Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / AccessControlEntry.cs / 1305376 / AccessControlEntry.cs
using System; using System.Collections; using System.Runtime.InteropServices; using System.ComponentModel; using System.Text; using System.Messaging.Interop; namespace System.Messaging { ////// /// public class AccessControlEntry { //const int customRightsMask = 0x0000ffff; const StandardAccessRights standardRightsMask = (StandardAccessRights)0x001f0000; const GenericAccessRights genericRightsMask = unchecked((GenericAccessRights)0xf0000000); internal int accessFlags = 0; Trustee trustee = null; AccessControlEntryType entryType = AccessControlEntryType.Allow; ///[To be supplied.] ////// /// public AccessControlEntry() { } ///[To be supplied.] ////// /// public AccessControlEntry(Trustee trustee) { this.Trustee = trustee; } ///[To be supplied.] ////// /// public AccessControlEntry(Trustee trustee, GenericAccessRights genericAccessRights, StandardAccessRights standardAccessRights, AccessControlEntryType entryType) { this.GenericAccessRights = genericAccessRights; this.StandardAccessRights = standardAccessRights; this.Trustee = trustee; this.EntryType = entryType; } ///[To be supplied.] ////// /// public AccessControlEntryType EntryType { get { return entryType; } set { if (!ValidationUtility.ValidateAccessControlEntryType(value)) throw new InvalidEnumArgumentException("value", (int)value, typeof(AccessControlEntryType)); entryType = value; } } ///[To be supplied.] ////// /// protected int CustomAccessRights { get { return accessFlags; } set { accessFlags = value; } } ///[To be supplied.] ////// /// public GenericAccessRights GenericAccessRights { get { return (GenericAccessRights)accessFlags & genericRightsMask; } set { // make sure these flags really are genericAccessRights if ((value & genericRightsMask) != value) throw new InvalidEnumArgumentException("value", (int)value, typeof(GenericAccessRights)); accessFlags = (accessFlags & (int)~genericRightsMask) | (int)value; } } ///[To be supplied.] ////// /// public StandardAccessRights StandardAccessRights { get { return (StandardAccessRights)accessFlags & standardRightsMask; } set { // make sure these flags really are standardAccessRights if ((value & standardRightsMask) != value) throw new InvalidEnumArgumentException("value", (int)value, typeof(StandardAccessRights)); accessFlags = (accessFlags & (int)~standardRightsMask) | (int)value; } } ///[To be supplied.] ////// /// public Trustee Trustee { get { return trustee; } set { if (value == null) throw new ArgumentNullException("value"); trustee = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Runtime.InteropServices; using System.ComponentModel; using System.Text; using System.Messaging.Interop; namespace System.Messaging { ///[To be supplied.] ////// /// public class AccessControlEntry { //const int customRightsMask = 0x0000ffff; const StandardAccessRights standardRightsMask = (StandardAccessRights)0x001f0000; const GenericAccessRights genericRightsMask = unchecked((GenericAccessRights)0xf0000000); internal int accessFlags = 0; Trustee trustee = null; AccessControlEntryType entryType = AccessControlEntryType.Allow; ///[To be supplied.] ////// /// public AccessControlEntry() { } ///[To be supplied.] ////// /// public AccessControlEntry(Trustee trustee) { this.Trustee = trustee; } ///[To be supplied.] ////// /// public AccessControlEntry(Trustee trustee, GenericAccessRights genericAccessRights, StandardAccessRights standardAccessRights, AccessControlEntryType entryType) { this.GenericAccessRights = genericAccessRights; this.StandardAccessRights = standardAccessRights; this.Trustee = trustee; this.EntryType = entryType; } ///[To be supplied.] ////// /// public AccessControlEntryType EntryType { get { return entryType; } set { if (!ValidationUtility.ValidateAccessControlEntryType(value)) throw new InvalidEnumArgumentException("value", (int)value, typeof(AccessControlEntryType)); entryType = value; } } ///[To be supplied.] ////// /// protected int CustomAccessRights { get { return accessFlags; } set { accessFlags = value; } } ///[To be supplied.] ////// /// public GenericAccessRights GenericAccessRights { get { return (GenericAccessRights)accessFlags & genericRightsMask; } set { // make sure these flags really are genericAccessRights if ((value & genericRightsMask) != value) throw new InvalidEnumArgumentException("value", (int)value, typeof(GenericAccessRights)); accessFlags = (accessFlags & (int)~genericRightsMask) | (int)value; } } ///[To be supplied.] ////// /// public StandardAccessRights StandardAccessRights { get { return (StandardAccessRights)accessFlags & standardRightsMask; } set { // make sure these flags really are standardAccessRights if ((value & standardRightsMask) != value) throw new InvalidEnumArgumentException("value", (int)value, typeof(StandardAccessRights)); accessFlags = (accessFlags & (int)~standardRightsMask) | (int)value; } } ///[To be supplied.] ////// /// public Trustee Trustee { get { return trustee; } set { if (value == null) throw new ArgumentNullException("value"); trustee = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
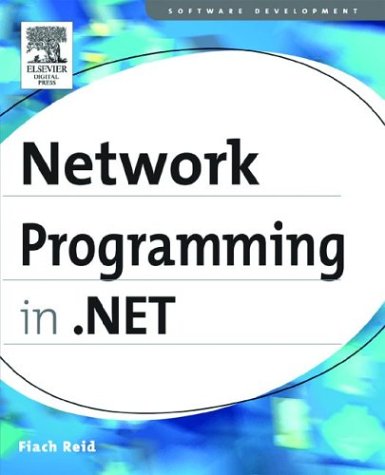
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DBConnection.cs
- PolygonHotSpot.cs
- SamlNameIdentifierClaimResource.cs
- WebPartDisplayModeCancelEventArgs.cs
- dtdvalidator.cs
- LoadedEvent.cs
- TreeNodeClickEventArgs.cs
- RemotingSurrogateSelector.cs
- NativeMethods.cs
- TimerElapsedEvenArgs.cs
- HttpException.cs
- BrushValueSerializer.cs
- UIElement.cs
- CustomLineCap.cs
- TraceSource.cs
- FieldBuilder.cs
- TabControlEvent.cs
- ParseChildrenAsPropertiesAttribute.cs
- SmiRequestExecutor.cs
- XmlLoader.cs
- UDPClient.cs
- GlyphRunDrawing.cs
- XmlConvert.cs
- Query.cs
- StorageTypeMapping.cs
- MonitoringDescriptionAttribute.cs
- Claim.cs
- IncrementalReadDecoders.cs
- ManualResetEvent.cs
- OutOfProcStateClientManager.cs
- DiscoveryReferences.cs
- ConfigurationSectionGroupCollection.cs
- DataServiceQueryProvider.cs
- ScriptingProfileServiceSection.cs
- SystemTcpConnection.cs
- FormsAuthenticationUserCollection.cs
- Padding.cs
- HttpStaticObjectsCollectionBase.cs
- PixelFormats.cs
- CubicEase.cs
- ElementAction.cs
- LookupBindingPropertiesAttribute.cs
- ControlParameter.cs
- CompressedStack.cs
- NetworkInformationPermission.cs
- UInt64Converter.cs
- ObjectReaderCompiler.cs
- CollectionViewGroup.cs
- WebMessageFormatHelper.cs
- Point3DAnimationUsingKeyFrames.cs
- AssemblyBuilderData.cs
- ExecutionEngineException.cs
- GeometryHitTestParameters.cs
- PropertyIDSet.cs
- TraceContext.cs
- GroupPartitionExpr.cs
- ZipPackage.cs
- CheckPair.cs
- TypeInfo.cs
- ImageList.cs
- SqlReferenceCollection.cs
- TypeConverterAttribute.cs
- SecureUICommand.cs
- OdbcHandle.cs
- ScalarRestriction.cs
- ThicknessAnimationUsingKeyFrames.cs
- ReadOnlyAttribute.cs
- _emptywebproxy.cs
- XmlNavigatorFilter.cs
- ChangeNode.cs
- DbUpdateCommandTree.cs
- SplitterCancelEvent.cs
- Int32KeyFrameCollection.cs
- SineEase.cs
- CommandField.cs
- PerformanceCounterManager.cs
- LinkClickEvent.cs
- SolidBrush.cs
- BasicAsyncResult.cs
- ScaleTransform.cs
- WbemException.cs
- IImplicitResourceProvider.cs
- VerificationException.cs
- WebPartsSection.cs
- ClientRoleProvider.cs
- NamedPermissionSet.cs
- odbcmetadatacollectionnames.cs
- UInt64.cs
- RefExpr.cs
- ErrorHandlerFaultInfo.cs
- recordstatescratchpad.cs
- IItemProperties.cs
- RemoteHelper.cs
- CompensationDesigner.cs
- DBCSCodePageEncoding.cs
- BitmapEffectInputConnector.cs
- TransformValueSerializer.cs
- ToolStripSeparator.cs
- HandleCollector.cs
- PerformanceCounterPermissionEntry.cs