Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / RefType.cs / 1 / RefType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; namespace System.Data.Metadata.Edm { ////// Class representing a ref type /// public sealed class RefType : EdmType { #region Constructors ////// The constructor for constructing a RefType object with the entity type it references /// /// The entity type that this ref type references ///Thrown if entityType argument is null internal RefType(EntityType entityType) : base(GetIdentity(EntityUtil.GenericCheckArgumentNull(entityType, "entityType")), EdmConstants.TransientNamespace, entityType.DataSpace) { _elementType = entityType; SetReadOnly(); } #endregion #region Fields private readonly EntityTypeBase _elementType; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.RefType; } } ////// The entity type that this ref type references /// [MetadataProperty(BuiltInTypeKind.EntityTypeBase, false)] public EntityTypeBase ElementType { get { return _elementType; } } #endregion #region Methods ////// Constructs the name of the collection type /// /// The entity type base that this ref type refers to ///The identity of the resulting ref type private static string GetIdentity(EntityTypeBase entityTypeBase) { StringBuilder builder = new StringBuilder(50); builder.Append("reference["); entityTypeBase.BuildIdentity(builder); builder.Append("]"); return builder.ToString(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; namespace System.Data.Metadata.Edm { ////// Class representing a ref type /// public sealed class RefType : EdmType { #region Constructors ////// The constructor for constructing a RefType object with the entity type it references /// /// The entity type that this ref type references ///Thrown if entityType argument is null internal RefType(EntityType entityType) : base(GetIdentity(EntityUtil.GenericCheckArgumentNull(entityType, "entityType")), EdmConstants.TransientNamespace, entityType.DataSpace) { _elementType = entityType; SetReadOnly(); } #endregion #region Fields private readonly EntityTypeBase _elementType; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.RefType; } } ////// The entity type that this ref type references /// [MetadataProperty(BuiltInTypeKind.EntityTypeBase, false)] public EntityTypeBase ElementType { get { return _elementType; } } #endregion #region Methods ////// Constructs the name of the collection type /// /// The entity type base that this ref type refers to ///The identity of the resulting ref type private static string GetIdentity(EntityTypeBase entityTypeBase) { StringBuilder builder = new StringBuilder(50); builder.Append("reference["); entityTypeBase.BuildIdentity(builder); builder.Append("]"); return builder.ToString(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
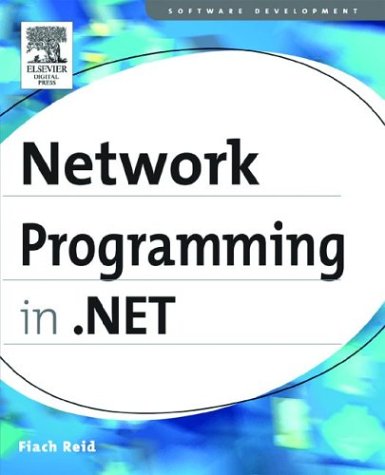
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowInstance.cs
- ProtocolsConfigurationHandler.cs
- EventLogQuery.cs
- TemplateBuilder.cs
- ADMembershipUser.cs
- CanExecuteRoutedEventArgs.cs
- ShapingEngine.cs
- XmlSchemaAppInfo.cs
- TraceContextEventArgs.cs
- Single.cs
- DomNameTable.cs
- HostingEnvironmentWrapper.cs
- ScriptResourceInfo.cs
- SQLInt64.cs
- FontStretch.cs
- InvalidWorkflowException.cs
- Keyboard.cs
- TextChangedEventArgs.cs
- CodePageEncoding.cs
- CacheHelper.cs
- Lease.cs
- SafeBitVector32.cs
- ApplicationBuildProvider.cs
- GridViewDeletedEventArgs.cs
- InvokeMemberBinder.cs
- GenericUriParser.cs
- XamlDesignerSerializationManager.cs
- FamilyTypefaceCollection.cs
- Convert.cs
- SystemIcmpV6Statistics.cs
- SchemaObjectWriter.cs
- mda.cs
- FlowDocumentFormatter.cs
- GridViewUpdateEventArgs.cs
- SignedPkcs7.cs
- Bits.cs
- WebConfigurationManager.cs
- ItemCollectionEditor.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- TabControl.cs
- QueryCursorEventArgs.cs
- PersonalizationStateInfo.cs
- DefaultTraceListener.cs
- NamedPipeTransportBindingElement.cs
- DbgUtil.cs
- HierarchicalDataSourceIDConverter.cs
- CollectionViewSource.cs
- Int64Converter.cs
- IntersectQueryOperator.cs
- SafeHandles.cs
- XmlSchemaAttribute.cs
- SplashScreen.cs
- srgsitem.cs
- FixedFindEngine.cs
- RectConverter.cs
- SrgsDocument.cs
- SchemaManager.cs
- ScriptRef.cs
- BaseConfigurationRecord.cs
- SamlAction.cs
- XPathNodeHelper.cs
- TextServicesManager.cs
- DataRecord.cs
- GroupBox.cs
- EntitySqlQueryCacheEntry.cs
- InfoCardBaseException.cs
- RemoteWebConfigurationHost.cs
- TextDecoration.cs
- SingletonConnectionReader.cs
- TextRangeSerialization.cs
- Variant.cs
- ExpressionBuilderCollection.cs
- SubpageParaClient.cs
- ConstraintEnumerator.cs
- RequiredAttributeAttribute.cs
- diagnosticsswitches.cs
- ViewLoader.cs
- DataObject.cs
- ByteRangeDownloader.cs
- PropVariant.cs
- ToolStripDropDownItem.cs
- FileLogRecordEnumerator.cs
- StorageEntityContainerMapping.cs
- TableColumn.cs
- PageThemeCodeDomTreeGenerator.cs
- LastQueryOperator.cs
- MarkedHighlightComponent.cs
- EmptyControlCollection.cs
- AspNetSynchronizationContext.cs
- MarkupExtensionParser.cs
- PluralizationServiceUtil.cs
- ZipIOLocalFileHeader.cs
- ServiceManagerHandle.cs
- DocumentationServerProtocol.cs
- XmlIlGenerator.cs
- altserialization.cs
- MimeTypeAttribute.cs
- OraclePermission.cs
- TextEditorDragDrop.cs
- BaseCodePageEncoding.cs