Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Reflection / Assembly.cs / 7 / Assembly.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: Assembly ** ** ** Purpose: For Assembly-related stuff. ** ** =============================================================================*/ using System; using System.Collections; using CultureInfo = System.Globalization.CultureInfo; using System.Security; using System.Security.Policy; using System.Security.Permissions; using System.IO; using System.Reflection.Emit; using System.Reflection.Cache; using StringBuilder = System.Text.StringBuilder; using System.Configuration.Assemblies; using StackCrawlMark = System.Threading.StackCrawlMark; using System.Runtime.InteropServices; using BinaryFormatter = System.Runtime.Serialization.Formatters.Binary.BinaryFormatter; using System.Runtime.CompilerServices; using SecurityZone = System.Security.SecurityZone; using IEvidenceFactory = System.Security.IEvidenceFactory; using System.Runtime.Serialization; using Microsoft.Win32; using System.Threading; using __HResults = System.__HResults; using System.Runtime.Versioning; namespace System.Reflection { [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public delegate Module ModuleResolveEventHandler(Object sender, ResolveEventArgs e); [Serializable()] [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_Assembly))] [System.Runtime.InteropServices.ComVisible(true)] public class Assembly : _Assembly, IEvidenceFactory, ICustomAttributeProvider, ISerializable { public override bool Equals(object o) { if (o == null) return false; if (!(o is Assembly)) return false; Assembly rhs = o as Assembly; rhs = rhs.InternalAssembly; return (object)InternalAssembly == (object)rhs; } public override int GetHashCode() { return base.GetHashCode(); } internal virtual Assembly InternalAssembly { get { return this; } } // READ ME // If you modify any of these fields, you must also update the // AssemblyBaseObject structure in object.h internal AssemblyBuilderData m__assemblyData; internal AssemblyBuilderData m_assemblyData { get { return InternalAssembly.m__assemblyData; } set { InternalAssembly.m__assemblyData = value; } } [method:SecurityPermissionAttribute( SecurityAction.LinkDemand, ControlAppDomain = true )] private event ModuleResolveEventHandler _ModuleResolve; private ModuleResolveEventHandler ModuleResolveEvent { get { return InternalAssembly._ModuleResolve; } } public event ModuleResolveEventHandler ModuleResolve { [method: SecurityPermissionAttribute(SecurityAction.LinkDemand, ControlAppDomain = true)] add { InternalAssembly._ModuleResolve += value; } [method: SecurityPermissionAttribute(SecurityAction.LinkDemand, ControlAppDomain = true)] remove { InternalAssembly._ModuleResolve -= value; } } private InternalCache m__cachedData; internal InternalCache m_cachedData { get { return InternalAssembly.m__cachedData; } set { InternalAssembly.m__cachedData = value; } } private IntPtr m__assembly; // slack for ptr datum on unmanaged side internal IntPtr m_assembly { get { return InternalAssembly.m__assembly; } set { InternalAssembly.m__assembly = value; } } private const String s_localFilePrefix = "file:"; public virtual String CodeBase { get { String codeBase = nGetCodeBase(false); VerifyCodeBaseDiscovery(codeBase); return codeBase; } } public virtual String EscapedCodeBase { get { return AssemblyName.EscapeCodeBase(CodeBase); } } public virtual AssemblyName GetName() { return GetName(false); } internal unsafe AssemblyHandle AssemblyHandle { get { return new AssemblyHandle((void*)m_assembly); } } // If the assembly is copied before it is loaded, the codebase will be set to the // actual file loaded if fCopiedName is true. If it is false, then the original code base // is returned. public virtual AssemblyName GetName(bool copiedName) { AssemblyName an = new AssemblyName(); String codeBase = nGetCodeBase(copiedName); VerifyCodeBaseDiscovery(codeBase); an.Init(nGetSimpleName(), nGetPublicKey(), null, // public key token GetVersion(), GetLocale(), nGetHashAlgorithm(), AssemblyVersionCompatibility.SameMachine, codeBase, nGetFlags() | AssemblyNameFlags.PublicKey, null); // strong name key pair an.ProcessorArchitecture = ComputeProcArchIndex(); return an; } public virtual String FullName { get { // If called by Object.ToString(), return val may be NULL. String s; if ((s = (String)Cache[CacheObjType.AssemblyName]) != null) return s; s = GetFullName(); if (s != null) Cache[CacheObjType.AssemblyName] = s; return s; } } [MethodImplAttribute(MethodImplOptions.InternalCall)] public extern static String CreateQualifiedName(String assemblyName, String typeName); public virtual MethodInfo EntryPoint { get { RuntimeMethodHandle methodHandle = nGetEntryPoint(); if (!methodHandle.IsNullHandle()) return (MethodInfo)RuntimeType.GetMethodBase(methodHandle); return null; } } public static Assembly GetAssembly(Type type) { if (type == null) throw new ArgumentNullException("type"); Module m = type.Module; if (m == null) return null; else return m.Assembly; } Type _Assembly.GetType() { return base.GetType(); } public virtual Type GetType(String name) { return GetType(name, false, false); } public virtual Type GetType(String name, bool throwOnError) { return GetType(name, throwOnError, false); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Type _GetType(String name, bool throwOnError, bool ignoreCase); public Type GetType(String name, bool throwOnError, bool ignoreCase) { return InternalAssembly._GetType(name, throwOnError, ignoreCase); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Type[] _GetExportedTypes(); public virtual Type[] GetExportedTypes() { return InternalAssembly._GetExportedTypes(); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly, ResourceScope.Machine | ResourceScope.Assembly)] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual Type[] GetTypes() { Module[] m = nGetModules(true, false); int iNumModules = m.Length; int iFinalLength = 0; StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Type[][] ModuleTypes = new Type[iNumModules][]; for (int i = 0; i < iNumModules; i++) { ModuleTypes[i] = m[i].GetTypesInternal(ref stackMark); iFinalLength += ModuleTypes[i].Length; } int iCurrent = 0; Type[] ret = new Type[iFinalLength]; for (int i = 0; i < iNumModules; i++) { int iLength = ModuleTypes[i].Length; Array.Copy(ModuleTypes[i], 0, ret, iCurrent, iLength); iCurrent += iLength; } return ret; } // Load a resource based on the NameSpace of the type. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual Stream GetManifestResourceStream(Type type, String name) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return GetManifestResourceStream(type, name, false, ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual Stream GetManifestResourceStream(String name) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return GetManifestResourceStream(name, ref stackMark, false); } public Assembly GetSatelliteAssembly(CultureInfo culture) { return InternalGetSatelliteAssembly(culture, null, true); } // Useful for binding to a very specific version of a satellite assembly public Assembly GetSatelliteAssembly(CultureInfo culture, Version version) { return InternalGetSatelliteAssembly(culture, version, true); } public virtual Evidence Evidence { [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] get { return nGetEvidence().Copy(); } } // ISerializable implementation [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual void GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) throw new ArgumentNullException("info"); UnitySerializationHolder.GetUnitySerializationInfo(info, UnitySerializationHolder.AssemblyUnity, this.FullName, this); } internal bool AptcaCheck(Assembly sourceAssembly) { return AssemblyHandle.AptcaCheck(sourceAssembly.AssemblyHandle); } [ComVisible(false)] public Module ManifestModule { get { // We don't need to return the "external" ModuleBuilder because // it is meant to be read-only ModuleHandle manifestModuleHandle = AssemblyHandle.GetManifestModule(); if (manifestModuleHandle == null || manifestModuleHandle.IsNullHandle()) return null; return manifestModuleHandle.GetModule(); } } public virtual Object[] GetCustomAttributes(bool inherit) { return CustomAttribute.GetCustomAttributes(this, typeof(object) as RuntimeType); } public virtual Object[] GetCustomAttributes(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); RuntimeType attributeRuntimeType = attributeType.UnderlyingSystemType as RuntimeType; if (attributeRuntimeType == null) throw new ArgumentException(Environment.GetResourceString("Arg_MustBeType"),"attributeType"); return CustomAttribute.GetCustomAttributes(this, attributeRuntimeType); } public virtual bool IsDefined(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); RuntimeType attributeRuntimeType = attributeType.UnderlyingSystemType as RuntimeType; if (attributeRuntimeType == null) throw new ArgumentException(Environment.GetResourceString("Arg_MustBeType"),"caType"); return CustomAttribute.IsDefined(this, attributeRuntimeType); } // Locate an assembly by the name of the file containing the manifest. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly LoadFrom(String assemblyFile) { // The stack mark is used for MDA filtering StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, null, // securityEvidence null, // hashValue AssemblyHashAlgorithm.None, false, // forIntrospection ref stackMark); } // Locate an assembly for reflection by the name of the file containing the manifest. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly ReflectionOnlyLoadFrom(String assemblyFile) { // The stack mark is ingored for ReflectionOnlyLoadFrom StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, null, //securityEvidence null, //hashValue AssemblyHashAlgorithm.None, true, //forIntrospection ref stackMark); } // Evidence is protected in Assembly.Load() [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly LoadFrom(String assemblyFile, Evidence securityEvidence) { // The stack mark is used for MDA filtering StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, securityEvidence, null, // hashValue AssemblyHashAlgorithm.None, false, // forIntrospection ref stackMark); } // Evidence is protected in Assembly.Load() [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly LoadFrom(String assemblyFile, Evidence securityEvidence, byte[] hashValue, AssemblyHashAlgorithm hashAlgorithm) { // The stack mark is used for MDA filtering StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, securityEvidence, hashValue, hashAlgorithm, false, ref stackMark); } private static Assembly InternalLoadFrom(String assemblyFile, Evidence securityEvidence, byte[] hashValue, AssemblyHashAlgorithm hashAlgorithm, bool forIntrospection, ref StackCrawlMark stackMark) { if (assemblyFile == null) throw new ArgumentNullException("assemblyFile"); AssemblyName an = new AssemblyName(); an.CodeBase = assemblyFile; an.SetHashControl(hashValue, hashAlgorithm); return InternalLoad(an, securityEvidence, ref stackMark, forIntrospection); } // Locate an assembly by the long form of the assembly name. // eg. "Toolbox.dll, version=1.1.10.1220, locale=en, publickey=1234567890123456789012345678901234567890" [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly Load(String assemblyString) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyString, null, ref stackMark, false); } // Locate an assembly for reflection by the long form of the assembly name. // eg. "Toolbox.dll, version=1.1.10.1220, locale=en, publickey=1234567890123456789012345678901234567890" // [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly ReflectionOnlyLoad(String assemblyString) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyString, null, ref stackMark, true /*forIntrospection*/); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly Load(String assemblyString, Evidence assemblySecurity) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyString, assemblySecurity, ref stackMark, false); } // Locate an assembly by its name. The name can be strong or // weak. The assembly is loaded into the domain of the caller. