Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Printing / PrintPageEvent.cs / 1 / PrintPageEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; ////// /// // NOTE: Please keep this class consistent with PaintEventArgs. public class PrintPageEventArgs : EventArgs { private bool hasMorePages; private bool cancel; private Graphics graphics; private readonly Rectangle marginBounds; private readonly Rectangle pageBounds; private readonly PageSettings pageSettings; ///Provides data for the ////// event. /// /// public PrintPageEventArgs(Graphics graphics, Rectangle marginBounds, Rectangle pageBounds, PageSettings pageSettings) { this.graphics = graphics; // may be null, see PrintController this.marginBounds = marginBounds; this.pageBounds = pageBounds; this.pageSettings = pageSettings; } ///Initializes a new instance of the ///class. /// /// public bool Cancel { get { return cancel;} set { cancel = value;} } ///Gets or sets a value indicating whether the print job should be canceled. ////// /// public Graphics Graphics { get { return graphics; } } ////// Gets the ////// used to paint the /// item. /// /// /// public bool HasMorePages { get { return hasMorePages;} set { hasMorePages = value;} } ///Gets or sets a value indicating whether an additional page should /// be printed. ////// /// public Rectangle MarginBounds { get { return marginBounds; } } ///Gets the rectangular area that represents the portion of the page between the margins. ////// /// public Rectangle PageBounds { get { return pageBounds; } } ////// Gets the rectangular area that represents the total area of the page. /// ////// /// public PageSettings PageSettings { get { return pageSettings; } } ///Gets /// the page settings for the current page. ////// /// // We want a way to dispose the GDI+ Graphics, but we don't want to create one // simply to dispose it internal void Dispose() { graphics.Dispose(); } internal void SetGraphics(Graphics value) { this.graphics = value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Disposes /// of the resources (other than memory) used by /// the ///.
Link Menu
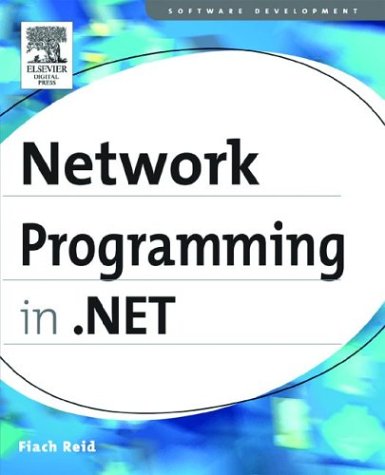
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditorAttribute.cs
- Contracts.cs
- Binding.cs
- UnknownBitmapDecoder.cs
- PeerTransportBindingElement.cs
- DoubleUtil.cs
- BitmapMetadataBlob.cs
- StreamGeometry.cs
- LinqExpressionNormalizer.cs
- TileModeValidation.cs
- RightNameExpirationInfoPair.cs
- Error.cs
- DefaultParameterValueAttribute.cs
- RelatedImageListAttribute.cs
- DataShape.cs
- BufferedOutputStream.cs
- TranslateTransform.cs
- InlineObject.cs
- ConsoleCancelEventArgs.cs
- PeerSecurityManager.cs
- XmlIlGenerator.cs
- Debugger.cs
- PEFileEvidenceFactory.cs
- ListParagraph.cs
- UInt32Converter.cs
- DataFieldEditor.cs
- ColumnMapCopier.cs
- AutoFocusStyle.xaml.cs
- ResolvedKeyFrameEntry.cs
- PageVisual.cs
- DataViewSettingCollection.cs
- EnumerableValidator.cs
- Identity.cs
- StorageAssociationSetMapping.cs
- ToolboxComponentsCreatingEventArgs.cs
- ExistsInCollection.cs
- DictionaryManager.cs
- ControlUtil.cs
- TextAction.cs
- XPathNode.cs
- Attributes.cs
- WebServiceBindingAttribute.cs
- HttpCacheParams.cs
- EmptyImpersonationContext.cs
- DropSource.cs
- IPPacketInformation.cs
- ConnectionManager.cs
- MultiView.cs
- FixedHyperLink.cs
- PersistNameAttribute.cs
- DataGridViewMethods.cs
- ContextMenu.cs
- DbDataAdapter.cs
- MetadataPropertyAttribute.cs
- SystemNetworkInterface.cs
- Constraint.cs
- XPathException.cs
- WindowsFormsSectionHandler.cs
- DbParameterHelper.cs
- ValidationError.cs
- File.cs
- XpsFontSubsetter.cs
- QueryOptionExpression.cs
- PrimitiveSchema.cs
- Debugger.cs
- ColumnResizeUndoUnit.cs
- EncoderReplacementFallback.cs
- EditingCoordinator.cs
- FontWeights.cs
- GridItem.cs
- WebPartDisplayModeCollection.cs
- InternalBase.cs
- Misc.cs
- CompatibleIComparer.cs
- RequestQueue.cs
- NativeMethods.cs
- RotateTransform.cs
- Point3D.cs
- TextLineBreak.cs
- ParallelTimeline.cs
- XmlIlVisitor.cs
- KeyTimeConverter.cs
- DragCompletedEventArgs.cs
- InkCanvasFeedbackAdorner.cs
- formatstringdialog.cs
- MethodSignatureGenerator.cs
- SessionStateUtil.cs
- TypeSystem.cs
- SimpleLine.cs
- Codec.cs
- ImageFormatConverter.cs
- DataListAutoFormat.cs
- InstanceDataCollection.cs
- TextFragmentEngine.cs
- AssemblyBuilder.cs
- Bitmap.cs
- Array.cs
- NonSerializedAttribute.cs
- TagMapCollection.cs
- Boolean.cs