Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Media / Animation / ControllableStoryboardAction.cs / 1305600 / ControllableStoryboardAction.cs
/****************************************************************************\ * * File: ControllableStoryboardAction.cs * * This object includes a named Storyboard references. When triggered, the * name is resolved and passed to a derived class method to perform the * actual action. * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.ComponentModel; // DefaultValueAttribute using System.Diagnostics; // Debug.Assert using System.Windows.Documents; // TableTemplate using System.Windows.Markup; // INameScope namespace System.Windows.Media.Animation { ////// A controllable storyboard action associates a trigger action with a /// Storyboard. The association from this object is a string that is the name /// of the Storyboard in a resource dictionary. /// public abstract class ControllableStoryboardAction : TriggerAction { ////// Internal constructor - nobody is supposed to ever create an instance /// of this class. Use a derived class instead. /// internal ControllableStoryboardAction() { } ////// Name to use for resolving the storyboard reference needed. This /// points to a BeginStoryboard instance, and we're controlling that one. /// [DefaultValue(null)] public string BeginStoryboardName { get { return _beginStoryboardName; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "ControllableStoryboardAction")); } // Null is allowed to wipe out existing name - as long as another // valid name is set before Invoke time. _beginStoryboardName = value; } } internal sealed override void Invoke( FrameworkElement fe, FrameworkContentElement fce, Style targetStyle, FrameworkTemplate frameworkTemplate, Int64 layer ) { Debug.Assert( fe != null || fce != null, "Caller of internal function failed to verify that we have a FE or FCE - we have neither." ); Debug.Assert( targetStyle != null || frameworkTemplate != null, "This function expects to be called when the associated action is inside a Style/Template. But it was not given a reference to anything." ); INameScope nameScope = null; if( targetStyle != null ) { nameScope = targetStyle; } else { Debug.Assert( frameworkTemplate != null ); nameScope = frameworkTemplate; } Invoke( fe, fce, GetStoryboard( fe, fce, nameScope ) ); } internal sealed override void Invoke( FrameworkElement fe ) { Debug.Assert( fe != null, "Invoke needs an object as starting point"); Invoke( fe, null, GetStoryboard( fe, null, null ) ); } internal virtual void Invoke( FrameworkElement containingFE, FrameworkContentElement containingFCE, Storyboard storyboard ) { } // Find a Storyboard object for this StoryboardAction to act on, using the // given BeginStoryboardName to find a BeginStoryboard instance and use // its Storyboard object reference. private Storyboard GetStoryboard( FrameworkElement fe, FrameworkContentElement fce, INameScope nameScope ) { if( BeginStoryboardName == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNameRequired)); } BeginStoryboard keyedBeginStoryboard = Storyboard.ResolveBeginStoryboardName( BeginStoryboardName, nameScope, fe, fce ); Storyboard storyboard = keyedBeginStoryboard.Storyboard; if( storyboard == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNoStoryboard, BeginStoryboardName)); } return storyboard; } private string _beginStoryboardName = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
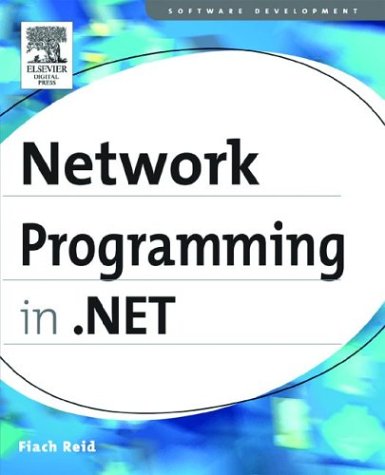
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CurrentChangingEventManager.cs
- UrlRoutingHandler.cs
- DigestComparer.cs
- ISFTagAndGuidCache.cs
- ByteConverter.cs
- FilterQueryOptionExpression.cs
- ProfileInfo.cs
- DetailsViewDeletedEventArgs.cs
- PathTooLongException.cs
- Listbox.cs
- DelegatingChannelListener.cs
- ExceptionUtility.cs
- DataGridViewCellLinkedList.cs
- Pair.cs
- DropDownList.cs
- ToolStripDropDownMenu.cs
- OpenFileDialog.cs
- CodeArgumentReferenceExpression.cs
- TemplateControl.cs
- Update.cs
- CursorInteropHelper.cs
- WSSecurityOneDotOneSendSecurityHeader.cs
- ParameterSubsegment.cs
- Attributes.cs
- AspCompat.cs
- SHA512Managed.cs
- ComponentResourceManager.cs
- AutomationElement.cs
- _NestedMultipleAsyncResult.cs
- WpfKnownTypeInvoker.cs
- OrderedDictionary.cs
- DescendantOverDescendantQuery.cs
- OdbcEnvironmentHandle.cs
- ParserStreamGeometryContext.cs
- HostVisual.cs
- BaseComponentEditor.cs
- PersonalizationStateQuery.cs
- EditorPartChrome.cs
- ReferentialConstraintRoleElement.cs
- sqlpipe.cs
- UriWriter.cs
- ToolStripDesignerUtils.cs
- FacetDescriptionElement.cs
- TypeDescriptorContext.cs
- BackgroundWorker.cs
- SemanticResultValue.cs
- ThousandthOfEmRealPoints.cs
- FormsAuthenticationCredentials.cs
- CreateSequence.cs
- HtmlInputReset.cs
- XmlAttributes.cs
- VirtualDirectoryMappingCollection.cs
- Size.cs
- FlowLayout.cs
- Int16.cs
- MaskedTextBox.cs
- AnnotationObservableCollection.cs
- xml.cs
- ProxyGenerator.cs
- figurelengthconverter.cs
- ReferenceList.cs
- DateTimeConverter.cs
- FrameworkElement.cs
- FileClassifier.cs
- MultiBinding.cs
- SqlSelectStatement.cs
- PeerCollaboration.cs
- Registry.cs
- ButtonBase.cs
- DESCryptoServiceProvider.cs
- Queue.cs
- XmlUrlResolver.cs
- StaticTextPointer.cs
- DataGridDetailsPresenterAutomationPeer.cs
- LocalFileSettingsProvider.cs
- AnnotationHighlightLayer.cs
- PageTheme.cs
- XmlDataCollection.cs
- SerializationObjectManager.cs
- TrustLevel.cs
- SizeChangedEventArgs.cs
- PartialCachingAttribute.cs
- DataGridHyperlinkColumn.cs
- BinHexDecoder.cs
- ModifierKeysConverter.cs
- CommandField.cs
- Panel.cs
- OdbcTransaction.cs
- VideoDrawing.cs
- PolicyDesigner.cs
- ArrayConverter.cs
- AudioDeviceOut.cs
- StringFunctions.cs
- CacheEntry.cs
- UmAlQuraCalendar.cs
- ConstructorNeedsTagAttribute.cs
- ServiceRouteHandler.cs
- TcpAppDomainProtocolHandler.cs
- FormsIdentity.cs
- WeakHashtable.cs