Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / Design / ComponentEditorPage.cs / 1 / ComponentEditorPage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Runtime.Remoting; using System.ComponentModel; using System.Diagnostics; using System; using System.Security.Permissions; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.ComponentModel; using System.ComponentModel.Design; using Microsoft.Win32; using System.Runtime.InteropServices; ////// /// [ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1012:AbstractTypesShouldNotHaveConstructors") // Shipped in Everett ] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] public abstract class ComponentEditorPage : Panel { IComponentEditorPageSite pageSite; IComponent component; bool firstActivate; bool loadRequired; int loading; Icon icon; bool commitOnDeactivate; ///Provides a base implementation for a ///. /// /// public ComponentEditorPage() : base() { commitOnDeactivate = false; firstActivate = true; loadRequired = false; loading = 0; Visible = false; } ////// Initializes a new instance of the ///class. /// /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public override bool AutoSize { get { return base.AutoSize; } set { base.AutoSize = value; } } ////// Hide the property /// ///[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// /// protected IComponentEditorPageSite PageSite { get { return pageSite; } set { pageSite = value; } } ///Gets or sets the page site. ////// /// protected IComponent Component { get { return component; } set { component = value; } } ///Gets or sets the component to edit. ////// /// protected bool FirstActivate { get { return firstActivate; } set { firstActivate = value; } } ///Indicates whether the page is being activated for the first time. ////// /// protected bool LoadRequired { get { return loadRequired; } set { loadRequired = value; } } ///Indicates whether a load is required previous to editing. ////// /// protected int Loading { get { return loading; } set { loading = value; } } ///Indicates if loading is taking place. ////// /// public bool CommitOnDeactivate { get { return commitOnDeactivate; } set { commitOnDeactivate = value; } } ///Indicates whether an editor should apply its /// changes before it is deactivated. ////// /// protected override CreateParams CreateParams { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { CreateParams cp = base.CreateParams; cp.Style &= ~(NativeMethods.WS_BORDER | NativeMethods.WS_OVERLAPPED | NativeMethods.WS_DLGFRAME); return cp; } } ///Gets or sets the creation parameters for this control. ////// /// public Icon Icon { get { if (icon == null) { icon = new Icon(typeof(ComponentEditorPage), "ComponentEditorPage.ico"); } return icon; } set { icon = value; } } ///Gets or sets the icon for this page. ////// /// public virtual string Title { get { return base.Text; } } ////// Gets or sets the title of the page. ////// /// Activates and displays the page. /// public virtual void Activate() { if (loadRequired) { EnterLoadingMode(); LoadComponent(); ExitLoadingMode(); loadRequired = false; } Visible = true; firstActivate = false; } ////// /// public virtual void ApplyChanges() { SaveComponent(); } ///Applies changes to all the components being edited. ////// /// public virtual void Deactivate() { Visible = false; } ///Deactivates and hides the page. ////// /// Increments the loading counter, which determines whether a page /// is in loading mode. /// protected void EnterLoadingMode() { loading++; } ////// /// Decrements the loading counter, which determines whether a page /// is in loading mode. /// protected void ExitLoadingMode() { Debug.Assert(loading > 0, "Unbalanced Enter/ExitLoadingMode calls"); loading--; } ////// /// public virtual Control GetControl() { return this; } ///Gets the control that represents the window for this page. ////// /// protected IComponent GetSelectedComponent() { return component; } ///Gets the component that is to be edited. ////// /// public virtual bool IsPageMessage(ref Message msg) { return PreProcessMessage(ref msg); } ///Processes messages that could be handled by the page. ////// /// protected bool IsFirstActivate() { return firstActivate; } ///Gets a value indicating whether the page is being activated for the first time. ////// /// protected bool IsLoading() { return loading != 0; } ///Gets a value indicating whether the page is being loaded. ////// /// protected abstract void LoadComponent(); ///Loads the component into the page UI. ////// /// public virtual void OnApplyComplete() { ReloadComponent(); } ////// Called when the page along with its sibling /// pages