Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / NavigationPropertySingletonExpression.cs / 3 / NavigationPropertySingletonExpression.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a navigation to a singleton property. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Linq; using System.Linq.Expressions; using System.Collections.Generic; using System.Diagnostics; ///ResourceSet Expression internal class NavigationPropertySingletonExpression : ResourceExpression { ///source expression private readonly Expression source; ///property member name private readonly Expression memberExpression; ////// Creates a NavigationPropertySingletonExpression expression /// /// the return type of the expression /// the source expression /// property member name /// expand paths for resource set /// custom query options for resourcse set internal NavigationPropertySingletonExpression(Type type, Expression source, Expression memberExpression, ListexpandPaths, Dictionary customQueryOptions) : base((ExpressionType)ResourceExpressionType.ResourceNavigationPropertySingleton, type, expandPaths, customQueryOptions) { this.source = source; this.memberExpression = memberExpression; } /// /// Gets the member expression. /// internal MemberExpression MemberExpression { get { return (MemberExpression)this.memberExpression; } } ////// Gets the source expression. /// internal Expression Source { get { return this.source; } } ////// The resource type of the singe instance produced by this singleton navigation. /// internal override Type ResourceType { get { return this.memberExpression.Type; } } ////// Singleton navigation properties always produce at most 1 result /// internal override bool IsSingleton { get { return true; } } ////// Does Singleton navigation have query options. /// internal override bool HasQueryOptions { get { return this.ExpandPaths.Count > 0 || this.CustomQueryOptions.Count > 0; } } ////// Cast changes the type of the ResourceExpression /// /// new type ///new NavigationPropertySingletonExpression internal override ResourceExpression Cast(Type type) { NavigationPropertySingletonExpression rse = new NavigationPropertySingletonExpression( type, this.source, this.MemberExpression, this.ExpandPaths.ToList(), this.CustomQueryOptions.ToDictionary(kvp => kvp.Key, kvp => kvp.Value)); return rse; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a navigation to a singleton property. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Linq; using System.Linq.Expressions; using System.Collections.Generic; using System.Diagnostics; ///ResourceSet Expression internal class NavigationPropertySingletonExpression : ResourceExpression { ///source expression private readonly Expression source; ///property member name private readonly Expression memberExpression; ////// Creates a NavigationPropertySingletonExpression expression /// /// the return type of the expression /// the source expression /// property member name /// expand paths for resource set /// custom query options for resourcse set internal NavigationPropertySingletonExpression(Type type, Expression source, Expression memberExpression, ListexpandPaths, Dictionary customQueryOptions) : base((ExpressionType)ResourceExpressionType.ResourceNavigationPropertySingleton, type, expandPaths, customQueryOptions) { this.source = source; this.memberExpression = memberExpression; } /// /// Gets the member expression. /// internal MemberExpression MemberExpression { get { return (MemberExpression)this.memberExpression; } } ////// Gets the source expression. /// internal