
- ListParagraph.cs
- NativeMethods.cs
- AttachInfo.cs
- SerializationHelper.cs
- SymLanguageVendor.cs
- ScriptingProfileServiceSection.cs
- TreeNodeCollection.cs
- BasicCommandTreeVisitor.cs
- ServicePointManagerElement.cs
- TypeSemantics.cs
- DataStreamFromComStream.cs
- UInt32.cs
- SafeBitVector32.cs
- ReferenceEqualityComparer.cs
- TemplateParser.cs
- EmptyEnumerator.cs
- XmlNodeReader.cs
- InkCanvasSelection.cs
- ClientType.cs
- ReliabilityContractAttribute.cs
- ResolveNameEventArgs.cs
- FocusChangedEventArgs.cs
- MetaForeignKeyColumn.cs
- ExtendedPropertyInfo.cs
- COM2IProvidePropertyBuilderHandler.cs
- ToolStripOverflow.cs
- EventLog.cs
- FocusManager.cs
- BaseTreeIterator.cs
- WindowsRegion.cs
- COAUTHIDENTITY.cs
- TypeDescriptionProvider.cs
- ValueType.cs
- ParallelEnumerableWrapper.cs
- CannotUnloadAppDomainException.cs
- IndicFontClient.cs
- fixedPageContentExtractor.cs
- RelatedPropertyManager.cs
- AnonymousIdentificationModule.cs
- addressfiltermode.cs
- Canvas.cs
- DocumentSequenceHighlightLayer.cs
- ExpressionCopier.cs
- TraceContextRecord.cs
- GlyphingCache.cs
- VectorCollectionConverter.cs
- XmlSchemaObjectTable.cs
- LambdaCompiler.Generated.cs
- OleDbInfoMessageEvent.cs