Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / OwnerDrawPropertyBag.cs / 1 / OwnerDrawPropertyBag.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms; using Microsoft.Win32; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// /// /// Class used to pass new font/color information around for "partial" ownerdraw list/treeview items. /// ///// [SuppressMessage("Microsoft.Usage", "CA2240:ImplementISerializableCorrectly")] [Serializable] public class OwnerDrawPropertyBag : MarshalByRefObject, ISerializable { Font font = null; Color foreColor = Color.Empty; Color backColor = Color.Empty; Control.FontHandleWrapper fontWrapper = null; private static object internalSyncObject = new object(); /** * Constructor used in deserialization * Has to be protected because OwnerDrawPropertyBag is not sealed. FxCop Rule CA2229. */ protected OwnerDrawPropertyBag(SerializationInfo info, StreamingContext context) { foreach (SerializationEntry entry in info) { if (entry.Name == "Font") { // SEC font = (Font) entry.Value; } else if (entry.Name =="ForeColor") { // SEC foreColor =(Color)entry.Value; } else if (entry.Name =="BackColor") { // SEC backColor = (Color)entry.Value; } } } internal OwnerDrawPropertyBag(){ } /// /// /// public Font Font { get { return font; } set { font = value; } } ///[To be supplied.] ////// /// public Color ForeColor { get { return foreColor; } set { foreColor = value; } } ///[To be supplied.] ////// /// public Color BackColor { get { return backColor; } set { backColor = value; } } internal IntPtr FontHandle { get { if (fontWrapper == null) { fontWrapper = new Control.FontHandleWrapper(Font); } return fontWrapper.Handle; } } ///[To be supplied.] ////// /// Returns whether or not this property bag contains all default values (is empty) /// public virtual bool IsEmpty() { return (Font == null && foreColor.IsEmpty && backColor.IsEmpty); } ////// /// Copies the bag. Always returns a valid ODPB object /// public static OwnerDrawPropertyBag Copy(OwnerDrawPropertyBag value) { lock(internalSyncObject) { OwnerDrawPropertyBag ret = new OwnerDrawPropertyBag(); if (value == null) return ret; ret.backColor = value.backColor; ret.foreColor = value.foreColor; ret.Font = value.font; return ret; } } ////// /// ISerializable private implementation /// ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] void ISerializable.GetObjectData(SerializationInfo si, StreamingContext context) { si.AddValue("BackColor", BackColor); si.AddValue("ForeColor", ForeColor); si.AddValue("Font", Font); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms; using Microsoft.Win32; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// /// /// Class used to pass new font/color information around for "partial" ownerdraw list/treeview items. /// ///// [SuppressMessage("Microsoft.Usage", "CA2240:ImplementISerializableCorrectly")] [Serializable] public class OwnerDrawPropertyBag : MarshalByRefObject, ISerializable { Font font = null; Color foreColor = Color.Empty; Color backColor = Color.Empty; Control.FontHandleWrapper fontWrapper = null; private static object internalSyncObject = new object(); /** * Constructor used in deserialization * Has to be protected because OwnerDrawPropertyBag is not sealed. FxCop Rule CA2229. */ protected OwnerDrawPropertyBag(SerializationInfo info, StreamingContext context) { foreach (SerializationEntry entry in info) { if (entry.Name == "Font") { // SEC font = (Font) entry.Value; } else if (entry.Name =="ForeColor") { // SEC foreColor =(Color)entry.Value; } else if (entry.Name =="BackColor") { // SEC backColor = (Color)entry.Value; } } } internal OwnerDrawPropertyBag(){ } /// /// /// public Font Font { get { return font; } set { font = value; } } ///[To be supplied.] ////// /// public