Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / Proxy.cs / 1 / Proxy.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Implement generic send of datagram and request messages using System; using System.Collections.Generic; using System.Diagnostics; using System.Net; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.ServiceModel; using System.ServiceModel.Dispatcher; using System.ServiceModel.Channels; using System.Transactions; using System.Xml; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Messaging { abstract class Proxy : ReferenceCountedObject { protected CoordinationService coordinationService; protected MessageVersion messageVersion; protected EndpointAddress to; protected EndpointAddress from; protected ProtocolVersion protocolVersion; protected CoordinationStrings coordinationStrings; protected AtomicTransactionStrings atomicTransactionStrings; protected Proxy(CoordinationService coordination, EndpointAddress to, EndpointAddress from) { this.coordinationService = coordination; this.to = to; this.from = from; this.protocolVersion = coordination.ProtocolVersion; this.coordinationStrings = CoordinationStrings.Version(coordination.ProtocolVersion); this.atomicTransactionStrings = AtomicTransactionStrings.Version(coordination.ProtocolVersion); } public MessageVersion MessageVersion { get { return this.messageVersion; } } public EndpointAddress To { get { return this.to; } } public EndpointAddress From { get { return this.from; } set { this.from = value; } } public ProtocolVersion ProtocolVersion { get { return this.protocolVersion; } } } abstract class Proxy: Proxy where T : class { ReferenceCountedChannel referenceCountedChannel; protected bool interoperating = true; IChannelFactory cf; protected Proxy(CoordinationService coordination, EndpointAddress to, EndpointAddress from) : base(coordination, to, from) { if (DebugTrace.Verbose) { EndpointIdentity identity = this.to.Identity; DebugTrace.Trace(TraceLevel.Verbose, "Creating {0} for {1} at {2}", this.GetType().Name, identity == null ? "host" : identity.ToString(), this.to.Uri); } this.cf = SelectChannelFactory(out this.messageVersion); if (this.interoperating && this.to.Identity != null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new CreateChannelFailureException(SR.GetString(SR.InvalidTrustIdentity))); } try { this.referenceCountedChannel = ReferenceCountedChannel .GetChannel(this); } // EPRs that are malformed in some way or mismatch with their associated binding // may cause ArgumentException to be thrown. // An example from mid-Beta 2: providing a TrustIdentity to the HTTPS channel factory catch (ArgumentException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new CreateChannelFailureException(SR.GetString(SR.ProxyCreationFailed), e)); } // We may see this exception if we're low on resources. E.g. we could be out of sockets catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new CreateChannelFailureException(SR.GetString(SR.ProxyCreationFailed), e)); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace(TraceLevel.Error, "Unhandled exception {0} creating a proxy: {1}", e.GetType().Name, e); // The channel cache is in a bad state. // Or maybe CreateChannel threw a random, undocumented exception. // Either way, our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. DiagnosticUtility.InvokeFinalHandler(e); } // This value should be reasonably large in order to survive nested request/replies. // Depending on the topology of a transaction tree, a single Register message could // trigger a number of nested Register/RegisterResponse exchanges upstream. if (this.coordinationService.Config.OperationTimeout != TimeSpan.Zero) { IContextChannel contextChannel = (IContextChannel)this.referenceCountedChannel.Channel; contextChannel.OperationTimeout = this.coordinationService.Config.OperationTimeout; } } protected abstract IChannelFactory SelectChannelFactory(out MessageVersion MessageVersion); public abstract ChannelMruCache ChannelCache { get; } protected T GetChannel(Message message) { if (message != null) { this.to.ApplyTo(message); } return this.referenceCountedChannel.Channel; } protected void OnChannelFailure() { this.referenceCountedChannel.OnChannelFailure(); } public T CreateChannel(EndpointAddress ea) { return this.cf.CreateChannel(ea); } protected override void Close() { this.referenceCountedChannel.Release(); } } class ReferenceCountedChannel : ReferenceCountedObject where TChannel : class { Proxy proxy; TChannel channel; ChannelMruCacheKey key; ReferenceCountedChannel(Proxy proxy, TChannel channel, ChannelMruCacheKey key) { this.proxy = proxy; this.channel = channel; this.key = key; } public TChannel Channel { get { return channel; } } public static ReferenceCountedChannel GetChannel(Proxy proxy) { EndpointAddress