Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / UnrecognizedAssertionsBindingElement.cs / 1 / UnrecognizedAssertionsBindingElement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Xml; using System.ServiceModel.Description; using System.Xml.Schema; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using WsdlNS = System.Web.Services.Description; using System.Text; using System.ComponentModel; using System.Diagnostics; internal class UnrecognizedAssertionsBindingElement : BindingElement { XmlQualifiedName wsdlBinding; ICollectionbindingAsserions; IDictionary > operationAssertions; IDictionary > messageAssertions; internal protected UnrecognizedAssertionsBindingElement(XmlQualifiedName wsdlBinding, ICollection bindingAsserions) { DiagnosticUtility.DebugAssert(wsdlBinding != null, ""); this.wsdlBinding = wsdlBinding; this.bindingAsserions = bindingAsserions; } internal XmlQualifiedName WsdlBinding { get { return this.wsdlBinding; } } internal ICollection BindingAsserions { get { if (this.bindingAsserions == null) this.bindingAsserions = new Collection (); return this.bindingAsserions; } } internal IDictionary > OperationAssertions { get { if (this.operationAssertions == null) this.operationAssertions = new Dictionary >(); return this.operationAssertions; } } internal IDictionary > MessageAssertions { get { if (this.messageAssertions == null) this.messageAssertions = new Dictionary >(); return this.messageAssertions; } } internal void Add(OperationDescription operation, ICollection assertions) { ICollection existent; if (!OperationAssertions.TryGetValue(operation, out existent)) { OperationAssertions.Add(operation, assertions); } else { foreach (XmlElement assertion in assertions) existent.Add(assertion); } } internal void Add(MessageDescription message, ICollection assertions) { ICollection existent; if (!MessageAssertions.TryGetValue(message, out existent)) { MessageAssertions.Add(message, assertions); } else { foreach (XmlElement assertion in assertions) existent.Add(assertion); } } protected UnrecognizedAssertionsBindingElement(UnrecognizedAssertionsBindingElement elementToBeCloned) : base(elementToBeCloned) { this.wsdlBinding = elementToBeCloned.wsdlBinding; this.bindingAsserions = elementToBeCloned.bindingAsserions; this.operationAssertions = elementToBeCloned.operationAssertions; this.messageAssertions = elementToBeCloned.messageAssertions; } public override T GetProperty (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } return context.GetInnerProperty (); } public override BindingElement Clone() { //throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.UnsupportedBindingElementClone, typeof(UnrecognizedAssertionsBindingElement).Name))); // do not allow Cloning, return an empty BindingElement return new UnrecognizedAssertionsBindingElement(new XmlQualifiedName(wsdlBinding.Name, wsdlBinding.Namespace), null); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
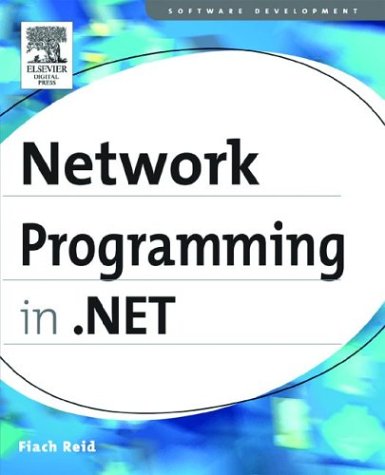
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StorageMappingItemCollection.cs
- UriWriter.cs
- DetailsViewUpdateEventArgs.cs
- ConfigXmlReader.cs
- Errors.cs
- Int32CollectionConverter.cs
- ConsoleTraceListener.cs
- PeerContact.cs
- StrongBox.cs
- Propagator.JoinPropagator.SubstitutingCloneVisitor.cs
- FunctionMappingTranslator.cs
- WebPartConnectVerb.cs
- DBCommandBuilder.cs
- FloatUtil.cs
- IndexedString.cs
- BinaryHeap.cs
- FunctionDetailsReader.cs
- XmlSignatureProperties.cs
- UserInitiatedRoutedEventPermission.cs
- GeometryCollection.cs
- ToolStripContentPanelDesigner.cs
- Socket.cs
- Comparer.cs
- BmpBitmapDecoder.cs
- CheckedListBox.cs
- DeclarationUpdate.cs
- QueryableDataSourceHelper.cs
- VirtualizingPanel.cs
- TextEditorThreadLocalStore.cs
- LayoutEvent.cs
- CopyCodeAction.cs
- InheritanceContextChangedEventManager.cs
- HttpVersion.cs
- CompletedAsyncResult.cs
- DataGridCellAutomationPeer.cs
- WmlObjectListAdapter.cs
- SafeSystemMetrics.cs
- UseLicense.cs
- XmlnsDefinitionAttribute.cs
- TransportContext.cs
- PreloadedPackages.cs
- Activity.cs
- WebBrowsableAttribute.cs
- GACMembershipCondition.cs
- MetadataArtifactLoaderResource.cs
- WebConfigurationManager.cs
- AccessKeyManager.cs
- ScriptControlDescriptor.cs
- SchemaMapping.cs
- ListBoxItemAutomationPeer.cs
- AuthorizationRule.cs
- SQLStringStorage.cs
- connectionpool.cs
- _ConnectStream.cs
- CharacterBufferReference.cs
- PageVisual.cs
- Substitution.cs
- ConfigXmlWhitespace.cs
- WindowsAltTab.cs
- Win32.cs
- ConfigurationLoaderException.cs
- XamlHostingSection.cs
- LinkDesigner.cs
- Command.cs
- TypedElement.cs
- HttpCapabilitiesEvaluator.cs
- Proxy.cs
- QfeChecker.cs
- ContextStaticAttribute.cs
- WebPartDisplayModeEventArgs.cs
- ReflectionUtil.cs
- NodeInfo.cs
- CatalogPartCollection.cs
- SpAudioStreamWrapper.cs
- JavaScriptObjectDeserializer.cs
- TemplateBuilder.cs
- XPathNodeInfoAtom.cs
- NotSupportedException.cs
- PrintPageEvent.cs
- InvalidOperationException.cs
- UriSectionData.cs
- CodeParameterDeclarationExpression.cs
- SqlCharStream.cs
- RectConverter.cs
- InputEventArgs.cs
- Boolean.cs
- ToolStripComboBox.cs
- RefreshEventArgs.cs
- AnonymousIdentificationSection.cs
- ImageFormatConverter.cs
- SecurityDescriptor.cs
- RecognizedWordUnit.cs
- KerberosRequestorSecurityToken.cs
- DbConnectionStringBuilder.cs
- WindowsListViewItem.cs
- CodeBinaryOperatorExpression.cs
- EventBuilder.cs
- CompModHelpers.cs
- DecimalAnimationBase.cs
- StringDictionary.cs