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(AssemblyName assemblyRef) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyRef, null, ref stackMark, false); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(AssemblyName assemblyRef, Evidence assemblySecurity) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyRef, assemblySecurity, ref stackMark, false); } // used by vm [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static unsafe private IntPtr LoadWithPartialNameHack(String partialName, bool cropPublicKey) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Assembly result = null; AssemblyName an = new AssemblyName(partialName); if (!IsSimplyNamed(an)) { if (cropPublicKey) { an.SetPublicKey(null); an.SetPublicKeyToken(null); } AssemblyName GACAssembly = EnumerateCache(an); if(GACAssembly != null) result = InternalLoad(GACAssembly, null, ref stackMark, false); } if (result == null) return (IntPtr)0; return (IntPtr)result.AssemblyHandle.Value; } [Obsolete("This method has been deprecated. Please use Assembly.Load() instead. http://go.microsoft.com/fwlink/?linkid=14202")] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly LoadWithPartialName(String partialName) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return LoadWithPartialNameInternal(partialName, null, ref stackMark); } [Obsolete("This method has been deprecated. Please use Assembly.Load() instead. http://go.microsoft.com/fwlink/?linkid=14202")] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly LoadWithPartialName(String partialName, Evidence securityEvidence) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return LoadWithPartialNameInternal(partialName, securityEvidence, ref stackMark); } static internal Assembly LoadWithPartialNameInternal(String partialName, Evidence securityEvidence, ref StackCrawlMark stackMark) { if (securityEvidence != null) new SecurityPermission( SecurityPermissionFlag.ControlEvidence ).Demand(); Assembly result = null; AssemblyName an = new AssemblyName(partialName); try { result = nLoad(an, null, securityEvidence, null, ref stackMark, true, false); } catch(Exception e) { if (e.IsTransient) throw e; if (IsSimplyNamed(an)) return null; AssemblyName GACAssembly = EnumerateCache(an); if(GACAssembly != null) return InternalLoad(GACAssembly, securityEvidence, ref stackMark, false); } return result; } // To not break compatibility with the V1 _Assembly interface we need to make this // new member ComVisible(false). This should be cleaned up in Whidbey. [ComVisible(false)] public virtual bool ReflectionOnly { get { return nReflection(); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool _nReflection(); internal bool nReflection() { return InternalAssembly._nReflection(); } static private AssemblyName EnumerateCache(AssemblyName partialName) { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); partialName.Version = null; ArrayList a = new ArrayList(); Fusion.ReadCache(a, partialName.FullName, ASM_CACHE.GAC); IEnumerator myEnum = a.GetEnumerator(); AssemblyName ainfoBest = null; CultureInfo refCI = partialName.CultureInfo; while (myEnum.MoveNext()) { AssemblyName ainfo = new AssemblyName((String)myEnum.Current); if (CulturesEqual(refCI, ainfo.CultureInfo)) { if (ainfoBest == null) ainfoBest = ainfo; else { // Choose highest version if (ainfo.Version > ainfoBest.Version) ainfoBest = ainfo; } } } return ainfoBest; } static private bool CulturesEqual(CultureInfo refCI, CultureInfo defCI) { bool defNoCulture = defCI.Equals(CultureInfo.InvariantCulture); // cultured asms aren't allowed to be bound to if // the ref doesn't ask for them specifically if ((refCI == null) || refCI.Equals(CultureInfo.InvariantCulture)) return defNoCulture; if (defNoCulture || ( !defCI.Equals(refCI) )) return false; return true; } static private bool IsSimplyNamed(AssemblyName partialName) { byte[] pk = partialName.GetPublicKeyToken(); if ((pk != null) && (pk.Length == 0)) return true; pk = partialName.GetPublicKey(); if ((pk != null) && (pk.Length == 0)) return true; return false; } // Loads the assembly with a COFF based IMAGE containing // an emitted assembly. The assembly is loaded into the domain // of the caller. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(byte[] rawAssembly) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, null, // symbol store null, // evidence ref stackMark, false // fIntrospection ); } // Loads the assembly for reflection with a COFF based IMAGE containing // an emitted assembly. The assembly is loaded into the domain // of the caller. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly ReflectionOnlyLoad(byte[] rawAssembly) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, null, // symbol store null, // evidence ref stackMark, true // fIntrospection ); } // Loads the assembly with a COFF based IMAGE containing // an emitted assembly. The assembly is loaded into the domain // of the caller. The second parameter is the raw bytes // representing the symbol store that matches the assembly. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(byte[] rawAssembly, byte[] rawSymbolStore) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, rawSymbolStore, null, // evidence ref stackMark, false // fIntrospection ); } [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.ControlEvidence)] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(byte[] rawAssembly, byte[] rawSymbolStore, Evidence securityEvidence) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, rawSymbolStore, securityEvidence, ref stackMark, false // fIntrospection ); } static public Assembly LoadFile(String path) { new FileIOPermission(FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read, path).Demand(); return nLoadFile(path, null); } [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.ControlEvidence)] static public Assembly LoadFile(String path, Evidence securityEvidence) { new FileIOPermission(FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read, path).Demand(); return nLoadFile(path, securityEvidence); } public Module LoadModule(String moduleName, byte[] rawModule) { return nLoadModule(moduleName, rawModule, null, Evidence); // does a ControlEvidence demand } public Module LoadModule(String moduleName, byte[] rawModule, byte[] rawSymbolStore) { return nLoadModule(moduleName, rawModule, rawSymbolStore, Evidence); // does a ControlEvidence demand } // // Locates a type from this assembly and creates an instance of it using // the system activator. // public Object CreateInstance(String typeName) { return CreateInstance(typeName, false, // ignore case BindingFlags.Public | BindingFlags.Instance, null, // binder null, // args null, // culture null); // activation attributes } public Object CreateInstance(String typeName, bool ignoreCase) { return CreateInstance(typeName, ignoreCase, BindingFlags.Public | BindingFlags.Instance, null, // binder null, // args null, // culture null); // activation attributes } public Object CreateInstance(String typeName, bool ignoreCase, BindingFlags bindingAttr, Binder binder, Object[] args, CultureInfo culture, Object[] activationAttributes) { Type t = GetType(typeName, false, ignoreCase); if (t == null) return null; return Activator.CreateInstance(t, bindingAttr, binder, args, culture, activationAttributes); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetLoadedModules() { return nGetModules(false, false); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetLoadedModules(bool getResourceModules) { return nGetModules(false, getResourceModules); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetModules() { return nGetModules(true, false); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetModules(bool getResourceModules) { return nGetModules(true, getResourceModules); } // Returns the module in this assembly with name 'name' [MethodImplAttribute(MethodImplOptions.InternalCall)] [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] internal extern Module _GetModule(String name); public Module GetModule(String name) { return GetModuleInternal(name); } internal virtual Module GetModuleInternal(String name) { return InternalAssembly._GetModule(name); } // Returns the file in the File table of the manifest that matches the // given name. (Name should not include path.) [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public virtual FileStream GetFile(String name) { Module m = GetModule(name); if (m == null) return null; return new FileStream(m.InternalGetFullyQualifiedName(), FileMode.Open, FileAccess.Read, FileShare.Read); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public virtual FileStream[] GetFiles() { return GetFiles(false); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public virtual FileStream[] GetFiles(bool getResourceModules) { Module[] m = nGetModules(true, getResourceModules); int iLength = m.Length; FileStream[] fs = new FileStream[iLength]; for(int i = 0; i < iLength; i++) fs[i] = new FileStream(m[i].InternalGetFullyQualifiedName(), FileMode.Open, FileAccess.Read, FileShare.Read); return fs; } // Returns the names of all the resources public virtual String[] GetManifestResourceNames() { return nGetManifestResourceNames(); } // Returns the names of all the resources [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String[] _nGetManifestResourceNames(); internal String[] nGetManifestResourceNames() { return InternalAssembly._nGetManifestResourceNames(); } /* * Get the assembly that the current code is running from. */ [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly GetExecutingAssembly() { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nGetExecutingAssembly(ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly GetCallingAssembly() { StackCrawlMark stackMark = StackCrawlMark.LookForMyCallersCaller; return nGetExecutingAssembly(ref stackMark); } public static Assembly GetEntryAssembly() { AppDomainManager domainManager = AppDomain.CurrentDomain.DomainManager; if (domainManager == null) domainManager = new AppDomainManager(); return domainManager.EntryAssembly; } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern AssemblyName[] _GetReferencedAssemblies(); public AssemblyName[] GetReferencedAssemblies() { return InternalAssembly._GetReferencedAssemblies(); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual ManifestResourceInfo GetManifestResourceInfo(String resourceName) { Assembly assemblyRef; String fileName; StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; int location = nGetManifestResourceInfo(resourceName, out assemblyRef, out fileName, ref stackMark); if (location == -1) return null; else return new ManifestResourceInfo(assemblyRef, fileName, (ResourceLocation) location); } public override String ToString() { String displayName = FullName; if (displayName == null) return base.ToString(); else return displayName; } public virtual String Location { get { String location = GetLocation(); if (location != null) new FileIOPermission( FileIOPermissionAccess.PathDiscovery, location ).Demand(); return location; } } // To not break compatibility with the V1 _Assembly interface we need to make this // new member ComVisible(false). This should be cleaned up in Whidbey. [ComVisible(false)] public virtual String ImageRuntimeVersion { get{ return nGetImageRuntimeVersion(); } } /* Returns true if the assembly was loaded from the global assembly cache. */ public bool GlobalAssemblyCache { get { return nGlobalAssemblyCache(); } } [ComVisible(false)] public Int64 HostContext { get { return GetHostContext(); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Int64 _GetHostContext(); private Int64 GetHostContext() { return InternalAssembly._GetHostContext(); } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] internal static String VerifyCodeBase(String codebase) { if(codebase == null) return null; int len = codebase.Length; if (len == 0) return null; int j = codebase.IndexOf(':'); // Check to see if the url has a prefix if( (j != -1) && (j+2 < len) && ((codebase[j+1] == '/') || (codebase[j+1] == '\\')) && ((codebase[j+2] == '/') || (codebase[j+2] == '\\')) ) return codebase; #if !PLATFORM_UNIX else if ((len > 2) && (codebase[0] == '\\') && (codebase[1] == '\\')) return "file://" + codebase; else return "file:///" + Path.GetFullPathInternal( codebase ); #else else return "file://" + Path.GetFullPathInternal( codebase ); #endif // !PLATFORM_UNIX } internal virtual Stream GetManifestResourceStream(Type type, String name, bool skipSecurityCheck, ref