ea = proxy.To; ChannelMruCacheKey key = new ChannelMruCacheKey(ea.Uri.AbsoluteUri, ea.Identity); ChannelMruCache channelCache = proxy.ChannelCache; ReferenceCountedChannel rcChannel; lock (channelCache) { if (channelCache.TryGetValue(key, out rcChannel)) { // AddRef inside the lock rcChannel.AddRef(); return rcChannel; } } // Create a channel outside the lock TChannel channel = proxy.CreateChannel(new EndpointAddress(ea.Uri, ea.Identity)); lock (channelCache) { if (!channelCache.TryGetValue(key, out rcChannel)) { rcChannel = new ReferenceCountedChannel (proxy, channel, key); channelCache.Add(key, rcChannel); } else { ((IChannel)channel).Close(); } // AddRef inside the lock rcChannel.AddRef(); } return rcChannel; } public void OnChannelFailure() { ChannelMruCache channelCache = this.proxy.ChannelCache; lock (channelCache) { channelCache.Remove(this.key); } } protected override void Close() { IChannel proxyChannel = (IChannel)channel; if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Closing {0}", proxyChannel.GetType().Name); } try { IAsyncResult result = proxyChannel.BeginClose(DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(OnCloseComplete)), proxyChannel); if (result.CompletedSynchronously) { CloseComplete(result); } } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); DebugTrace.Trace( TraceLevel.Warning, "Exception {0} closing a proxy: {1}", e.GetType().Name, e.Message ); } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); DebugTrace.Trace( TraceLevel.Warning, "Exception {0} closing a proxy: {1}", e.GetType().Name, e.Message ); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} closing a proxy: {1}", e.GetType().Name, e ); // Closing a channel threw a random, undocumented exception. // Our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. DiagnosticUtility.InvokeFinalHandler(e); } } void OnCloseComplete(IAsyncResult result) { if (!result.CompletedSynchronously) { CloseComplete(result); } } void CloseComplete(IAsyncResult result) { try { IChannel proxyChannel = (IChannel)result.AsyncState; proxyChannel.EndClose(result); if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Closed {0}", this.GetType().Name); } } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); DebugTrace.Trace( TraceLevel.Warning, "Exception {0} closing a proxy: {1}", e.GetType().Name, e.Message ); } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); DebugTrace.Trace( TraceLevel.Warning, "Exception {0} closing a proxy: {1}", e.GetType().Name, e.Message ); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} closing a proxy: {1}", e.GetType().Name, e ); // Closing a channel threw a random, undocumented exception. // Our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. DiagnosticUtility.InvokeFinalHandler(e); } } } class ChannelMruCacheKey : IEqualityComparer { string address; EndpointIdentity identity; public ChannelMruCacheKey(string address, EndpointIdentity identity) { this.address = address; this.identity = identity; } public bool Equals(ChannelMruCacheKey x, ChannelMruCacheKey y) { if (!x.address.Equals(y.address)) { return false; } return x.identity != null ? x.identity.Equals(y.identity) : y.identity == null; } public int GetHashCode(ChannelMruCacheKey obj) { int result = obj.address.GetHashCode(); if (obj.identity != null) { result ^= obj.identity.GetHashCode(); } return result; } } class ChannelMruCache : MruCache > where TItem : class { // We considered making the low/high watermarks used for the channel cache configurable, // but we decided not to do that for v1 of the product. // See MB 42922 for more details public ChannelMruCache() : base(40, 50, new ChannelMruCacheKey(null, null)) { } protected override void OnSingleItemRemoved(ReferenceCountedChannel referenceCountedObject) { referenceCountedObject.Release(); } } abstract class RequestReplyProxy : Proxy { protected RequestReplyProxy(CoordinationService coordination, EndpointAddress to) : base(coordination, to, null) { } public override ChannelMruCache ChannelCache { get { return this.coordinationService.RequestReplyChannelCache; } } public Message SendRequest(Message message, string replyAction) { Message reply; try { reply = GetChannel(message).SendRequest(message); } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } catch (QuotaExceededException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } catch (FaultException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatFaultException(e.CreateMessageFault(), e.Action)); } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} in RequestReplyProxy.SendRequest: {1}", e.GetType().Name, e ); OnChannelFailure(); // We tried to send a message, but our channel threw a random, undocumented exception. // Our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. DiagnosticUtility.InvokeFinalHandler(e); return