Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / TypedServiceChannelBuilder.cs / 1 / TypedServiceChannelBuilder.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- #pragma warning disable 1634, 1691 namespace System.ServiceModel.ComIntegration { using System; using System.ServiceModel.Description; using System.Reflection; using System.Collections.Generic; using System.Threading; using System.ServiceModel; using System.ServiceModel.Channels; using System.Runtime.Remoting.Proxies; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Diagnostics; using System.ServiceModel.Diagnostics; internal class TypedServiceChannelBuilder : IProxyCreator, IProvideChannelBuilderSettings, ICreateServiceChannel { ServiceChannelFactory serviceChannelFactory = null; Type contractType = null; RealProxy serviceProxy = null; ServiceEndpoint serviceEndpoint = null; KeyedByTypeCollectionbehaviors = new KeyedByTypeCollection (); Binding binding = null; string configurationName = null ; string address = null; EndpointIdentity identity = null; void IDisposable.Dispose () { if (serviceProxy != null) { IChannel channel = serviceProxy.GetTransparentProxy () as IChannel; if (channel == null) { DiagnosticUtility.DebugAssert("serviceProxy MUST support IChannel"); #pragma warning suppress 56519 // throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } channel.Close (); } } //Suppressing PreSharp warning that property get methods should not throw #pragma warning disable 6503 ServiceChannelFactory IProvideChannelBuilderSettings.ServiceChannelFactoryReadWrite { get { if (serviceProxy != null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new COMException (SR.GetString (SR.TooLate), HR.RPC_E_TOO_LATE)); return serviceChannelFactory; } } #pragma warning restore 6503 ServiceChannelFactory IProvideChannelBuilderSettings.ServiceChannelFactoryReadOnly { get { return serviceChannelFactory; } } //Suppressing PreSharp warning that property get methods should not throw #pragma warning disable 6503 KeyedByTypeCollection IProvideChannelBuilderSettings.Behaviors { get { if (serviceProxy != null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new COMException (SR.GetString (SR.TooLate), HR.RPC_E_TOO_LATE)); return behaviors; } } #pragma warning restore 6503 ServiceChannel IProvideChannelBuilderSettings.ServiceChannel { get { return null; } } RealProxy ICreateServiceChannel.CreateChannel () { if (serviceProxy == null) { lock (this) { if (serviceProxy == null) { try { if (serviceChannelFactory == null) { FaultInserviceChannelFactory(); } if (serviceChannelFactory == null) { DiagnosticUtility.DebugAssert("ServiceChannelFactory cannot be null at this point"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } serviceChannelFactory.Open (); if (contractType == null) { DiagnosticUtility.DebugAssert("contractType cannot be null"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } if (serviceEndpoint == null) { DiagnosticUtility.DebugAssert("serviceEndpoint cannot be null"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } object transparentProxy = serviceChannelFactory.CreateChannel (contractType, new EndpointAddress(serviceEndpoint.Address.Uri, serviceEndpoint.Address.Identity, serviceEndpoint.Address.Headers), serviceEndpoint.Address.Uri); ComPlusChannelCreatedTrace.Trace(TraceEventType.Verbose, TraceCode.ComIntegrationChannelCreated, SR.TraceCodeComIntegrationChannelCreated, serviceEndpoint.Address.Uri, contractType); RealProxy localProxy = RemotingServices.GetRealProxy (transparentProxy); Thread.MemoryBarrier(); serviceProxy = localProxy; if (serviceProxy == null) { DiagnosticUtility.DebugAssert("serviceProxy MUST derive from RealProxy"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } } finally { if ((serviceProxy == null) && (serviceChannelFactory != null)) serviceChannelFactory.Close (); } } } } return serviceProxy; } private ServiceEndpoint CreateServiceEndpoint() { TypeLoader loader = new TypeLoader(); ContractDescription contractDescription = loader.LoadContractDescription(contractType); ServiceEndpoint endpoint = new ServiceEndpoint(contractDescription); if (address != null) endpoint.Address = new EndpointAddress(new Uri(address), identity); if (binding != null) endpoint.Binding = binding; if (configurationName != null) { ConfigLoader configLoader = new ConfigLoader(); configLoader.LoadChannelBehaviors(endpoint, configurationName); } ComPlusTypedChannelBuilderTrace.Trace(TraceEventType.Verbose, TraceCode.ComIntegrationTypedChannelBuilderLoaded, SR.TraceCodeComIntegrationTypedChannelBuilderLoaded, contractType, binding); return endpoint; } private ServiceChannelFactory CreateServiceChannelFactory () { ServiceChannelFactory serviceChannelFactory = ServiceChannelFactory.BuildChannelFactory(serviceEndpoint) as ServiceChannelFactory; if (serviceChannelFactory == null) { DiagnosticUtility.DebugAssert("We should get a ServiceChannelFactory back"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } return serviceChannelFactory; } void FaultInserviceChannelFactory () { if (contractType == null) { DiagnosticUtility.DebugAssert("contractType should not be null"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } if (serviceEndpoint == null) { serviceEndpoint = CreateServiceEndpoint(); } foreach (IEndpointBehavior behavior in behaviors) serviceEndpoint.Behaviors.Add(behavior); serviceChannelFactory = CreateServiceChannelFactory (); } internal void ResolveTypeIfPossible (Dictionary propertyTable ) { string typeIID; propertyTable.TryGetValue (MonikerHelper.MonikerAttribute.Contract, out typeIID); Guid iid; if (!string.IsNullOrEmpty(typeIID)) { try { dispatchEnabled = true; iid = new Guid (typeIID); TypeCacheManager.Provider.FindOrCreateType (iid, out contractType, true, false); serviceEndpoint = CreateServiceEndpoint(); } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MonikerSyntaxException (SR.GetString(SR.TypeLoadForContractTypeIIDFailedWith, typeIID, e.Message))); } } } internal TypedServiceChannelBuilder (Dictionary propertyTable ) { string bindingType = null; string bindingConfigName = null; string spnIdentity = null; string upnIdentity = null; string dnsIdentity = null; propertyTable.TryGetValue (MonikerHelper.MonikerAttribute.Address, out address); propertyTable.TryGetValue (MonikerHelper.MonikerAttribute.Binding, out bindingType); propertyTable.TryGetValue (MonikerHelper.MonikerAttribute.BindingConfiguration, out bindingConfigName); propertyTable.TryGetValue (MonikerHelper.MonikerAttribute.SpnIdentity, out spnIdentity); propertyTable.TryGetValue (MonikerHelper.MonikerAttribute.UpnIdentity, out upnIdentity); propertyTable.TryGetValue (MonikerHelper.MonikerAttribute.DnsIdentity, out dnsIdentity); if (!string.IsNullOrEmpty(bindingType)) { try { binding = ConfigLoader.LookupBinding (bindingType, bindingConfigName); } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MonikerSyntaxException (SR.GetString(SR.BindingLoadFromConfigFailedWith, bindingType, e.Message))); } if (binding == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MonikerSyntaxException (SR.GetString(SR.BindingNotFoundInConfig, bindingType, bindingConfigName))); } if (binding == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MonikerSyntaxException(SR.GetString(SR.BindingNotSpecified))); if (string.IsNullOrEmpty(address)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MonikerSyntaxException(SR.GetString(SR.AddressNotSpecified))); if (!string.IsNullOrEmpty(spnIdentity )) { if ((!string.IsNullOrEmpty(upnIdentity))||(!string.IsNullOrEmpty(dnsIdentity))) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new MonikerSyntaxException (SR.GetString (SR.MonikerIncorrectServerIdentity))); identity = EndpointIdentity.CreateSpnIdentity(spnIdentity); } else if (!string.IsNullOrEmpty(upnIdentity)) { if ((!string.IsNullOrEmpty(spnIdentity))||(!string.IsNullOrEmpty(dnsIdentity))) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new MonikerSyntaxException (SR.GetString (SR.MonikerIncorrectServerIdentity))); identity = EndpointIdentity.CreateUpnIdentity(upnIdentity); } else if (!string.IsNullOrEmpty(dnsIdentity)) { if ((!string.IsNullOrEmpty(spnIdentity))||(!string.IsNullOrEmpty(upnIdentity))) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError (new MonikerSyntaxException (SR.GetString (SR.MonikerIncorrectServerIdentity))); identity = EndpointIdentity.CreateDnsIdentity(dnsIdentity); } else identity = null; ResolveTypeIfPossible (propertyTable); } bool dispatchEnabled = false; private bool CheckDispatch (ref Guid riid) { if ((dispatchEnabled) && (riid == InterfaceID.idIDispatch)) return true; else return false; } ComProxy IProxyCreator.CreateProxy (IntPtr outer, ref Guid riid) { if (outer == IntPtr.Zero) { DiagnosticUtility.DebugAssert("OuterProxy cannot be null"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } // No contract Fault on in if (contractType == null) TypeCacheManager.Provider.FindOrCreateType (riid, out contractType, true, false); if ((contractType.GUID != riid) && !(CheckDispatch (ref riid))) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidCastException(SR.GetString(SR.NoInterface, riid))); Type proxiedType = EmitterCache.TypeEmitter.FindOrCreateType (contractType); ComProxy comProxy = null; TearOffProxy tearoffProxy = null; try { tearoffProxy = new TearOffProxy (this , proxiedType); comProxy = ComProxy.Create (outer, tearoffProxy.GetTransparentProxy(), tearoffProxy); return comProxy; } finally { if ((comProxy == null) && (tearoffProxy != null)) ((IDisposable)tearoffProxy).Dispose (); } } bool IProxyCreator.SupportsErrorInfo (ref Guid riid) { if (contractType == null) return false; else { if ((contractType.GUID != riid) && !(CheckDispatch (ref riid))) return false; else return true; } } bool IProxyCreator.SupportsDispatch () { return dispatchEnabled; } bool IProxyCreator.SupportsIntrinsics () { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
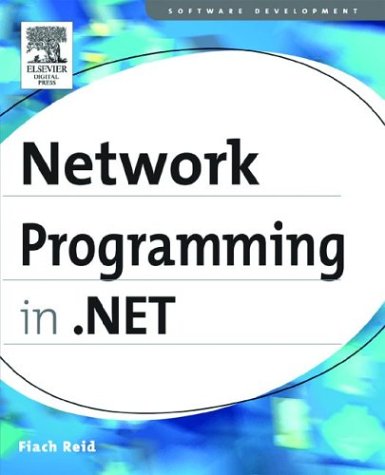
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TileBrush.cs
- OrderedDictionary.cs
- ProtectedConfigurationProviderCollection.cs
- XPathChildIterator.cs
- TextBreakpoint.cs
- ProfileInfo.cs
- FrameworkTextComposition.cs
- XmlDocumentType.cs
- Stopwatch.cs
- ParsedAttributeCollection.cs
- Style.cs
- TreeNodeCollection.cs
- XmlAnyElementAttribute.cs
- SetMemberBinder.cs
- DocumentPage.cs
- ActiveXSite.cs
- ToolStripControlHost.cs
- EventLogHandle.cs
- GridViewDeletedEventArgs.cs
- ServicePoint.cs
- LogRecordSequence.cs
- PermissionSetEnumerator.cs
- QilReference.cs
- ClientScriptItemCollection.cs
- TextFormatterHost.cs
- IncrementalCompileAnalyzer.cs
- MulticastNotSupportedException.cs
- PageHandlerFactory.cs
- SafeFileMappingHandle.cs
- VirtualDirectoryMapping.cs
- CommandEventArgs.cs
- SamlAuthenticationStatement.cs
- Int32CollectionConverter.cs
- ExtensionDataReader.cs
- Brush.cs
- ChannelManager.cs
- VirtualPathProvider.cs
- AttachInfo.cs
- NameValueCollection.cs
- PolygonHotSpot.cs
- DynamicPropertyHolder.cs
- EncoderBestFitFallback.cs
- SiteMapPath.cs
- HostingEnvironment.cs
- PathSegment.cs
- ObjectContext.cs
- SqlMetaData.cs
- ValueExpressions.cs
- DataGridColumn.cs
- WebBrowser.cs
- CacheDependency.cs
- TextParagraphCache.cs
- LayoutDump.cs
- Operator.cs
- WindowPattern.cs
- WebPartEditorOkVerb.cs
- TableRowsCollectionEditor.cs
- Transform3DGroup.cs
- MaskedTextProvider.cs
- DefaultTextStore.cs
- ReadOnlyTernaryTree.cs
- TableItemStyle.cs
- ListBox.cs
- CursorConverter.cs
- SupportsPreviewControlAttribute.cs
- Utility.cs
- PenContext.cs
- DataGridViewColumnEventArgs.cs
- QuestionEventArgs.cs
- URLAttribute.cs
- UshortList2.cs
- CommonObjectSecurity.cs
- TokenFactoryBase.cs
- SequentialUshortCollection.cs
- Int16AnimationBase.cs
- DataRowComparer.cs
- ConditionalWeakTable.cs
- XhtmlBasicPanelAdapter.cs
- Set.cs
- FlowNode.cs
- RemotingSurrogateSelector.cs
- ConsoleCancelEventArgs.cs
- DataGridViewColumnStateChangedEventArgs.cs
- EncryptedReference.cs
- X509AsymmetricSecurityKey.cs
- BamlLocalizableResource.cs
- Compiler.cs
- XamlPathDataSerializer.cs
- LambdaCompiler.ControlFlow.cs
- Util.cs
- WebBrowsableAttribute.cs
- LineSegment.cs
- Nodes.cs
- ListViewGroup.cs
- ServicePrincipalNameElement.cs
- CursorConverter.cs
- AuthenticationServiceManager.cs
- AnnotationResource.cs
- UniqueEventHelper.cs
- SpellerError.cs