Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / SelfIssuedAuthRSAPKCS1SignatureFormatter.cs / 1 / SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // namespace Microsoft.InfoCards { using System; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.ServiceModel; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using System.Runtime.InteropServices; using System.Security.Cryptography; using System.IdentityModel; using System.Security.Cryptography.Xml; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Collections.ObjectModel; using System.Collections.Generic; // // Summary: // This class implements the mechanism to call SelfIssuedAuthRSACryptoProvider's SignHash // internal class SelfIssuedAuthRSAPKCS1SignatureFormatter : RSAPKCS1SignatureFormatter { private RSA m_rsaKey; private string m_strOID; // // public constructors // public SelfIssuedAuthRSAPKCS1SignatureFormatter() : base() { } public SelfIssuedAuthRSAPKCS1SignatureFormatter( AsymmetricAlgorithm key ) : base( key ) { m_rsaKey = ( RSA )key; } // // public methods // public override void SetKey( AsymmetricAlgorithm key ) { base.SetKey( key ); m_rsaKey = ( RSA )key; } public override void SetHashAlgorithm( string strName ) { base.SetHashAlgorithm( strName ); m_strOID = CryptoConfig.MapNameToOID( strName ); } public override byte[ ] CreateSignature( byte[ ] rgbHash ) { if ( !( null == m_strOID || null == m_rsaKey || null == rgbHash ) && m_rsaKey is SelfIssuedAuthRSACryptoProvider ) { return ( ( SelfIssuedAuthRSACryptoProvider )m_rsaKey ).SignHash( rgbHash, m_strOID ); } else { return base.CreateSignature( rgbHash ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
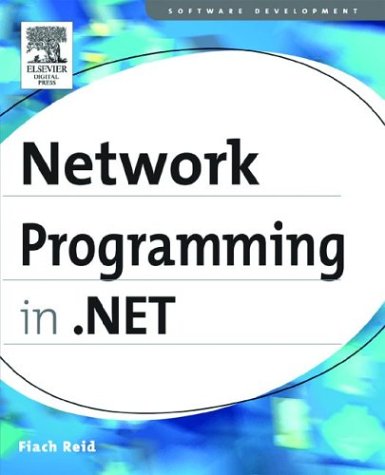
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlNullResolver.cs
- StaticSiteMapProvider.cs
- OrthographicCamera.cs
- PropertyEmitter.cs
- StreamSecurityUpgradeInitiatorAsyncResult.cs
- CollectionViewGroup.cs
- TextTrailingWordEllipsis.cs
- HostedHttpContext.cs
- InputLangChangeRequestEvent.cs
- DummyDataSource.cs
- Encoder.cs
- BatchParser.cs
- BackStopAuthenticationModule.cs
- SelectionManager.cs
- RequestResizeEvent.cs
- XPathMultyIterator.cs
- UpdateExpressionVisitor.cs
- DayRenderEvent.cs
- DataGridViewMethods.cs
- ValidatorUtils.cs
- RadioButtonBaseAdapter.cs
- Vector.cs
- InstanceDataCollectionCollection.cs
- DBParameter.cs
- FacetDescriptionElement.cs
- ProgressBarAutomationPeer.cs
- MeshGeometry3D.cs
- SymmetricKeyWrap.cs
- SettingsProviderCollection.cs
- Directory.cs
- MenuScrollingVisibilityConverter.cs
- Tokenizer.cs
- OdbcFactory.cs
- MsmqIntegrationSecurityElement.cs
- XmlDomTextWriter.cs
- RecognizerBase.cs
- UriScheme.cs
- PropertyDescriptorGridEntry.cs
- EnvironmentPermission.cs
- DataGridViewCellEventArgs.cs
- ScriptServiceAttribute.cs
- GridViewRowCollection.cs
- InputLangChangeEvent.cs
- StyleTypedPropertyAttribute.cs
- DesignerActionUIStateChangeEventArgs.cs
- RootBuilder.cs
- SQLCharsStorage.cs
- HtmlValidationSummaryAdapter.cs
- IncrementalReadDecoders.cs
- StylusCollection.cs
- MemberInfoSerializationHolder.cs
- UrlMapping.cs
- TransformerInfoCollection.cs
- Propagator.Evaluator.cs
- CategoryEditor.cs
- Menu.cs
- CodeObjectCreateExpression.cs
- OleDbCommand.cs
- RelationshipManager.cs
- CurrentChangedEventManager.cs
- XmlElementList.cs
- Utils.cs
- ResolveMatchesApril2005.cs
- ReaderOutput.cs
- ArrayList.cs
- TextViewBase.cs
- RoleGroup.cs
- RankException.cs
- embossbitmapeffect.cs
- ListControl.cs
- NodeLabelEditEvent.cs
- ProtocolsSection.cs
- CommonGetThemePartSize.cs
- DataViewManager.cs
- XmlSchemaSimpleTypeList.cs
- ExpressionVisitor.cs
- ClrPerspective.cs
- ToolStripRendererSwitcher.cs
- GlyphRunDrawing.cs
- SettingsPropertyNotFoundException.cs
- SettingsContext.cs
- MergablePropertyAttribute.cs
- COM2EnumConverter.cs
- ProtocolsConfiguration.cs
- DummyDataSource.cs
- OrthographicCamera.cs
- ImageUrlEditor.cs
- BinaryExpression.cs
- PropertyStore.cs
- RadialGradientBrush.cs
- ResourcesChangeInfo.cs
- xmlNames.cs
- CollectionContainer.cs
- BaseDataListDesigner.cs
- SqlClientPermission.cs
- ValidationManager.cs
- TemplateColumn.cs
- Latin1Encoding.cs
- StdValidatorsAndConverters.cs
- Message.cs