Color ForeColor { get { return foreColor; } set { foreColor = value; } } ///[To be supplied.] ////// /// public Color BackColor { get { return backColor; } set { backColor = value; } } internal IntPtr FontHandle { get { if (fontWrapper == null) { fontWrapper = new Control.FontHandleWrapper(Font); } return fontWrapper.Handle; } } ///[To be supplied.] ////// /// Returns whether or not this property bag contains all default values (is empty) /// public virtual bool IsEmpty() { return (Font == null && foreColor.IsEmpty && backColor.IsEmpty); } ////// /// Copies the bag. Always returns a valid ODPB object /// public static OwnerDrawPropertyBag Copy(OwnerDrawPropertyBag value) { lock(internalSyncObject) { OwnerDrawPropertyBag ret = new OwnerDrawPropertyBag(); if (value == null) return ret; ret.backColor = value.backColor; ret.foreColor = value.foreColor; ret.Font = value.font; return ret; } } ////// /// ISerializable private implementation /// ///[SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] void ISerializable.GetObjectData(SerializationInfo si, StreamingContext context) { si.AddValue("BackColor", BackColor); si.AddValue("ForeColor", ForeColor); si.AddValue("Font", Font); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
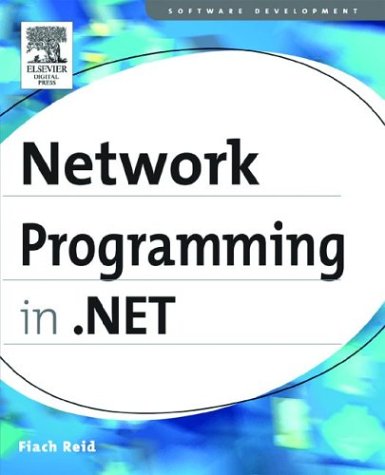
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeTypeDeclaration.cs
- EntityDataSourceValidationException.cs
- CommandLibraryHelper.cs
- Drawing.cs
- EventProviderBase.cs
- SetterBaseCollection.cs
- SelectionItemPattern.cs
- Utility.cs
- HttpContextBase.cs
- HttpCookie.cs
- ServiceOperationWrapper.cs
- SafeHandles.cs
- SchemaLookupTable.cs
- ListViewTableRow.cs
- PipelineModuleStepContainer.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- ToolStripItemRenderEventArgs.cs
- HttpCapabilitiesBase.cs
- WindowsListViewItem.cs
- TransactionBridgeSection.cs
- NegotiateStream.cs
- MultipleViewProviderWrapper.cs
- BamlResourceContent.cs
- MethodToken.cs
- DetailsViewCommandEventArgs.cs
- HtmlCalendarAdapter.cs
- GPStream.cs
- SiteOfOriginContainer.cs
- Validator.cs
- WebPartTracker.cs
- CallSiteHelpers.cs
- CfgParser.cs
- HtmlTableCell.cs
- PowerModeChangedEventArgs.cs
- RegexGroupCollection.cs
- AssemblyBuilder.cs
- TreeNodeEventArgs.cs
- RulePatternOps.cs
- ListDictionaryInternal.cs
- PtsContext.cs
- BlurBitmapEffect.cs
- KeyboardDevice.cs
- ActiveXMessageFormatter.cs
- CompositeFontParser.cs
- CaretElement.cs
- ActionNotSupportedException.cs
- CodeDirectoryCompiler.cs
- ObjectToIdCache.cs
- EnglishPluralizationService.cs
- DataGridViewComboBoxColumnDesigner.cs
- MobileControlPersister.cs
- RowCache.cs
- StrokeIntersection.cs
- _Events.cs
- SubMenuStyleCollection.cs
- KnowledgeBase.cs
- DetailsView.cs
- ClockController.cs
- Model3DGroup.cs
- PropertySet.cs
- RuleElement.cs
- BufferedStream.cs
- ComponentResourceKeyConverter.cs
- DbInsertCommandTree.cs
- PointLight.cs
- DesignTimeType.cs
- DataGridViewSelectedCellCollection.cs
- AlignmentXValidation.cs
- DataGridViewCellValueEventArgs.cs
- CancelAsyncOperationRequest.cs
- XmlILOptimizerVisitor.cs
- PartialCachingAttribute.cs
- UpDownBase.cs
- BulletChrome.cs
- PrintPreviewDialog.cs
- ParamArrayAttribute.cs
- RelationHandler.cs
- CertificateReferenceElement.cs
- TextTreeInsertUndoUnit.cs
- RootAction.cs
- Polygon.cs
- TimelineClockCollection.cs
- COM2EnumConverter.cs
- TextLineResult.cs
- UTF32Encoding.cs
- Package.cs
- SqlServer2KCompatibilityCheck.cs
- ToolStripOverflow.cs
- MimeTypeMapper.cs
- ContentType.cs
- SessionStateItemCollection.cs
- UpdateProgress.cs
- InvalidFilterCriteriaException.cs
- DBSqlParserColumnCollection.cs
- MasterPageBuildProvider.cs
- DependencyPropertyDescriptor.cs
- XmlSortKeyAccumulator.cs
- __ConsoleStream.cs
- Polyline.cs
- WebPartConnectVerb.cs