StackCrawlMark stackMark) { StringBuilder sb = new StringBuilder(); if(type == null) { if (name == null) throw new ArgumentNullException("type"); } else { String nameSpace = type.Namespace; if(nameSpace != null) { sb.Append(nameSpace); if(name != null) sb.Append(Type.Delimiter); } } if(name != null) sb.Append(name); return GetManifestResourceStream(sb.ToString(), ref stackMark, skipSecurityCheck); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Module _nLoadModule(String moduleName, byte[] rawModule, byte[] rawSymbolStore, Evidence securityEvidence); private Module nLoadModule(String moduleName, byte[] rawModule, byte[] rawSymbolStore, Evidence securityEvidence) { return InternalAssembly._nLoadModule(moduleName, rawModule, rawSymbolStore, securityEvidence); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool _nGlobalAssemblyCache(); private bool nGlobalAssemblyCache() { return InternalAssembly._nGlobalAssemblyCache(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetImageRuntimeVersion(); private String nGetImageRuntimeVersion() { return InternalAssembly._nGetImageRuntimeVersion(); } internal Assembly() { } // Create a new module in which to emit code. This module will not contain the manifest. [MethodImplAttribute(MethodImplOptions.InternalCall)] static private extern Module _nDefineDynamicModule(Assembly containingAssembly, bool emitSymbolInfo, String filename, ref StackCrawlMark stackMark); static internal Module nDefineDynamicModule(Assembly containingAssembly, bool emitSymbolInfo, String filename, ref StackCrawlMark stackMark) { return _nDefineDynamicModule(containingAssembly.InternalAssembly, emitSymbolInfo, filename, ref stackMark); } // The following functions are native helpers for creating on-disk manifest [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nPrepareForSavingManifestToDisk(Module assemblyModule); // module to contain assembly information if assembly is embedded internal void nPrepareForSavingManifestToDisk(Module assemblyModule) { if (assemblyModule != null) assemblyModule = assemblyModule.InternalModule; InternalAssembly._nPrepareForSavingManifestToDisk(assemblyModule); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nSaveToFileList(String strFileName); internal int nSaveToFileList(String strFileName) { return InternalAssembly._nSaveToFileList(strFileName); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nSetHashValue(int tkFile, String strFullFileName); internal int nSetHashValue(int tkFile, String strFullFileName) { return InternalAssembly._nSetHashValue(tkFile, strFullFileName); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nSaveExportedType(String strComTypeName, int tkAssemblyRef, int tkTypeDef, TypeAttributes flags); internal int nSaveExportedType(String strComTypeName, int tkAssemblyRef, int tkTypeDef, TypeAttributes flags) { return InternalAssembly._nSaveExportedType(strComTypeName, tkAssemblyRef, tkTypeDef, flags); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nSavePermissionRequests(byte[] required, byte[] optional, byte[] refused); internal void nSavePermissionRequests(byte[] required, byte[] optional, byte[] refused) { InternalAssembly._nSavePermissionRequests(required, optional, refused); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nSaveManifestToDisk( String strFileName, int entryPoint, int fileKind, int portableExecutableKind, int ImageFileMachine); internal void nSaveManifestToDisk( String strFileName, int entryPoint, int fileKind, int portableExecutableKind, int ImageFileMachine) { InternalAssembly._nSaveManifestToDisk( strFileName, entryPoint, fileKind, portableExecutableKind, ImageFileMachine); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nAddFileToInMemoryFileList(String strFileName, Module module); internal int nAddFileToInMemoryFileList(String strFileName, Module module) { if (module != null) module = module.InternalModule; return InternalAssembly._nAddFileToInMemoryFileList(strFileName, module); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Module _nGetOnDiskAssemblyModule(); internal Module nGetOnDiskAssemblyModule() { return InternalAssembly._nGetOnDiskAssemblyModule(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Module _nGetInMemoryAssemblyModule(); internal Module nGetInMemoryAssemblyModule() { return InternalAssembly._nGetInMemoryAssemblyModule(); } #if !FEATURE_PAL // Functions for defining unmanaged resources. [MethodImplAttribute(MethodImplOptions.InternalCall)] static internal extern String nDefineVersionInfoResource(String filename, String title, String iconFilename, String description, String copyright, String trademark, String company, String product, String productVersion, String fileVersion, int lcid, bool isDll); #endif // !FEATURE_PAL private static void DecodeSerializedEvidence( Evidence evidence, byte[] serializedEvidence ) { BinaryFormatter formatter = new BinaryFormatter(); Evidence asmEvidence = null; PermissionSet permSet = new PermissionSet( false ); permSet.SetPermission( new SecurityPermission( SecurityPermissionFlag.SerializationFormatter ) ); permSet.PermitOnly(); permSet.Assert(); try { using(MemoryStream ms = new MemoryStream( serializedEvidence )) asmEvidence = (Evidence)formatter.Deserialize( ms ); } catch { } if (asmEvidence != null) { IEnumerator enumerator = asmEvidence.GetAssemblyEnumerator(); while (enumerator.MoveNext()) { Object obj = enumerator.Current; evidence.AddAssembly( obj ); } } } #if !FEATURE_PAL private static void AddX509Certificate( Evidence evidence, byte[] cert ) { evidence.AddHost(new Publisher(new System.Security.Cryptography.X509Certificates.X509Certificate(cert))); } #endif // !FEATURE_PAL private static void AddStrongName(Evidence evidence, byte[] blob, String strSimpleName, int major, int minor, int build, int revision, Assembly assembly) { StrongName sn = new StrongName(new StrongNamePublicKeyBlob(blob), strSimpleName, new Version(major, minor, build, revision), assembly); evidence.AddHost(sn); } private static Evidence CreateSecurityIdentity(Assembly asm, String strUrl, int zone, byte[] cert, byte[] publicKeyBlob, String strSimpleName, int major, int minor, int build, int revision, byte[] serializedEvidence, Evidence additionalEvidence) { Evidence evidence = new Evidence(); if (zone != -1) evidence.AddHost( new Zone((SecurityZone)zone) ); if (strUrl != null) { evidence.AddHost( new Url(strUrl, true) ); // Only create a site piece of evidence if we are not loading from a file. if (String.Compare( strUrl, 0, s_localFilePrefix, 0, 5, StringComparison.OrdinalIgnoreCase) != 0) evidence.AddHost( Site.CreateFromUrl( strUrl ) ); } #if !FEATURE_PAL if (cert != null) AddX509Certificate( evidence, cert ); #endif // !FEATURE_PAL // Determine if it's in the GAC and add some evidence about it if(asm != null && System.Runtime.InteropServices.RuntimeEnvironment.FromGlobalAccessCache(asm)) evidence.AddHost( new GacInstalled() ); // This code was moved to a different function because: // 1) it is rarely called so we should only JIT it if we need it. // 2) it references lots of classes that otherwise aren't loaded. if (serializedEvidence != null) DecodeSerializedEvidence( evidence, serializedEvidence ); if ((publicKeyBlob != null) && (publicKeyBlob.Length != 0)) { AddStrongName(evidence, publicKeyBlob, strSimpleName, major, minor, build, revision, asm); } #if !FEATURE_PAL if (asm != null && !asm.nIsDynamic()) evidence.AddHost(new Hash(asm)); #endif // !FEATURE_PAL // If the host (caller of Assembly.Load) provided evidence, merge it // with the evidence we've just created. The host evidence takes // priority. if (additionalEvidence != null) evidence.MergeWithNoDuplicates(additionalEvidence); if (asm != null) { // The host might want to modify the evidence of the assembly through // the HostSecurityManager provided in AppDomainManager, so take that into account. HostSecurityManager securityManager = AppDomain.CurrentDomain.HostSecurityManager; if ((securityManager.Flags & HostSecurityManagerOptions.HostAssemblyEvidence) == HostSecurityManagerOptions.HostAssemblyEvidence) return securityManager.ProvideAssemblyEvidence(asm, evidence); } return evidence; } ////// Determine if this assembly was loaded from a location under the AppBase for security /// purposes. For instance, strong name bypass is disabled for assemblies considered outside /// the AppBase. /// /// This method is called from the VM: AssemblySecurityDescriptor::WasAssemblyLoadedFromAppBase /// [FileIOPermission(SecurityAction.Assert, Unrestricted = true)] private bool IsAssemblyUnderAppBase() { string assemblyLocation = GetLocation(); // Assemblies that are loaded without a location will be considered part of the app if (String.IsNullOrEmpty(assemblyLocation)) { return true; } // Compare the paths using the FileIOAccess mechanism that FileIOPermission uses internally. If // assemblyAccess is a subset of appbaseAccess (in FileIOPermission terms, appBaseAccess is the // demand set, and assemblyAccess is the grant set) that means assembly must be under the AppBase. FileIOAccess assemblyAccess = new FileIOAccess(Path.GetFullPathInternal(assemblyLocation)); FileIOAccess appBaseAccess = new FileIOAccess(Path.GetFullPathInternal(AppDomain.CurrentDomain.BaseDirectory)); return assemblyAccess.IsSubsetOf(appBaseAccess); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern bool IsStrongNameVerified(); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern static Assembly nGetExecutingAssembly(ref StackCrawlMark stackMark); internal unsafe virtual Stream GetManifestResourceStream(String name, ref StackCrawlMark stackMark, bool skipSecurityCheck) { ulong length = 0; byte* pbInMemoryResource = GetResource(name, out length, ref stackMark, skipSecurityCheck); if (pbInMemoryResource != null) { //Console.WriteLine("Creating an unmanaged memory stream of length "+length); if (length > Int64.MaxValue) throw new NotImplementedException(Environment.GetResourceString("NotImplemented_ResourcesLongerThan2^63")); //For cases where we're loading an embedded resource from an assembly, // in V1 we do not have any serious lifetime issues with the // UnmanagedMemoryStream. If the Stream is only used // in the AppDomain that contains the assembly, then if that AppDomain // is unloaded, we will collect all of the objects in the AppDomain first // before unloading assemblies. If the Stream is shared across AppDomains, // then the original AppDomain was unloaded, accesses to this Stream will // throw an exception saying the appdomain was unloaded. This is // guaranteed be EE AppDomain goo. And for shared assemblies like // mscorlib, their lifetime is the lifetime of the process, so the // assembly will NOT be unloaded, so the resource will always be in memory. return new UnmanagedMemoryStream(pbInMemoryResource, (long)length, (long)length, FileAccess.Read, true); } //Console.WriteLine("GetManifestResourceStream: Blob "+name+" not found..."); return null; } internal Version GetVersion() { int majorVer, minorVer, build, revision; nGetVersion(out majorVer, out minorVer, out build, out revision); return new Version (majorVer, minorVer, build, revision); } internal CultureInfo GetLocale() { String locale = nGetLocale(); if (locale == null) return CultureInfo.InvariantCulture; return new CultureInfo(locale); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetLocale(); private String nGetLocale() { return InternalAssembly._nGetLocale(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nGetVersion(out int majVer, out int minVer, out int buildNum, out int revNum); internal void nGetVersion(out int majVer, out int minVer, out int buildNum, out int revNum) { InternalAssembly._nGetVersion(out majVer, out minVer, out buildNum, out revNum); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool _nIsDynamic(); internal bool nIsDynamic() { return InternalAssembly._nIsDynamic(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nGetManifestResourceInfo(String resourceName, out Assembly assemblyRef, out String fileName, ref StackCrawlMark stackMark); private int nGetManifestResourceInfo(String resourceName, out Assembly assemblyRef, out String fileName, ref StackCrawlMark stackMark) { return InternalAssembly._nGetManifestResourceInfo( resourceName, out assemblyRef, out fileName, ref stackMark); } private void