Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Win32 / UnsafeNativeMethodsPenimc.cs / 1 / UnsafeNativeMethodsPenimc.cs
//#define OLD_ISF using System; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using MS.Win32; namespace MS.Win32.Penimc { internal static class UnsafeNativeMethods { ////// Critical to prevent inadvertant spread to transparent code /// [SecurityCritical] private static IPimcManager _pimcManager; ////// Critical to prevent inadvertant spread to transparent code /// [SecurityCritical] [ThreadStatic] private static IPimcManager _pimcManagerThreadStatic; ////// Make sure we load penimc.dll from COM registered location to avoid two instances of it. /// ////// Critical calls COM interop code that uses suppress unmanaged code security attributes /// [SecurityCritical] static UnsafeNativeMethods() { // This static contructor was added to make sure we load the proper version of Penimc.dll. // // Details: // P/invoke will use LoadLibrary to load penimc.dll and it will check the current // application directory before checking the system32 directory where this DLL // is installed to. If penimc.dll happens to be in the application directory as well // as the system32 directory we'll actually end up loaded two versions of // penimc.dll. One that we'd use for P/invokes and one that we'd use for COM. // If this happens then our Stylus code will fail since it relies on both P/invoke and COM // calls to talk to penimc.dll and it requires just one instance of this DLL to work. // So to make sure this doesn't happen we want to ensure we load the DLL using the COM // registered path before doing any P/invokes into it. _pimcManager = (IPimcManager)new PimcManager(); } ////// Returns IPimcManager interface. Creates this object the first time per thread. /// ////// Critical - returns critial data _pimcManager. /// internal static IPimcManager PimcManager { [SecurityCritical] get { if (_pimcManagerThreadStatic == null) { _pimcManagerThreadStatic = (IPimcManager)new PimcManager(); } return _pimcManagerThreadStatic; } } #if OLD_ISF ////// Managed wrapper for IsfCompressPropertyData /// /// Input byte array /// number of bytes in byte array /// /// In: Preferred algorithm Id /// Out: Best algorithm with parameters /// /// /// In: output buffer size (of pbOutput) /// Out: Actual number of bytes needed for compressed data /// /// Buffer to hold the output ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfCompressPropertyData( [In] byte [] pbInput, uint cbInput, ref byte pnAlgoByte, ref uint pcbOutput, [In, Out] byte [] pbOutput ); ////// Managed wrapper for IsfDecompressPropertyData /// /// Input buffer to be decompressed /// Number of bytes in the input buffer to be decompressed /// /// In: Output buffer capacity /// Out: Actual number of bytes required to hold uncompressed bytes /// /// Output buffer /// Algorithm id and parameters ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfDecompressPropertyData( [In] byte [] pbCompressed, uint cbCompressed, ref uint pcbOutput, [In, Out] byte [] pbOutput, ref byte pnAlgoByte ); ////// Managed wrapper for IsfCompressPacketData /// /// Handle to the compression engine (null is ok) /// Input buffer /// Number of bytes in the input buffer /// /// In: Preferred compression algorithm byte /// Out: Actual compression algorithm byte /// /// /// In: Output buffer capacity /// Out: Actual number of bytes required to hold compressed bytes /// /// Output buffer ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfCompressPacketData( CompressorSafeHandle hCompress, [In] int [] pbInput, [In] uint cInCount, ref byte pnAlgoByte, ref uint pcbOutput, [In, Out] byte [] pbOutput ); ////// Managed wrapper for IsfDecompressPacketData /// /// Handle to the compression engine (null is ok) /// Input buffer of compressed bytes /// /// In: Size of the input buffer /// Out: Actual number of compressed bytes decompressed. /// /// Count of int's in the compressed buffer /// Output