Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / Ink / InkSerializedFormat / LZCodec.cs / 1 / LZCodec.cs
using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using MS.Internal.Ink; using System.Collections.Generic; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// LZCodec /// internal class LZCodec { ////// LZCodec /// internal LZCodec() { } ////// Uncompress /// /// /// ///internal byte[] Uncompress(byte[] input, int inputIndex) { //first things first Debug.Assert(input != null); Debug.Assert(input.Length > 1); Debug.Assert(inputIndex < input.Length); Debug.Assert(inputIndex >= 0); List output = new List (); BitStreamWriter writer = new BitStreamWriter(output); BitStreamReader reader = new BitStreamReader(input, inputIndex); //decode int index = 0, countBytes = 0, start = 0; byte byte1 = 0, byte2 = 0; _maxMatchLength = FirstMaxMatchLength; // initialize the ring buffer for (index = 0; index < RingBufferLength - _maxMatchLength; index++) { _ringBuffer[index] = 0; } //initialize decoding globals _flags = 0; _currentRingBufferPosition = RingBufferLength - _maxMatchLength; while (!reader.EndOfStream) { byte1 = reader.ReadByte(Native.BitsPerByte); // High order byte counts the number of bits used in the low order // byte. if (((_flags >>= 1) & 0x100) == 0) { // Set bit mask describing the next 8 bytes. _flags = (((int)byte1) | 0xff00); byte1 = reader.ReadByte(Native.BitsPerByte); } if ((_flags & 1) != 0) { // Just store the literal byte in the buffer. writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } else { // Extract the offset and count to copy from the ring buffer. byte2 = reader.ReadByte(Native.BitsPerByte); countBytes = (int)byte2; start = (countBytes & 0xf0) << 4 | (int)byte1; countBytes = (countBytes & 0x0f) + MaxLiteralLength; for (index = 0; index <= countBytes; index++) { byte1 = _ringBuffer[(start + index) & (RingBufferLength - 1)]; writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } } } return output.ToArray(); } /// /// Privates /// private byte[] _ringBuffer = new byte[RingBufferLength]; private int _maxMatchLength = 0; private int _flags = 0; private int _currentRingBufferPosition = 0; ////// Statics / constants /// private static readonly int FirstMaxMatchLength = 0x10; private static readonly int RingBufferLength = 4069; private static readonly int MaxLiteralLength = 2; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using MS.Internal.Ink; using System.Collections.Generic; using System.Diagnostics; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// LZCodec /// internal class LZCodec { ////// LZCodec /// internal LZCodec() { } ////// Uncompress /// /// /// ///internal byte[] Uncompress(byte[] input, int inputIndex) { //first things first Debug.Assert(input != null); Debug.Assert(input.Length > 1); Debug.Assert(inputIndex < input.Length); Debug.Assert(inputIndex >= 0); List output = new List (); BitStreamWriter writer = new BitStreamWriter(output); BitStreamReader reader = new BitStreamReader(input, inputIndex); //decode int index = 0, countBytes = 0, start = 0; byte byte1 = 0, byte2 = 0; _maxMatchLength = FirstMaxMatchLength; // initialize the ring buffer for (index = 0; index < RingBufferLength - _maxMatchLength; index++) { _ringBuffer[index] = 0; } //initialize decoding globals _flags = 0; _currentRingBufferPosition = RingBufferLength - _maxMatchLength; while (!reader.EndOfStream) { byte1 = reader.ReadByte(Native.BitsPerByte); // High order byte counts the number of bits used in the low order // byte. if (((_flags >>= 1) & 0x100) == 0) { // Set bit mask describing the next 8 bytes. _flags = (((int)byte1) | 0xff00); byte1 = reader.ReadByte(Native.BitsPerByte); } if ((_flags & 1) != 0) { // Just store the literal byte in the buffer. writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } else { // Extract the offset and count to copy from the ring buffer. byte2 = reader.ReadByte(Native.BitsPerByte); countBytes = (int)byte2; start = (countBytes & 0xf0) << 4 | (int)byte1; countBytes = (countBytes & 0x0f) + MaxLiteralLength; for (index = 0; index <= countBytes; index++) { byte1 = _ringBuffer[(start + index) & (RingBufferLength - 1)]; writer.Write(byte1, Native.BitsPerByte); _ringBuffer[_currentRingBufferPosition++] = byte1; _currentRingBufferPosition &= RingBufferLength - 1; } } } return output.ToArray(); } /// /// Privates /// private byte[] _ringBuffer = new byte[RingBufferLength]; private int _maxMatchLength = 0; private int _flags = 0; private int _currentRingBufferPosition = 0; ////// Statics / constants /// private static readonly int FirstMaxMatchLength = 0x10; private static readonly int RingBufferLength = 4069; private static readonly int MaxLiteralLength = 2; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
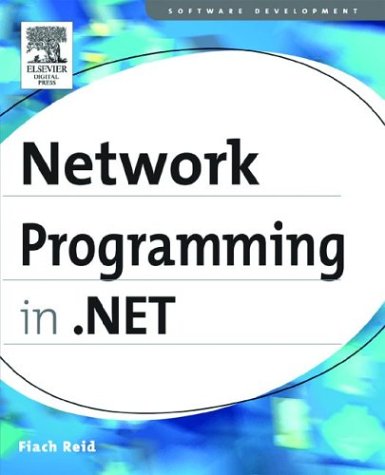
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LocalBuilder.cs
- LineGeometry.cs
- ZipIORawDataFileBlock.cs
- VisualStyleTypesAndProperties.cs
- DataSet.cs
- AutomationProperty.cs
- VariableDesigner.xaml.cs
- WindowsListBox.cs
- DataViewListener.cs
- TemplatePropertyEntry.cs
- DesignerHost.cs
- InstanceHandle.cs
- CodeStatementCollection.cs
- TypeFieldSchema.cs
- UpdateTranslator.cs
- TrackingAnnotationCollection.cs
- HMACRIPEMD160.cs
- WebConfigurationHostFileChange.cs
- ToolStripGrip.cs
- HandlerFactoryWrapper.cs
- UnknownBitmapEncoder.cs
- SqlDataSourceConfigureFilterForm.cs
- X509SubjectKeyIdentifierClause.cs
- DesignerVerbCollection.cs
- DictionaryContent.cs
- MetaData.cs
- ObjectContextServiceProvider.cs
- HitTestParameters3D.cs
- ObjectViewEntityCollectionData.cs
- OdbcConnectionOpen.cs
- Speller.cs
- SafeFileHandle.cs
- CancellationHandler.cs
- WebPartEventArgs.cs
- WindowsBrush.cs
- ConfigurationLocation.cs
- XmlElementList.cs
- SqlBulkCopyColumnMappingCollection.cs
- FixedBufferAttribute.cs
- TypedElement.cs
- ProjectionQueryOptionExpression.cs
- CharUnicodeInfo.cs
- ViewStateModeByIdAttribute.cs
- HttpCookie.cs
- IsolatedStorageException.cs
- DataList.cs
- ColorConvertedBitmapExtension.cs
- login.cs
- StylusPointPropertyInfo.cs
- SchemaImporter.cs
- DocumentPageTextView.cs
- HelpKeywordAttribute.cs
- _Rfc2616CacheValidators.cs
- BinaryWriter.cs
- ToolStripPanel.cs
- SortableBindingList.cs
- QilSortKey.cs
- ClientSponsor.cs
- AccessViolationException.cs
- WebPartExportVerb.cs
- PathFigure.cs
- HScrollBar.cs
- MultipartIdentifier.cs
- SAPIEngineTypes.cs
- EventTrigger.cs
- TableLayoutCellPaintEventArgs.cs
- SoapSchemaMember.cs
- EventMap.cs
- TableLayoutStyle.cs
- TextContainer.cs
- VisualStateManager.cs
- BezierSegment.cs
- MailMessageEventArgs.cs
- Dispatcher.cs
- VirtualPathUtility.cs
- ScrollableControlDesigner.cs
- CapiSymmetricAlgorithm.cs
- TextViewSelectionProcessor.cs
- RemotingServices.cs
- DataRelationCollection.cs
- DefaultValueAttribute.cs
- CompressedStack.cs
- ImpersonationContext.cs
- StylusPoint.cs
- ValidationEventArgs.cs
- DataFormats.cs
- HtmlDocument.cs
- DocumentPageTextView.cs
- FormsAuthentication.cs
- CompoundFileStorageReference.cs
- PeerObject.cs
- TypeConverterValueSerializer.cs
- ControlAdapter.cs
- NullableDoubleMinMaxAggregationOperator.cs
- CheckBox.cs
- MailMessageEventArgs.cs
- ManipulationDelta.cs
- M3DUtil.cs
- SignedInfo.cs
- cookie.cs