Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / Emitters / TypeReference.cs / 1 / TypeReference.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Common.Utils; namespace System.Data.EntityModel.Emitters { ////// Summary description for TypeReferences. /// internal class TypeReference { #region Fields internal static readonly Type ObjectContextBaseClassType = typeof(System.Data.Services.Client.DataServiceContext); public const string FQMetaDataWorkspaceTypeName = "System.Data.Metadata.Edm.MetadataWorkspace"; private static CodeTypeReference _byteArray; private static CodeTypeReference _dateTime; private static CodeTypeReference _guid; private static CodeTypeReference _objectContext; private readonly Memoizer_forTypeMemoizer; private readonly Memoizer _nullableForTypeMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringGenericMemoizer; #endregion #region Constructors internal TypeReference() { _forTypeMemoizer = new Memoizer (ComputeForType, null); _fromStringMemoizer = new Memoizer , CodeTypeReference>(ComputeFromString, null); _nullableForTypeMemoizer = new Memoizer (ComputeNullableForType, null); _fromStringGenericMemoizer = new Memoizer , CodeTypeReference>(ComputeFromStringGeneric, null); } #endregion #region Public Methods /// /// Get TypeReference for a type represented by a Type object /// /// the type object ///the associated TypeReference object public CodeTypeReference ForType(Type type) { return _forTypeMemoizer.Evaluate(type); } private CodeTypeReference ComputeForType(Type type) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); return value; } ////// Get TypeReference for a type represented by a Generic Type object. /// We don't cache the TypeReference for generic type object since the type would be the same /// irresepective of the generic arguments. We could potentially cache it using both the type name /// and generic type arguments. /// /// the generic type object ///the associated TypeReference object public CodeTypeReference ForType(Type generic, params CodeTypeReference[] argument) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference typeRef = new CodeTypeReference(generic, CodeTypeReferenceOptions.GlobalReference); if ((null != argument) && (0 < argument.Length)) { typeRef.TypeArguments.AddRange(argument); } return typeRef; } ////// Get TypeReference for a type represented by a namespace quailifed string /// /// namespace qualified string ///the TypeReference public CodeTypeReference FromString(string type) { return FromString(type, false); } ////// Get TypeReference for a type represented by a namespace quailifed string, /// with optional global qualifier /// /// namespace qualified string /// indicates whether the global qualifier should be added ///the TypeReference public CodeTypeReference FromString(string type, bool addGlobalQualifier) { return _fromStringMemoizer.Evaluate(new KeyValuePair(type, addGlobalQualifier)); } private CodeTypeReference ComputeFromString(KeyValuePair arguments) { string type = arguments.Key; bool addGlobalQualifier = arguments.Value; CodeTypeReference value; if (addGlobalQualifier) { value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); } else { value = new CodeTypeReference(type); } return value; } /// /// Get TypeReference for a framework type /// /// unqualified name of the framework type ///the TypeReference public CodeTypeReference AdoFrameworkType(string name) { return FromString(Utils.WebFrameworkNamespace + "." + name, true); } ////// Get TypeReference for a bound generic framework class /// /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class public CodeTypeReference AdoFrameworkGenericClass(string name, CodeTypeReference typeParameter) { return FrameworkGenericClass(Utils.WebFrameworkNamespace, name, typeParameter); } ////// Get TypeReference for a bound generic framework class /// /// the namespace of the generic framework class /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class public CodeTypeReference FrameworkGenericClass(string namespaceName, string name, CodeTypeReference typeParameter) { return _fromStringGenericMemoizer.Evaluate(new KeyValuePair(namespaceName + "." + name, typeParameter)); } private CodeTypeReference ComputeFromStringGeneric(KeyValuePair arguments) { string name = arguments.Key; CodeTypeReference typeParameter = arguments.Value; CodeTypeReference typeRef = ComputeFromString(new KeyValuePair (name, true)); typeRef.TypeArguments.Add(typeParameter); return typeRef; } /// /// Get TypeReference for a bound Nullable<T> /// /// Type of the Nullable<T> type parameter ///TypeReference for a bound Nullable<T> public CodeTypeReference NullableForType(Type innerType) { return _nullableForTypeMemoizer.Evaluate(innerType); } private CodeTypeReference ComputeNullableForType(Type innerType) { // can't use FromString because it will return the same Generic type reference // but it will already have a previous type parameter (because of caching) CodeTypeReference typeRef = new CodeTypeReference(typeof(System.Nullable<>), CodeTypeReferenceOptions.GlobalReference); typeRef.TypeArguments.Add(ForType(innerType)); return typeRef; } #endregion #region Public Properties ////// Gets a CodeTypeReference to the System.Byte[] type. /// ///public CodeTypeReference ByteArray { get { if (_byteArray == null) _byteArray = ForType(typeof(byte[])); return _byteArray; } } /// /// Gets a CodeTypeReference object for the System.DateTime type. /// public CodeTypeReference DateTime { get { if (_dateTime == null) _dateTime = ForType(typeof(System.DateTime)); return _dateTime; } } ////// Gets a CodeTypeReference object for the System.Guid type. /// public CodeTypeReference Guid { get { if (_guid == null) _guid = ForType(typeof(System.Guid)); return _guid; } } ////// TypeReference for the Framework's ObjectContext class /// public CodeTypeReference ObjectContext { get { if (_objectContext == null) { _objectContext = AdoFrameworkType("DataServiceContext"); } return _objectContext; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Common.Utils; namespace System.Data.EntityModel.Emitters { ////// Summary description for TypeReferences. /// internal class TypeReference { #region Fields internal static readonly Type ObjectContextBaseClassType = typeof(System.Data.Services.Client.DataServiceContext); public const string FQMetaDataWorkspaceTypeName = "System.Data.Metadata.Edm.MetadataWorkspace"; private static CodeTypeReference _byteArray; private static CodeTypeReference _dateTime; private static CodeTypeReference _guid; private static CodeTypeReference _objectContext; private readonly Memoizer_forTypeMemoizer; private readonly Memoizer _nullableForTypeMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringMemoizer; private readonly Memoizer , CodeTypeReference> _fromStringGenericMemoizer; #endregion #region Constructors internal TypeReference() { _forTypeMemoizer = new Memoizer (ComputeForType, null); _fromStringMemoizer = new Memoizer , CodeTypeReference>(ComputeFromString, null); _nullableForTypeMemoizer = new Memoizer (ComputeNullableForType, null); _fromStringGenericMemoizer = new Memoizer , CodeTypeReference>(ComputeFromStringGeneric, null); } #endregion #region Public Methods /// /// Get TypeReference for a type represented by a Type object /// /// the type object ///the associated TypeReference object public CodeTypeReference ForType(Type type) { return _forTypeMemoizer.Evaluate(type); } private CodeTypeReference ComputeForType(Type type) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); return value; } ////// Get TypeReference for a type represented by a Generic Type object. /// We don't cache the TypeReference for generic type object since the type would be the same /// irresepective of the generic arguments. We could potentially cache it using both the type name /// and generic type arguments. /// /// the generic type object ///the associated TypeReference object public CodeTypeReference ForType(Type generic, params CodeTypeReference[] argument) { // we know that we can safely global:: qualify this because it was already // compiled before we are emitting or else we wouldn't have a Type object CodeTypeReference typeRef = new CodeTypeReference(generic, CodeTypeReferenceOptions.GlobalReference); if ((null != argument) && (0 < argument.Length)) { typeRef.TypeArguments.AddRange(argument); } return typeRef; } ////// Get TypeReference for a type represented by a namespace quailifed string /// /// namespace qualified string ///the TypeReference public CodeTypeReference FromString(string type) { return FromString(type, false); } ////// Get TypeReference for a type represented by a namespace quailifed string, /// with optional global qualifier /// /// namespace qualified string /// indicates whether the global qualifier should be added ///the TypeReference public CodeTypeReference FromString(string type, bool addGlobalQualifier) { return _fromStringMemoizer.Evaluate(new KeyValuePair(type, addGlobalQualifier)); } private CodeTypeReference ComputeFromString(KeyValuePair arguments) { string type = arguments.Key; bool addGlobalQualifier = arguments.Value; CodeTypeReference value; if (addGlobalQualifier) { value = new CodeTypeReference(type, CodeTypeReferenceOptions.GlobalReference); } else { value = new CodeTypeReference(type); } return value; } /// /// Get TypeReference for a framework type /// /// unqualified name of the framework type ///the TypeReference public CodeTypeReference AdoFrameworkType(string name) { return FromString(Utils.WebFrameworkNamespace + "." + name, true); } ////// Get TypeReference for a bound generic framework class /// /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class public CodeTypeReference AdoFrameworkGenericClass(string name, CodeTypeReference