null; // Keep the compiler happy } try { ValidateReply(reply, replyAction); } catch { reply.Close(); throw; } return reply; } protected IAsyncResult BeginSendRequest(Message message, AsyncCallback callback, object state) { AddRef(); try { return GetChannel(message).BeginRequest(message, callback, state); } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); return new SendMessageFailureAsyncResult(e, callback, state); } catch (QuotaExceededException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); return new SendMessageFailureAsyncResult(e, callback, state); } catch (FaultException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); return new SendMessageFailureAsyncResult(e, callback, state); } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); return new SendMessageFailureAsyncResult(e, callback, state); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} in RequestReplyProxy.BeginSendRequest: {1}", e.GetType().Name, e ); OnChannelFailure(); // We tried to send a message, but our channel threw a random, undocumented exception. // Our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. throw DiagnosticUtility.InvokeFinalHandler(e); } } protected Message EndSendRequest(IAsyncResult ar, string replyAction) { Message reply; try { SendMessageFailureAsyncResult synchronousFailure = ar as SendMessageFailureAsyncResult; if (synchronousFailure != null) { // This will always throw an exception synchronousFailure.End(); reply = null; // Keep the compiler happy } else { reply = GetChannel(null).EndRequest(ar); } } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } catch (QuotaExceededException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } catch (FaultException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatFaultException(e.CreateMessageFault(), e.Action)); } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); OnChannelFailure(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} in RequestReplyProxy.ReadReply: {1}", e.GetType().Name, e ); OnChannelFailure(); // We tried to send a message, but our channel threw a random, undocumented exception. // Our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. throw DiagnosticUtility.InvokeFinalHandler(e); } finally { // Balance the AddRef from BeginSendRequest Release(); } try { ValidateReply(reply, replyAction); } catch { reply.Close(); throw; } return reply; } void ValidateReply(Message reply, string replyAction) { if (this.interoperating) { // Apply manual correlation check for interop replies // We do this because composite duplex and transport security // do not provide automatic correlation of requests and replies if (!this.coordinationService.Security.CheckIdentity(this, reply)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new WsatReceiveFailureException(SR.GetString(SR.ReplyServerCredentialMismatch))); } // Apply manual access check against global ACL for interop replies // We do this because interop EPRs don't have trust identities // Therefore, we cannot check an interop EPR's membership in the global ACL // before sending the endpoint a message. if (!this.coordinationService.GlobalAcl.AccessCheckReply(reply, BindingStrings.InteropBindingQName(this.protocolVersion))) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new WsatReceiveFailureException(SR.GetString(SR.ReplyServerIdentityAccessDenied, this.to.Uri))); } } string action = reply.Headers.Action; if (reply.IsFault || action == this.atomicTransactionStrings.FaultAction || action == this.coordinationStrings.FaultAction) { MessageFault fault = MessageFault.CreateFault(reply, CoordinationBinding.MaxFaultSize); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatFaultException(fault, action)); } if (action != replyAction) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new WsatReceiveFailureException(SR.GetString(SR.InvalidMessageAction, action))); } } } abstract class DatagramProxy : Proxy { static EndpointAddress noneAddress = new EndpointAddress(EndpointAddress.NoneUri); protected DatagramProxy(CoordinationService coordination, EndpointAddress to, EndpointAddress from) : base(coordination, to, from) { } public override ChannelMruCache ChannelCache { get { return this.coordinationService.DatagramChannelCache; } } protected override IChannelFactory SelectChannelFactory(out MessageVersion messageVersion) { messageVersion = this.coordinationService.InteropDatagramBinding.MessageVersion; return this.coordinationService.InteropDatagramChannelFactory; } public IAsyncResult BeginSendMessage(Message message, AsyncCallback callback, object state) { // Set the datagram's reply to info if (this.from != null) { MessagingVersionHelper.SetReplyAddress(message, from, this.protocolVersion); } if (this.protocolVersion == ProtocolVersion.Version11) { // Section 