Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Text / SimpleLine.cs / 1 / SimpleLine.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SimpleLine.cs // // Description: Text line formatter. // // History: // 09/10/2003 : grzegorz - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.Text { // --------------------------------------------------------------------- // Text line formatter. // --------------------------------------------------------------------- internal sealed class SimpleLine : Line { // ------------------------------------------------------------------ // // TextSource Implementation // // ----------------------------------------------------------------- #region TextSource Implementation // ------------------------------------------------------------------ // Get a text run at specified text source position. // ------------------------------------------------------------------ public override TextRun GetTextRun(int dcp) { Debug.Assert(dcp >= 0, "Character index must be non-negative."); TextRun run; // There is only one run of text. if (dcp < _content.Length) { // LineLayout may ask for dcp != 0. This case may only happen during partial // validation of TextRunCache. // Example: // 1) TextRunCache and LineMetrics array were created during measure process. // 2) Before OnRender is called somebody invalidates render only property. // This invalidates TextRunCache. // 3) Before OnRender is called InputHitTest is invoked. Because LineMetrics // array is valid, we don't have to recreate all lines. There is only // need to recreate the N-th line (line that has been hit). // During line recreation LineLayout will not refetch all runs from the // beginning of TextBlock control - it will ask for the run at the beginning // of the current line. // For this reason set 'offsetToFirstChar' to 'dcp' value. run = new TextCharacters(_content, dcp, _content.Length - dcp, _textProps); } else { run = new TextEndOfParagraph(_syntheticCharacterLength); } return run; } // ----------------------------------------------------------------- // Get text immediately before specified text source position. // ------------------------------------------------------------------ public override TextSpanGetPrecedingText(int dcp) { Debug.Assert(dcp >= 0, "Character index must be non-negative."); CharacterBufferRange charString = CharacterBufferRange.Empty; CultureInfo culture = null; if (dcp > 0) { charString = new CharacterBufferRange( _content, 0, Math.Min(dcp, _content.Length) ); culture = _textProps.CultureInfo; } return new TextSpan ( dcp, new CultureSpecificCharacterBufferRange(culture, charString) ); } /// /// TextFormatter to map a text source character index to a text effect character index /// /// text source character index ///the text effect index corresponding to the text effect character index public override int GetTextEffectCharacterIndexFromTextSourceCharacterIndex( int textSourceCharacterIndex ) { return textSourceCharacterIndex; } #endregion TextSource Implementation //------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods // ----------------------------------------------------------------- // Constructor. // // owner - owner of the line. // ------------------------------------------------------------------ internal SimpleLine(System.Windows.Controls.TextBlock owner, string content, TextRunProperties textProps) : base(owner) { Debug.Assert(content != null); _content = content; _textProps = textProps; } #endregion Internal Methods //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields // ------------------------------------------------------------------ // Content of the line. // ----------------------------------------------------------------- private readonly string _content; // ------------------------------------------------------------------ // Text properties. // ----------------------------------------------------------------- private readonly TextRunProperties _textProps; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SimpleLine.cs // // Description: Text line formatter. // // History: // 09/10/2003 : grzegorz - created. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.Windows; using System.Windows.Controls; using System.Windows.Media; using System.Windows.Media.TextFormatting; namespace MS.Internal.Text { // --------------------------------------------------------------------- // Text line formatter. // --------------------------------------------------------------------- internal sealed class SimpleLine : Line { // ------------------------------------------------------------------ // // TextSource Implementation // // ----------------------------------------------------------------- #region TextSource Implementation // ------------------------------------------------------------------ // Get a text run at specified text source position. // ------------------------------------------------------------------ public override TextRun GetTextRun(int dcp) { Debug.Assert(dcp >= 0, "Character index must be non-negative."); TextRun run; // There is only one run of text. if (dcp < _content.Length) { // LineLayout may ask for dcp != 0. This case may only happen during partial // validation of TextRunCache. // Example: // 