VerifyCodeBaseDiscovery(String codeBase) { if ((codeBase != null) && (String.Compare( codeBase, 0, s_localFilePrefix, 0, 5, StringComparison.OrdinalIgnoreCase) == 0)) { System.Security.Util.URLString urlString = new System.Security.Util.URLString( codeBase, true ); new FileIOPermission( FileIOPermissionAccess.PathDiscovery, urlString.GetFileName() ).Demand(); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _GetLocation(); internal String GetLocation() { return InternalAssembly._GetLocation(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern byte[] _nGetPublicKey(); internal byte[] nGetPublicKey() { return InternalAssembly._nGetPublicKey(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetSimpleName(); internal String nGetSimpleName() { return InternalAssembly._nGetSimpleName(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetCodeBase(bool fCopiedName); internal String nGetCodeBase(bool fCopiedName) { return InternalAssembly._nGetCodeBase(fCopiedName); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern AssemblyHashAlgorithm _nGetHashAlgorithm(); internal AssemblyHashAlgorithm nGetHashAlgorithm() { return InternalAssembly._nGetHashAlgorithm(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern AssemblyNameFlags _nGetFlags(); internal AssemblyNameFlags nGetFlags() { return InternalAssembly._nGetFlags(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern void _nGetGrantSet(out PermissionSet newGrant, out PermissionSet newDenied); internal void nGetGrantSet(out PermissionSet newGrant, out PermissionSet newDenied) { InternalAssembly._nGetGrantSet(out newGrant, out newDenied); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _GetFullName(); internal String GetFullName() { return InternalAssembly._GetFullName(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private unsafe extern void* _nGetEntryPoint(); private unsafe RuntimeMethodHandle nGetEntryPoint() { return new RuntimeMethodHandle(_nGetEntryPoint()); } // GetResource will return a handle to a file (or -1) and set the length. // It will also return a pointer to the resources if they're in memory. [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern Evidence _nGetEvidence(); internal Evidence nGetEvidence() { return InternalAssembly._nGetEvidence(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private unsafe extern byte* _GetResource(String resourceName, out ulong length, ref StackCrawlMark stackMark, bool skipSecurityCheck); private unsafe byte* GetResource(String resourceName, out ulong length, ref StackCrawlMark stackMark, bool skipSecurityCheck) { return InternalAssembly._GetResource(resourceName, out length, ref stackMark, skipSecurityCheck); } internal static Assembly InternalLoad(String assemblyString, Evidence assemblySecurity, ref StackCrawlMark stackMark, bool forIntrospection) { if (assemblyString == null) throw new ArgumentNullException("assemblyString"); if ((assemblyString.Length == 0) || (assemblyString[0] == '\0')) throw new ArgumentException(Environment.GetResourceString("Format_StringZeroLength")); AssemblyName an = new AssemblyName(); Assembly assembly = null; an.Name = assemblyString; int hr = an.nInit(out assembly, forIntrospection, true); if (hr == System.__HResults.FUSION_E_INVALID_NAME) { return assembly; } else return InternalLoad(an, assemblySecurity, ref stackMark, forIntrospection); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] internal static Assembly InternalLoad(AssemblyName assemblyRef, Evidence assemblySecurity, ref StackCrawlMark stackMark, bool forIntrospection) { if (assemblyRef == null) throw new ArgumentNullException("assemblyRef"); assemblyRef = (AssemblyName)assemblyRef.Clone(); if (assemblySecurity != null) new SecurityPermission( SecurityPermissionFlag.ControlEvidence ).Demand(); String codeBase = VerifyCodeBase(assemblyRef.CodeBase); if (codeBase != null) { if (String.Compare( codeBase, 0, s_localFilePrefix, 0, 5, StringComparison.OrdinalIgnoreCase) != 0) { IPermission perm = CreateWebPermission( assemblyRef.EscapedCodeBase ); perm.Demand(); } else { System.Security.Util.URLString urlString = new System.Security.Util.URLString( codeBase, true ); new FileIOPermission( FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read , urlString.GetFileName() ).Demand(); } } return nLoad(assemblyRef, codeBase, assemblySecurity, null, ref stackMark, true, forIntrospection); } // demandFlag: // 0 demand PathDiscovery permission only // 1 demand Read permission only // 2 demand both Read and PathDiscovery // 3 demand Web permission only [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] private static void DemandPermission(String codeBase, bool havePath, int demandFlag) { FileIOPermissionAccess access = FileIOPermissionAccess.PathDiscovery; switch(demandFlag) { case 0: // default break; case 1: access = FileIOPermissionAccess.Read; break; case 2: access = FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read; break; case 3: IPermission perm = CreateWebPermission(AssemblyName.EscapeCodeBase(codeBase)); perm.Demand(); return; } if (!havePath) { System.Security.Util.URLString urlString = new System.Security.Util.URLString( codeBase, true ); codeBase = urlString.GetFileName(); } codeBase = Path.GetFullPathInternal(codeBase); // canonicalize new FileIOPermission(access, codeBase).Demand(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern Assembly _nLoad(AssemblyName fileName, String codeBase, Evidence assemblySecurity, Assembly locationHint, ref StackCrawlMark stackMark, bool throwOnFileNotFound, bool forIntrospection); [ResourceExposure(ResourceScope.None)] private static Assembly nLoad(AssemblyName fileName, String codeBase, Evidence assemblySecurity, Assembly locationHint, ref StackCrawlMark stackMark, bool throwOnFileNotFound, bool forIntrospection) { if (locationHint != null) locationHint = locationHint.InternalAssembly; return _nLoad(fileName, codeBase, assemblySecurity, locationHint, ref stackMark, throwOnFileNotFound, forIntrospection); } private static IPermission CreateWebPermission( String codeBase ) { BCLDebug.Assert( codeBase != null, "Must pass in a valid CodeBase" ); Assembly sys = Assembly.Load("System, Version=" + ThisAssembly.Version + ", Culture=neutral, PublicKeyToken=" + AssemblyRef.EcmaPublicKeyToken); Type type = sys.GetType("System.Net.NetworkAccess", true); IPermission retval = null; if (!type.IsEnum || !type.IsVisible) goto Exit; Object[] webArgs = new Object[2]; webArgs[0] = (Enum) Enum.Parse(type, "Connect", true); if (webArgs[0] == null) goto Exit; webArgs[1] = codeBase; type = sys.GetType("System.Net.WebPermission", true); if (!type.IsVisible) goto Exit; retval = (IPermission) Activator.CreateInstance(type, webArgs); Exit: if (retval == null) { BCLDebug.Assert( false, "Unable to create WebPermission" ); throw new ExecutionEngineException(); } return retval; } private Module OnModuleResolveEvent(String moduleName) { ModuleResolveEventHandler moduleResolve =ModuleResolveEvent; if (moduleResolve == null) return null; Delegate[] ds = moduleResolve.GetInvocationList(); int len = ds.Length; for (int i = 0; i < len; i++) { Module ret = ((ModuleResolveEventHandler) ds[i])(this, new ResolveEventArgs(moduleName)); if (ret != null) return ret.InternalModule; } return null; } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable internal Assembly InternalGetSatelliteAssembly(CultureInfo culture, Version version, bool throwOnFileNotFound) { if (culture == null) throw new ArgumentNullException("culture"); AssemblyName an = new AssemblyName(); an.SetPublicKey(nGetPublicKey()); an.Flags = nGetFlags() | AssemblyNameFlags.PublicKey; if (version == null) an.Version = GetVersion(); else an.Version = version; an.CultureInfo = culture; an.Name = nGetSimpleName() + ".resources"; StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Assembly a = nLoad(an, null, null, this, ref stackMark, throwOnFileNotFound, false); if (a == this) { throw new FileNotFoundException(String.Format(culture, Environment.GetResourceString("IO.FileNotFound_FileName"), an.Name)); } return a; } internal InternalCache Cache { get { // This grabs an internal copy of m_cachedData and uses // that instead of looking at m_cachedData directly because // the cache may get cleared asynchronously. This prevents // us from having to take a lock. InternalCache cache = m_cachedData; if (cache == null) { cache = new InternalCache("Assembly"); m_cachedData = cache; GC.ClearCache += new ClearCacheHandler(OnCacheClear); } return cache; } } internal void OnCacheClear(Object sender, ClearCacheEventArgs cacheEventArgs) { m_cachedData = null; } [MethodImplAttribute(MethodImplOptions.InternalCall)] static internal extern Assembly nLoadFile(String path, Evidence evidence); [MethodImplAttribute(MethodImplOptions.InternalCall)] static internal extern Assembly nLoadImage(byte[] rawAssembly, byte[] rawSymbolStore, Evidence evidence, ref StackCrawlMark stackMark, bool fIntrospection); // Add an entry to assembly's manifestResource table for a stand alone resource. [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nAddStandAloneResource(String strName, String strFileName, String strFullFileName, int attribute); internal void nAddStandAloneResource(String strName, String strFileName, String strFullFileName, int attribute) { InternalAssembly._nAddStandAloneResource(strName, strFileName, strFullFileName, attribute); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] internal virtual Module[] nGetModules(bool loadIfNotFound, bool getResourceModules) { return InternalAssembly._nGetModules(loadIfNotFound, getResourceModules); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern Module[] _nGetModules(bool loadIfNotFound, bool getResourceModules); internal ProcessorArchitecture ComputeProcArchIndex() { PortableExecutableKinds pek; ImageFileMachine ifm; Module manifestModule = ManifestModule; if(manifestModule != null) { if(manifestModule.MDStreamVersion > 0x10000) { ManifestModule.GetPEKind(out pek, out ifm); if((pek & System.Reflection.PortableExecutableKinds.PE32Plus) == System.Reflection.PortableExecutableKinds.PE32Plus) { switch(ifm) { case System.Reflection.ImageFileMachine.IA64: return ProcessorArchitecture.IA64; case System.Reflection.ImageFileMachine.AMD64: return ProcessorArchitecture.Amd64; case System.Reflection.ImageFileMachine.I386: if ((pek & System.Reflection.PortableExecutableKinds.ILOnly) == System.Reflection.PortableExecutableKinds.ILOnly) return ProcessorArchitecture.MSIL; break; } } else { if(ifm == System.Reflection.ImageFileMachine.I386) { if((pek & System.Reflection.PortableExecutableKinds.Required32Bit) == System.Reflection.PortableExecutableKinds.Required32Bit) return ProcessorArchitecture.X86; if((pek & System.Reflection.PortableExecutableKinds.ILOnly) == System.Reflection.PortableExecutableKinds.ILOnly) return ProcessorArchitecture.MSIL; return ProcessorArchitecture.X86; } } } } return ProcessorArchitecture.None; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: Assembly ** ** ** Purpose: For Assembly-related stuff. ** ** =============================================================================*/ using System; using System.Collections; using CultureInfo = System.Globalization.CultureInfo; using System.Security; using System.Security.Policy; using System.Security.Permissions; using System.IO; using System.Reflection.Emit; using System.Reflection.Cache; using StringBuilder = System.Text.StringBuilder; using System.Configuration.Assemblies; using StackCrawlMark = System.Threading.StackCrawlMark; using System.Runtime.InteropServices; using BinaryFormatter = System.Runtime.Serialization.Formatters.Binary.BinaryFormatter; using System.Runtime.CompilerServices; using SecurityZone = System.Security.SecurityZone; using IEvidenceFactory = System.Security.IEvidenceFactory; using System.Runtime.Serialization; using Microsoft.Win32; using System.Threading; using __HResults = System.__HResults; using System.Runtime.Versioning; namespace System.Reflection { [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public delegate Module ModuleResolveEventHandler(Object sender, ResolveEventArgs e); [Serializable()] [ClassInterface(ClassInterfaceType.None)] [ComDefaultInterface(typeof(_Assembly))] [System.Runtime.InteropServices.ComVisible(true)] public class Assembly : _Assembly, IEvidenceFactory, ICustomAttributeProvider, ISerializable { public override bool Equals(object o) { if (o == null) return false; if (!