8: Both terminal and non-terminal notification messages // MUST include a [reply endpoint] property whose [address] property is set to 'http://www.w3.org/2005/08/addressing/none� message.Headers.ReplyTo = noneAddress; } AddRef(); try { return GetChannel(message).BeginSend(message, callback, state); } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); return new SendMessageFailureAsyncResult(e, callback, state); } catch (QuotaExceededException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); return new SendMessageFailureAsyncResult(e, callback, state); } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); return new SendMessageFailureAsyncResult(e, callback, state); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} in DatagramProxy.BeginSendMessage: {1}", e.GetType().Name, e ); // We tried to send a message, but our channel threw a random, undocumented exception. // Our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. throw DiagnosticUtility.InvokeFinalHandler(e); } } public IAsyncResult BeginSendFault(UniqueId messageID, Fault fault, AsyncCallback callback, object state) { Message message = Library.CreateFaultMessage(messageID, this.messageVersion, fault); return BeginSendMessage(message, callback, state); } public void EndSendMessage(IAsyncResult ar) { try { SendMessageFailureAsyncResult datagram = ar as SendMessageFailureAsyncResult; if (datagram != null) { datagram.End(); } else { this.GetChannel(null).EndSend(ar); } } catch (TimeoutException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } catch (QuotaExceededException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } catch (CommunicationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatSendFailureException(e)); } #pragma warning suppress 56500 // Only catch Exception for InvokeFinalHandler catch (Exception e) { DebugTrace.Trace( TraceLevel.Error, "Unhandled exception {0} in DatagramProxy.EndSendMessage: {1}", e.GetType().Name, e ); // We tried to send a message, but our channel threw a random, undocumented exception. // Our underlying infrastructure is crumbling underneath us. // We cannot continue processing if we cannot trust our underlying comms layer. DiagnosticUtility.InvokeFinalHandler(e); } finally { // Balance the AddRef from BeginSendMessage Release(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
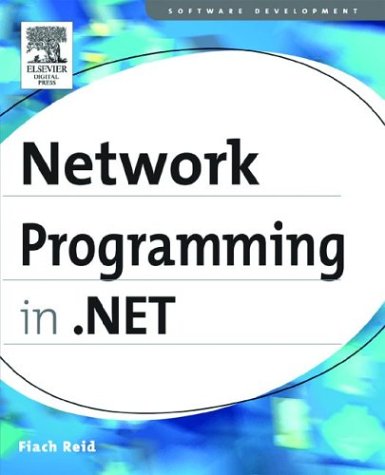
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Wildcard.cs
- TypeExtension.cs
- AssemblyInfo.cs
- XsltCompileContext.cs
- CodeDirectoryCompiler.cs
- FormatterServicesNoSerializableCheck.cs
- SoapIgnoreAttribute.cs
- BaseEntityWrapper.cs
- DynamicActivityProperty.cs
- CompressEmulationStream.cs
- FlowLayoutSettings.cs
- HorizontalAlignConverter.cs
- CompilerWrapper.cs
- TextHidden.cs
- sqlnorm.cs
- XamlSerializationHelper.cs
- ClaimComparer.cs
- GridViewRowPresenter.cs
- SortFieldComparer.cs
- DispatcherProcessingDisabled.cs
- DeclarativeConditionsCollection.cs
- ConfigsHelper.cs
- LayoutUtils.cs
- CssClassPropertyAttribute.cs
- MaterialGroup.cs
- CommandBinding.cs
- DescendantBaseQuery.cs
- ProjectionAnalyzer.cs
- RuleCache.cs
- PrivateFontCollection.cs
- SchemaObjectWriter.cs
- Queue.cs
- PathNode.cs
- XmlReaderSettings.cs
- SafePointer.cs
- AppliedDeviceFiltersDialog.cs
- TraceSwitch.cs
- VisualStyleTypesAndProperties.cs
- GridViewColumnHeaderAutomationPeer.cs
- BCLDebug.cs
- SerializableAttribute.cs
- ServiceHost.cs
- PropertiesTab.cs
- ItemChangedEventArgs.cs
- Line.cs
- Events.cs
- TextContainer.cs
- VisualStyleRenderer.cs
- _ConnectStream.cs
- TableCellAutomationPeer.cs
- EditorZoneDesigner.cs
- HttpListener.cs
- SafeNativeMethods.cs
- basemetadatamappingvisitor.cs
- _ConnectStream.cs
- SqlRetyper.cs
- CountdownEvent.cs
- FontUnitConverter.cs
- OleDbSchemaGuid.cs
- TextParagraphView.cs
- ProcessingInstructionAction.cs
- LogEntryHeaderDeserializer.cs
- NetNamedPipeBindingCollectionElement.cs
- CounterSetInstanceCounterDataSet.cs
- WmlFormAdapter.cs
- GenericIdentity.cs
- StatusStrip.cs
- DesignTimeParseData.cs
- AnimationException.cs
- PeerNameRecordCollection.cs
- SapiGrammar.cs
- IdentityReference.cs
- CryptoHelper.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- DataServiceKeyAttribute.cs
- PriorityQueue.cs
- ContractUtils.cs
- DataControlCommands.cs
- DrawingContext.cs
- ProviderException.cs
- KnownBoxes.cs
- TypeUtils.cs
- XmlSchemaSimpleContent.cs
- EmptyStringExpandableObjectConverter.cs
- Duration.cs
- CustomCategoryAttribute.cs
- _FtpControlStream.cs
- TraceRecords.cs
- DelegateBodyWriter.cs
- PersonalizationStateInfo.cs
- ProcessHostConfigUtils.cs
- TdsValueSetter.cs
- ICspAsymmetricAlgorithm.cs
- Misc.cs
- Range.cs
- OleTxTransactionInfo.cs
- ServiceHttpModule.cs
- MarkupCompilePass2.cs
- EmptyElement.cs
- UrlAuthFailedErrorFormatter.cs