Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / InternalDuplexChannelFactory.cs / 1 / InternalDuplexChannelFactory.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel.Dispatcher; using System.Runtime.Serialization; using System.Threading; sealed class InternalDuplexChannelFactory : LayeredChannelFactory{ static long channelCount = 0; InputChannelDemuxer channelDemuxer; IChannelFactory innerChannelFactory; IChannelListener innerChannelListener; LocalAddressProvider localAddressProvider; bool providesCorrelation; internal InternalDuplexChannelFactory(InternalDuplexBindingElement bindingElement, BindingContext context, InputChannelDemuxer channelDemuxer, IChannelFactory innerChannelFactory, LocalAddressProvider localAddressProvider) : base(context.Binding, innerChannelFactory) { this.channelDemuxer = channelDemuxer; this.innerChannelFactory = innerChannelFactory; ChannelDemuxerFilter demuxFilter = new ChannelDemuxerFilter(new MatchNoneMessageFilter(), int.MinValue); this.innerChannelListener = this.channelDemuxer.BuildChannelListener (demuxFilter); this.localAddressProvider = localAddressProvider; this.providesCorrelation = bindingElement.ProvidesCorrelation; } void CreateUniqueLocalAddress(out EndpointAddress address, out int priority) { long tempChannelCount = Interlocked.Increment(ref channelCount); if (tempChannelCount > 1) { AddressHeader uniqueEndpointHeader = AddressHeader.CreateAddressHeader(XD.UtilityDictionary.UniqueEndpointHeaderName, XD.UtilityDictionary.UniqueEndpointHeaderNamespace, tempChannelCount); address = new EndpointAddress(this.innerChannelListener.Uri, uniqueEndpointHeader); priority = 1; } else { address = new EndpointAddress(this.innerChannelListener.Uri); priority = 0; } } protected override IDuplexChannel OnCreateChannel(EndpointAddress address, Uri via) { EndpointAddress localAddress; int priority; MessageFilter filter; if (localAddressProvider != null) { localAddress = localAddressProvider.LocalAddress; filter = localAddressProvider.Filter; priority = localAddressProvider.Priority; } else { CreateUniqueLocalAddress(out localAddress, out priority); filter = new MatchAllMessageFilter(); } return this.CreateChannel(address, via, localAddress, filter, priority); } public IDuplexChannel CreateChannel(EndpointAddress address, Uri via, MessageFilter filter, int priority) { return this.CreateChannel(address, via, new EndpointAddress(this.innerChannelListener.Uri), filter, priority); } public IDuplexChannel CreateChannel(EndpointAddress remoteAddress, Uri via, EndpointAddress localAddress, MessageFilter filter, int priority) { ChannelDemuxerFilter demuxFilter = new ChannelDemuxerFilter(new AndMessageFilter(new EndpointAddressMessageFilter(localAddress, true), filter), priority); IDuplexChannel newChannel = null; IOutputChannel innerOutputChannel = null; IChannelListener innerInputListener = null; IInputChannel innerInputChannel = null; try { innerOutputChannel = this.innerChannelFactory.CreateChannel(remoteAddress, via); innerInputListener = this.channelDemuxer.BuildChannelListener (demuxFilter); innerInputListener.Open(); innerInputChannel = innerInputListener.AcceptChannel(); newChannel = new ClientCompositeDuplexChannel(this, innerInputChannel, innerInputListener, localAddress, innerOutputChannel); } finally { if (newChannel == null) // need to cleanup { if (innerOutputChannel != null) { innerOutputChannel.Close(); } if (innerInputListener != null) { innerInputListener.Close(); } if (innerInputChannel != null) { innerInputChannel.Close(); } } } return newChannel; } protected override void OnAbort() { base.OnAbort(); this.innerChannelListener.Abort(); } protected override void OnOpen(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnOpen(timeoutHelper.RemainingTime()); this.innerChannelListener.Open(timeoutHelper.RemainingTime()); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedOpenAsyncResult(timeout, callback, state, base.OnBeginOpen, base.OnEndOpen, this.innerChannelListener); } protected override void OnEndOpen(IAsyncResult result) { ChainedOpenAsyncResult.End(result); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnClose(timeoutHelper.RemainingTime()); this.innerChannelListener.Close(timeoutHelper.RemainingTime()); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedCloseAsyncResult(timeout, callback, state, base.OnBeginClose, base.OnEndClose, this.innerChannelListener); } protected