(o is Assembly)) return false; Assembly rhs = o as Assembly; rhs = rhs.InternalAssembly; return (object)InternalAssembly == (object)rhs; } public override int GetHashCode() { return base.GetHashCode(); } internal virtual Assembly InternalAssembly { get { return this; } } // READ ME // If you modify any of these fields, you must also update the // AssemblyBaseObject structure in object.h internal AssemblyBuilderData m__assemblyData; internal AssemblyBuilderData m_assemblyData { get { return InternalAssembly.m__assemblyData; } set { InternalAssembly.m__assemblyData = value; } } [method:SecurityPermissionAttribute( SecurityAction.LinkDemand, ControlAppDomain = true )] private event ModuleResolveEventHandler _ModuleResolve; private ModuleResolveEventHandler ModuleResolveEvent { get { return InternalAssembly._ModuleResolve; } } public event ModuleResolveEventHandler ModuleResolve { [method: SecurityPermissionAttribute(SecurityAction.LinkDemand, ControlAppDomain = true)] add { InternalAssembly._ModuleResolve += value; } [method: SecurityPermissionAttribute(SecurityAction.LinkDemand, ControlAppDomain = true)] remove { InternalAssembly._ModuleResolve -= value; } } private InternalCache m__cachedData; internal InternalCache m_cachedData { get { return InternalAssembly.m__cachedData; } set { InternalAssembly.m__cachedData = value; } } private IntPtr m__assembly; // slack for ptr datum on unmanaged side internal IntPtr m_assembly { get { return InternalAssembly.m__assembly; } set { InternalAssembly.m__assembly = value; } } private const String s_localFilePrefix = "file:"; public virtual String CodeBase { get { String codeBase = nGetCodeBase(false); VerifyCodeBaseDiscovery(codeBase); return codeBase; } } public virtual String EscapedCodeBase { get { return AssemblyName.EscapeCodeBase(CodeBase); } } public virtual AssemblyName GetName() { return GetName(false); } internal unsafe AssemblyHandle AssemblyHandle { get { return new AssemblyHandle((void*)m_assembly); } } // If the assembly is copied before it is loaded, the codebase will be set to the // actual file loaded if fCopiedName is true. If it is false, then the original code base // is returned. public virtual AssemblyName GetName(bool copiedName) { AssemblyName an = new AssemblyName(); String codeBase = nGetCodeBase(copiedName); VerifyCodeBaseDiscovery(codeBase); an.Init(nGetSimpleName(), nGetPublicKey(), null, // public key token GetVersion(), GetLocale(), nGetHashAlgorithm(), AssemblyVersionCompatibility.SameMachine, codeBase, nGetFlags() | AssemblyNameFlags.PublicKey, null); // strong name key pair an.ProcessorArchitecture = ComputeProcArchIndex(); return an; } public virtual String FullName { get { // If called by Object.ToString(), return val may be NULL. String s; if ((s = (String)Cache[CacheObjType.AssemblyName]) != null) return s; s = GetFullName(); if (s != null) Cache[CacheObjType.AssemblyName] = s; return s; } } [MethodImplAttribute(MethodImplOptions.InternalCall)] public extern static String CreateQualifiedName(String assemblyName, String typeName); public virtual MethodInfo EntryPoint { get { RuntimeMethodHandle methodHandle = nGetEntryPoint(); if (!methodHandle.IsNullHandle()) return (MethodInfo)RuntimeType.GetMethodBase(methodHandle); return null; } } public static Assembly GetAssembly(Type type) { if (type == null) throw new ArgumentNullException("type"); Module m = type.Module; if (m == null) return null; else return m.Assembly; } Type _Assembly.GetType() { return base.GetType(); } public virtual Type GetType(String name) { return GetType(name, false, false); } public virtual Type GetType(String name, bool throwOnError) { return GetType(name, throwOnError, false); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Type _GetType(String name, bool throwOnError, bool ignoreCase); public Type GetType(String name, bool throwOnError, bool ignoreCase) { return InternalAssembly._GetType(name, throwOnError, ignoreCase); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Type[] _GetExportedTypes(); public virtual Type[] GetExportedTypes() { return InternalAssembly._GetExportedTypes(); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly, ResourceScope.Machine | ResourceScope.Assembly)] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual Type[] GetTypes() { Module[] m = nGetModules(true, false); int iNumModules = m.Length; int iFinalLength = 0; StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Type[][] ModuleTypes = new Type[iNumModules][]; for (int i = 0; i < iNumModules; i++) { ModuleTypes[i] = m[i].GetTypesInternal(ref stackMark); iFinalLength += ModuleTypes[i].Length; } int iCurrent = 0; Type[] ret = new Type[iFinalLength]; for (int i = 0; i < iNumModules; i++) { int iLength = ModuleTypes[i].Length; Array.Copy(ModuleTypes[i], 0, ret, iCurrent, iLength); iCurrent += iLength; } return ret; } // Load a resource based on the NameSpace of the type. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual Stream GetManifestResourceStream(Type type, String name) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return GetManifestResourceStream(type, name, false, ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual Stream GetManifestResourceStream(String name) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return GetManifestResourceStream(name, ref stackMark, false); } public Assembly GetSatelliteAssembly(CultureInfo culture) { return InternalGetSatelliteAssembly(culture, null, true); } // Useful for binding to a very specific version of a satellite assembly public Assembly GetSatelliteAssembly(CultureInfo culture, Version version) { return InternalGetSatelliteAssembly(culture, version, true); } public virtual Evidence Evidence { [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] get { return nGetEvidence().Copy(); } } // ISerializable implementation [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public virtual void GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) throw new ArgumentNullException("info"); UnitySerializationHolder.GetUnitySerializationInfo(info, UnitySerializationHolder.AssemblyUnity, this.FullName, this); } internal bool AptcaCheck(Assembly sourceAssembly) { return AssemblyHandle.AptcaCheck(sourceAssembly.AssemblyHandle); } [ComVisible(false)] public Module ManifestModule { get { // We don't need to return the "external" ModuleBuilder because // it is meant to be read-only ModuleHandle manifestModuleHandle = AssemblyHandle.GetManifestModule(); if (manifestModuleHandle == null || manifestModuleHandle.IsNullHandle()) return null; return manifestModuleHandle.GetModule(); } } public virtual Object[] GetCustomAttributes(bool inherit) { return CustomAttribute.GetCustomAttributes(this, typeof(object) as RuntimeType); } public virtual Object[] GetCustomAttributes(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); RuntimeType attributeRuntimeType = attributeType.UnderlyingSystemType as RuntimeType; if (attributeRuntimeType == null) throw new ArgumentException(Environment.GetResourceString("Arg_MustBeType"),"attributeType"); return CustomAttribute.GetCustomAttributes(this, attributeRuntimeType); } public virtual bool IsDefined(Type attributeType, bool inherit) { if (attributeType == null) throw new ArgumentNullException("attributeType"); RuntimeType attributeRuntimeType = attributeType.UnderlyingSystemType as RuntimeType; if (attributeRuntimeType == null) throw new ArgumentException(Environment.GetResourceString("Arg_MustBeType"),"caType"); return CustomAttribute.IsDefined(this, attributeRuntimeType); } // Locate an assembly by the name of the file containing the manifest. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly LoadFrom(String assemblyFile) { // The stack mark is used for MDA filtering StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, null, // securityEvidence null, // hashValue AssemblyHashAlgorithm.None, false, // forIntrospection ref stackMark); } // Locate an assembly for reflection by the name of the file containing the manifest. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly ReflectionOnlyLoadFrom(String assemblyFile) { // The stack mark is ingored for ReflectionOnlyLoadFrom StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, null, //securityEvidence null, //hashValue AssemblyHashAlgorithm.None, true, //forIntrospection ref stackMark); } // Evidence is protected in Assembly.Load() [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly LoadFrom(String assemblyFile, Evidence securityEvidence) { // The stack mark is used for MDA filtering StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, securityEvidence, null, // hashValue AssemblyHashAlgorithm.None, false, // forIntrospection ref stackMark); } // Evidence is protected in Assembly.Load() [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly LoadFrom(String assemblyFile, Evidence securityEvidence, byte[] hashValue, AssemblyHashAlgorithm hashAlgorithm) { // The stack mark is used for MDA filtering StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoadFrom(assemblyFile, securityEvidence, hashValue, hashAlgorithm, false, ref stackMark); } private static Assembly InternalLoadFrom(String assemblyFile, Evidence securityEvidence, byte[] hashValue, AssemblyHashAlgorithm hashAlgorithm, bool forIntrospection, ref StackCrawlMark stackMark) { if (assemblyFile == null) throw new ArgumentNullException("assemblyFile"); AssemblyName an = new AssemblyName(); an.CodeBase = assemblyFile; an.SetHashControl(hashValue, hashAlgorithm); return InternalLoad(an, securityEvidence, ref stackMark, forIntrospection); } // Locate an assembly by the long form of the assembly name. // eg. "Toolbox.dll, version=1.1.10.1220, locale=en, publickey=1234567890123456789012345678901234567890" [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly Load(String assemblyString) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyString, null, ref stackMark, false); } // Locate an assembly for reflection by the long form of the assembly name. // eg. "Toolbox.dll, version=1.1.10.1220, locale=en, publickey=1234567890123456789012345678901234567890" // [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly ReflectionOnlyLoad(String assemblyString) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyString, null, ref stackMark, true /*forIntrospection*/); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly Load(String assemblyString, Evidence assemblySecurity) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyString, assemblySecurity, ref stackMark, false); } // Locate an assembly by its name. The name can be strong or // weak. The assembly is loaded into the domain of the caller. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(AssemblyName assemblyRef) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyRef, null, ref stackMark, false); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(AssemblyName assemblyRef, Evidence assemblySecurity) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return InternalLoad(assemblyRef, assemblySecurity, ref stackMark, false); } // used by vm [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static unsafe private IntPtr LoadWithPartialNameHack(String partialName, bool cropPublicKey) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Assembly result = null; AssemblyName an = new AssemblyName(partialName); if (!IsSimplyNamed(an)) { if (cropPublicKey) { an.SetPublicKey(null); an.SetPublicKeyToken(null); } AssemblyName GACAssembly = EnumerateCache(an); if(GACAssembly != null) result = InternalLoad(GACAssembly, null, ref stackMark, false); } if (result == null) return (IntPtr)0; return (IntPtr)result.AssemblyHandle.Value; } [Obsolete("This method has been deprecated. Please use Assembly.Load() instead. http://go.microsoft.com/fwlink/?linkid=14202")] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly LoadWithPartialName(String partialName) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return LoadWithPartialNameInternal(partialName, null, ref stackMark); } [Obsolete("This method has been deprecated. Please use Assembly.Load() instead. http://go.microsoft.com/fwlink/?linkid=14202")] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly LoadWithPartialName(String partialName, Evidence securityEvidence) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return LoadWithPartialNameInternal(partialName, securityEvidence, ref stackMark); } static internal Assembly LoadWithPartialNameInternal(String partialName, Evidence securityEvidence, ref StackCrawlMark stackMark) { if (securityEvidence != null) new SecurityPermission( SecurityPermissionFlag.ControlEvidence ).Demand(); Assembly result = null; AssemblyName an = new AssemblyName(partialName); try { result = nLoad(an, null, securityEvidence, null, ref stackMark, true, false); } catch(Exception e) { if (e.IsTransient) throw e; if (IsSimplyNamed(an)) return null; AssemblyName GACAssembly = EnumerateCache(an); if(GACAssembly != null) return InternalLoad(GACAssembly, securityEvidence, ref stackMark, false); } return result; } // To not break compatibility with the V1 _Assembly interface we need to make this // new member ComVisible(false). This should be cleaned up in Whidbey. [ComVisible(false)] public virtual bool ReflectionOnly { get { return nReflection(); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool _nReflection(); internal bool nReflection() { return InternalAssembly._nReflection(); } static private AssemblyName EnumerateCache(AssemblyName partialName) { new SecurityPermission(SecurityPermissionFlag.UnmanagedCode).Assert(); partialName.Version = null; ArrayList a = new ArrayList(); Fusion.ReadCache(a, partialName.FullName, ASM_CACHE.GAC); IEnumerator myEnum = a.GetEnumerator(); AssemblyName ainfoBest = null; CultureInfo refCI = partialName.CultureInfo; while (myEnum.MoveNext()) { AssemblyName ainfo = new AssemblyName((String)myEnum.Current); if (CulturesEqual(refCI, ainfo.CultureInfo)) { if (ainfoBest == null) ainfoBest = ainfo; else { // Choose highest version if (ainfo.Version > ainfoBest.Version) ainfoBest = ainfo; } } } return ainfoBest; } static private bool CulturesEqual(CultureInfo refCI, CultureInfo defCI) { bool defNoCulture = defCI.Equals(CultureInfo.InvariantCulture); // cultured asms aren't allowed to be bound to if // the ref doesn't ask for them specifically if ((refCI == null) || refCI.Equals(CultureInfo.InvariantCulture)) return defNoCulture; if (defNoCulture || ( !defCI.Equals(refCI) )) return false; return true; } static private bool IsSimplyNamed(AssemblyName partialName) { byte[] pk = partialName.GetPublicKeyToken(); if ((pk != null) && (pk.Length == 0)) return true; pk = partialName.GetPublicKey(); if ((pk != null) && (pk.Length == 0)) return true; return false; } // Loads the assembly with a COFF based IMAGE containing // an emitted assembly. The assembly is loaded into the domain // of the caller. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(byte[] rawAssembly) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, null, // symbol store null, // evidence ref stackMark, false // fIntrospection ); } // Loads the assembly for reflection with a COFF based IMAGE containing // an emitted assembly. The assembly is loaded into the domain // of the caller. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly ReflectionOnlyLoad(byte[] rawAssembly) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, null, // symbol store null, // evidence ref stackMark, true // fIntrospection ); } // Loads the assembly with a COFF based IMAGE containing // an emitted assembly. The assembly is loaded into the domain // of the caller. The second parameter is the raw bytes // representing the symbol store that matches the assembly. [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(byte[] rawAssembly, byte[] rawSymbolStore) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, rawSymbolStore, null, // evidence ref stackMark, false // fIntrospection ); } [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.ControlEvidence)] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable static public Assembly Load(byte[] rawAssembly, byte[] rawSymbolStore, Evidence securityEvidence) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nLoadImage(rawAssembly, rawSymbolStore, securityEvidence, ref stackMark, false // fIntrospection ); } static public Assembly LoadFile(String path) { new FileIOPermission(FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read, path).Demand(); return nLoadFile(path, null); } [SecurityPermissionAttribute(SecurityAction.Demand, Flags=SecurityPermissionFlag.ControlEvidence)] static public Assembly LoadFile(String path, Evidence securityEvidence) { new FileIOPermission(FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read, path).Demand(); return nLoadFile(path, securityEvidence); } public Module LoadModule(String moduleName, byte[] rawModule) { return nLoadModule(moduleName, rawModule, null, Evidence); // does a ControlEvidence demand } public Module LoadModule(String moduleName, byte[] rawModule, byte[] rawSymbolStore) { return nLoadModule(moduleName, rawModule, rawSymbolStore, Evidence); // does a ControlEvidence demand } // // Locates a type from this assembly and creates an instance of it using // the system activator. // public Object CreateInstance(String typeName) { return CreateInstance(typeName, false, // ignore case BindingFlags.Public | BindingFlags.Instance, null, // binder null, // args null, // culture null); // activation attributes } public Object CreateInstance(String typeName, bool ignoreCase) { return CreateInstance(typeName, ignoreCase, BindingFlags.Public | BindingFlags.Instance, null, // binder null, // args null, // culture null); // activation attributes } public Object CreateInstance(String typeName, bool ignoreCase, BindingFlags bindingAttr, Binder binder, Object[] args, CultureInfo culture, Object[] activationAttributes) { Type t = GetType(typeName, false, ignoreCase); if (t == null) return null; return Activator.CreateInstance(t, bindingAttr, binder, args, culture, activationAttributes); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetLoadedModules() { return nGetModules(false, false); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetLoadedModules(bool getResourceModules) { return nGetModules(false, getResourceModules); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetModules() { return nGetModules(true, false); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public Module[] GetModules(bool getResourceModules) { return nGetModules(true, getResourceModules); } // Returns the module in this assembly with name 'name' [MethodImplAttribute(MethodImplOptions.InternalCall)] [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] internal extern Module _GetModule(String name); public Module GetModule(String name) { return GetModuleInternal(name); } internal virtual Module GetModuleInternal(String name) { return InternalAssembly._GetModule(name); } // Returns the file in the File table of the manifest that matches the // given name. (Name should not include path.) [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public virtual FileStream GetFile(String name) { Module m = GetModule(name); if (m == null) return null; return new FileStream(m.InternalGetFullyQualifiedName(), FileMode.Open, FileAccess.Read, FileShare.Read); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public virtual FileStream[] GetFiles() { return GetFiles(false); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] [ResourceConsumption(ResourceScope.Machine | ResourceScope.Assembly)] public virtual FileStream[] GetFiles(bool getResourceModules) { Module[] m = nGetModules(true, getResourceModules); int iLength = m.Length; FileStream[] fs = new FileStream[iLength]; for(int i = 0; i < iLength; i++) fs[i] = new FileStream(m[i].InternalGetFullyQualifiedName(), FileMode.Open, FileAccess.Read, FileShare.Read); return fs; } // Returns the names of all the resources public virtual String[] GetManifestResourceNames() { return nGetManifestResourceNames(); } // Returns the names of all the resources [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String[] _nGetManifestResourceNames(); internal String[] nGetManifestResourceNames() { return InternalAssembly._nGetManifestResourceNames(); } /* * Get the assembly that the current code is running from. */ [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly GetExecutingAssembly() { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; return nGetExecutingAssembly(ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public static Assembly GetCallingAssembly() { StackCrawlMark stackMark = StackCrawlMark.LookForMyCallersCaller; return nGetExecutingAssembly(ref stackMark); } public static Assembly GetEntryAssembly() { AppDomainManager domainManager = AppDomain.CurrentDomain.DomainManager; if (domainManager == null) domainManager = new AppDomainManager(); return domainManager.EntryAssembly; } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern AssemblyName[] _GetReferencedAssemblies(); public AssemblyName[] GetReferencedAssemblies() { return InternalAssembly._GetReferencedAssemblies(); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public virtual ManifestResourceInfo GetManifestResourceInfo(String resourceName) { Assembly assemblyRef; String fileName; StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; int location = nGetManifestResourceInfo(resourceName, out assemblyRef, out fileName, ref stackMark); if (location == -1) return null; else return new ManifestResourceInfo(assemblyRef, fileName, (ResourceLocation) location); } public override String ToString() { String displayName = FullName; if (displayName == null) return base.ToString(); else return displayName; } public virtual String Location { get { String location = GetLocation(); if (location != null) new FileIOPermission( FileIOPermissionAccess.PathDiscovery, location ).Demand(); return location; } } // To not break compatibility with the V1 _Assembly interface we need to make this // new member ComVisible(false). This should be cleaned up in Whidbey. [ComVisible(false)] public virtual String ImageRuntimeVersion { get{ return nGetImageRuntimeVersion(); } } /* Returns true if the assembly was loaded from the global assembly cache. */ public bool GlobalAssemblyCache { get { return nGlobalAssemblyCache(); } } [ComVisible(false)] public Int64 HostContext { get { return GetHostContext(); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Int64 _GetHostContext(); private Int64 GetHostContext() { return InternalAssembly._GetHostContext(); } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] internal static String VerifyCodeBase(String codebase) { if(codebase == null) return null; int len = codebase.Length; if (len == 0) return null; int j = codebase.IndexOf(':'); // Check to see if the url has a prefix if( (j != -1) && (j+2 < len) && ((codebase[j+1] == '/') || (codebase[j+1] == '\\')) && ((codebase[j+2] == '/') || (codebase[j+2] == '\\')) ) return codebase; #if !PLATFORM_UNIX else if ((len > 2) && (codebase[0] == '\\') && (codebase[1] == '\\')) return "file://" + codebase; else return "file:///" + Path.GetFullPathInternal( codebase ); #else else return "file://" + Path.GetFullPathInternal( codebase ); #endif // !PLATFORM_UNIX } internal virtual Stream GetManifestResourceStream(Type type, String name, bool skipSecurityCheck, ref StackCrawlMark stackMark) { StringBuilder sb = new StringBuilder(); if(type == null) { if (name == null) throw new ArgumentNullException("type"); } else { String nameSpace = type.Namespace; if(nameSpace != null) { sb.Append(nameSpace); if(name != null) sb.Append(Type.Delimiter); } } if(name != null) sb.Append(name); return GetManifestResourceStream(sb.ToString(), ref stackMark, skipSecurityCheck); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Module _nLoadModule(String moduleName, byte[] rawModule, byte[] rawSymbolStore, Evidence securityEvidence); private Module nLoadModule(String moduleName, byte[] rawModule, byte[] rawSymbolStore, Evidence securityEvidence) { return InternalAssembly._nLoadModule(moduleName, rawModule, rawSymbolStore, securityEvidence); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool _nGlobalAssemblyCache(); private bool nGlobalAssemblyCache() { return InternalAssembly._nGlobalAssemblyCache(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetImageRuntimeVersion(); private String nGetImageRuntimeVersion() { return InternalAssembly._nGetImageRuntimeVersion(); } internal