have applied their changes. ////// /// protected virtual void ReloadComponent() { if (Visible == false) { loadRequired = true; } } ///Called when the current component may have changed elsewhere /// and needs to be reloded into the UI. ////// /// protected abstract void SaveComponent(); ///Saves the component from the page UI. ////// /// protected virtual void SetDirty() { if (IsLoading() == false) { pageSite.SetDirty(); } } ///Sets the page to be in dirty state. ////// /// public virtual void SetComponent(IComponent component) { this.component = component; loadRequired = true; } ///Sets the component to be edited. ////// /// Sets the site for this page. /// public virtual void SetSite(IComponentEditorPageSite site) { this.pageSite = site; pageSite.GetControl().Controls.Add(this); } ////// /// public virtual void ShowHelp() { } ////// Provides help information to the help system. ////// /// public virtual bool SupportsHelp() { return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Gets a value indicating whether the editor supports Help. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Runtime.Remoting; using System.ComponentModel; using System.Diagnostics; using System; using System.Security.Permissions; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.ComponentModel; using System.ComponentModel.Design; using Microsoft.Win32; using System.Runtime.InteropServices; ////// /// [ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1012:AbstractTypesShouldNotHaveConstructors") // Shipped in Everett ] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] public abstract class ComponentEditorPage : Panel { IComponentEditorPageSite pageSite; IComponent component; bool firstActivate; bool loadRequired; int loading; Icon icon; bool commitOnDeactivate; ///Provides a base implementation for a ///. /// /// public ComponentEditorPage() : base() { commitOnDeactivate = false; firstActivate = true; loadRequired = false; loading = 0; Visible = false; } ////// Initializes a new instance of the ///class. /// /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public override bool AutoSize { get { return base.AutoSize; } set { base.AutoSize = value; } } ////// Hide the property /// ///[Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] new public event EventHandler AutoSizeChanged { add { base.AutoSizeChanged += value; } remove { base.AutoSizeChanged -= value; } } /// /// /// protected IComponentEditorPageSite PageSite { get { return pageSite; } set { pageSite = value; } } ///Gets or sets the page site. ////// /// protected IComponent Component { get { return component; } set { component = value; } } ///Gets or sets the component to edit. ////// /// protected bool FirstActivate { get { return firstActivate; } set { firstActivate = value; } } ///Indicates whether the page is being activated for the first time. ////// /// protected bool LoadRequired { get { return loadRequired; } set { loadRequired = value; } } ///Indicates whether a load is required previous to editing. ////// /// protected int Loading { get { return loading; } set { loading = value; } } ///Indicates if loading is taking place. ////// /// public bool CommitOnDeactivate { get { return commitOnDeactivate; } set { commitOnDeactivate = value; } } ///Indicates whether an editor should apply its /// changes before it is deactivated. ////// /// protected override CreateParams CreateParams { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { CreateParams cp = base.CreateParams; cp.Style &= ~(NativeMethods.WS_BORDER | NativeMethods.WS_OVERLAPPED | NativeMethods.WS_DLGFRAME); return cp; } } ///Gets or sets the creation parameters for this control. ////// /// public Icon Icon { get { if (icon == null) { icon = new Icon(typeof(ComponentEditorPage), "ComponentEditorPage.ico"); } return icon; } set { icon = value; } } ///Gets or sets the icon for this page. ////// /// public virtual string Title { get { return base.Text; } } ////// Gets or sets the title of the page. ////// /// Activates and displays the page. /// public virtual void Activate() { if (loadRequired) { EnterLoadingMode(); LoadComponent(); ExitLoadingMode(); loadRequired = false; } Visible = true; firstActivate = false; } ////// /// public virtual void ApplyChanges() { SaveComponent(); } ///Applies changes to all the components being edited. ////// /// public virtual void Deactivate() { Visible = false; } ///Deactivates and hides the page. ////// /// Increments the loading counter, which determines whether a page /// is in loading mode. /// protected void EnterLoadingMode() { loading++; } ////// /// Decrements the loading counter, which