override void OnEndClose(IAsyncResult result) { ChainedCloseAsyncResult.End(result); } public override T GetProperty () { if (typeof(T) == typeof(IChannelListener)) { return (T)(object)innerChannelListener; } if (typeof(T) == typeof(ISecurityCapabilities) && !this.providesCorrelation) { return InternalDuplexBindingElement.GetSecurityCapabilities (base.GetProperty ()); } T baseProperty = base.GetProperty (); if (baseProperty != null) { return baseProperty; } IChannelListener channelListener = innerChannelListener; if (channelListener != null) { return channelListener.GetProperty (); } else { return default(T); } } class ClientCompositeDuplexChannel : LayeredDuplexChannel { IChannelListener innerInputListener; public ClientCompositeDuplexChannel(ChannelManagerBase channelManager, IInputChannel innerInputChannel, IChannelListener innerInputListener, EndpointAddress localAddress, IOutputChannel innerOutputChannel) : base(channelManager, innerInputChannel, localAddress, innerOutputChannel) { this.innerInputListener = innerInputListener; } protected override void OnAbort() { base.OnAbort(); this.innerInputListener.Abort(); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedAsyncResult(timeout, callback, state, base.OnBeginClose, base.OnEndClose, this.innerInputListener.BeginClose, this.innerInputListener.EndClose); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnClose(timeoutHelper.RemainingTime()); this.innerInputListener.Close(timeoutHelper.RemainingTime()); } protected override void OnEndClose(IAsyncResult result) { ChainedAsyncResult.End(result); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
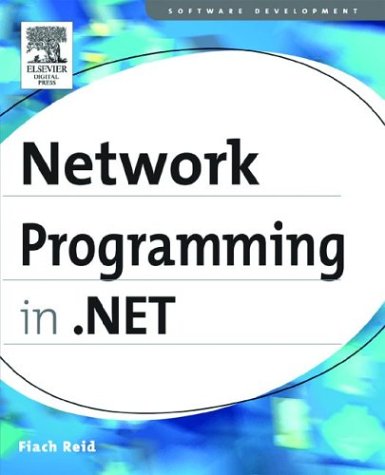
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeTypeReference.cs
- HtmlInputCheckBox.cs
- XmlSchemaSubstitutionGroup.cs
- SafeCoTaskMem.cs
- CanonicalFontFamilyReference.cs
- ServicePointManagerElement.cs
- MethodBuilder.cs
- StateBag.cs
- FrameworkElement.cs
- TargetParameterCountException.cs
- HexParser.cs
- DbInsertCommandTree.cs
- WebPartConnectionsConfigureVerb.cs
- CommandDevice.cs
- BigInt.cs
- SqlDuplicator.cs
- JavaScriptObjectDeserializer.cs
- CharEntityEncoderFallback.cs
- WizardPanel.cs
- safelinkcollection.cs
- CreateUserWizard.cs
- NavigationEventArgs.cs
- SafeTimerHandle.cs
- HttpResponseInternalBase.cs
- SystemGatewayIPAddressInformation.cs
- DataGridComboBoxColumn.cs
- StrokeCollection2.cs
- FixedSOMPage.cs
- PackagePart.cs
- RedistVersionInfo.cs
- TypeInfo.cs
- QueryInterceptorAttribute.cs
- OwnerDrawPropertyBag.cs
- FileFormatException.cs
- Buffer.cs
- AddressHeaderCollection.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- AvTraceDetails.cs
- ArrayEditor.cs
- OneOfTypeConst.cs
- CriticalExceptions.cs
- ValidatorUtils.cs
- TokenBasedSetEnumerator.cs
- CodeParameterDeclarationExpression.cs
- Clipboard.cs
- TextServicesCompartmentEventSink.cs
- Attribute.cs
- RuleSettings.cs
- KeySplineConverter.cs
- TableHeaderCell.cs
- ColorConvertedBitmap.cs
- WebPartVerbCollection.cs
- AddInAdapter.cs
- OrderedDictionaryStateHelper.cs
- mactripleDES.cs
- DecimalConstantAttribute.cs
- UrlMapping.cs
- RecordManager.cs
- StringUtil.cs
- DataViewManager.cs
- TrackingServices.cs
- storepermissionattribute.cs
- RolePrincipal.cs
- SpecularMaterial.cs
- XpsPackagingException.cs
- X509SecurityToken.cs
- FilterRepeater.cs
- PageHandlerFactory.cs
- AuthorizationSection.cs
- CircleHotSpot.cs
- EntitySqlQueryState.cs
- Transactions.cs
- Tablet.cs
- SqlStream.cs
- EmissiveMaterial.cs
- ReachSerializer.cs
- WebRequestModuleElementCollection.cs
- SecurityPermission.cs
- CachedFontFamily.cs
- MissingMemberException.cs
- ListControlConvertEventArgs.cs
- XmlElementCollection.cs
- ProviderSettings.cs
- WebPartChrome.cs
- DependentList.cs
- Brushes.cs
- ObjectToModelValueConverter.cs
- NodeFunctions.cs
- DBAsyncResult.cs
- CompositeCollectionView.cs
- FieldNameLookup.cs
- WebPartZoneDesigner.cs
- TableColumn.cs
- CodeArrayCreateExpression.cs
- HttpCacheParams.cs
- CollectionDataContract.cs
- LingerOption.cs
- DbSetClause.cs
- LinearQuaternionKeyFrame.cs
- CharUnicodeInfo.cs