Assembly() { } // Create a new module in which to emit code. This module will not contain the manifest. [MethodImplAttribute(MethodImplOptions.InternalCall)] static private extern Module _nDefineDynamicModule(Assembly containingAssembly, bool emitSymbolInfo, String filename, ref StackCrawlMark stackMark); static internal Module nDefineDynamicModule(Assembly containingAssembly, bool emitSymbolInfo, String filename, ref StackCrawlMark stackMark) { return _nDefineDynamicModule(containingAssembly.InternalAssembly, emitSymbolInfo, filename, ref stackMark); } // The following functions are native helpers for creating on-disk manifest [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nPrepareForSavingManifestToDisk(Module assemblyModule); // module to contain assembly information if assembly is embedded internal void nPrepareForSavingManifestToDisk(Module assemblyModule) { if (assemblyModule != null) assemblyModule = assemblyModule.InternalModule; InternalAssembly._nPrepareForSavingManifestToDisk(assemblyModule); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nSaveToFileList(String strFileName); internal int nSaveToFileList(String strFileName) { return InternalAssembly._nSaveToFileList(strFileName); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nSetHashValue(int tkFile, String strFullFileName); internal int nSetHashValue(int tkFile, String strFullFileName) { return InternalAssembly._nSetHashValue(tkFile, strFullFileName); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nSaveExportedType(String strComTypeName, int tkAssemblyRef, int tkTypeDef, TypeAttributes flags); internal int nSaveExportedType(String strComTypeName, int tkAssemblyRef, int tkTypeDef, TypeAttributes flags) { return InternalAssembly._nSaveExportedType(strComTypeName, tkAssemblyRef, tkTypeDef, flags); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nSavePermissionRequests(byte[] required, byte[] optional, byte[] refused); internal void nSavePermissionRequests(byte[] required, byte[] optional, byte[] refused) { InternalAssembly._nSavePermissionRequests(required, optional, refused); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nSaveManifestToDisk( String strFileName, int entryPoint, int fileKind, int portableExecutableKind, int ImageFileMachine); internal void nSaveManifestToDisk( String strFileName, int entryPoint, int fileKind, int portableExecutableKind, int ImageFileMachine) { InternalAssembly._nSaveManifestToDisk( strFileName, entryPoint, fileKind, portableExecutableKind, ImageFileMachine); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nAddFileToInMemoryFileList(String strFileName, Module module); internal int nAddFileToInMemoryFileList(String strFileName, Module module) { if (module != null) module = module.InternalModule; return InternalAssembly._nAddFileToInMemoryFileList(strFileName, module); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Module _nGetOnDiskAssemblyModule(); internal Module nGetOnDiskAssemblyModule() { return InternalAssembly._nGetOnDiskAssemblyModule(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern Module _nGetInMemoryAssemblyModule(); internal Module nGetInMemoryAssemblyModule() { return InternalAssembly._nGetInMemoryAssemblyModule(); } #if !FEATURE_PAL // Functions for defining unmanaged resources. [MethodImplAttribute(MethodImplOptions.InternalCall)] static internal extern String nDefineVersionInfoResource(String filename, String title, String iconFilename, String description, String copyright, String trademark, String company, String product, String productVersion, String fileVersion, int lcid, bool isDll); #endif // !FEATURE_PAL private static void DecodeSerializedEvidence( Evidence evidence, byte[] serializedEvidence ) { BinaryFormatter formatter = new BinaryFormatter(); Evidence asmEvidence = null; PermissionSet permSet = new PermissionSet( false ); permSet.SetPermission( new SecurityPermission( SecurityPermissionFlag.SerializationFormatter ) ); permSet.PermitOnly(); permSet.Assert(); try { using(MemoryStream ms = new MemoryStream( serializedEvidence )) asmEvidence = (Evidence)formatter.Deserialize( ms ); } catch { } if (asmEvidence != null) { IEnumerator enumerator = asmEvidence.GetAssemblyEnumerator(); while (enumerator.MoveNext()) { Object obj = enumerator.Current; evidence.AddAssembly( obj ); } } } #if !FEATURE_PAL private static void AddX509Certificate( Evidence evidence, byte[] cert ) { evidence.AddHost(new Publisher(new System.Security.Cryptography.X509Certificates.X509Certificate(cert))); } #endif // !FEATURE_PAL private static void AddStrongName(Evidence evidence, byte[] blob, String strSimpleName, int major, int minor, int build, int revision, Assembly assembly) { StrongName sn = new StrongName(new StrongNamePublicKeyBlob(blob), strSimpleName, new Version(major, minor, build, revision), assembly); evidence.AddHost(sn); } private static Evidence CreateSecurityIdentity(Assembly asm, String strUrl, int zone, byte[] cert, byte[] publicKeyBlob, String strSimpleName, int major, int minor, int build, int revision, byte[] serializedEvidence, Evidence additionalEvidence) { Evidence evidence = new Evidence(); if (zone != -1) evidence.AddHost( new Zone((SecurityZone)zone) ); if (strUrl != null) { evidence.AddHost( new Url(strUrl, true) ); // Only create a site piece of evidence if we are not loading from a file. if (String.Compare( strUrl, 0, s_localFilePrefix, 0, 5, StringComparison.OrdinalIgnoreCase) != 0) evidence.AddHost( Site.CreateFromUrl( strUrl ) ); } #if !FEATURE_PAL if (cert != null) AddX509Certificate( evidence, cert ); #endif // !FEATURE_PAL // Determine if it's in the GAC and add some evidence about it if(asm != null && System.Runtime.InteropServices.RuntimeEnvironment.FromGlobalAccessCache(asm)) evidence.AddHost( new GacInstalled() ); // This code was moved to a different function because: // 1) it is rarely called so we should only JIT it if we need it. // 2) it references lots of classes that otherwise aren't loaded. if (serializedEvidence != null) DecodeSerializedEvidence( evidence, serializedEvidence ); if ((publicKeyBlob != null) && (publicKeyBlob.Length != 0)) { AddStrongName(evidence, publicKeyBlob, strSimpleName, major, minor, build, revision, asm); } #if !FEATURE_PAL if (asm != null && !asm.nIsDynamic()) evidence.AddHost(new Hash(asm)); #endif // !FEATURE_PAL // If the host (caller of Assembly.Load) provided evidence, merge it // with the evidence we've just created. The host evidence takes // priority. if (additionalEvidence != null) evidence.MergeWithNoDuplicates(additionalEvidence); if (asm != null) { // The host might want to modify the evidence of the assembly through // the HostSecurityManager provided in AppDomainManager, so take that into account. HostSecurityManager securityManager = AppDomain.CurrentDomain.HostSecurityManager; if ((securityManager.Flags & HostSecurityManagerOptions.HostAssemblyEvidence) == HostSecurityManagerOptions.HostAssemblyEvidence) return securityManager.ProvideAssemblyEvidence(asm, evidence); } return evidence; } ////// Determine if this assembly was loaded from a location under the AppBase for security /// purposes. For instance, strong name bypass is disabled for assemblies considered outside /// the AppBase. /// /// This method is called from the VM: AssemblySecurityDescriptor::WasAssemblyLoadedFromAppBase /// [FileIOPermission(SecurityAction.Assert, Unrestricted = true)] private bool IsAssemblyUnderAppBase() { string assemblyLocation = GetLocation(); // Assemblies that are loaded without a location will be considered part of the app if (String.IsNullOrEmpty(assemblyLocation)) { return true; } // Compare the paths using the FileIOAccess mechanism that FileIOPermission uses internally. If // assemblyAccess is a subset of appbaseAccess (in FileIOPermission terms, appBaseAccess is the // demand set, and assemblyAccess is the grant set) that means assembly must be under the AppBase. FileIOAccess assemblyAccess = new FileIOAccess(Path.GetFullPathInternal(assemblyLocation)); FileIOAccess appBaseAccess = new FileIOAccess(Path.GetFullPathInternal(AppDomain.CurrentDomain.BaseDirectory)); return assemblyAccess.IsSubsetOf(appBaseAccess); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern bool IsStrongNameVerified(); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern static Assembly nGetExecutingAssembly(ref StackCrawlMark stackMark); internal unsafe virtual Stream GetManifestResourceStream(String name, ref StackCrawlMark stackMark, bool skipSecurityCheck) { ulong length = 0; byte* pbInMemoryResource = GetResource(name, out length, ref stackMark, skipSecurityCheck); if (pbInMemoryResource != null) { //Console.WriteLine("Creating an unmanaged memory stream of length "+length); if (length > Int64.MaxValue) throw new NotImplementedException(Environment.GetResourceString("NotImplemented_ResourcesLongerThan2^63")); //For cases where we're loading an embedded resource from an assembly, // in V1 we do not have any serious lifetime issues with the // UnmanagedMemoryStream. If the Stream is only used // in the AppDomain that contains the assembly, then if that AppDomain // is unloaded, we will collect all of the objects in the AppDomain first // before unloading assemblies. If the Stream is shared across AppDomains, // then the original AppDomain was unloaded, accesses to this Stream will // throw an exception saying the appdomain was unloaded. This is // guaranteed be EE AppDomain goo. And for shared assemblies like // mscorlib, their lifetime is the lifetime of the process, so the // assembly will NOT be unloaded, so the resource will always be in memory. return new UnmanagedMemoryStream(pbInMemoryResource, (long)length, (long)length, FileAccess.Read, true); } //Console.WriteLine("GetManifestResourceStream: Blob "+name+" not found..."); return null; } internal Version GetVersion() { int majorVer, minorVer, build, revision; nGetVersion(out majorVer, out minorVer, out build, out revision); return new Version (majorVer, minorVer, build, revision); } internal CultureInfo GetLocale() { String locale = nGetLocale(); if (locale == null) return CultureInfo.InvariantCulture; return new CultureInfo(locale); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetLocale(); private String nGetLocale() { return InternalAssembly._nGetLocale(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nGetVersion(out int majVer, out int minVer, out int buildNum, out int revNum); internal void nGetVersion(out int majVer, out int minVer, out int buildNum, out int revNum) { InternalAssembly._nGetVersion(out majVer, out minVer, out buildNum, out revNum); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool _nIsDynamic(); internal bool nIsDynamic() { return InternalAssembly._nIsDynamic(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern int _nGetManifestResourceInfo(String resourceName, out Assembly assemblyRef, out String fileName, ref StackCrawlMark stackMark); private int nGetManifestResourceInfo(String resourceName, out Assembly assemblyRef, out String fileName, ref StackCrawlMark stackMark) { return InternalAssembly._nGetManifestResourceInfo( resourceName, out assemblyRef, out fileName, ref stackMark); } private void VerifyCodeBaseDiscovery(String codeBase) { if ((codeBase != null) && (String.Compare( codeBase, 0, s_localFilePrefix, 0, 5, StringComparison.OrdinalIgnoreCase) == 0)) { System.Security.Util.URLString urlString = new System.Security.Util.URLString( codeBase, true ); new FileIOPermission( FileIOPermissionAccess.PathDiscovery, urlString.GetFileName() ).Demand(); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _GetLocation(); internal String GetLocation() { return InternalAssembly._GetLocation(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern byte[] _nGetPublicKey(); internal byte[] nGetPublicKey() { return InternalAssembly._nGetPublicKey(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetSimpleName(); internal String nGetSimpleName() { return InternalAssembly._nGetSimpleName(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _nGetCodeBase(bool fCopiedName); internal String nGetCodeBase(bool fCopiedName) { return InternalAssembly._nGetCodeBase(fCopiedName); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern AssemblyHashAlgorithm _nGetHashAlgorithm(); internal AssemblyHashAlgorithm