Expression Source { get { return this.source; } } ////// The resource type of the singe instance produced by this singleton navigation. /// internal override Type ResourceType { get { return this.memberExpression.Type; } } ////// Singleton navigation properties always produce at most 1 result /// internal override bool IsSingleton { get { return true; } } ////// Does Singleton navigation have query options. /// internal override bool HasQueryOptions { get { return this.ExpandPaths.Count > 0 || this.CustomQueryOptions.Count > 0; } } ////// Cast changes the type of the ResourceExpression /// /// new type ///new NavigationPropertySingletonExpression internal override ResourceExpression Cast(Type type) { NavigationPropertySingletonExpression rse = new NavigationPropertySingletonExpression( type, this.source, this.MemberExpression, this.ExpandPaths.ToList(), this.CustomQueryOptions.ToDictionary(kvp => kvp.Key, kvp => kvp.Value)); return rse; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
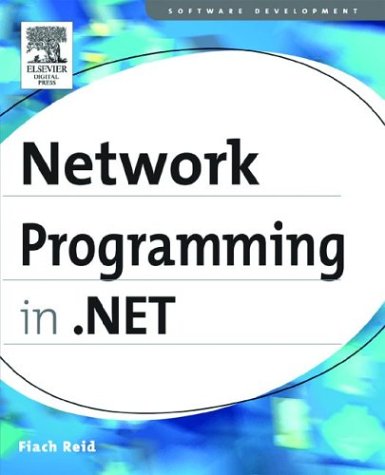
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ManagedFilter.cs
- KeyInterop.cs
- basenumberconverter.cs
- ExtenderProvidedPropertyAttribute.cs
- RtType.cs
- DateBoldEvent.cs
- M3DUtil.cs
- SmiMetaData.cs
- ISFTagAndGuidCache.cs
- EnvelopedSignatureTransform.cs
- DescendantOverDescendantQuery.cs
- IsolatedStorageFile.cs
- SQLSingleStorage.cs
- SpecialFolderEnumConverter.cs
- odbcmetadatacolumnnames.cs
- FileDialog_Vista.cs
- Container.cs
- DragDrop.cs
- SqlUdtInfo.cs
- DataServiceEntityAttribute.cs
- CompilationSection.cs
- BuildManager.cs
- EntityCommandDefinition.cs
- StorageAssociationTypeMapping.cs
- WizardPanelChangingEventArgs.cs
- WpfSharedXamlSchemaContext.cs
- RequestCachePolicy.cs
- InteropAutomationProvider.cs
- UIPermission.cs
- ExpressionConverter.cs
- EventLogTraceListener.cs
- InstanceHandleConflictException.cs
- Calendar.cs
- TextEditorCharacters.cs
- OleDbSchemaGuid.cs
- DeclarativeConditionsCollection.cs
- EnumerableCollectionView.cs
- MetadataSource.cs
- ToolStripHighContrastRenderer.cs
- TcpClientSocketManager.cs
- PersistenceTypeAttribute.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- HyperLink.cs
- BitArray.cs
- Events.cs
- PerformanceCounterLib.cs
- ZoneButton.cs
- ExtensibleClassFactory.cs
- RequestCacheValidator.cs
- Helpers.cs
- StylusPlugInCollection.cs
- ToolTipService.cs
- FunctionParameter.cs
- ProviderSettings.cs
- TableNameAttribute.cs
- HostUtils.cs
- NetNamedPipeBindingCollectionElement.cs
- XmlSchemaDocumentation.cs
- DataGridViewColumnEventArgs.cs
- HttpClientCertificate.cs
- AsmxEndpointPickerExtension.cs
- PeerNameRecordCollection.cs
- PropertySet.cs
- Int16KeyFrameCollection.cs
- SrgsToken.cs
- PublisherIdentityPermission.cs
- DetailsViewUpdateEventArgs.cs
- EpmTargetPathSegment.cs
- ClassImporter.cs
- MatrixStack.cs
- TreeViewImageIndexConverter.cs
- AlignmentXValidation.cs
- Properties.cs
- PrincipalPermissionMode.cs
- RectangleGeometry.cs
- CodeTypeDeclaration.cs
- bidPrivateBase.cs
- ProtocolInformationWriter.cs
- InputProcessorProfilesLoader.cs
- StackBuilderSink.cs
- ToolboxCategory.cs
- CompilationSection.cs
- Token.cs
- DataGridViewCellFormattingEventArgs.cs
- ResourceAssociationType.cs
- ComponentResourceManager.cs
- WaitHandle.cs
- WmlListAdapter.cs
- HwndMouseInputProvider.cs
- hebrewshape.cs
- SecurityContextCookieSerializer.cs
- DataPagerFieldItem.cs
- TickBar.cs
- ColorConverter.cs
- XamlReaderHelper.cs
- WebPartConnectionsCancelVerb.cs
- BitmapEffectInput.cs
- FileDialog_Vista.cs
- SendOperation.cs
- PathFigureCollection.cs