Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebControls / SqlDataSourceParameterParser.cs / 1 / SqlDataSourceParameterParser.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls { using System; using System.Collections; using System.Diagnostics; using System.IO; using System.Web.UI.WebControls; // ////// Helper class to get list of parameters from an arbitrary SQL query. /// internal static class SqlDataSourceParameterParser { ////// Parses a command and returns an array of parameter names. /// public static Parameter[] ParseCommandText(string providerName, string commandText) { if (String.IsNullOrEmpty(providerName)) { providerName = SqlDataSourceDesigner.DefaultProviderName; } if (String.IsNullOrEmpty(commandText)) { commandText = String.Empty; } ParameterParser parser = null; switch (providerName.ToLowerInvariant()) { case "system.data.sqlclient": parser = new SqlClientParameterParser(); break; case "system.data.odbc": case "system.data.oledb": parser = new MiscParameterParser(); break; case "system.data.oracleclient": parser = new OracleClientParameterParser(); break; } if (parser == null) { Debug.Fail("Trying to parse parameters for unknown provider: " + providerName); return new Parameter[0]; } return parser.ParseCommandText(commandText); } ////// Base class for parameter parsing classes. /// private abstract class ParameterParser { public abstract Parameter[] ParseCommandText(string commandText); } ////// Parses SqlClient parameters of the form: /// select * from authors where col1 = @param1 /// private sealed class SqlClientParameterParser : ParameterParser { ////// List of states for parser. /// private enum State { InText, InQuote, InDoubleQuote, InBracket, InParameter, } ////// Returns true is a character is valid for a named parameter. /// private static bool IsValidParamNameChar(char c) { return (Char.IsLetterOrDigit(c)) || (c == '@') || (c == '$') || (c == '#') || (c == '_'); } public override Parameter[] ParseCommandText(string commandText) { int index = 0; int length = commandText.Length; State state = State.InText; System.Collections.Generic.Listparameters = new System.Collections.Generic.List (); System.Collections.Specialized.StringCollection parameterNames = new System.Collections.Specialized.StringCollection(); while (index < length) { switch (state) { case State.InText: if (commandText[index] == '\'') { state = State.InQuote; } else if (commandText[index] == '"') { state = State.InDoubleQuote; } else if (commandText[index] == '[') { state = State.InBracket; } else if (commandText[index] == '@') { state = State.InParameter; } else { index++; } break; case State.InQuote: index++; while (index < length && commandText[index] != '\'') { index++; } index++; state = State.InText; break; case State.InDoubleQuote: index++; while (index < length && commandText[index] != '"') { index++; } index++; state = State.InText; break; case State.InBracket: index++; while (index < length && commandText[index] != ']') { index++; } index++; state = State.InText; break; case State.InParameter: index++; string paramName = String.Empty; while ((index < length) && (IsValidParamNameChar(commandText[index]))) { paramName += commandText[index]; index++; } // Ignore @@functions since they aren't parameters if (!paramName.StartsWith("@", StringComparison.Ordinal)) { Parameter p = new Parameter(paramName); // Ignore duplicate parameters if (!parameterNames.Contains(paramName)) { parameters.Add(p); parameterNames.Add(paramName); } } state = State.InText; break; } } return parameters.ToArray(); } } /// /// Parses ODBC and OLEDB parameters of the form: /// select * from authors where col1 = ? /// private sealed class MiscParameterParser : ParameterParser { ////// List of states for parser. /// private enum State { InText, InQuote, InDoubleQuote, InBracket, InQuestion, } public override Parameter[] ParseCommandText(string commandText) { int index = 0; int length = commandText.Length; State state = State.InText; System.Collections.Generic.Listparameters = new System.Collections.Generic.List (); while (index < length) { switch (state) { case State.InText: if (commandText[index] == '\'') { state = State.InQuote; } else if (commandText[index] == '"') { state = State.InDoubleQuote; } else if (commandText[index] == '[') { state = State.InBracket; } else if (commandText[index] == '?') { state = State.InQuestion; } else { index++; } break; case State.InQuote: index++; while (index < length && commandText[index] != '\'') { index++; } index++; state = State.InText; break; case State.InDoubleQuote: index++; while (index < length && commandText[index] != '"') { index++; } index++; state = State.InText; break; case State.InBracket: index++; while (index < length && commandText[index] != ']') { index++; } index++; state = State.InText; break; case State.InQuestion: index++; parameters.Add(new Parameter("?")); state = State.InText; break; } } return parameters.ToArray(); } } /// /// Parses OracleClient parameters of the form: /// select * from authors where col1 = :param1 /// private sealed class OracleClientParameterParser : ParameterParser { ////// List of states for parser. /// private enum State { InText, InQuote, InDoubleQuote, InBracket, InParameter, } ////// Returns true is a character is valid for a named parameter. /// private static bool IsValidParamNameChar(char c) { // return (Char.IsLetterOrDigit(c)) || (c == '_'); } public override Parameter[] ParseCommandText(string commandText) { int index = 0; int length = commandText.Length; State state = State.InText; System.Collections.Generic.Listparameters = new System.Collections.Generic.List (); System.Collections.Specialized.StringCollection parameterNames = new System.Collections.Specialized.StringCollection(); while (index < length) { switch (state) { case State.InText: if (commandText[index] == '\'') { state = State.InQuote; } else if (commandText[index] == '"') { state = State.InDoubleQuote; } else if (commandText[index] == '[') { state = State.InBracket; } else if (commandText[index] == ':') { state = State.InParameter; } else { index++; } break; case State.InQuote: index++; while (index < length && commandText[index] != '\'') { index++; } index++; state = State.InText; break; case State.InDoubleQuote: index++; while (index < length && commandText[index] != '"') { index++; } index++; state = State.InText; break; case State.InBracket: index++; while (index < length && commandText[index] != ']') { index++; } index++; state = State.InText; break; case State.InParameter: index++; string paramName = String.Empty; while ((index < length) && (IsValidParamNameChar(commandText[index]))) { paramName += commandText[index]; index++; } Parameter p = new Parameter(paramName); // Ignore duplicate parameters if (!parameterNames.Contains(paramName)) { parameters.Add(p); parameterNames.Add(paramName); } state = State.InText; break; } } return parameters.ToArray(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
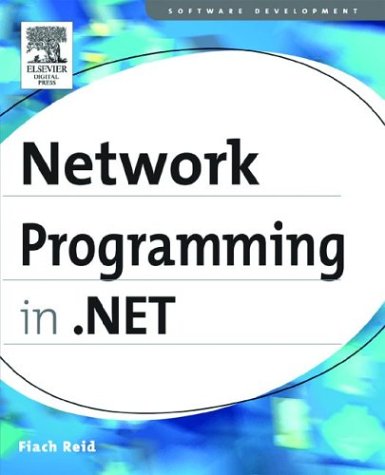
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextPenaltyModule.cs
- TileModeValidation.cs
- AttachedPropertyMethodSelector.cs
- FontSource.cs
- ArgumentNullException.cs
- PipelineModuleStepContainer.cs
- XmlWhitespace.cs
- BindingUtils.cs
- AddInControllerImpl.cs
- DataGridView.cs
- ToolBar.cs
- OptimalTextSource.cs
- UnauthorizedAccessException.cs
- DbDataReader.cs
- ChannelPool.cs
- HitTestParameters.cs
- IndexedString.cs
- Label.cs
- StatusBar.cs
- MsmqException.cs
- SystemException.cs
- CopyOnWriteList.cs
- VideoDrawing.cs
- OptimisticConcurrencyException.cs
- TextElementCollection.cs
- Char.cs
- _RegBlobWebProxyDataBuilder.cs
- FlowLayout.cs
- PropertyInfoSet.cs
- MarkupProperty.cs
- DependencyObjectPropertyDescriptor.cs
- StringUtil.cs
- UrlAuthorizationModule.cs
- DefaultClaimSet.cs
- PropertyInfoSet.cs
- MediaTimeline.cs
- RetrieveVirtualItemEventArgs.cs
- FreeFormDesigner.cs
- TextBox.cs
- QueryOpeningEnumerator.cs
- ProjectionQueryOptionExpression.cs
- _StreamFramer.cs
- XamlGridLengthSerializer.cs
- CommandBinding.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- Message.cs
- FloaterParagraph.cs
- CurrencyManager.cs
- GridViewDeleteEventArgs.cs
- ListViewGroup.cs
- WebPartAddingEventArgs.cs
- AuthorizationSection.cs
- SubordinateTransaction.cs
- LocalBuilder.cs
- PeerNameResolver.cs
- CodeTryCatchFinallyStatement.cs
- Vector3DAnimationUsingKeyFrames.cs
- Span.cs
- DocumentViewerHelper.cs
- versioninfo.cs
- CollectionChange.cs
- RadioButton.cs
- ScriptResourceInfo.cs
- ScrollProperties.cs
- TranslateTransform.cs
- BasicCellRelation.cs
- LinkLabel.cs
- EncryptedHeader.cs
- GregorianCalendarHelper.cs
- TransformCollection.cs
- Thickness.cs
- CrossAppDomainChannel.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- XmlElementCollection.cs
- BamlLocalizationDictionary.cs
- XmlSchemaDocumentation.cs
- EnlistmentTraceIdentifier.cs
- ComponentCollection.cs
- ImageButton.cs
- SmtpSection.cs
- CompiledRegexRunner.cs
- FileLevelControlBuilderAttribute.cs
- HtmlInputSubmit.cs
- TreeViewImageIndexConverter.cs
- M3DUtil.cs
- IconBitmapDecoder.cs
- WindowPatternIdentifiers.cs
- InputScope.cs
- UnicodeEncoding.cs
- SafeFileMappingHandle.cs
- ThrowOnMultipleAssignment.cs
- TaskHelper.cs
- Operand.cs
- WebColorConverter.cs
- WsatAdminException.cs
- KeyboardNavigation.cs
- SqlMethodTransformer.cs
- SqlClientPermission.cs
- ExpressionBindingCollection.cs
- _CommandStream.cs