nGetHashAlgorithm() { return InternalAssembly._nGetHashAlgorithm(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern AssemblyNameFlags _nGetFlags(); internal AssemblyNameFlags nGetFlags() { return InternalAssembly._nGetFlags(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern void _nGetGrantSet(out PermissionSet newGrant, out PermissionSet newDenied); internal void nGetGrantSet(out PermissionSet newGrant, out PermissionSet newDenied) { InternalAssembly._nGetGrantSet(out newGrant, out newDenied); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern String _GetFullName(); internal String GetFullName() { return InternalAssembly._GetFullName(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private unsafe extern void* _nGetEntryPoint(); private unsafe RuntimeMethodHandle nGetEntryPoint() { return new RuntimeMethodHandle(_nGetEntryPoint()); } // GetResource will return a handle to a file (or -1) and set the length. // It will also return a pointer to the resources if they're in memory. [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern Evidence _nGetEvidence(); internal Evidence nGetEvidence() { return InternalAssembly._nGetEvidence(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private unsafe extern byte* _GetResource(String resourceName, out ulong length, ref StackCrawlMark stackMark, bool skipSecurityCheck); private unsafe byte* GetResource(String resourceName, out ulong length, ref StackCrawlMark stackMark, bool skipSecurityCheck) { return InternalAssembly._GetResource(resourceName, out length, ref stackMark, skipSecurityCheck); } internal static Assembly InternalLoad(String assemblyString, Evidence assemblySecurity, ref StackCrawlMark stackMark, bool forIntrospection) { if (assemblyString == null) throw new ArgumentNullException("assemblyString"); if ((assemblyString.Length == 0) || (assemblyString[0] == '\0')) throw new ArgumentException(Environment.GetResourceString("Format_StringZeroLength")); AssemblyName an = new AssemblyName(); Assembly assembly = null; an.Name = assemblyString; int hr = an.nInit(out assembly, forIntrospection, true); if (hr == System.__HResults.FUSION_E_INVALID_NAME) { return assembly; } else return InternalLoad(an, assemblySecurity, ref stackMark, forIntrospection); } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] internal static Assembly InternalLoad(AssemblyName assemblyRef, Evidence assemblySecurity, ref StackCrawlMark stackMark, bool forIntrospection) { if (assemblyRef == null) throw new ArgumentNullException("assemblyRef"); assemblyRef = (AssemblyName)assemblyRef.Clone(); if (assemblySecurity != null) new SecurityPermission( SecurityPermissionFlag.ControlEvidence ).Demand(); String codeBase = VerifyCodeBase(assemblyRef.CodeBase); if (codeBase != null) { if (String.Compare( codeBase, 0, s_localFilePrefix, 0, 5, StringComparison.OrdinalIgnoreCase) != 0) { IPermission perm = CreateWebPermission( assemblyRef.EscapedCodeBase ); perm.Demand(); } else { System.Security.Util.URLString urlString = new System.Security.Util.URLString( codeBase, true ); new FileIOPermission( FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read , urlString.GetFileName() ).Demand(); } } return nLoad(assemblyRef, codeBase, assemblySecurity, null, ref stackMark, true, forIntrospection); } // demandFlag: // 0 demand PathDiscovery permission only // 1 demand Read permission only // 2 demand both Read and PathDiscovery // 3 demand Web permission only [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] private static void DemandPermission(String codeBase, bool havePath, int demandFlag) { FileIOPermissionAccess access = FileIOPermissionAccess.PathDiscovery; switch(demandFlag) { case 0: // default break; case 1: access = FileIOPermissionAccess.Read; break; case 2: access = FileIOPermissionAccess.PathDiscovery | FileIOPermissionAccess.Read; break; case 3: IPermission perm = CreateWebPermission(AssemblyName.EscapeCodeBase(codeBase)); perm.Demand(); return; } if (!havePath) { System.Security.Util.URLString urlString = new System.Security.Util.URLString( codeBase, true ); codeBase = urlString.GetFileName(); } codeBase = Path.GetFullPathInternal(codeBase); // canonicalize new FileIOPermission(access, codeBase).Demand(); } [MethodImplAttribute(MethodImplOptions.InternalCall)] private static extern Assembly _nLoad(AssemblyName fileName, String codeBase, Evidence assemblySecurity, Assembly locationHint, ref StackCrawlMark stackMark, bool throwOnFileNotFound, bool forIntrospection); [ResourceExposure(ResourceScope.None)] private static Assembly nLoad(AssemblyName fileName, String codeBase, Evidence assemblySecurity, Assembly locationHint, ref StackCrawlMark stackMark, bool throwOnFileNotFound, bool forIntrospection) { if (locationHint != null) locationHint = locationHint.InternalAssembly; return _nLoad(fileName, codeBase, assemblySecurity, locationHint, ref stackMark, throwOnFileNotFound, forIntrospection); } private static IPermission CreateWebPermission( String codeBase ) { BCLDebug.Assert( codeBase != null, "Must pass in a valid CodeBase" ); Assembly sys = Assembly.Load("System, Version=" + ThisAssembly.Version + ", Culture=neutral, PublicKeyToken=" + AssemblyRef.EcmaPublicKeyToken); Type type = sys.GetType("System.Net.NetworkAccess", true); IPermission retval = null; if (!type.IsEnum || !type.IsVisible) goto Exit; Object[] webArgs = new Object[2]; webArgs[0] = (Enum) Enum.Parse(type, "Connect", true); if (webArgs[0] == null) goto Exit; webArgs[1] = codeBase; type = sys.GetType("System.Net.WebPermission", true); if (!type.IsVisible) goto Exit; retval = (IPermission) Activator.CreateInstance(type, webArgs); Exit: if (retval == null) { BCLDebug.Assert( false, "Unable to create WebPermission" ); throw new ExecutionEngineException(); } return retval; } private Module OnModuleResolveEvent(String moduleName) { ModuleResolveEventHandler moduleResolve =ModuleResolveEvent; if (moduleResolve == null) return null; Delegate[] ds = moduleResolve.GetInvocationList(); int len = ds.Length; for (int i = 0; i < len; i++) { Module ret = ((ModuleResolveEventHandler) ds[i])(this, new ResolveEventArgs(moduleName)); if (ret != null) return ret.InternalModule; } return null; } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable internal Assembly InternalGetSatelliteAssembly(CultureInfo culture, Version version, bool throwOnFileNotFound) { if (culture == null) throw new ArgumentNullException("culture"); AssemblyName an = new AssemblyName(); an.SetPublicKey(nGetPublicKey()); an.Flags = nGetFlags() | AssemblyNameFlags.PublicKey; if (version == null) an.Version = GetVersion(); else an.Version = version; an.CultureInfo = culture; an.Name = nGetSimpleName() + ".resources"; StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; Assembly a = nLoad(an, null, null, this, ref stackMark, throwOnFileNotFound, false); if (a == this) { throw new FileNotFoundException(String.Format(culture, Environment.GetResourceString("IO.FileNotFound_FileName"), an.Name)); } return a; } internal InternalCache Cache { get { // This grabs an internal copy of m_cachedData and uses // that instead of looking at m_cachedData directly because // the cache may get cleared asynchronously. This prevents // us from having to take a lock. InternalCache cache = m_cachedData; if (cache == null) { cache = new InternalCache("Assembly"); m_cachedData = cache; GC.ClearCache += new ClearCacheHandler(OnCacheClear); } return cache; } } internal void OnCacheClear(Object sender, ClearCacheEventArgs cacheEventArgs) { m_cachedData = null; } [MethodImplAttribute(MethodImplOptions.InternalCall)] static internal extern Assembly nLoadFile(String path, Evidence evidence); [MethodImplAttribute(MethodImplOptions.InternalCall)] static internal extern Assembly nLoadImage(byte[] rawAssembly, byte[] rawSymbolStore, Evidence evidence, ref StackCrawlMark stackMark, bool fIntrospection); // Add an entry to assembly's manifestResource table for a stand alone resource. [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void _nAddStandAloneResource(String strName, String strFileName, String strFullFileName, int attribute); internal void nAddStandAloneResource(String strName, String strFileName, String strFullFileName, int attribute) { InternalAssembly._nAddStandAloneResource(strName, strFileName, strFullFileName, attribute); } [ResourceExposure(ResourceScope.Machine | ResourceScope.Assembly)] internal virtual Module[] nGetModules(bool loadIfNotFound, bool getResourceModules) { return InternalAssembly._nGetModules(loadIfNotFound, getResourceModules); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal extern Module[] _nGetModules(bool loadIfNotFound, bool getResourceModules); internal ProcessorArchitecture ComputeProcArchIndex() { PortableExecutableKinds pek; ImageFileMachine ifm; Module manifestModule = ManifestModule; if(manifestModule != null) { if(manifestModule.MDStreamVersion > 0x10000) { ManifestModule.GetPEKind(out pek, out ifm); if((pek & System.Reflection.PortableExecutableKinds.PE32Plus) == System.Reflection.PortableExecutableKinds.PE32Plus) { switch(ifm) { case System.Reflection.ImageFileMachine.IA64: return ProcessorArchitecture.IA64; case System.Reflection.ImageFileMachine.AMD64: return ProcessorArchitecture.Amd64; case System.Reflection.ImageFileMachine.I386: if ((pek & System.Reflection.PortableExecutableKinds.ILOnly) == System.Reflection.PortableExecutableKinds.ILOnly) return ProcessorArchitecture.MSIL; break; } } else { if(ifm == System.Reflection.ImageFileMachine.I386) { if((pek & System.Reflection.PortableExecutableKinds.Required32Bit) == System.Reflection.PortableExecutableKinds.Required32Bit) return ProcessorArchitecture.X86; if((pek & System.Reflection.PortableExecutableKinds.ILOnly) == System.Reflection.PortableExecutableKinds.ILOnly) return ProcessorArchitecture.MSIL; return ProcessorArchitecture.X86; } } } } return ProcessorArchitecture.None; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
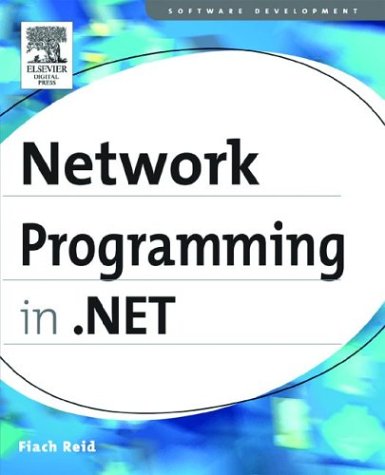
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ComEventsInfo.cs
- ContextMenuStrip.cs
- CssClassPropertyAttribute.cs
- IconHelper.cs
- HtmlContainerControl.cs
- HyperLinkField.cs
- AutomationElementIdentifiers.cs
- SiteMap.cs
- ActivityExecutionFilter.cs
- _PooledStream.cs
- URLBuilder.cs
- XmlStringTable.cs
- RangeValidator.cs
- WsdlInspector.cs
- SurrogateSelector.cs
- CursorConverter.cs
- EntityKeyElement.cs
- OwnerDrawPropertyBag.cs
- LoginName.cs
- UriScheme.cs
- OdbcCommand.cs
- SspiWrapper.cs
- embossbitmapeffect.cs
- URLIdentityPermission.cs
- AtomEntry.cs
- Dispatcher.cs
- ObfuscateAssemblyAttribute.cs
- _HeaderInfo.cs
- StateChangeEvent.cs
- HttpListenerRequest.cs
- TreeViewEvent.cs
- ImageKeyConverter.cs
- CultureInfoConverter.cs
- ListViewGroupConverter.cs
- XmlEntity.cs
- BindStream.cs
- MessageQueuePermissionAttribute.cs
- SqlDataSourceWizardForm.cs
- XmlSchemaExporter.cs
- ExpandSegment.cs
- ClientScriptItem.cs
- Scripts.cs
- ValidationRule.cs
- XPathExpr.cs
- PageTextBox.cs
- ContractMapping.cs
- SqlCharStream.cs
- StringValidator.cs
- DataGridViewElement.cs
- CheckBoxBaseAdapter.cs
- ManipulationCompletedEventArgs.cs
- TemplateApplicationHelper.cs
- UriScheme.cs
- Camera.cs
- RewritingProcessor.cs
- PlaceHolder.cs
- ToolStripItemClickedEventArgs.cs
- SQLResource.cs
- CaseStatementSlot.cs
- FontWeights.cs
- _SafeNetHandles.cs
- OutputCacheModule.cs
- OdbcTransaction.cs
- DataKeyCollection.cs
- AnnotationResourceCollection.cs
- ComplexLine.cs
- TemplatePropertyEntry.cs
- XmlSchemaComplexContentRestriction.cs
- DiagnosticsConfiguration.cs
- DbCommandDefinition.cs
- SafeFindHandle.cs
- EntityKeyElement.cs
- Application.cs
- PrintControllerWithStatusDialog.cs
- TextServicesPropertyRanges.cs
- BmpBitmapDecoder.cs
- Crypto.cs
- TypeHelpers.cs
- DataMisalignedException.cs
- CriticalHandle.cs
- SwitchAttribute.cs
- BaseTemplateBuildProvider.cs
- HostDesigntimeLicenseContext.cs
- UnsafeMethods.cs
- StatusBarAutomationPeer.cs
- OneOfTypeConst.cs
- SafeIUnknown.cs
- CredentialCache.cs
- ConfigurationException.cs
- COM2AboutBoxPropertyDescriptor.cs
- PointCollection.cs
- FlowSwitch.cs
- HideDisabledControlAdapter.cs
- ConfigXmlText.cs
- FixedElement.cs
- CleanUpVirtualizedItemEventArgs.cs
- DataSourceControl.cs
- CanExpandCollapseAllConverter.cs
- ContentType.cs
- XPathAncestorIterator.cs