Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Routing / System / ServiceModel / Routing / SendOperation.cs / 1305376 / SendOperation.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Routing { using System; using System.Collections.Generic; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.Transactions; using System.Runtime; using System.Configuration; class SendOperation { ListendpointTraits; int currentIndex; bool sent; Dictionary exceptions; OperationContext operationContext; Type routerContract; public SendOperation(IEnumerable endpoints, Type routerContract, OperationContext operationContext) { this.operationContext = operationContext; this.routerContract = routerContract; this.endpointTraits = new List (); foreach (ServiceEndpoint endpoint in endpoints) { this.endpointTraits.Add(new RoutingEndpointTrait(routerContract, endpoint, operationContext)); } if (this.endpointTraits.Count == 0) { throw FxTrace.Exception.AsError(new ConfigurationErrorsException(SR.BackupListEmpty)); } } public RoutingEndpointTrait CurrentEndpoint { get { Fx.Assert(this.currentIndex < this.endpointTraits.Count, "CurrentEndpoint should not be accessed after TryMoveToAlternate returned false!"); RoutingEndpointTrait trait = this.endpointTraits[this.currentIndex]; return trait; } } public bool HasAlternate { get { return this.currentIndex < (this.endpointTraits.Count - 1); } } public bool Sent { get { return this.sent; } } public void PrepareMessage(Message message) { if (this.exceptions != null) { message.Properties["Exceptions"] = this.exceptions; } } public void TransmitSucceeded(Transaction sendTransaction) { if (sendTransaction == null) { this.sent = true; } } public bool TryMoveToAlternate(Exception exception) { if (this.exceptions == null) { this.exceptions = new Dictionary (); } this.exceptions[this.CurrentEndpoint.Endpoint.Name] = exception; this.sent = false; if (++this.currentIndex < this.endpointTraits.Count) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
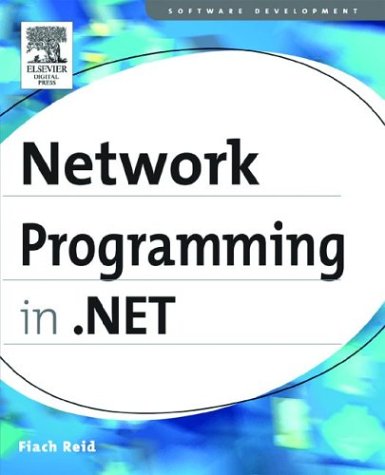
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Operand.cs
- BufferedGraphicsContext.cs
- VectorAnimationUsingKeyFrames.cs
- SequenceFullException.cs
- HtmlTernaryTree.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- StylusDevice.cs
- ModelEditingScope.cs
- ToolStripControlHost.cs
- TextHintingModeValidation.cs
- EncryptedReference.cs
- TrailingSpaceComparer.cs
- NavigationEventArgs.cs
- HttpModule.cs
- DataGridTableCollection.cs
- Int64KeyFrameCollection.cs
- EditorAttribute.cs
- IssuedTokenParametersEndpointAddressElement.cs
- ConsoleEntryPoint.cs
- XsltQilFactory.cs
- StrokeNodeEnumerator.cs
- ADRole.cs
- WebConfigurationHostFileChange.cs
- Expander.cs
- OdbcInfoMessageEvent.cs
- BufferAllocator.cs
- DependencySource.cs
- ControlBindingsCollection.cs
- EnumMember.cs
- DataViewListener.cs
- SqlConnectionStringBuilder.cs
- WindowShowOrOpenTracker.cs
- XmlSchemaAny.cs
- ColorEditor.cs
- SessionSwitchEventArgs.cs
- _CommandStream.cs
- MenuRendererStandards.cs
- XmlCustomFormatter.cs
- XmlSchemaObjectTable.cs
- MatrixTransform3D.cs
- DrawingGroupDrawingContext.cs
- MD5.cs
- SmiEventSink_Default.cs
- DataGridViewCell.cs
- Columns.cs
- DeviceContext.cs
- BamlRecordWriter.cs
- AssemblyHash.cs
- FileUtil.cs
- InternalDispatchObject.cs
- XmlNodeList.cs
- EmptyElement.cs
- DuplexSecurityProtocolFactory.cs
- AttachInfo.cs
- AsyncContentLoadedEventArgs.cs
- ThreadSafeList.cs
- InfoCardHelper.cs
- PcmConverter.cs
- EntityDataSourceQueryBuilder.cs
- InternalControlCollection.cs
- SymbolMethod.cs
- CurrentTimeZone.cs
- CheckableControlBaseAdapter.cs
- TypeLibConverter.cs
- DeviceContext.cs
- CfgParser.cs
- PathTooLongException.cs
- SqlDataSourceQueryEditor.cs
- AutomationPropertyInfo.cs
- Int16Storage.cs
- ResourceDictionary.cs
- DefaultTypeArgumentAttribute.cs
- HtmlObjectListAdapter.cs
- WindowsMenu.cs
- AppSettingsExpressionEditor.cs
- ContainsRowNumberChecker.cs
- FormatVersion.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- CalloutQueueItem.cs
- PlatformCulture.cs
- WindowsToolbarItemAsMenuItem.cs
- ListCommandEventArgs.cs
- ScrollableControl.cs
- XmlEntityReference.cs
- DiscreteKeyFrames.cs
- DefaultPropertyAttribute.cs
- ByteAnimationUsingKeyFrames.cs
- NativeMethods.cs
- InternalConfigHost.cs
- CapiNative.cs
- CompilationRelaxations.cs
- StylusPointPropertyId.cs
- ExpressionHelper.cs
- HttpModuleAction.cs
- BindToObject.cs
- EntryIndex.cs
- DSACryptoServiceProvider.cs
- BuildProviderAppliesToAttribute.cs
- BitmapEffect.cs
- XmlBinaryReader.cs