determines whether a page /// is in loading mode. /// protected void ExitLoadingMode() { Debug.Assert(loading > 0, "Unbalanced Enter/ExitLoadingMode calls"); loading--; } ////// /// public virtual Control GetControl() { return this; } ///Gets the control that represents the window for this page. ////// /// protected IComponent GetSelectedComponent() { return component; } ///Gets the component that is to be edited. ////// /// public virtual bool IsPageMessage(ref Message msg) { return PreProcessMessage(ref msg); } ///Processes messages that could be handled by the page. ////// /// protected bool IsFirstActivate() { return firstActivate; } ///Gets a value indicating whether the page is being activated for the first time. ////// /// protected bool IsLoading() { return loading != 0; } ///Gets a value indicating whether the page is being loaded. ////// /// protected abstract void LoadComponent(); ///Loads the component into the page UI. ////// /// public virtual void OnApplyComplete() { ReloadComponent(); } ////// Called when the page along with its sibling /// pages have applied their changes. ////// /// protected virtual void ReloadComponent() { if (Visible == false) { loadRequired = true; } } ///Called when the current component may have changed elsewhere /// and needs to be reloded into the UI. ////// /// protected abstract void SaveComponent(); ///Saves the component from the page UI. ////// /// protected virtual void SetDirty() { if (IsLoading() == false) { pageSite.SetDirty(); } } ///Sets the page to be in dirty state. ////// /// public virtual void SetComponent(IComponent component) { this.component = component; loadRequired = true; } ///Sets the component to be edited. ////// /// Sets the site for this page. /// public virtual void SetSite(IComponentEditorPageSite site) { this.pageSite = site; pageSite.GetControl().Controls.Add(this); } ////// /// public virtual void ShowHelp() { } ////// Provides help information to the help system. ////// /// public virtual bool SupportsHelp() { return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Gets a value indicating whether the editor supports Help. ///
Link Menu
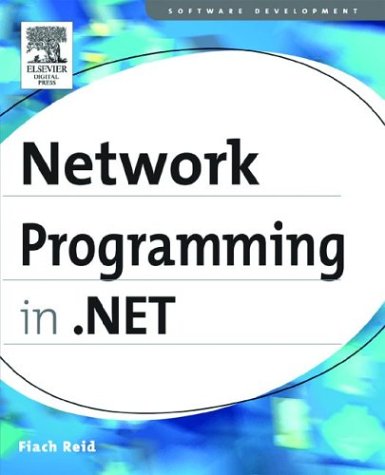
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuleSettings.cs
- MethodBody.cs
- GridEntry.cs
- ProxySimple.cs
- UnauthorizedWebPart.cs
- InputBinding.cs
- CallTemplateAction.cs
- FontCacheUtil.cs
- SchemaElement.cs
- NumberFunctions.cs
- Helper.cs
- CursorConverter.cs
- SoapExtensionTypeElementCollection.cs
- EditorZoneBase.cs
- BitmapCodecInfoInternal.cs
- mansign.cs
- ContractNamespaceAttribute.cs
- XmlTextEncoder.cs
- AsymmetricSignatureFormatter.cs
- AmbientLight.cs
- BamlRecords.cs
- GroupBoxRenderer.cs
- CqlLexer.cs
- IdentifierElement.cs
- AdjustableArrowCap.cs
- SrgsRuleRef.cs
- ClientCredentialsSecurityTokenManager.cs
- ConnectorDragDropGlyph.cs
- AbstractDataSvcMapFileLoader.cs
- _AuthenticationState.cs
- Matrix3D.cs
- ProtocolElementCollection.cs
- TableDetailsRow.cs
- SQLMoneyStorage.cs
- InputLanguageProfileNotifySink.cs
- ResXResourceWriter.cs
- TreeWalkHelper.cs
- OverflowException.cs
- DbConnectionInternal.cs
- ObjectParameter.cs
- SignerInfo.cs
- GridItemPatternIdentifiers.cs
- ListItemCollection.cs
- Image.cs
- BitConverter.cs
- Propagator.Evaluator.cs
- ClientBuildManager.cs
- DependencyProperty.cs
- ConsoleKeyInfo.cs
- ExpandoObject.cs
- BasicHttpSecurityElement.cs
- TimeZoneInfo.cs
- TableItemPatternIdentifiers.cs
- LinkedResource.cs
- FontUnitConverter.cs
- SchemaConstraints.cs
- RandomNumberGenerator.cs
- SerializationException.cs
- ConfigurationManagerHelperFactory.cs
- RoleGroup.cs
- VoiceInfo.cs
- TrailingSpaceComparer.cs
- TileModeValidation.cs
- TypeConverterHelper.cs
- UserControlCodeDomTreeGenerator.cs
- ContextProperty.cs
- SqlBulkCopyColumnMappingCollection.cs
- DataReaderContainer.cs
- VisualStyleRenderer.cs
- TerminateDesigner.cs
- MailWebEventProvider.cs
- ConfigurationElement.cs
- TrustManager.cs
- MasterPageBuildProvider.cs
- ByeOperation11AsyncResult.cs
- PinnedBufferMemoryStream.cs
- EntityContainerEntitySet.cs
- PerformanceCounterNameAttribute.cs
- StrokeNodeEnumerator.cs
- SBCSCodePageEncoding.cs
- VectorConverter.cs
- HttpHeaderCollection.cs
- LocatorPart.cs
- ProviderException.cs
- SafeLocalMemHandle.cs
- XslTransformFileEditor.cs
- SafeFileMapViewHandle.cs
- AdornerLayer.cs
- GeneralTransform.cs
- ServiceCredentials.cs
- RegistrationServices.cs
- ToolBar.cs
- GiveFeedbackEvent.cs
- DefaultProxySection.cs
- PenLineJoinValidation.cs
- FixedSOMSemanticBox.cs
- TextContainer.cs
- CreateUserErrorEventArgs.cs
- BinaryMethodMessage.cs
- RepeaterItem.cs