typeParameter) { return FrameworkGenericClass(Utils.WebFrameworkNamespace, name, typeParameter); } ////// Get TypeReference for a bound generic framework class /// /// the namespace of the generic framework class /// the name of the generic framework class /// the type parameter for the framework class ///TypeReference for the bound framework class public CodeTypeReference FrameworkGenericClass(string namespaceName, string name, CodeTypeReference typeParameter) { return _fromStringGenericMemoizer.Evaluate(new KeyValuePair(namespaceName + "." + name, typeParameter)); } private CodeTypeReference ComputeFromStringGeneric(KeyValuePair arguments) { string name = arguments.Key; CodeTypeReference typeParameter = arguments.Value; CodeTypeReference typeRef = ComputeFromString(new KeyValuePair (name, true)); typeRef.TypeArguments.Add(typeParameter); return typeRef; } /// /// Get TypeReference for a bound Nullable<T> /// /// Type of the Nullable<T> type parameter ///TypeReference for a bound Nullable<T> public CodeTypeReference NullableForType(Type innerType) { return _nullableForTypeMemoizer.Evaluate(innerType); } private CodeTypeReference ComputeNullableForType(Type innerType) { // can't use FromString because it will return the same Generic type reference // but it will already have a previous type parameter (because of caching) CodeTypeReference typeRef = new CodeTypeReference(typeof(System.Nullable<>), CodeTypeReferenceOptions.GlobalReference); typeRef.TypeArguments.Add(ForType(innerType)); return typeRef; } #endregion #region Public Properties ////// Gets a CodeTypeReference to the System.Byte[] type. /// ///public CodeTypeReference ByteArray { get { if (_byteArray == null) _byteArray = ForType(typeof(byte[])); return _byteArray; } } /// /// Gets a CodeTypeReference object for the System.DateTime type. /// public CodeTypeReference DateTime { get { if (_dateTime == null) _dateTime = ForType(typeof(System.DateTime)); return _dateTime; } } ////// Gets a CodeTypeReference object for the System.Guid type. /// public CodeTypeReference Guid { get { if (_guid == null) _guid = ForType(typeof(System.Guid)); return _guid; } } ////// TypeReference for the Framework's ObjectContext class /// public CodeTypeReference ObjectContext { get { if (_objectContext == null) { _objectContext = AdoFrameworkType("DataServiceContext"); } return _objectContext; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
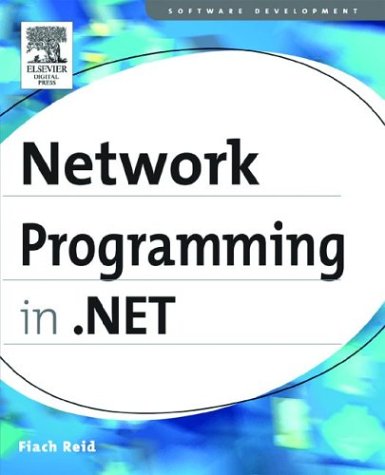
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSerializerSection.cs
- ResizeBehavior.cs
- IntSecurity.cs
- RegisteredArrayDeclaration.cs
- SqlBinder.cs
- ScriptManagerProxy.cs
- HashAlgorithm.cs
- TextBoxAutoCompleteSourceConverter.cs
- TypedColumnHandler.cs
- Bitmap.cs
- TreeBuilderXamlTranslator.cs
- DataSysAttribute.cs
- GeometryDrawing.cs
- XmlQuerySequence.cs
- Simplifier.cs
- MessageQueue.cs
- FixedSOMTableCell.cs
- XPathNavigator.cs
- FixedPageProcessor.cs
- ActiveXContainer.cs
- ReflectEventDescriptor.cs
- CurrentTimeZone.cs
- PersonalizationStateInfoCollection.cs
- HGlobalSafeHandle.cs
- BinaryCommonClasses.cs
- HttpRequestTraceRecord.cs
- Color.cs
- ReachSerializationCacheItems.cs
- KnowledgeBase.cs
- LogArchiveSnapshot.cs
- SByte.cs
- ASCIIEncoding.cs
- RichTextBoxConstants.cs
- Encoder.cs
- OleDbConnection.cs
- SqlAggregateChecker.cs
- SimpleBitVector32.cs
- CallbackValidator.cs
- CfgRule.cs
- EventHandlersStore.cs
- MediaContext.cs
- TargetConverter.cs
- HostingEnvironmentSection.cs
- InstanceOwnerException.cs
- PeerNearMe.cs
- DataGridColumnCollection.cs
- HtmlGenericControl.cs
- CodeNamespaceCollection.cs
- NamedElement.cs
- DataGridRowEventArgs.cs
- HttpRuntimeSection.cs
- MetricEntry.cs
- SqlDataAdapter.cs
- SqlBuilder.cs
- DayRenderEvent.cs
- SByte.cs
- ConfigurationLockCollection.cs
- BufferedGraphicsManager.cs
- ReachIDocumentPaginatorSerializer.cs
- VariantWrapper.cs
- WebConfigurationHostFileChange.cs
- SourceFileInfo.cs
- PartialClassGenerationTaskInternal.cs
- selecteditemcollection.cs
- CompilerParameters.cs
- DataGridBoolColumn.cs
- FormsIdentity.cs
- ListComponentEditor.cs
- MemoryPressure.cs
- HttpInputStream.cs
- CounterSampleCalculator.cs
- ObjectQuery_EntitySqlExtensions.cs
- GenericPrincipal.cs
- AstTree.cs
- CollectionView.cs
- ReadOnlyCollectionBase.cs
- EarlyBoundInfo.cs
- ListViewGroup.cs
- SelectionProcessor.cs
- ColumnHeaderConverter.cs
- Vector3DValueSerializer.cs
- AutomationProperties.cs
- Substitution.cs
- TabletCollection.cs
- EventArgs.cs
- MatrixConverter.cs
- EventLogEntry.cs
- COM2FontConverter.cs
- ContractMapping.cs
- AttributeData.cs
- TripleDES.cs
- ToolStripOverflowButton.cs
- NullableDecimalAverageAggregationOperator.cs
- XmlSchemaExporter.cs
- NavigatingCancelEventArgs.cs
- ProjectionAnalyzer.cs
- DockAndAnchorLayout.cs
- CollectionViewSource.cs
- TreeNodeCollectionEditorDialog.cs
- ByteStreamMessageEncoderFactory.cs