buffer to receive the decompressed int's /// Algorithm bytes ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfDecompressPacketData( CompressorSafeHandle hCompress, [In] byte [] pbCompressed, ref uint pcbCompressed, uint cInCount, [In, Out] int [] pbOutput, ref byte pnAlgoData ); ////// Managed wrapper for IsfLoadCompressor /// /// Input buffer where compressor is saved /// /// In: Size of the input buffer /// Out: Number of bytes in the input buffer decompressed to construct compressor /// ///Handle to the compression engine loaded ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern CompressorSafeHandle IsfLoadCompressor( [In] byte [] pbInput, ref uint pcbInput ); ////// Managed wrapper for IsfReleaseCompressor /// /// Handle to the Compression Engine to be released ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern void IsfReleaseCompressor( IntPtr hCompress ); #endif ////// Managed wrapper for GetPenEvent /// /// Win32 event handle to wait on for new stylus input. /// Win32 event the signals a reset. /// Stylus event that was triggered. /// Stylus Device ID that triggered input. /// Count of packets returned. /// Byte count of packet data returned. /// Array of ints containing the packet data. ///true if succeeded, false if failed or shutting down. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool GetPenEvent( IntPtr commHandle, IntPtr handleReset, out int evt, out int stylusPointerId, out int cPackets, out int cbPacket, out IntPtr pPackets); ////// Managed wrapper for GetPenEventMultiple /// /// Count of elements in commHandles. /// Array of Win32 event handles to wait on for new stylus input. /// Win32 event the signals a reset. /// Index to the handle that triggered return. /// Stylus event that was triggered. /// Stylus Device ID that triggered input. /// Count of packets returned. /// Byte count of packet data returned. /// Array of ints containing the packet data. ///true if succeeded, false if failed or shutting down. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool GetPenEventMultiple( int cCommHandles, IntPtr[] commHandles, IntPtr handleReset, out int iHandle, out int evt, out int stylusPointerId, out int cPackets, out int cbPacket, out IntPtr pPackets); ////// Managed wrapper for GetLastSystemEventData /// /// Specifies PimcContext object handle to get event data on. /// ID of system event that was triggered. /// keyboar modifier (unused). /// Keyboard key (unused). /// X position in device units of gesture. /// Y position in device units of gesture. /// Mode of the cursor. /// State of stylus buttons (flick returns custom data in this). ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool GetLastSystemEventData( IntPtr commHandle, out int evt, out int modifier, out int key, out int x, out int y, out int cursorMode, out int buttonState); ////// Managed wrapper for CreateResetEvent /// /// Win32 event handle created. ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool CreateResetEvent(out IntPtr handle); ////// Managed wrapper for DestroyResetEvent /// /// Win32 event handle to destroy. ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool DestroyResetEvent(IntPtr handle); ////// Managed wrapper for RaiseResetEvent /// /// Win32 event handle to set. ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool RaiseResetEvent(IntPtr handle); } #if OLD_ISF internal class CompressorSafeHandle: SafeHandle { ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand /// [SecurityCritical] private CompressorSafeHandle() : this(true) { } ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand /// [SecurityCritical] private CompressorSafeHandle(bool ownHandle) : base(IntPtr.Zero, ownHandle) { } // Do not provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. ////// Critical:This code calls into a base class which is protected by link demand an by inheritance demand /// TreatAsSafe: It's safe to give out a boolean value. /// public override bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] get { return IsClosed || handle == IntPtr.Zero; } } ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand. /// [SecurityCritical] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] override protected bool ReleaseHandle() { // // return code from this is void. // internally it just calls delete on // the compressor pointer // UnsafeNativeMethods.IsfReleaseCompressor(handle); handle = IntPtr.Zero; return true; } ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand. /// public static CompressorSafeHandle Null { [SecurityCritical] get { return new CompressorSafeHandle(false); } } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //#define OLD_ISF using System; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using MS.Win32; namespace MS.Win32.Penimc { internal static class UnsafeNativeMethods { ////// Critical to prevent inadvertant spread to transparent code /// [SecurityCritical] private static IPimcManager _pimcManager; ////// Critical to prevent inadvertant spread to transparent code /// [SecurityCritical] [ThreadStatic] private static IPimcManager _pimcManagerThreadStatic; ////// Make sure we load penimc.dll from COM registered location to avoid two instances of it. /// ////// Critical calls COM interop code that uses suppress unmanaged code security attributes /// [SecurityCritical] static UnsafeNativeMethods() { // This static contructor was added to make sure we load the proper version of Penimc.dll. // // Details: // P/invoke will use LoadLibrary to load penimc.dll and it will check the current // application directory before checking the system32 directory where this DLL // is installed to. If penimc.dll happens to be in the application directory as well // as the system32 directory we'll actually end up loaded two versions of // penimc.dll. One that we'd use for P/invokes and one that we'd use for COM. // If this happens then our Stylus code will fail since it relies on both P/invoke and COM // calls to talk to penimc.dll and it requires just one instance of this DLL to work. // So to make sure this doesn't happen we want to ensure we load the DLL using the COM // registered path before doing any P/invokes into it. _pimcManager = (IPimcManager)new PimcManager(); } ////// Returns IPimcManager interface. Creates this object the first time per thread. /// ////// Critical - returns critial data _pimcManager. /// internal static IPimcManager PimcManager { [SecurityCritical] get { if (_pimcManagerThreadStatic == null) { _pimcManagerThreadStatic = (IPimcManager)new PimcManager(); } return _pimcManagerThreadStatic; } } #if OLD_ISF ////// Managed wrapper for IsfCompressPropertyData /// /// Input byte array /// number of bytes in byte array /// /// In: Preferred algorithm Id /// Out: Best algorithm with parameters /// /// /// In: output buffer size (of pbOutput) /// Out: Actual number of bytes needed for compressed data /// /// Buffer to hold the output ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfCompressPropertyData( [In] byte [] pbInput, uint cbInput, ref byte pnAlgoByte, ref uint pcbOutput, [In, Out] byte [] pbOutput ); ////// Managed wrapper for IsfDecompressPropertyData /// /// Input buffer to be decompressed /// Number of bytes in the input buffer to be decompressed /// /// In: Output buffer capacity /// Out: Actual number of bytes required to hold uncompressed bytes /// /// Output buffer /// Algorithm id and parameters ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfDecompressPropertyData( [In] byte [] pbCompressed, uint cbCompressed, ref uint pcbOutput, [In, Out] byte [] pbOutput, ref byte pnAlgoByte ); ////// Managed wrapper for IsfCompressPacketData /// /// Handle to the compression engine (null is ok) /// Input buffer /// Number of bytes in the input buffer /// /// In: Preferred compression algorithm byte /// Out: Actual compression algorithm byte /// /// /// In: Output buffer capacity /// Out: Actual number of bytes required to hold compressed