Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Windows / Input / TraversalRequest.cs / 1 / TraversalRequest.cs
using System; namespace System.Windows.Input { ////// Represents a request to an element to move focus to another control. /// [Serializable()] public class TraversalRequest { ////// Constructor that requests passing FocusNavigationDirection /// /// Type of focus traversal to perform public TraversalRequest(FocusNavigationDirection focusNavigationDirection) { if (focusNavigationDirection != FocusNavigationDirection.Next && focusNavigationDirection != FocusNavigationDirection.Previous && focusNavigationDirection != FocusNavigationDirection.First && focusNavigationDirection != FocusNavigationDirection.Last && focusNavigationDirection != FocusNavigationDirection.Left && focusNavigationDirection != FocusNavigationDirection.Right && focusNavigationDirection != FocusNavigationDirection.Up && focusNavigationDirection != FocusNavigationDirection.Down) { throw new System.ComponentModel.InvalidEnumArgumentException("focusNavigationDirection", (int)focusNavigationDirection, typeof(FocusNavigationDirection)); } _focusNavigationDirection = focusNavigationDirection; } ////// true if reached the end of child elements that should have focus /// public bool Wrapped { get{return _wrapped;} set{_wrapped = value;} } ////// Determine how to move the focus /// public FocusNavigationDirection FocusNavigationDirection { get { return _focusNavigationDirection; } } private bool _wrapped; private FocusNavigationDirection _focusNavigationDirection; } ////// Determine how to move the focus /// public enum FocusNavigationDirection { ////// Move the focus to the next Control in Tab order. /// Next, ////// Move the focus to the previous Control in Tab order. Shift+Tab /// Previous, ////// Move the focus to the first Control in Tab order inside the subtree. /// First, ////// Move the focus to the last Control in Tab order inside the subtree. /// Last, ////// Move the focus to the left. /// Left, ////// Move the focus to the right. /// Right, ////// Move the focus to the up. /// Up, ////// Move the focus to the down. /// Down, // If you add a new value you should also add a validation check to TraversalRequest constructor } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows.Input { ////// Represents a request to an element to move focus to another control. /// [Serializable()] public class TraversalRequest { ////// Constructor that requests passing FocusNavigationDirection /// /// Type of focus traversal to perform public TraversalRequest(FocusNavigationDirection focusNavigationDirection) { if (focusNavigationDirection != FocusNavigationDirection.Next && focusNavigationDirection != FocusNavigationDirection.Previous && focusNavigationDirection != FocusNavigationDirection.First && focusNavigationDirection != FocusNavigationDirection.Last && focusNavigationDirection != FocusNavigationDirection.Left && focusNavigationDirection != FocusNavigationDirection.Right && focusNavigationDirection != FocusNavigationDirection.Up && focusNavigationDirection != FocusNavigationDirection.Down) { throw new System.ComponentModel.InvalidEnumArgumentException("focusNavigationDirection", (int)focusNavigationDirection, typeof(FocusNavigationDirection)); } _focusNavigationDirection = focusNavigationDirection; } ////// true if reached the end of child elements that should have focus /// public bool Wrapped { get{return _wrapped;} set{_wrapped = value;} } ////// Determine how to move the focus /// public FocusNavigationDirection FocusNavigationDirection { get { return _focusNavigationDirection; } } private bool _wrapped; private FocusNavigationDirection _focusNavigationDirection; } ////// Determine how to move the focus /// public enum FocusNavigationDirection { ////// Move the focus to the next Control in Tab order. /// Next, ////// Move the focus to the previous Control in Tab order. Shift+Tab /// Previous, ////// Move the focus to the first Control in Tab order inside the subtree. /// First, ////// Move the focus to the last Control in Tab order inside the subtree. /// Last, ////// Move the focus to the left. /// Left, ////// Move the focus to the right. /// Right, ////// Move the focus to the up. /// Up, ////// Move the focus to the down. /// Down, // If you add a new value you should also add a validation check to TraversalRequest constructor } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
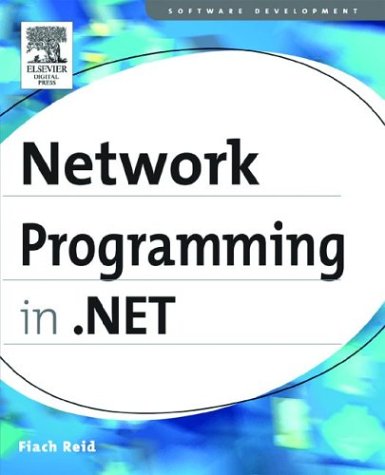
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientScriptManagerWrapper.cs
- X509CertificateValidator.cs
- DeclaredTypeValidatorAttribute.cs
- propertyentry.cs
- ListDictionary.cs
- ToolStripItemRenderEventArgs.cs
- ToolStripStatusLabel.cs
- XMLUtil.cs
- XmlValidatingReader.cs
- SqlGenerator.cs
- ImageClickEventArgs.cs
- DbException.cs
- Base64Encoder.cs
- TemplateBindingExpression.cs
- AspCompat.cs
- TreeNodeMouseHoverEvent.cs
- StringOutput.cs
- EntityDataSourceDataSelection.cs
- RegistrySecurity.cs
- XmlDataCollection.cs
- ObjectDataSourceWizardForm.cs
- SignalGate.cs
- InputLanguageManager.cs
- UIHelper.cs
- StylusButtonCollection.cs
- ProgressBar.cs
- DbReferenceCollection.cs
- XsdDataContractImporter.cs
- GacUtil.cs
- InputScopeAttribute.cs
- ChoiceConverter.cs
- counter.cs
- PersonalizationState.cs
- HttpResponse.cs
- Adorner.cs
- IfJoinedCondition.cs
- FixedTextBuilder.cs
- EntityConnectionStringBuilder.cs
- ToolbarAUtomationPeer.cs
- InvalidPrinterException.cs
- FormParameter.cs
- DataGridViewSelectedRowCollection.cs
- DependencyPropertyValueSerializer.cs
- UserNameSecurityToken.cs
- bindurihelper.cs
- Point.cs
- UnauthorizedWebPart.cs
- Transform3DGroup.cs
- StrokeNodeOperations.cs
- WmlObjectListAdapter.cs
- TextTrailingCharacterEllipsis.cs
- XmlSchemaImporter.cs
- Matrix3D.cs
- XmlSchemaSimpleContent.cs
- UIElement3D.cs
- SoapAttributeAttribute.cs
- GeneralTransform.cs
- RadioButtonPopupAdapter.cs
- CreateUserWizard.cs
- PropertyStore.cs
- BinarySerializer.cs
- SEHException.cs
- ConfigurationValue.cs
- SqlRowUpdatedEvent.cs
- CompositeTypefaceMetrics.cs
- TemplateBuilder.cs
- DBBindings.cs
- ApplicationFileParser.cs
- DataGrid.cs
- LeaseManager.cs
- InvokeMethodActivityDesigner.cs
- MetadataCollection.cs
- PtsContext.cs
- IconHelper.cs
- FilteredAttributeCollection.cs
- TranslateTransform.cs
- PermissionSet.cs
- SocketManager.cs
- BoolExpression.cs
- InfoCardBinaryReader.cs
- MethodBody.cs
- ArrayElementGridEntry.cs
- ClientUrlResolverWrapper.cs
- CanonicalFontFamilyReference.cs
- StylusButton.cs
- StatusBarPanelClickEvent.cs
- AnnouncementEventArgs.cs
- WindowsComboBox.cs
- Input.cs
- Region.cs
- EDesignUtil.cs
- CodeDefaultValueExpression.cs
- ParameterReplacerVisitor.cs
- DiagnosticsConfigurationHandler.cs
- PropertyEmitter.cs
- HttpConfigurationContext.cs
- PointAnimationBase.cs
- IdnMapping.cs
- VirtualPath.cs
- DebuggerAttributes.cs