1) TextRunCache and LineMetrics array were created during measure process. // 2) Before OnRender is called somebody invalidates render only property. // This invalidates TextRunCache. // 3) Before OnRender is called InputHitTest is invoked. Because LineMetrics // array is valid, we don't have to recreate all lines. There is only // need to recreate the N-th line (line that has been hit). // During line recreation LineLayout will not refetch all runs from the // beginning of TextBlock control - it will ask for the run at the beginning // of the current line. // For this reason set 'offsetToFirstChar' to 'dcp' value. run = new TextCharacters(_content, dcp, _content.Length - dcp, _textProps); } else { run = new TextEndOfParagraph(_syntheticCharacterLength); } return run; } // ----------------------------------------------------------------- // Get text immediately before specified text source position. // ------------------------------------------------------------------ public override TextSpanGetPrecedingText(int dcp) { Debug.Assert(dcp >= 0, "Character index must be non-negative."); CharacterBufferRange charString = CharacterBufferRange.Empty; CultureInfo culture = null; if (dcp > 0) { charString = new CharacterBufferRange( _content, 0, Math.Min(dcp, _content.Length) ); culture = _textProps.CultureInfo; } return new TextSpan ( dcp, new CultureSpecificCharacterBufferRange(culture, charString) ); } /// /// TextFormatter to map a text source character index to a text effect character index /// /// text source character index ///the text effect index corresponding to the text effect character index public override int GetTextEffectCharacterIndexFromTextSourceCharacterIndex( int textSourceCharacterIndex ) { return textSourceCharacterIndex; } #endregion TextSource Implementation //------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods // ----------------------------------------------------------------- // Constructor. // // owner - owner of the line. // ------------------------------------------------------------------ internal SimpleLine(System.Windows.Controls.TextBlock owner, string content, TextRunProperties textProps) : base(owner) { Debug.Assert(content != null); _content = content; _textProps = textProps; } #endregion Internal Methods //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields // ------------------------------------------------------------------ // Content of the line. // ----------------------------------------------------------------- private readonly string _content; // ------------------------------------------------------------------ // Text properties. // ----------------------------------------------------------------- private readonly TextRunProperties _textProps; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
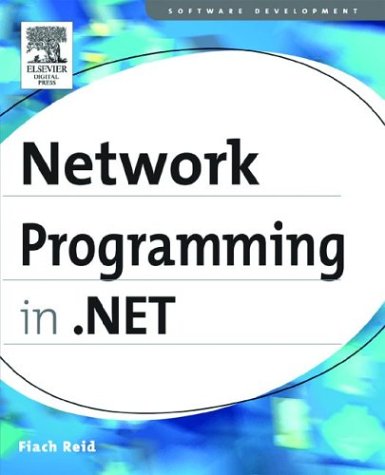
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThrowHelper.cs
- SchemaElement.cs
- PopupRootAutomationPeer.cs
- TableRow.cs
- WebBrowserBase.cs
- XpsPackagingPolicy.cs
- WebPartChrome.cs
- ClientSideQueueItem.cs
- FacetDescription.cs
- ExtensionWindowResizeGrip.cs
- IPCCacheManager.cs
- FunctionDescription.cs
- ImpersonateTokenRef.cs
- EventMappingSettingsCollection.cs
- CompilerTypeWithParams.cs
- DecoderFallbackWithFailureFlag.cs
- ArrayList.cs
- PopupRootAutomationPeer.cs
- LoginView.cs
- HtmlInputButton.cs
- FrameworkElement.cs
- TrustManager.cs
- FontWeightConverter.cs
- ExtendedProtectionPolicyTypeConverter.cs
- Color.cs
- XmlAnyElementAttribute.cs
- SettingsPropertyNotFoundException.cs
- HtmlInputText.cs
- WebPartZone.cs
- DataTableExtensions.cs
- RegisteredScript.cs
- BufferedReadStream.cs
- Binding.cs
- WebPartsPersonalization.cs
- MulticastNotSupportedException.cs
- SqlDataSourceView.cs
- StringAnimationUsingKeyFrames.cs
- SchemaHelper.cs
- DispatcherFrame.cs
- XPathDocumentBuilder.cs
- DataBinder.cs
- JsonSerializer.cs
- SizeFConverter.cs
- RegexCapture.cs
- AlternateView.cs
- XPathDescendantIterator.cs
- WorkflowViewManager.cs
- mediaeventargs.cs
- TemplateParser.cs
- FindSimilarActivitiesVerb.cs
- LinkArea.cs
- XhtmlTextWriter.cs
- DataList.cs
- ControlValuePropertyAttribute.cs
- MimeObjectFactory.cs
- AppearanceEditorPart.cs
- CompositionAdorner.cs
- RegisteredHiddenField.cs
- GeneratedCodeAttribute.cs
- NativeMethods.cs
- SqlReferenceCollection.cs
- SingleAnimationBase.cs
- SkewTransform.cs
- LinkButton.cs
- Int32Rect.cs
- IsolatedStorageException.cs
- XmlQualifiedNameTest.cs
- WebPartMenuStyle.cs
- ItemCollection.cs
- Delegate.cs
- Char.cs
- ScriptingAuthenticationServiceSection.cs
- ServiceMetadataExtension.cs
- EncoderBestFitFallback.cs
- columnmapkeybuilder.cs
- SchemaManager.cs
- HttpCapabilitiesBase.cs
- WizardDesigner.cs
- SamlSubjectStatement.cs
- TwoPhaseCommit.cs
- StrongNamePublicKeyBlob.cs
- ClientBuildManagerCallback.cs
- ListBase.cs
- Span.cs
- RelatedPropertyManager.cs
- LinqDataSourceDisposeEventArgs.cs
- ResourceManager.cs
- BitStack.cs
- MetadataSource.cs
- SourceItem.cs
- UnsafeNativeMethods.cs
- MaterializeFromAtom.cs
- ArrangedElementCollection.cs
- TypeName.cs
- NegotiationTokenAuthenticatorStateCache.cs
- Literal.cs
- DataSourceView.cs
- ImmutableObjectAttribute.cs
- InstanceHandleConflictException.cs
- RenderData.cs