Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ChangeToolStripParentVerb.cs / 1 / ChangeToolStripParentVerb.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Design; using Accessibility; using System.ComponentModel; using System.Diagnostics; using System; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel.Design; using System.Windows.Forms; using System.Runtime.Serialization; using System.ComponentModel.Design.Serialization; using System.Drawing; using System.Windows.Forms.Design.Behavior; using System.Drawing.Design; using System.Diagnostics.CodeAnalysis; ////// Internal class to provide 'Embed in ToolStripContainer" verb for ToolStrips & MenuStrips. /// internal class ChangeToolStripParentVerb { private ToolStripDesigner _designer; private IDesignerHost _host; private IComponentChangeService componentChangeSvc; private IServiceProvider _provider; ////// Create one of these things... /// internal ChangeToolStripParentVerb(string text, ToolStripDesigner designer) { Debug.Assert(designer != null, "Can't have a StandardMenuStripVerb without an associated designer"); this._designer = designer; this._provider = designer.Component.Site; this._host = (IDesignerHost)_provider.GetService(typeof(IDesignerHost)); componentChangeSvc = (IComponentChangeService)_provider.GetService(typeof(IComponentChangeService)); } ////// When the verb is invoked, change the parent of the ToolStrip. /// [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes")] [SuppressMessage("Microsoft.Security", "CA2102:CatchNonClsCompliantExceptionsInGeneralHandlers")] // This is actually called... [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public void ChangeParent() { Cursor current = Cursor.Current; // create a transaction so this happens as an atomic unit. DesignerTransaction changeParent = _host.CreateTransaction("Add ToolStripContainer Transaction"); try { Cursor.Current = Cursors.WaitCursor; //Add a New ToolStripContainer to the RootComponent ... Control root = _host.RootComponent as Control; ParentControlDesigner rootDesigner = _host.GetDesigner(root) as ParentControlDesigner; if (rootDesigner != null) { // close the DAP first - this is so that the autoshown panel on drag drop here is not conflicting with the currently opened panel // if the verb was called from the panel ToolStrip toolStrip = _designer.Component as ToolStrip; if(toolStrip != null && _designer != null && _designer.Component != null && _provider != null) { DesignerActionUIService dapuisvc = _provider.GetService(typeof(DesignerActionUIService)) as DesignerActionUIService; dapuisvc.HideUI(toolStrip); } // Get OleDragHandler ... ToolboxItem tbi = new ToolboxItem(typeof(System.Windows.Forms.ToolStripContainer)); OleDragDropHandler ddh = rootDesigner.GetOleDragHandler(); if (ddh != null) { IComponent[] newComp = ddh.CreateTool(tbi, root, 0, 0, 0, 0, false, false); ToolStripContainer tsc = newComp[0] as ToolStripContainer; if (tsc != null) { if(toolStrip != null) { IComponentChangeService changeSvc = _provider.GetService(typeof(IComponentChangeService)) as IComponentChangeService; Control newParent = GetParent(tsc, toolStrip); PropertyDescriptor controlsProp = TypeDescriptor.GetProperties(newParent)["Controls"]; Control oldParent = toolStrip.Parent; if (oldParent != null) { changeSvc.OnComponentChanging(oldParent, controlsProp); //remove control from the old parent oldParent.Controls.Remove(toolStrip); } if (newParent != null) { changeSvc.OnComponentChanging(newParent, controlsProp); //finally add & relocate the control with the new parent newParent.Controls.Add(toolStrip); } //fire our comp changed events if (changeSvc != null && oldParent != null && newParent != null) { changeSvc.OnComponentChanged(oldParent, controlsProp, null, null); changeSvc.OnComponentChanged(newParent, controlsProp, null, null); } //Set the Selection on the new Parent ... so that the selection is restored to the new item, ISelectionService selSvc = _provider.GetService(typeof(ISelectionService)) as ISelectionService; if (selSvc != null) { selSvc.SetSelectedComponents(new IComponent[] { tsc }); } } } } } } catch (Exception e){ if (e is System.InvalidOperationException) { IUIService uiService = (IUIService)_provider.GetService(typeof(IUIService)); uiService.ShowError(e.Message); } if (changeParent != null) { changeParent.Cancel(); changeParent = null; } } finally { if (changeParent != null) { changeParent.Commit(); changeParent = null; } Cursor.Current = current; } } private Control GetParent(ToolStripContainer container, Control c) { Control newParent = container.ContentPanel; DockStyle dock = c.Dock; if (c.Parent is ToolStripPanel) { dock = c.Parent.Dock; } foreach(Control panel in container.Controls) { if (panel is ToolStripPanel) { if (panel.Dock == dock) { newParent = panel; break; } } } return newParent; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
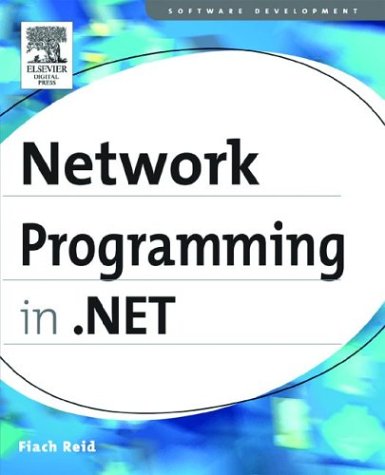
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompositeDataBoundControl.cs
- WebPartEditVerb.cs
- DataSourceComponent.cs
- CaseStatementProjectedSlot.cs
- NameTable.cs
- CapiSymmetricAlgorithm.cs
- SqlUtils.cs
- HtmlInputButton.cs
- ListCollectionView.cs
- ChameleonKey.cs
- DrawingBrush.cs
- XmlConvert.cs
- MailMessageEventArgs.cs
- SharedDp.cs
- Timeline.cs
- IPEndPointCollection.cs
- XPathNavigator.cs
- WebPartDescription.cs
- FlagsAttribute.cs
- QueryCacheEntry.cs
- FragmentQuery.cs
- SQLDecimalStorage.cs
- CorrelationManager.cs
- Transform.cs
- CodeGeneratorOptions.cs
- LongCountAggregationOperator.cs
- Graph.cs
- OutputWindow.cs
- HandlerFactoryWrapper.cs
- TrackingProfile.cs
- AuthenticationConfig.cs
- PanelStyle.cs
- KeyBinding.cs
- KeyedHashAlgorithm.cs
- XPathMessageFilterElement.cs
- mda.cs
- CustomErrorsSection.cs
- WebPartActionVerb.cs
- HttpListenerContext.cs
- WindowsClientElement.cs
- XPathAncestorQuery.cs
- PageTheme.cs
- LicenseProviderAttribute.cs
- SecurityAlgorithmSuiteConverter.cs
- OleDbEnumerator.cs
- BitmapCodecInfo.cs
- DependencyPropertyAttribute.cs
- Compilation.cs
- PropertyCondition.cs
- XmlAttributes.cs
- Source.cs
- SoapEnumAttribute.cs
- WebPartEventArgs.cs
- DataControlFieldTypeEditor.cs
- AttributeEmitter.cs
- XmlHelper.cs
- StringArrayEditor.cs
- HashCodeCombiner.cs
- WebPartEditorApplyVerb.cs
- DictionaryMarkupSerializer.cs
- basecomparevalidator.cs
- serverconfig.cs
- MenuItemCollectionEditorDialog.cs
- OleDbPropertySetGuid.cs
- smtppermission.cs
- AutomationAttributeInfo.cs
- DataGridViewDesigner.cs
- KeyValuePair.cs
- DrawingImage.cs
- IdnMapping.cs
- XmlQualifiedName.cs
- MexTcpBindingCollectionElement.cs
- SupportingTokenChannel.cs
- CollectionViewProxy.cs
- keycontainerpermission.cs
- SoapReflectionImporter.cs
- KerberosSecurityTokenProvider.cs
- WindowsListViewGroupHelper.cs
- SessionPageStateSection.cs
- WebConfigurationHostFileChange.cs
- MaskedTextBox.cs
- DataRowChangeEvent.cs
- DragCompletedEventArgs.cs
- TextContainerChangedEventArgs.cs
- FontInfo.cs
- ConnectionStringsSection.cs
- AuthStoreRoleProvider.cs
- NegotiateStream.cs
- Queue.cs
- SchemaElement.cs
- EntitySetDataBindingList.cs
- RsaElement.cs
- DecodeHelper.cs
- WebConfigurationHostFileChange.cs
- WindowsIdentity.cs
- Query.cs
- EditorPartDesigner.cs
- IISUnsafeMethods.cs
- ScrollableControl.cs
- AbstractSvcMapFileLoader.cs