bytes /// /// Output buffer ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfCompressPacketData( CompressorSafeHandle hCompress, [In] int [] pbInput, [In] uint cInCount, ref byte pnAlgoByte, ref uint pcbOutput, [In, Out] byte [] pbOutput ); ////// Managed wrapper for IsfDecompressPacketData /// /// Handle to the compression engine (null is ok) /// Input buffer of compressed bytes /// /// In: Size of the input buffer /// Out: Actual number of compressed bytes decompressed. /// /// Count of int's in the compressed buffer /// Output buffer to receive the decompressed int's /// Algorithm bytes ///Status ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern int IsfDecompressPacketData( CompressorSafeHandle hCompress, [In] byte [] pbCompressed, ref uint pcbCompressed, uint cInCount, [In, Out] int [] pbOutput, ref byte pnAlgoData ); ////// Managed wrapper for IsfLoadCompressor /// /// Input buffer where compressor is saved /// /// In: Size of the input buffer /// Out: Number of bytes in the input buffer decompressed to construct compressor /// ///Handle to the compression engine loaded ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern CompressorSafeHandle IsfLoadCompressor( [In] byte [] pbInput, ref uint pcbInput ); ////// Managed wrapper for IsfReleaseCompressor /// /// Handle to the Compression Engine to be released ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern void IsfReleaseCompressor( IntPtr hCompress ); #endif ////// Managed wrapper for GetPenEvent /// /// Win32 event handle to wait on for new stylus input. /// Win32 event the signals a reset. /// Stylus event that was triggered. /// Stylus Device ID that triggered input. /// Count of packets returned. /// Byte count of packet data returned. /// Array of ints containing the packet data. ///true if succeeded, false if failed or shutting down. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool GetPenEvent( IntPtr commHandle, IntPtr handleReset, out int evt, out int stylusPointerId, out int cPackets, out int cbPacket, out IntPtr pPackets); ////// Managed wrapper for GetPenEventMultiple /// /// Count of elements in commHandles. /// Array of Win32 event handles to wait on for new stylus input. /// Win32 event the signals a reset. /// Index to the handle that triggered return. /// Stylus event that was triggered. /// Stylus Device ID that triggered input. /// Count of packets returned. /// Byte count of packet data returned. /// Array of ints containing the packet data. ///true if succeeded, false if failed or shutting down. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool GetPenEventMultiple( int cCommHandles, IntPtr[] commHandles, IntPtr handleReset, out int iHandle, out int evt, out int stylusPointerId, out int cPackets, out int cbPacket, out IntPtr pPackets); ////// Managed wrapper for GetLastSystemEventData /// /// Specifies PimcContext object handle to get event data on. /// ID of system event that was triggered. /// keyboar modifier (unused). /// Keyboard key (unused). /// X position in device units of gesture. /// Y position in device units of gesture. /// Mode of the cursor. /// State of stylus buttons (flick returns custom data in this). ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool GetLastSystemEventData( IntPtr commHandle, out int evt, out int modifier, out int key, out int x, out int y, out int cursorMode, out int buttonState); ////// Managed wrapper for CreateResetEvent /// /// Win32 event handle created. ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool CreateResetEvent(out IntPtr handle); ////// Managed wrapper for DestroyResetEvent /// /// Win32 event handle to destroy. ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool DestroyResetEvent(IntPtr handle); ////// Managed wrapper for RaiseResetEvent /// /// Win32 event handle to set. ///true if succeeded, false if failed. ////// Critical as suppressing UnmanagedCodeSecurity /// [SecurityCritical, SuppressUnmanagedCodeSecurity] [DllImport(ExternDll.Penimc, CharSet=CharSet.Auto)] internal static extern bool RaiseResetEvent(IntPtr handle); } #if OLD_ISF internal class CompressorSafeHandle: SafeHandle { ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand /// [SecurityCritical] private CompressorSafeHandle() : this(true) { } ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand /// [SecurityCritical] private CompressorSafeHandle(bool ownHandle) : base(IntPtr.Zero, ownHandle) { } // Do not provide a finalizer - SafeHandle's critical finalizer will // call ReleaseHandle for you. ////// Critical:This code calls into a base class which is protected by link demand an by inheritance demand /// TreatAsSafe: It's safe to give out a boolean value. /// public override bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] get { return IsClosed || handle == IntPtr.Zero; } } ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand. /// [SecurityCritical] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] override protected bool ReleaseHandle() { // // return code from this is void. // internally it just calls delete on // the compressor pointer // UnsafeNativeMethods.IsfReleaseCompressor(handle); handle = IntPtr.Zero; return true; } ////// Critical: This code calls into a base class which is protected by link demand and by inheritance demand. /// public static CompressorSafeHandle Null { [SecurityCritical] get { return new CompressorSafeHandle(false); } } } #endif } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
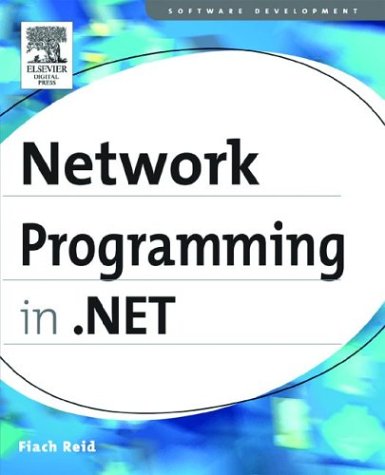
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExtensibleClassFactory.cs
- Stackframe.cs
- ListViewContainer.cs
- ManifestResourceInfo.cs
- SqlDataSourceParameterParser.cs
- RequestCacheEntry.cs
- ScriptingProfileServiceSection.cs
- ActivityInterfaces.cs
- EncryptedKey.cs
- Drawing.cs
- CurrentChangedEventManager.cs
- TemplateAction.cs
- ConfigurationStrings.cs
- RenderingEventArgs.cs
- PhonemeEventArgs.cs
- FormattedTextSymbols.cs
- KoreanCalendar.cs
- CounterCreationDataCollection.cs
- XmlBinaryReaderSession.cs
- AnnotationObservableCollection.cs
- ProjectionCamera.cs
- Properties.cs
- IgnorePropertiesAttribute.cs
- FormViewUpdateEventArgs.cs
- Vector.cs
- XmlSequenceWriter.cs
- CachedBitmap.cs
- NameValuePair.cs
- Maps.cs
- ViewManager.cs
- EntityModelBuildProvider.cs
- TransactionScope.cs
- SchemaConstraints.cs
- ProviderSettings.cs
- HtmlTableRow.cs
- SecurityTokenResolver.cs
- UnaryNode.cs
- ManipulationInertiaStartingEventArgs.cs
- DateRangeEvent.cs
- MailMessage.cs
- Function.cs
- ASCIIEncoding.cs
- FactoryGenerator.cs
- ScrollChrome.cs
- TrustSection.cs
- SweepDirectionValidation.cs
- TypedTableHandler.cs
- Line.cs
- InputBindingCollection.cs
- SoundPlayer.cs
- BasicHttpBindingElement.cs
- OleDbDataAdapter.cs
- NamespaceList.cs
- FrameworkContentElement.cs
- GACMembershipCondition.cs
- DEREncoding.cs
- InputLanguageSource.cs
- AstTree.cs
- ActionItem.cs
- XmlAnyElementAttribute.cs
- XmlLoader.cs
- ControllableStoryboardAction.cs
- TableParagraph.cs
- ColorTransform.cs
- HierarchicalDataTemplate.cs
- SchemeSettingElement.cs
- MsmqHostedTransportConfiguration.cs
- ApplicationProxyInternal.cs
- GridViewColumnHeaderAutomationPeer.cs
- DataTableNewRowEvent.cs
- DataServiceException.cs
- ActivationServices.cs
- Pen.cs
- CodePrimitiveExpression.cs
- CmsInterop.cs
- QueryConverter.cs
- oledbmetadatacolumnnames.cs
- StylusPointProperty.cs
- RegisteredExpandoAttribute.cs
- ObjectDataSourceDesigner.cs
- WindowInteropHelper.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ClientBuildManager.cs
- CredentialCache.cs
- PointUtil.cs
- CompositeScriptReferenceEventArgs.cs
- SectionVisual.cs
- ToolStripItemRenderEventArgs.cs
- SQLMembershipProvider.cs
- BaseConfigurationRecord.cs
- ObjectSecurity.cs
- AnimationClock.cs
- MarshalByValueComponent.cs
- Wizard.cs
- DockPanel.cs
- CodeDomDecompiler.cs
- WsatConfiguration.cs
- ISO2022Encoding.cs
- PersonalizableAttribute.cs
- CustomValidator.cs